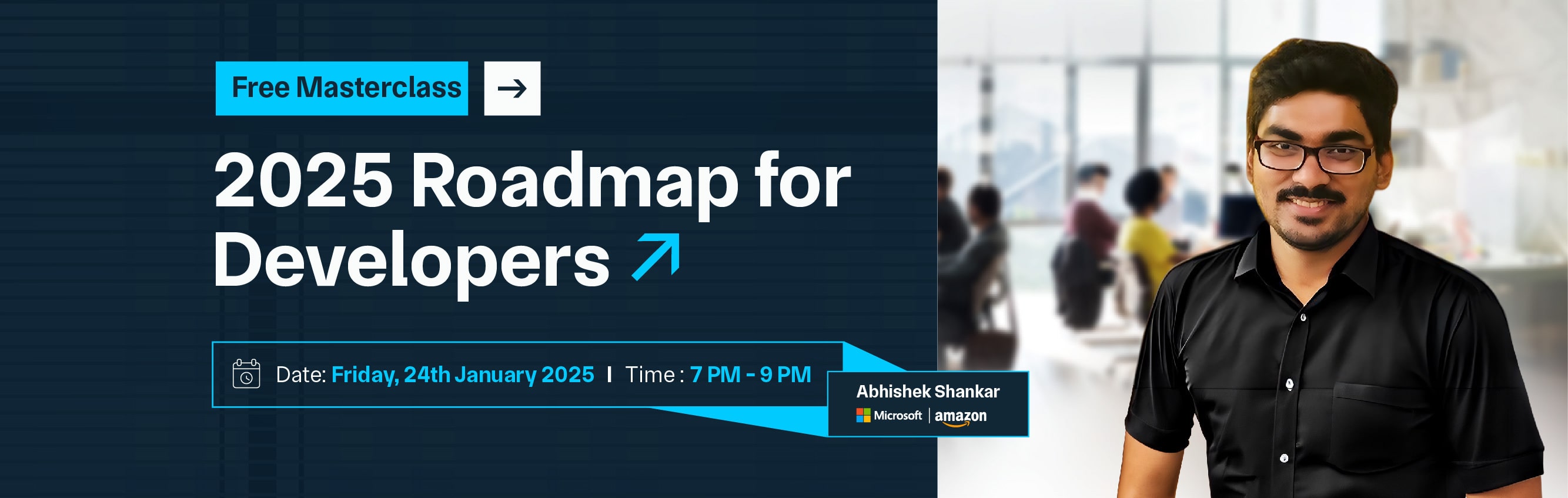

The yield keyword converts a normal function to a generator type of function. Used in generator functions to return the values in sequence when requested for values using the next() function. The yield statement returns a generator object to the one who calls the function that contains yield instead of simply returning a value.
def gen_func(x):
for i in range(x):
yield i
Functions benefit from the yield keyword during generator operations to provision multiple value sequences into sequence through function execution. The function cannot erase the original values stored in local variables. The execution begins at the yield expression when any function is invoked. Generator functions are functions that include the yield keyword in their code.
Generator function creation requires the use of yield keyword syntax. The generator function operates with an iterator structure that maintains memory efficiency while functioning like object iterators. The yield keyword allows the function execution to continue until reaching the end, unlike the return keyword, which terminates function processing.
Yield operates as a Python feature in generator functions to provide sequential value retrieval through lazy evaluation methods. The function pauses at yield statements while preserving its current state and then pauses before each subsequent value return. The function pauses at its previous yield statement before returning each successive value that gets requested.
def simple_generator():
yield 1
yield 2
yield 3
gen = simple_generator()
for value in gen:
print(value)
1
2
3
The yield keyword is used to make a function generator. It allows to return of a value in sequence. Instead of computing all values at once, it returns in sequence.
Yield is a function that generates values one at a time when requested, saves its state, and acts as an iterable behavior, allowing iteration over variables and code positions. Produces values lazily: The function does not return the final result immediately.
Here’s how yield works:
def count_up_to(n):
count = 1
while count <= n:
yield count # Yield the current value of the count
count += 1 # Increment count
# Using the generator function
counter = count_up_to(3)
for number in counter:
print(number)
1
2
3
Python uses both the yield and return send values from a function. A key difference in both is the use cases we see in the following table.
A generator function helps developers to produce a sequence of values via yield statements instead of executing entire values simultaneously. The generator function achieves exceptional results for processing extensive data volumes.
def countdown(n):
while n > 0:
yield n # Yield the current value of n
n -= 1 # Decrease n by 1
print("Done!")
# Create a generator object
counter = countdown(5)
# Iterate through the generator
for num in counter:
print(num)
5
4
3
2
1
Done!
The following example demonstrates the utility of generator functions together with the yield keyword.
A generator function needs creation to produce the first n square numbers via the yield protocol.
def generate_squares(n):
for i in range(1, n + 1):
yield i * i # Yield the square of each number from 1 to n
# Create a generator object
squares_generator = generate_squares(5)
# Iterate through the generator to print the squares
for square in squares_generator:
print(square)
1
4
9
16
25
In Python, you can use a generator function to create an infinite sequence of values. Since generators yield values one by one and do not store the entire sequence in memory, they are perfect for creating infinite sequences. Let's create an example that generates an infinite sequence of natural numbers starting from 1.
def infinite_counter():
count = 1
while True:
yield count # Yield the current count, then increment
count += 1 # Increment the counter
# Create a generator object
counter = infinite_counter()
# Print the first 10 numbers from the infinite sequence
for i in range(10):
print(next(counter))
1
2
3
4
5
6
7
8
9
10
In this example, let's use the yield keyword to generate a filtered list of even numbers from a list of integers.
Given a list of numbers, we want to use a generator function to yield only the even numbers from that list one by one.
def even_numbers(numbers):
for num in numbers:
if num % 2 == 0: # Check if the number is even
yield num # Yield the even number
# List of numbers
numbers_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Create a generator object
even_gen = even_numbers(numbers_list)
# Iterate through the generator to print even numbers
for even in even_gen:
print(even)
2
4
6
8
10
In this example, we’ll use the yield keyword in a generator function to generate Boolean values based on some condition, such as checking if numbers in a range are divisible by a given number.
Create a generator function that checks if numbers in a given range are divisible by a specified divisor and yields True or False accordingly.
def is_divisible_by(numbers, divisor):
for num in numbers:
if num % divisor == 0:
yield True # Yield True if the number is divisible by the divisor
else:
yield False # Yield False if the number is not divisible by the divisor
# List of numbers to check
numbers_list = [10, 15, 20, 25, 30, 35, 40]
# Divisor
divisor = 5
# Create a generator object
divisibility_gen = is_divisible_by(numbers_list, divisor)
# Iterate through the generator and print the Boolean results
for result in divisibility_gen:
print(result)
True
True
True
False
True
False
True
A generator function creates lazy value sequences using the yield keyword while preserving its state and execution point until a request for the next value appears. A defined generator function operates in a manner distinct from traditional functions because of its yield keyword.
A generator function remains inactive during its initial execution call. The generator function produces an iterator object, also known as a generator object, which functions as an object that can generate values upon request. The function remains stopped at this stage before starting its execution.
The generator values need to be retrieved by moving through the generator object. There exist two methods to execute this operation.
The generator function stops running whenever it reaches the yield instruction in both situations. The function stops its execution to let the caller receive the yielded value. After its suspension by a call from either a loop or next(), the generator function continues execution from the position it stopped at the last yield.
A generator function allows resuming execution by using the syntax Yield every time a new value is requested.
An exception known as StopIteration alerts the program that the end of iteration has arrived since no additional values await generation. A generator ends its operation after it exhausts all yield statements in the definition.
The ability of generators to operate lazily provides significant advantages for working with enormous datasets or continuous streams of data, which avoids complete memory usage. The value generation process progresses in a one-at-a-time manner.
Use yield is good in some scenarios. Let's see when and why to use the yield keyword.
yield produces memory-efficient value generation that lets programmers manage big datasets along with infinite sequences while minimally using system memory.
The yield keyword both makes stateful iteration easier to implement and delivers better performance through postponed computational tasks. The code maintains both efficiency and cleanness because of yield functionality.
The debugging process may become more complex because the yield functionality pauses and resumes execution during computation.
Functions working with a complete dataset find yield incompatible, while new programmers face challenges in understanding its behavior. The process to access values through iteration might not suit every situation since it raises compatibility issues.
The Yield keyword in Python enables efficient on-demand value production, which keeps memory utilization low and boosts system performance, mostly when working with extensive data collections or endless sequences. The yield keyword simplifies stateful iteration but produces cleaner code while it makes debugging harder and requires compatibility testing for various functions.
Copy and paste below code to page Head section
A Python generator function requires yield as its main keyword for creation. From within the function, execution pauses when yield produces values sequentially so the function can recover from the point where it is suspended when asked to return.
In comparison to the return's exit method, yield pauses the function execution for a value generation that lets the function continue from its last suspension point during value requests. This enables lazy evaluation.
Yield serves memory-efficient data handling of big datasets and endless sequences as well as data processing through incremental steps.
The definition of a generator function includes at least one yield syntax within its execution. Using yield allows the function to return a generator object with automatic lazy value generation upon loop iteration.
Generator functions hold the capacity to provide sequential values to their users. The function pauses when it encounters yield statements to return their values. A generator resumes its operation from the exact point of interruption when getting new value requests.
A generator works successfully within the structure of a for loop. A for loop carries out automatic next() function calls to the generator until it depletes all available values.