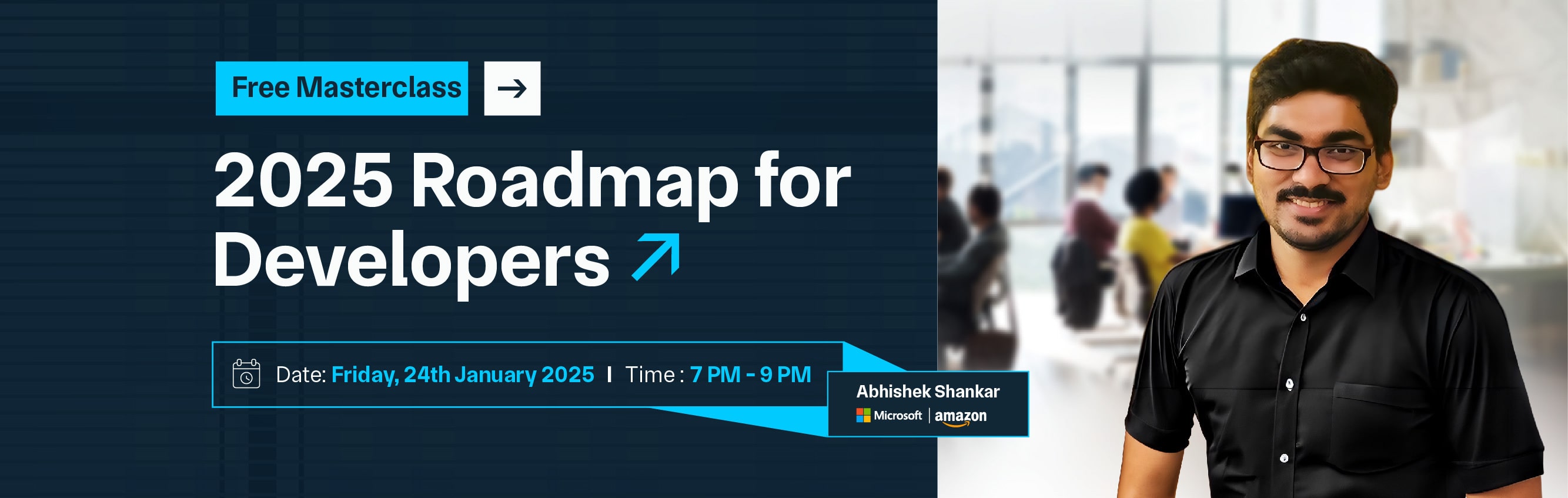

In React, the DOM (Document Object Model) refers to the structure of the web page that React manipulates to display content. However, React doesn’t directly interact with the traditional browser DOM; instead, it uses a concept called the Virtual DOM. The Virtual DOM is a lightweight, in-memory representation of the actual DOM. When a component's state or props change, React creates a new Virtual DOM tree and compares it with the previous one using an algorithm known as reconciliation.
This process helps React identify what parts of the real DOM need to be updated, minimizing the number of changes and improving performance. Instead of updating the entire page, React only updates the specific elements that have changed, resulting in faster rendering and a smoother user experience. The updates are then efficiently applied to the real DOM, which in turn reflects these changes on the webpage.
The use of the Virtual DOM is one of the key features that make React highly efficient and performant, especially for dynamic web applications where frequent updates to the UI are required. It abstracts the complexities of DOM manipulation, allowing developers to focus more on building components rather than worrying about optimizing direct DOM updates.
In React, DOM stands for the Document Object Model, which is a programming interface for web documents. The DOM represents the structure of a webpage as a tree of nodes, where each node corresponds to an element or part of the page, such as text, images, or buttons. However, React doesn't directly manipulate the real DOM (the browser's version of the DOM). Instead, React uses a concept called the Virtual DOM.
The Virtual DOM is a lightweight, in-memory copy of the real DOM. When a change occurs in the React application (for example, when a user interacts with a component), React updates the Virtual DOM first rather than the real DOM. React then compares the new Virtual DOM with the previous one using a process called reconciliation.
This allows React to figure out which parts of the real DOM need to be updated. Instead of re-rendering the entire page, React only updates the specific elements that have changed, making the process more efficient. This approach helps to improve the performance of React applications, especially for complex or dynamic interfaces that require frequent updates.
The Virtual DOM in React is an in-memory representation of the real DOM. It is a lightweight copy of the actual Document Object Model (DOM) used by browsers to represent the structure of a webpage. React uses the Virtual DOM to optimize rendering and update the user interface (UI) efficiently.
Here's how it works:
The Virtual DOM makes React fast and efficient by reducing the number of updates to the real DOM, which can be a slow process, especially with large or complex applications.
Here's a comparison between the DOM in Traditional JavaScript and React's Virtual DOM in a table format:
Re-rendering in web development refers to the process of updating the user interface (UI) whenever there is a change in state, props, or data. While re-rendering is necessary to reflect changes to the user interface, it can significantly impact performance, especially in applications that frequently update the UI or have complex structures. Here's how re-rendering impacts performance and the challenges it introduces:
Re-rendering in traditional JavaScript directly updates the real DOM. Each time a change occurs whether it’s due to user interaction or state changes the browser recalculates the layout, reflows elements, and repaints the page. This can be particularly slow if the application has complex UI elements or numerous DOM nodes.
In applications where frequent re-renders happen, this direct manipulation of the DOM can lead to performance degradation and slower user experiences, as every small change triggers costly layout recalculations.
Re-rendering can create significant performance bottlenecks, especially when the changes involve many components or large datasets. In traditional JavaScript applications, the browser is responsible for updating the entire page when a change occurs, which can be inefficient for complex UIs.
The more components that re-render, the greater the strain on the browser’s rendering engine, which can lead to noticeable lag or delays in the UI. Optimizing how and when re-renders happen is crucial to avoiding performance issues in larger or more interactive applications.
When a DOM element changes in size, position, or visibility, the browser may need to perform a reflow and repaint. A reflow occurs when the browser recalculates the layout, while a repaint adjusts the visual styles, such as colors and visibility. Both operations are expensive in terms of performance, particularly if multiple elements are affected by each change.
If re-renders frequently trigger these processes, the application can become sluggish, especially on low-powered devices or browsers with less optimization. This is why optimizing re-renders to avoid triggering unnecessary reflows and repaints is critical for maintaining smooth performance.
In modern frameworks like React, state changes trigger re-renders of components to reflect updated data. However, if state updates happen too frequently or if components have large, complex trees, the UI may re-render more often than necessary. This can result in unnecessary computational overhead and cause performance problems in applications where the state is changing rapidly.
While React’s reconciliation algorithm helps optimize this process by diffing the Virtual DOM, developers must still be mindful of how often re-renders happen, especially for high-performance applications like dashboards or real-time systems.
React introduces the concept of the Virtual DOM to optimize the re-rendering process. When state or props change, React first updates a lightweight in-memory copy of the DOM, which is much faster to manipulate than the real DOM. React then compares the new Virtual DOM with the previous version and calculates the minimum number of changes required to update the real DOM.
This process, known as reconciliation, reduces the amount of direct manipulation needed, improving performance by avoiding unnecessary DOM updates and keeping the application responsive even with frequent changes.
To improve performance, React provides tools and techniques to avoid unnecessary re-renders. For example, React. A memo can be used to prevent functional components from re-rendering unless their props have changed. Similarly, the shouldComponentUpdate lifecycle method in class components allows developers to decide when a component should re-render.
Using these optimization techniques helps reduce the computational load, ensuring that only components that truly need to update will do so. This is especially important in large applications where even small performance improvements can significantly enhance the overall user experience.
Frequent re-renders that negatively impact performance can lead to a poor user experience. If the UI feels sluggish or unresponsive, users may become frustrated, leading to decreased engagement with the application. This is particularly noticeable in interactions like form submissions, scrolling, or real-time updates, where a delay or visual stutter can be irritating.
To ensure a smooth user experience, it’s essential to optimize rendering behavior and minimize unnecessary updates to the UI, providing fast feedback and interactions with minimal delay.
The Virtual DOM and the Real DOM are both fundamental concepts in web development, especially when working with libraries like React. Below is a detailed comparison between the two:
Here’s a comparison table between React Virtual DOM and Shadow DOM:
In React, state management and DOM rendering are key concepts that help create dynamic, interactive user interfaces. Here's how React manages state and renders components to the DOM:
In React, state refers to the internal data of a component that can change over time. It determines how a component behaves and is typically used to store information that changes in response to user interactions or events.
Each component in React can manage its state, and when the state changes, React automatically triggers a re-render of the component to reflect the updated state.
import React, { Component } from 'react';
Class Counter extends Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
export default Counter;
In this example:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
When React needs to update the UI in response to state or prop changes, it uses an efficient mechanism involving the Virtual DOM.
Instead of updating the real DOM directly, React first makes changes to an in-memory representation of the DOM (the Virtual DOM), compares it to the previous version, and then applies the minimal set of changes to the real DOM.
When a React app starts, it generates the Virtual DOM based on the initial state and JSX of the components. This Virtual DOM is then compared to the real DOM, and React efficiently updates the real DOM to match the changes.
When state or props change, React creates a new Virtual DOM tree. It then compares this updated Virtual DOM with the previous version using an algorithm called reconciliation.
React identifies the differences (called diffing), and only the parts of the real DOM that need to change are updated. This reduces unnecessary updates and makes the UI faster and more efficient.
Let's look at how React renders a simple counter:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1); // triggers re-render with updated state
};
return (
<div>
<p>Count: {count}</p> {/* React updates this part of the DOM */}
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
React’s use of the Virtual DOM, combined with efficient state management, ensures that re-renders happen in a way that minimizes performance bottlenecks. However, frequent updates to state or props can still negatively impact performance if not managed properly.
React batches multiple state updates together, meaning it processes several state changes in a single render cycle. This is important because it reduces the number of re-renders and optimizes performance.
To avoid unnecessary re-renders, React provides tools like PureComponent (for class components) and React. Memo (for functional components). These tools prevent a component from re-rendering unless its props or state have changed.
import React, { memo } from 'react';
const CounterDisplay = memo(({ count }) => {
console.log('Rendering CounterDisplay');
return <p>Count: {count}</p>;
});
function Counter() {
const [count, setCount] = useState(0);
const increment = () => setCount(count + 1);
return (
<div>
<CounterDisplay count={count} />
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
In React, props are read-only data passed from parent components to child components. Props allow data to flow from parent to child, and when props change, the child component will re-render to reflect the updated data.
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
function App() {
const [name, setName] = useState('John');
return (
<div>
<Greeting name={name} />
<button onClick={() => setName('Jane')}>Change Name</button>
</div>
);
}
This illustrates how changes to props can trigger re-renders in child components and how React ensures that the UI reflects the latest state or props.
React’s Diffing Algorithm is a mechanism that optimizes how React updates the DOM by comparing the new Virtual DOM with the previous version and determining the minimal changes needed. This process helps React render updates efficiently without re-rendering the entire UI, improving performance.
This approach makes React fast by minimizing direct manipulation of the real DOM, allowing for faster updates and smoother user experiences.
In React, the Real DOM is the actual DOM used by the browser to display the user interface. Direct manipulation of the Real DOM can be slow and inefficient, especially for large applications with frequent updates. React solves this problem by using a Virtual DOM, which is a lightweight, in-memory representation of the Real DOM. The Virtual DOM allows React to optimize updates and only apply necessary changes to the Real DOM rather than re-rendering everything from scratch. When an update occurs (for example, when state or props change), React first updates the Virtual DOM. After that, it compares the new Virtual DOM with the previous version using a process called diffing.
The Diffing Algorithm identifies the differences between the two Virtual DOM trees and calculates the minimal set of changes required to update the Real DOM. Once the differences are identified, React applies only those specific updates to the Real DOM rather than re-rendering the entire UI. This approach significantly reduces the number of operations performed on the Real DOM, leading to faster rendering and improved performance. React's use of batching updates further enhances efficiency.
Instead of applying each change immediately, React groups multiple state updates and processes them in a single render cycle, reducing unnecessary re-renders. Additionally, component reusability ensures that if a component's state or props have not changed, it is not re-rendered, which further minimizes unnecessary updates to the Real DOM.By using the Virtual DOM and efficient update strategies, React ensures that only the parts of the UI that need to change are updated, resulting in better performance and a smoother user experience.
React is designed to optimize the way web applications interact with the browser's rendering cycle, ensuring smooth performance even when there are frequent updates. The browser's rendering cycle involves a series of steps that include parsing HTML, applying styles, computing layout, and painting the content on the screen.
React helps to make this process more efficient by minimizing unnecessary operations on the DOM and by carefully controlling when and how changes are applied to the UI.
Here’s how React fits into the browser’s rendering cycle:
When a React application is first loaded, the initial render begins. React uses JSX (a JavaScript syntax extension) to define the structure of the UI. It then creates a Virtual DOM, a lightweight in-memory representation of the UI, which is compared with the Real DOM to determine the minimal set of changes needed.
React's diffing algorithm compares the new Virtual DOM with the previous version to identify what has changed. It then updates the Real DOM only with the minimal necessary changes, avoiding costly reflows and repaints and improving performance.
When there’s a change in state or props (which could happen through user interaction, API calls, etc.), React re-renders the affected components. However, React doesn’t immediately apply these updates to the Real DOM.
Instead, it:
This process is crucial in preventing unnecessary browser reflows, which can be very slow.
When the Real DOM is updated, the browser must go through a process called reflow and repaint:
These steps are resource-intensive. React minimizes their impact by updating only the parts of the DOM that have changed, thus avoiding full-page reflows and repaints whenever possible.
For example, if only the text in a <div> changes, React will only update the text in the Real DOM, not the entire structure or layout. This ensures better performance, especially in dynamic applications with frequent updates.
JavaScript operates in a single-threaded environment via the event loop, meaning that only one operation can be performed at a time. When an event (like a click or form submission) triggers a state change in React, React queues an update and re-renders the component. React batches these updates and schedules them for processing in the next event loop cycle.
React makes use of asynchronous rendering and batched updates to ensure that multiple state changes are handled together instead of triggering a re-render after every change. This optimizes performance by reducing the number of reflows and repaints triggered by the browser.
To ensure efficient rendering, React utilizes the Virtual DOM and diffing algorithm to minimize the number of updates made to the Real DOM. By calculating the minimum necessary changes and applying them in a single update, React helps to:
React provides a declarative way to manage and update the DOM. Instead of directly manipulating the DOM like in traditional JavaScript, React relies on its Virtual DOM to efficiently update the real DOM.
However, you may need to interact with the real DOM directly, for example, to integrate third-party libraries, manage focus, or handle animations. Here are some practical examples of working with the DOM in React:
In React, you can use refs to interact with DOM elements directly. A ref provides a way to access a DOM node or React component instance directly, allowing you to read values, manage focus, or trigger DOM methods like scrollIntoView().
In this example, we'll create an input field and a button that focuses on the input when clicked.
import React, { useRef } from 'react';
function FocusInput() {
// Create a ref for the input element
const inputRef = useRef(null);
const focusInput = () => {
// Access the DOM node and set focus
inputRef.current.focus();
};
return (
<div>
<input ref={inputRef} type="text" placeholder="Click the button to focus" />
<button onClick={focusInput}>Focus the Input</button>
</div>
);
}
export default FocusInput;
React has its way of handling events, which is slightly different from how it’s done in traditional JavaScript. In React, event handlers are passed as props and are synthetic events that normalize across browsers.
In this example, we handle a click event to update a counter.
import React, { useState } from 'react';
function ClickCounter() {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(count + 1);
};
return (
<div>
<button onClick={handleClick}>Clicked {count} times</button>
</div>
);
}
export default ClickCounter;
React provides a prop called dangerouslySetInnerHTML for directly inserting HTML content into a component. This can be useful when you need to insert raw HTML from a string (like from an API response). However, use this feature carefully, as it can expose your application to XSS (Cross-Site Scripting) vulnerabilities.
import React from 'react';
function RawHtmlRenderer() {
const rawHtml = "<h1>This is <em>dangerous</em> HTML content</h1>";
return (
<div dangerouslySetInnerHTML={{ __html: rawHtml }} />
);
}
export default RawHtmlRenderer;
You might need to manipulate DOM elements to add animations. While React itself doesn’t directly handle animations, you can use libraries like React Transition Group or Framer Motion for smooth animations in your components. For basic DOM manipulations, you can also use JavaScript and CSS.
Here’s an example of animating an element’s opacity when it’s displayed:
import React, { useState, useEffect } from 'react';
import './FadeIn.css'; // A CSS file for animations
function FadeInComponent() {
const [show, setShow] = useState(false);
useEffect(() => {
// Start animation after 1 second
const timer = setTimeout(() => {
setShow(true);
}, 1000);
return () => clearTimeout(timer);
}, []);
return (
<div className={`fade-in ${show ? 'visible' : ''}`}>
Hello, I'm fading in!
</div>
);
}
export default FadeInComponent;
CSS (FadeIn.css):
.fade-in {
opacity: 0;
transition: opacity 1s ease;
}
.fade-in.visible {
opacity: 1;
}
React allows you to apply styles to DOM elements based on component state dynamically. You can achieve this by directly manipulating the style attribute or by conditionally applying CSS class names.
import React, { useState } from 'react';
function ColorChanger() {
const [color, setColor] = useState('blue');
const changeColor = () => {
setColor(color === 'blue' ? 'green': 'blue');
};
return (
<div>
<button onClick={changeColor}>Change Background Color</button>
<div style={{ backgroundColor: color, height: '100px', width: '100px' }} />
</div>
);
}
export default ColorChanger;
Sometimes, you may need to integrate third-party libraries that require direct DOM manipulation (like jQuery plugins, charts, or custom animations). React allows you to use refs to interact with these libraries.
import React, { useEffect, useRef } from 'react';
Import $ from 'jquery';
function JQueryExample() {
const divRef = useRef(null);
useEffect(() => {
$(divRef.current).fadeIn(); // Using jQuery to fade in the div
}, []);
return <div ref={divRef} style={{ display: 'none' }}>This is a jQuery animated element</div>;
}
export default JQueryExample;
In React, the DOM refers to the structure of the web page, but React uses a Virtual DOM to manage updates efficiently. Instead of directly manipulating the real DOM, React compares the Virtual DOM with the real DOM and only applies changes where necessary, improving performance and responsiveness.
Copy and paste below code to page Head section
The DOM (Document Object Model) in React refers to the representation of the web page’s structure. React uses a Virtual DOM, which is a lightweight copy of the real DOM. It helps React efficiently update only the parts of the UI that need to change, improving performance.
The Virtual DOM is a concept in React where a virtual copy of the actual DOM is kept in memory. React compares the Virtual DOM with the real DOM and updates only the necessary elements rather than re-rendering the entire page. This results in faster updates and better performance.
React uses the Virtual DOM to detect changes in state or props. It then calculates the difference (diffing) between the Virtual DOM and the real DOM, updating only the affected parts of the real DOM, which minimizes re-renders and enhances performance.
React uses the Virtual DOM to optimize UI rendering. By comparing the current Virtual DOM with the previous one, React can minimize the number of updates to the real DOM, making the app faster and more efficient.
Real DOM: Direct manipulation of the web page’s structure, which can be slow when frequent changes are made. Virtual DOM: A lightweight, in-memory copy of the DOM used by React to optimize updates by minimizing direct manipulations of the real DOM.
While React encourages using state and props to update the UI, you can still manipulate the real DOM directly using refs. Refs allow direct access to DOM elements when necessary, such as focusing an input or integrating with third-party libraries.