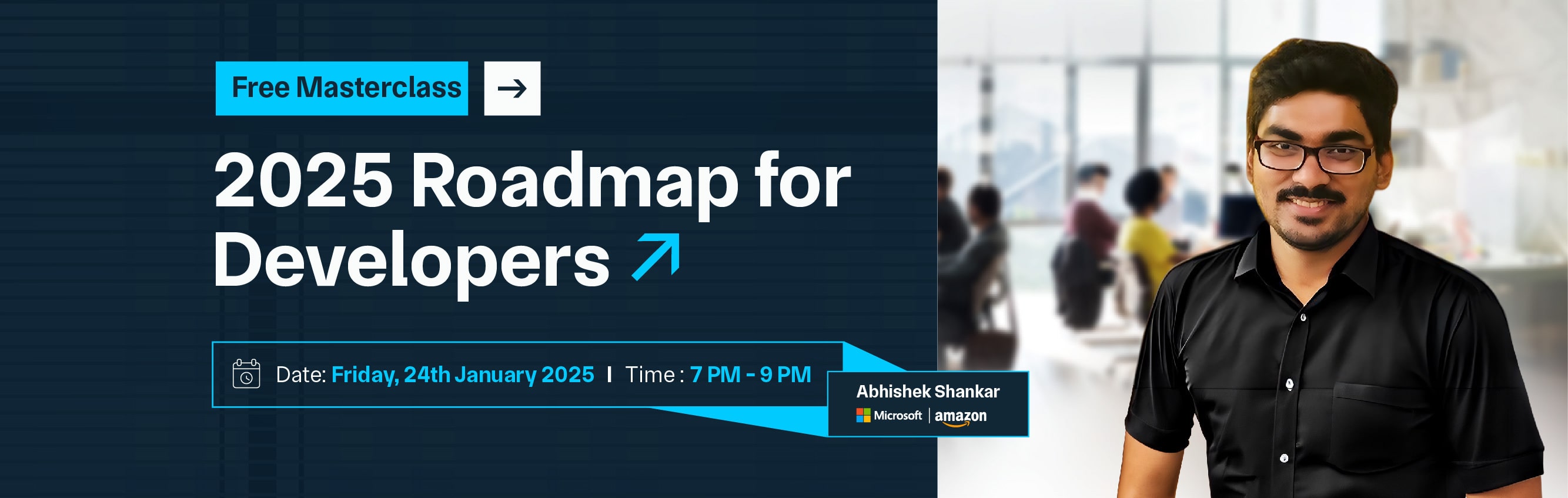

In React, a component is a core building block for creating user interfaces. It allows developers to break down complex UIs into smaller, manageable pieces, each encapsulating its structure, behavior, and styles. Components in React come in two main types: functional and class components. Functional components are simpler and defined as JavaScript functions that accept props (input data) and return React elements, which describe what should appear on the screen.
Class components, on the other hand, are ES6 classes that extend React.Component and include a render method to output elements. They also support state management and lifecycle methods, which are useful for handling data changes and executing code at various stages of a component's lifecycle, such as mounting, updating, or unmounting.
Components use props to pass data from parent to child components, making them reusable across different parts of the application. State within a component allows it to manage local data that can change over time, enabling dynamic and interactive UIs. By breaking down UIs into components, React promotes modularity and code reusability, leading to cleaner, more maintainable code and easier debugging.
JSX (JavaScript XML) is a syntax extension for JavaScript used in React to describe what the user interface should look like. It allows developers to write HTML-like code within JavaScript files, making it easier to create and visualize React components. JSX combines the expressive power of JavaScript with the declarative nature of HTML, providing a more intuitive way to structure UI components.
HTML-Like Syntax: JSX looks similar to HTML, allowing developers to use familiar tags like < div >, < h1 >, and < button > directly within JavaScript code. This makes it easier to design and understand the structure of the UI.
const element = <h1>Hello, world!</h1>;
Embedded Expressions: JSX allows for embedding JavaScript expressions within curly braces {}. This enables dynamic content rendering based on component state or props.
const name = "Alice";
const element = <h1>Hello, {name}!</h1>;
Component Usage: JSX is used to create and render React components. You can use it to define the layout and structure of a component and include other components within it.
function Welcome(props) {
return <h1>Welcome, {props.name}!</h1>;
}
Attributes: JSX supports attributes similar to HTML attributes. However, JSX uses camelCase naming conventions for attribute names and supports passing JavaScript expressions as values.
const element = <img src={imageUrl} alt="Description" />;
Compilation: JSX is not natively understood by browsers. It needs to be transpired into standard JavaScript using tools like Babel before it can be executed. This compilation process converts JSX into React.createElement calls, which generate the corresponding React elements.
// JSX code
const element = <h1>Hello, world!</h1>;
// Compiled JavaScript code
const element = React.createElement('h1', null, 'Hello, world!');
JSX (JavaScript XML) is used in React to simplify the process of building user interfaces by combining the declarative nature of HTML with the full power of JavaScript. Here are the key reasons why JSX is used and its advantages:
JSX provides a more intuitive and readable syntax for defining the structure of UI components. Its HTML-like appearance makes it easier for developers to visualize and write the layout of a component directly within JavaScript code. This close resemblance to HTML reduces the cognitive load and makes the code more accessible and understandable.
JSX allows developers to describe what the UI should look like based on the current state and props of the components rather than focusing on how to update the UI. This declarative approach simplifies component design by abstracting away the low-level details of DOM manipulation and rendering.
JSX integrates seamlessly with JavaScript, enabling developers to embed JavaScript expressions and logic directly within the UI markup. This allows dynamic content generation and conditional rendering in a straightforward manner.
For example:
const name = "Alice";
const element = <h1>Hello, {name}!</h1>;
JSX makes it easy to compose complex UIs by combining smaller, reusable components. It allows developers to nest components and pass data between them using props, promoting modularity and reusability.
For instance:
function App() {
return (
<div>
<Header title="Welcome" />
<MainContent />
<Footer />
</div>
);
}
Modern development tools and editors support JSX, providing features like syntax highlighting, auto-completion, and error checking. This enhances the development experience by catching errors early and improving code quality.
JSX is compiled into standard JavaScript using tools like Babel. This compilation process transforms JSX into React.createElement calls, which React uses to create and manage virtual DOM elements efficiently. This allows JSX to be a syntactic sugar over JavaScript functions, optimizing rendering performance.
Using JSX helps maintain a clear separation of concerns by keeping the UI structure closely tied to the component logic. This improves maintainability by making the codebase easier to navigate and understand. Changes in UI structure can be made directly within the component code without needing to switch between HTML and JavaScript files.
In React, a component is a fundamental building block used to construct user interfaces. Components are reusable, self-contained pieces of code that define how a section of the user interface should appear and behave. They encapsulate the structure, logic, and style of parts of a UI, making it easier to build complex applications by breaking them into smaller, manageable parts.
1. Encapsulation: Components encapsulate their structure, behavior, and style. This means that a component manages its state, handles its events, and styles itself independently. This encapsulation promotes modularity and reusability.
2. Reusability: Once a component is created, it can be used multiple times throughout an application. Components can be nested within each other, allowing for the composition of complex UIs from simple, reusable parts.
3. Declarative: Components describe what the UI should look like based on the current state and props (properties). This declarative approach abstracts away the complexities of direct DOM manipulation, focusing on what the output should be rather than how to achieve it.
4. State and Props:
5. Lifecycle Methods (Class Components): In class components, lifecycle methods are special functions that get called at different stages of a component's lifecycle (e.g., when a component mounts, updates, or unmounts). These methods are useful for managing side effects and performing actions at specific points in a component's life.
6. Functional vs. Class Components:
In React, components are the building blocks of the UI. There are primarily two types of components: Functional Components and Class Components. Each type has its own use cases, features, and benefits. Here’s an overview of each:
Functional components are the simpler of the two types. They are JavaScript functions that accept props as arguments and return React elements. They are often used for presentational purposes where state and lifecycle methods are not required.
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
With Hooks:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Class components are ES6 classes that extend React.Component. They are more feature-rich and can be used when you need to manage a local state or use lifecycle methods. Class components were the primary way to handle state and side effects in React before Hooks were introduced.
class Greeting extends React.Component {
constructor(props) {
super(props);
this.state = { name: 'World' };
}
render() {
return <h1>Hello, {this.state.name}!</h1>;
}
}
class PureGreeting extends React.PureComponent {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
function withUser(Component) {
return class extends React.Component {
render() {
return <Component user="John Doe" {...this.props} />;
}
};
}
const UserProfile = (props) => <h1>{props.user}</h1>;
const EnhancedUserProfile = withUser(UserProfile);
function ControlledForm() {
const [value, setValue] = useState('');
const handleChange = (event) => {
setValue(event.target.value);
};
return <input type="text" value={value} onChange={handleChange} />;
}
Functional Components in React are a simpler way to define components as JavaScript functions. They allow developers to create UI elements without the complexity of class-based components. Here’s an overview of functional components, their features, and how they are used:
1. Simplicity:
2. Stateless:
3. Hooks:
Functional components are defined as JavaScript functions. Here’s a basic example:
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
With Hooks, functional components can manage local state and perform side effects:
import React, { useState, useEffect } from 'react';
function Counter() {
const [count, setCount] = useState(0); // useState Hook
useEffect(() => {
// Side effect: Update document title
document.title = `Count: ${count}`;
}, [count]); // Dependency array
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
1. Conciseness:
2. Performance:
3. Ease of Testing:
4. Hooks Integration:
5. Better Practice:
Class Components in React are a way to define components using ES6 classes. They offer a more feature-rich approach compared to functional components, especially before the introduction of Hooks. Class components can manage state and use lifecycle methods, making them suitable for more complex scenarios. Here’s an overview of class components, their features, and usage:
1. State Management:
2. Lifecycle Methods:
Class components have access to lifecycle methods that allow you to perform actions at different stages of a component’s lifecycle. These methods include:
3. Event Handling:
4. Render Method:
Class components are defined using ES6 classes. Here’s a basic example of a class component:
import React from 'react';
class Greeting extends React.Component {
constructor(props) {
super(props);
this.state = { name: 'World' };
}
handleChange = (event) => {
this.setState({ name: event.target.value });
};
render() {
return (
<div>
<h1>Hello, {this.state.name}!</h1>
<input type="text" value={this.state.name} onChange={this.handleChange} />
</div>
);
}
Here’s an example using lifecycle methods:
import React from 'react';
class DataFetcher extends React.Component {
constructor(props) {
super(props);
this.state = { data: null, loading: true };
}
componentDidMount() {
// Fetch data after component mounts
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => this.setState({ data, loading: false }));
}
render() {
const { data, loading } = this.state;
return (
<div>
{loading ? <p>Loading...</p> : <pre>{JSON.stringify(data, null, 2)}</pre>}
</div>
);
}
}
State and Lifecycle Management:
Method Binding:
Compatibility:
Legacy Codebases:
With the introduction of Hooks in React 16.8, many functionalities previously exclusive to class components are now available in functional components. This has led to a trend toward using functional components with Hooks for new development due to their simplicity and ease of use. However, class components are still widely used and supported in React.
Class components in React are a versatile way to define components and have been used extensively, especially before the introduction of Hooks. While functional components with Hooks have become more popular for many use cases, class components still offer valuable functionality and are particularly useful in various scenarios. Here are the key uses of class components:
Class components are ideal for managing local state, especially when the state needs to be complex or involves multiple variables. The state is maintained within the component and updated using this.setState(). This allows components to dynamically change their output based on user interactions or other events.
Example:
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
Class components offer lifecycle methods, which are functions that execute at specific points in a component’s life cycle. These methods are useful for performing side effects, such as data fetching, setting up subscriptions, or cleaning up resources.
Key Lifecycle Methods:
Example:
class DataFetcher extends React.Component {
constructor(props) {
super(props);
this.state = { data: null, loading: true };
}
componentDidMount() {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => this.setState({ data, loading: false }));
}
render() {
const { data, loading } = this.state;
return <div>{loading ? 'Loading...' : <pre>{JSON.stringify(data, null, 2)}</pre>}</div>;
}
}
Class components use methods for event handling, and you can define and bind event handlers within the class. This approach is particularly useful when you need to manage complex interactions and state updates triggered by user actions.
Example:
class Form extends React.Component {
constructor(props) {
super(props);
this.state = { value: '' };
}
handleChange = (event) => {
this.setState({ value: event.target.value });
};
handleSubmit = (event) => {
event.preventDefault();
alert('A name was submitted: ' + this.state.value);
};
render() {
return (
<form onSubmit={this.handleSubmit}>
<label>
Name:
<input type="text" value={this.state.value} onChange={this.handleChange} />
</label>
<button type="submit">Submit</button>
</form>
);
}
}
Class components support composition by nesting other components and passing data through props. This allows for creating complex UIs by combining smaller, reusable class components.
Example:
class Header extends React.Component {
render() {
return <header><h1>{this.props.title}</h1></header>;
}
}
class App extends React.Component {
render() {
return (
<div>
<Header title="Welcome to My App" />
<main>
<p>Content goes here.</p>
</main>
</div>
);
}
}
Class components are used to create error boundaries, which catch JavaScript errors anywhere in their child component tree and display a fallback UI instead of crashing the entire app. This feature is not directly available in functional components without using Hooks or higher-order components.
Example:
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError() {
// Update state to show fallback UI
return { hasError: true };
}
componentDidCatch(error, info) {
// Log error to an error reporting service
console.error(error, info);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
Rendering React Components involves converting React components into actual DOM elements for display on a web page. Here’s a brief overview of how it works:
This process ensures React applications are fast, efficient, and responsive.
In React, a constructor is a special method in class components that is used to initialize the component’s state and bind methods to the component instance. It is part of the ES6 class syntax, which React class components are based on. Here’s a detailed look at its role and usage:
1. Initialize State:
2. Bind Methods:
3. Setup Initial Values:
Here’s a basic example of a React class component using a constructor:
import React from 'react';
class MyComponent extends React.Component {
constructor(props) {
super(props); // Calls the constructor of the parent class (React.Component)
// Initialize state
this.state = {
count: 0
};
// Bind method to the class instance
this.handleClick = this.handleClick.bind(this);
}
// Method to handle button click
handleClick() {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.handleClick}>Increment</button>
</div>
);
}
}
export default MyComponent;
Composition in React refers to the practice of building complex UIs by combining smaller, reusable components. This approach allows you to create more manageable and maintainable code by breaking down the user interface into smaller, self-contained pieces.
Composition is a fundamental concept in React that promotes the use of components to encapsulate logic and rendering, making the codebase more modular and easier to understand.
1. Component Reusability:
2. Parent-Child Relationships:
3. Props and Children:
4. Container and Presentational Components:
1. Reusability:
2. Modularity:
3. Separation of Concerns:
4. Encapsulation:
5. Ease of Testing:
6. Improved Readability:
Components are the cornerstone of React’s architecture, offering a robust framework for building dynamic, efficient, and maintainable user interfaces. By embracing component-based design, developers can leverage the advantages of reusability, modularity, and encapsulation, leading to cleaner and more organized code. Components facilitate the separation of concerns, ensuring that logic and presentation are managed independently, which enhances code maintainability and readability.
The ability to dynamically compose and scale components allows for flexible and adaptive UIs, while React's lifecycle methods and hooks provide precise control over component behavior. Overall, the component-based approach in React not only streamlines development and testing but also promotes a scalable architecture that can grow with the application. This modular methodology aligns with best practices in modern web development, making React a powerful tool for creating sophisticated and interactive web applications.
Copy and paste below code to page Head section
A React component is a reusable, self-contained piece of UI that can be rendered to create a user interface. Components can be either class-based or functional and can manage their own state and lifecycle methods or hooks.
JSX (JavaScript XML) is a syntax extension for JavaScript that looks similar to HTML. It allows developers to write UI elements in a way that is both readable and expressive, and it gets compiled into JavaScript by React.
Props (short for properties) are read-only attributes used to pass data and event handlers from parent components to child components. They allow for the dynamic rendering of UI elements based on the data passed.
The render method in class components returns the JSX that defines what should be displayed on the screen. In functional components, this is done implicitly by returning JSX from the function.
Lifecycle methods are special methods in class components that allow you to hook into different stages of a component’s life, such as componentDidMount, componentDidUpdate, and componentWillUnmount. Functional components use hooks like useEffect to achieve similar functionality.
The Virtual DOM is a lightweight in-memory representation of the actual DOM. React uses it to optimize updates by comparing the current Virtual DOM with a new one and applying a minimal set of changes to the real DOM.