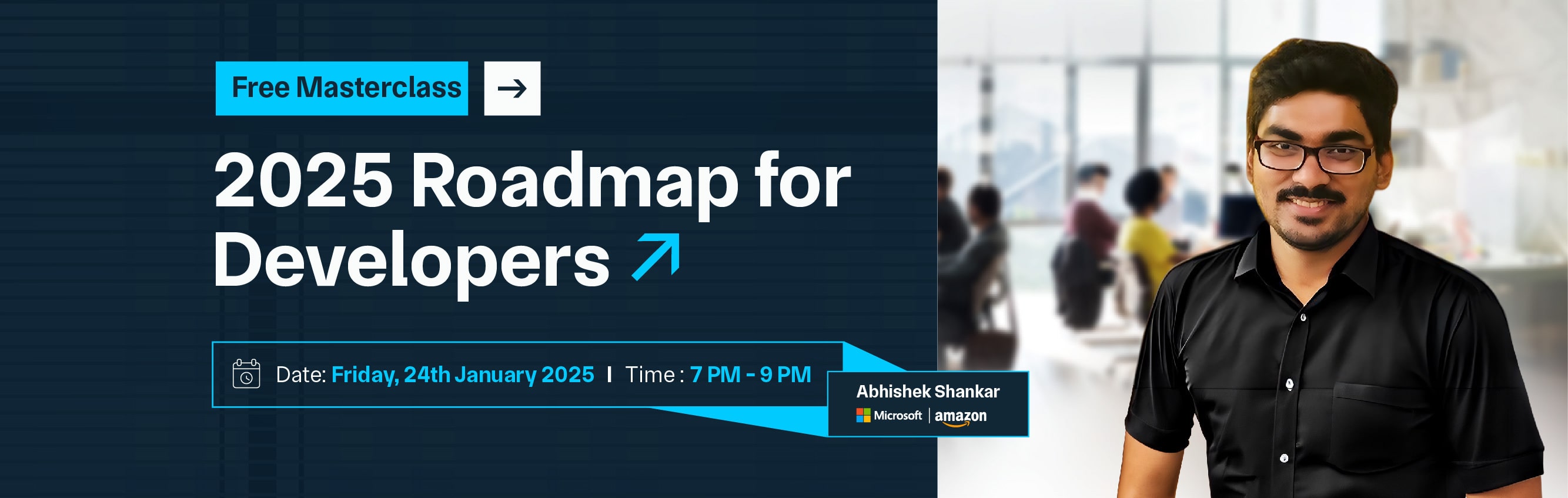

In Angular, a component is a fundamental building block of the application that encapsulates the HTML structure, logic, and styles required to define a part of the user interface. A component consists of three main parts: the template, the class, and the metadata. The template is the HTML view that outlines how the component will appear in the browser, and it often includes Angular directives like ngIf or ngFor to display data dynamically or control the flow of the view.
The class is written in TypeScript and contains the logic of the component, including properties and methods that control the behavior of the template, such as handling user interactions or fetching data from an API. The metadata is provided by the @Component decorator, which includes important information about the component, like its selector (the tag name used to embed the component in an HTML file), the associated template, and the styles.
Components in Angular are essential for building modular, reusable, and maintainable applications. They allow for clean separation of concerns by dividing an app into smaller, self-contained units. Components can communicate with one another through inputs and outputs, allowing for easy data flow between parent and child components, making Angular apps scalable and efficient to develop.
A component in Angular is a key building block of an Angular application. It is responsible for defining a part of the user interface and encapsulating the logic and styles associated with it. Each component in Angular has three core elements:
Components are modular and reusable, allowing developers to break down the user interface into smaller, manageable units. They can also communicate with each other through inputs and outputs, enabling easy data sharing between parent and child components. Components are central to building scalable and maintainable Angular applications.
Here’s an example of a simple Angular component:
To create a component in Angular, you can use the Angular CLI command:
Ng generates component my-component
This will create the necessary files for the component (HTML, CSS, TypeScript, and testing files).
Here’s an example of what each part of the component might look like:
import { Component } from '@angular/core';
@Component({
selector: 'app-my-component', // The selector to be used in HTML
templateUrl: './my-component.component.html', // Path to the HTML template
styleUrls: ['./my-component.component.css'] // Path to the CSS styles
})
export class MyComponent {
title: string = 'Hello, Angular!';
count: number = 0;
// Method to increment count
increment() {
this.count++;
}
// Method to reset count
reset() {
this.count = 0;
}
}
<div class="component-container">
<h1>{{ title }}</h1> <!-- Binding the title property -->
<p>Current count: {{ count }}</p> <!-- Binding the count property -->
<button (click)="increment()">Increment</button> <!-- Calling increment method -->
<button (click)="reset()">Reset</button> <!-- Calling reset method -->
</div>
.component-container {
padding: 20px;
background-color: #f0f0f0;
border-radius: 5px;
}
button {
margin: 5px;
padding: 10px 15px;
background-color: #007bff;
color: white;
border: none;
cursor: pointer;
}
button: hover {
background-color: #0056b3;
}
Once the component is created, you can use the component in the app by placing its selector tag (<app-my-component>) in the parent component’s template (e.g., app.component.html):
<!-- app.component.html -->
<app-my-component></app-my-component>
When the component is rendered in the browser:
This simple example demonstrates how Angular components can be used to structure your application, combining HTML, TypeScript, and CSS in a modular way.
An Angular component is made up of several key parts that work together to define its behavior, structure, and appearance. Below are the main parts of an Angular component:
The class in Angular is written in TypeScript and contains the logic for the component. This is where you define properties, methods, and lifecycle hooks that control the component’s behavior and interact with the view.
Example:
import { Component } from '@angular/core';
@Component({
selector: 'app-my-component,'
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css']
})
export class MyComponent {
title: string = 'Welcome to Angular!';
count: number = 0;
increment() {
this.count++;
}
reset() {
this.count = 0;
}
}
The template is an HTML file that defines the view or structure of the component. It controls how the component’s data and UI elements are displayed. Angular provides directives like ngIf, ngFor, and two-way data binding to update the view based on the component’s state dynamically.
Example:
<div class="component-container">
<h1>{{ title }}</h1> <!-- Binding title property from the class -->
<p>Current count: {{ count }}</p> <!-- Binding count property -->
<button (click)="increment()">Increment</button> <!-- Event binding for button click -->
<button (click)="reset()">Reset</button> <!-- Event binding for button click -->
</div>
The metadata is provided by the @Component decorator, which contains important information about the component. This includes:
The @Component decorator is crucial for Angular to understand how to treat the class as a component.
Example:
@Component({
selector: 'app-my-component', // Custom tag to embed the component
templateUrl: './my-component.component.html', // Path to the template file
styleUrls: ['./my-component.component.css'] // Path to the style file
})
The styles of an Angular component are written in a separate CSS or SCSS file. These styles apply only to the component, ensuring encapsulation. Angular automatically scopes styles to the component’s template, preventing style leaks and conflicts between components.
Example:
/* my-component.component.css */
.component-container {
padding: 20px;
background-color: #f0f0f0;
border-radius: 5px;
}
button {
margin: 5px;
padding: 10px 15px;
background-color: #007bff;
color: white;
border: none;
cursor: pointer;
}
button: hover {
background-color: #0056b3;
}
The selector is a unique tag used in the HTML to embed the component. It is defined in the @Component decorator and is used to insert the component into the parent component's template.
Example:
@Component({
selector: 'app-my-component', // Custom HTML tag to use the component
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css']
})
In the parent component’s HTML, the component is used as:
<app-my-component></app-my-component> <!-- Using the selector -->
The component lifecycle refers to the series of stages that a component goes through, from creation to destruction. Angular provides several lifecycle events (or hooks) that allow developers to tap into specific moments during the component's lifecycle to run custom logic.
These lifecycle hooks can be implemented in a component's class to handle tasks like initialization, change detection, or cleanup.
Here’s an overview of the main Angular component lifecycle hooks:
ngOnChanges(changes: SimpleChanges): void {
console.log('Changes detected:', changes);
}
ngOnInit(): void {
console.log('Component initialized!');
}
ngDoCheck(): void {
console.log('Change detection cycle triggered!');
}
ngAfterContentInit(): void {
console.log('Content projected into the component!');
}
ngAfterContentChecked(): void {
console.log('Projected content checked!');
}
ngAfterViewInit(): void {
console.log('Component view initialized!');
}
ngAfterViewChecked(): void {
console.log('Component view checked!');
}
ngOnDestroy(): void {
console.log('Component is about to be destroyed!');
}
In Angular, component communication refers to the ways in which components interact and share data or trigger actions. The framework provides multiple methods to achieve this communication, depending on whether components have a parent-child relationship or if they are unrelated.
The most common methods include using @Input() and @Output() properties for parent-child communication, and using services for communication between sibling components or across the app.
Additionally, services provide a way for non-hierarchical communication. A service is a singleton class that can be injected into multiple components. This allows different parts of the application to share data or trigger actions without needing to be directly related in a parent-child structure.
View encapsulation in Angular controls how the styles defined in a component's template affect the component and its child components. Angular supports three different modes of view encapsulation: Emulated, None, and Shadow DOM.
Each mode serves different use cases, and choosing the right encapsulation mode depends on whether you want to isolate styles to a component, apply them globally, or use native browser shadow DOM functionality.
Building clean, maintainable, and scalable Angular applications requires following best practices when working with components. These best practices help in enhancing code readability, reducing bugs, improving performance, and ensuring consistency across the application. Below are the key best practices for Angular components:
Each Angular component should be designed to do one thing and do it well. Avoid making components too large or handling multiple responsibilities. The single Responsibility Principle suggests that a component should focus on a specific task. This makes components easier to test, maintain, and reuse. If a component becomes too large or complex, consider breaking it into smaller, reusable components.
Example: Instead of a UserProfileComponent that handles user data, user settings, and activity logs, create separate components like UserDataComponent, UserSettingsComponent, and UserActivityComponent.
For parent-child communication, use @Input() to pass data from a parent component to a child component and @Output() with EventEmitter to send data or events back to the parent. This pattern is clear simple, and keeps components decoupled.
Example:
// Parent Component
@Component({
selector: 'app-parent',
template: `<app-child [data]="parentData" (notify)="handleNotification($event)"></app-child>`
})
export class ParentComponent {
parentData = 'Hello from Parent!';
handleNotification(message: string) {
console.log('Received from child:', message);
}
}
// Child Component
@Component({
selector: 'app-child',
template: `<button (click)="sendMessage()">Send Message</button>`
})
export class ChildComponent {
@Input() data: string;
@Output() notify = new EventEmitter<string>();
sendMessage() {
this.notify.emit('Hello from Child!');
}
}
While Angular templates provide powerful features like data binding, it’s important to keep the logic in the component class, not in the template. The template should only be responsible for rendering the UI, while the class handles the logic, business rules, and data manipulation. This separation keeps the code clean and easier to test.
Example: Instead of adding complex conditionals or loops in the template, move them to the component class.
// Bad Example (logic in template)
@Component({
selector: 'app-example',
template: `<p>{{ someCondition ? 'Active': 'Inactive' }}</p>`
})
// Better Example (logic in class)
@Component({
selector: 'app-example',
template: `<p>{{ status }}</p>`
})
export class ExampleComponent {
status: string;
ngOnInit() {
This. Status = this.someCondition? 'Active': 'Inactive';
}
}
Move business logic and data management outside of the components by using services. Services handle data fetching, state management, and interaction with APIs, while components remain responsible for presenting the UI and receiving user interactions. This separation helps with code reusability, testability, and decoupling.
Example:
@Injectable({
provided: 'root,'
})
export class DataService {
constructor(private http: HttpClient) {}
fetchData() {
return this.http.get('https://api.example.com/data');
}
}
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
})
export class ExampleComponent {
data: any;
constructor(private dataService: DataService) {}
ngOnInit() {
this.dataService.fetchData().subscribe(response => {
this.data = response;
});
}
}
Leverage Angular’s lifecycle hooks like ngOnInit() for initialization and ngOnDestroy() for cleanup tasks. ngOnInit() is perfect for fetching data or initializing the state when a component is loaded. At the same time, ngOnDestroy() is ideal for cleaning up subscriptions, timers, and other resources to avoid memory leaks.
Example:
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
})
Export class ExampleComponent implements OnInit, OnDestroy {
subscription: Subscription;
ngOnInit() {
this.subscription = this.someService.getData().subscribe();
}
ngOnDestroy() {
this.subscription.unsubscribe(); // Clean up subscriptions
}
}
Angular provides lazy loading of modules to improve the performance of your application by loading modules only when needed. Lazy loading reduces the initial loading time by splitting your app into smaller bundles, which are loaded on demand. To implement lazy loading, use Angular’s RouterModule with the loadChildren property in your routing configuration.
Example:
const routes: Routes = [
{
path: 'feature',
loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule)
}
];
Angular supports view encapsulation, which helps prevent style leaks between components. By default, Angular uses Emulated encapsulation to scope styles to the component. If needed, you can opt for None or Shadow DOM encapsulation based on your requirements.
Use the appropriate encapsulation to ensure that your component’s styles don’t interfere with other parts of the app.
In Angular, styles can be applied to components to control the visual appearance of their templates. Angular allows you to define styles for each component in a modular and encapsulated way. There are several approaches to applying styles to Angular components, each providing a level of control over how styles are scoped and applied.
You can define styles directly within the component using the styles property in the component’s metadata. These styles are scoped only to the component, thanks to Angular’s view encapsulation. This means that the styles won’t leak out to other parts of the application.
Example:
@Component({
selector: 'app-example',
template: `<div class="message">Hello, Angular!</div>`,
styles: [
`.message { color: blue; font-size: 20px; }`
]
})
export class ExampleComponent {}
Here, the styles are directly embedded in the component and will apply only to this component's template.
Instead of writing styles inline, you can link to external CSS or SCSS files in the styleUrls array. This keeps the component’s styles separated from the logic, making the code cleaner and more maintainable.
Example:
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent {}
Here, the example.component.css file contains the styles that will only apply to this component.
Angular’s view encapsulation controls how styles are scoped and applied to components. There are three encapsulation strategies in Angular:
Example:
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css'],
Encapsulation: ViewEncapsulation.Emulated // Default
})
export class ExampleComponent {}
Using Emulated or Shadow DOM ensures that the styles are scoped only to the component, while None makes them global.
Another way to ensure styles are only applied within a component is by using specific CSS classes within the component's template. This helps prevent styles from affecting other components even if the encapsulation mode is set to None.
Example:
/* example.component.css */
.container {
background color: light blue;
padding: 20px;
}
<!-- example.component.html -->
<div class="container">This is a styled container</div>
To implement a component in Angular, you need to follow a series of steps to define its functionality, structure, and style. Below is a step-by-step guide to creating and implementing a component in Angular:
You can generate a new Angular component using Angular CLI by running the following command:
Ng generate component component-name
This will create a new folder with four files:
Alternatively, you can manually create these files if you prefer.
Inside the TypeScript file (component-name.component.ts), define the component's class, template, and style. Here’s a basic example:
import { Component } from '@angular/core';
@Component({
selector: 'app-component-name,'
templateUrl: './component-name.component.html',
styleUrls: ['./component-name.component.css']
})
export class ComponentNameComponent {
title: string = 'Hello, Angular!';
constructor() {}
}
In this example:
In the HTML file (component-name.component.html), define the structure of the component, including any Angular bindings or directives.
<h1>{{ title }}</h1>
<p>Welcome to the Angular Component!</p>
The {{ title }} syntax is an installation that binds the value of the title from the component class to the view.
In the CSS file (component-name.component.css), define the styles for the component.
h1 {
color: dark blue;
}
p {
font-size: 16px;
}
These styles will only apply to this component, as Angular uses view encapsulation by default.
Once the component is created, you can use it within the application by adding its selector in any parent component's template.
For example, if you want to include your new component in the app.component.html, use its selector as an HTML tag:
<!-- app.component.html -->
<app-component-name></app-component-name>
By default, when you generate a new component using Angular CLI, it will automatically be declared in the nearest Angular module (usually app.module.ts). Ensure that your component is declared in the @NgModule declarations array.
@NgModule({
declarations: [
AppComponent,
ComponentNameComponent // Ensure your component is listed here
],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
Once the component is implemented and the module is updated, you can run the Angular application using the following command:
ng serve
Open the browser at http://localhost:4200 to see your new component in action.
In Angular, components are reusable building blocks that encapsulate HTML templates, CSS styles, and logic. They manage the user interface and handle user interactions. Components help modularize code, improve maintainability, and promote reusability, making it easier to build scalable and dynamic applications with Angular's powerful features like data binding and dependency injection.
Copy and paste below code to page Head section
An Angular component is a building block of an Angular application. It encapsulates the HTML template, CSS styles, and business logic in a single unit, making it reusable and modular. Components define how the user interface (UI) behaves and interacts with the application.
You can create a component using Angular CLI by running the command: Ng generate component component-name This will generate four files: TypeScript, HTML, CSS/SCSS, and spec.ts for testing.
An Angular component consists of the following: Component Class: Contains logic, data, and methods. Template: Defines the HTML structure of the component. Styles: Defines the appearance using CSS or SCSS. Metadata: Provided through the @Component decorator to define how Angular should treat the component.
View encapsulation in Angular controls how styles are scoped to components. By default, Angular uses Emulated encapsulation, which isolates the styles to the component, ensuring they don’t leak out or affect other parts of the app. You can also use None or Shadow DOM for different behaviors.
Components can communicate in several ways: Input/Output: Parent-child communication using @Input() and @Output() decorators. Services: For sharing data or functionality between non-related components using Dependency Injection.
Angular components have lifecycle hooks, such as ngOnInit(), ngOnChanges(), and ngOnDestroy(), which allow developers to manage the component's state during its creation, update, and destruction phases.