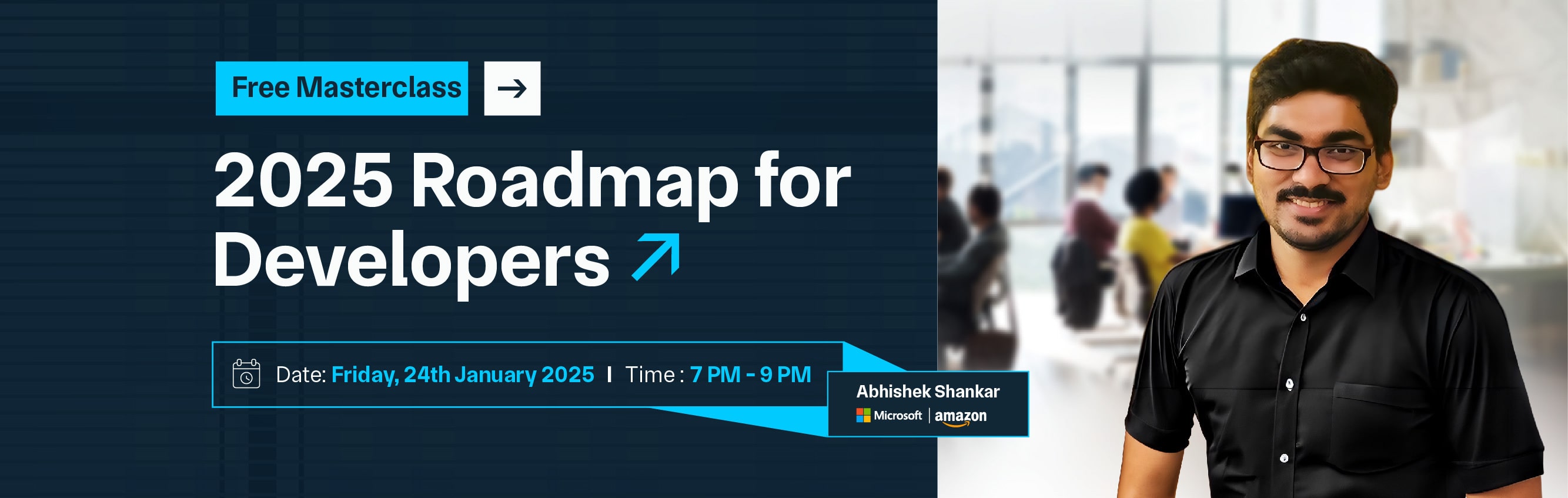

useLayoutEffect is a React hook that provides a way to perform side effects in function components, similar to useEffect, but with a critical difference in timing. While useEffect runs after the DOM has been updated and painted, useLayoutEffect fires synchronously after all DOM mutations but before the browser has painted. This makes useLayoutEffect ideal for tasks that require direct interaction with the DOM or need to measure layout properties, such as calculating element sizes or positions before the user sees the changes.
This hook ensures that any layout changes are applied immediately, avoiding potential visual inconsistencies or flickers that might occur if the DOM is painted before the changes are made. However, because it runs synchronously, it can block the browser’s paint process, potentially leading to performance issues if overused or misused.
Therefore, useLayoutEffect should be used only when necessary, particularly when you need to read layout values or make changes to the DOM that must be completed before the screen updates. For most side effects, useEffect is preferred due to its non-blocking nature.
useLayoutEffect is a React hook designed to manage side effects in function components with a specific focus on layout calculations and DOM measurements. It operates similarly to useEffect, but it differs in timing and use cases.
When you use useLayoutEffect, the code inside the hook executes synchronously after the DOM has been updated but before the browser has a chance to paint those updates to the screen. This means it runs immediately after the DOM changes, allowing you to make necessary updates or measurements before the user sees any changes.
Here’s why useLayoutEffect is useful:
However, because it runs synchronously and can block the browser’s rendering process, it should be used sparingly and only when necessary. For most side effects that don’t involve direct DOM measurements or layout manipulations, useEffect is usually sufficient and more performant.
The useLayoutEffect hook in React has a straightforward syntax and accepts the same parameters as useEffect. Here’s a detailed look at its syntax and parameters:
useLayoutEffect(effect, dependencies);
effect: This is a callback function that contains the code you want to run after the DOM has been updated. This function is executed synchronously, immediately after the DOM changes but before the browser paints those changes.
useLayoutEffect(() => {
// Your code here
}, [dependencies]);
dependencies: This is an optional array of dependencies. The effect will re-run whenever any value in this array changes. If you omit this array, the effect will run after every render. If you pass an empty array [], the effect will only run once after the initial render.
useLayoutEffect(() => {
// Your effect code
}, [dependency1, dependency2]);
import React, { useLayoutEffect, useRef, useState } from 'react';
function ExampleComponent() {
const [size, setSize] = useState(0);
const divRef = useRef(null);
useLayoutEffect(() => {
if (divRef.current) {
setSize(divRef.current.offsetWidth);
}
}, []); // Empty array means this effect runs once after the initial render
return (
<div ref={divRef} style={{ width: '100px' }}>
Width: {size}px
</div>
);
}
In this example, useLayoutEffect is used to measure the width of a div immediately after it is rendered, ensuring the size is updated accurately before the browser paints the component.
useLayoutEffect is a powerful React hook for managing side effects that need to interact directly with the DOM. Here’s when you should consider using useLayoutEffect:
1. Measuring Layouts and Dimensions: UseLayoutEffect when you need to measure or calculate layout properties (like element width, height, or position) after the DOM updates but before the browser paints. This ensures that your calculations are accurate and up-to-date.
useLayoutEffect(() => {
const width = elementRef.current.getBoundingClientRect().width;
console.log('Element width:', width);
}, []);
2. Preventing Layout Shifts: If your side effects involve making DOM changes that could impact the layout, using useLayoutEffect can help avoid visual inconsistencies. For example, if you need to set scroll positions or update styles based on component sizes, doing this synchronously helps prevent flickers or jumps.
useLayoutEffect(() => {
window.scrollTo(0, 0); // Ensure scroll position is correct before paint
}, []);
3. Synchronizing with External Libraries: When integrating with third-party libraries that perform DOM manipulations or rely on accurate layout calculations (such as D3 or chart libraries), useLayoutEffect ensures that the library's operations are in sync with the component's layout.
useLayoutEffect(() => {
library.initialize(elementRef.current);
}, [dependencies]);
4. Avoiding Layout Thrashing: If you need to perform multiple DOM reads and writes, using useLayoutEffect helps prevent layout thrashing. This is because it consolidates reads and writes before the browser paints, avoiding performance issues related to reflows.
useLayoutEffect(() => {
const rect = elementRef.current.getBoundingClientRect();
elementRef.current.style.width = `${rect.width}px`;
}, [dependencies]);
By understanding these use cases and carefully choosing when to use useLayoutEffect, you can enhance your React components' performance and visual stability.
useLayoutEffect and useEffect are both hooks in React used to handle side effects in function components, but they differ significantly in their timing and use cases. Here’s a detailed comparison:
useEffect: Executes after the DOM has been updated and painted. This means that the effect runs asynchronously after the render is complete, allowing the browser to paint the updates before the effect runs.
useEffect(() => {
// Side effect code
}, [dependencies]);
useLayoutEffect: Executes synchronously immediately after the DOM has been updated but before the browser paints. This ensures that the effect code runs before the user sees any changes, allowing for more precise control over layout and visual consistency.
useLayoutEffect(() => {
// Side effect code
}, [dependencies]);
useEffect: Suitable for effects that do not require immediate DOM manipulation or layout measurements. Common uses include data fetching, setting up subscriptions, or performing cleanups after the component unmounts.
useEffect(() => {
fetchData().then(data => setData(data));
return () => cleanup();
}, [dependencies]);
useLayoutEffect: Ideal for tasks that need to interact directly with the DOM, such as measuring dimensions, synchronizing with third-party libraries, or making changes that must be visible immediately to avoid visual inconsistencies.
useLayoutEffect(() => {
const rect = elementRef.current.getBoundingClientRect();
// Use rect to adjust layout
}, [dependencies]);
Use Case: Fetching data when the component mounts or when dependencies change.
Hook: useEffect
import React, { useEffect, useState } from 'react';
function DataFetcher() {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data when the component mounts
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
}
fetchData();
}, []); // Empty dependency array means this runs once
return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>;
}
Explanation: useEffect is used here because fetching data does not require immediate DOM manipulation. It runs asynchronously after the component renders and is, therefore, a good fit for this type of operation.
Use Case: Measuring the dimensions of an element to adjust layout or styles.
Hook: useLayoutEffect
import React, { useLayoutEffect, useRef, useState } from 'react';
function MeasureElement() {
const [size, setSize] = useState({ width: 0, height: 0 });
const elementRef = useRef(null);
useLayoutEffect(() => {
if (elementRef.current) {
const { offsetWidth, offsetHeight } = elementRef.current;
setSize({ width: offsetWidth, height: offsetHeight });
}
}, []); // Empty dependency array means this runs once
return (
<div ref={elementRef} style={{ width: '100px', height: '100px', backgroundColor: 'lightblue' }}>
Width: {size.width}px, Height: {size.height}px
</div>
);
}
Explanation: useLayoutEffect is used to measure the dimensions of the element before the browser paints the update. This ensures that the size calculations are accurate and prevents visual inconsistencies.
When using useLayoutEffect in React, it's important to be aware of potential pitfalls and follow best practices to ensure your application remains performant and bug-free. Here are some common issues and recommendations:
1. Blocking the Browser Paint Process
2. Unnecessary Use of useLayoutEffect
3. Creating Infinite Loops
4. Ignoring Cleanup
5. Inconsistent Layout Updates
1. Use useLayoutEffect Judiciously
2. Measure and Update Layout Safely
3. Optimize Dependencies
4. Provide Cleanup
5. Profile Performance
6. Prefer useEffect When Possible
By following these best practices and avoiding common pitfalls, you can effectively use useLayoutEffect to manage your component’s side effects while maintaining optimal performance and a smooth user experience.
Debugging and optimizing useLayoutEffect in React requires careful attention to the timing of effects, performance implications, and potential issues with rendering. Here’s a comprehensive guide on how to debug and optimize useLayoutEffect:
1. Check Timing Issues
useLayoutEffect(() => {
console.log('useLayoutEffect running');
// Your effect code here
}, []);
2. Inspect DOM Changes
3. Verify Dependencies
4. Check for Infinite Loops
5. Handle Errors Gracefully
useLayoutEffect(() => {
try {
// Your effect code here
} catch (error) {
console.error('Error in useLayoutEffect:', error);
}
}, []);
1. Minimize Heavy Calculations
2. Optimize Dependency Arrays
3. Avoid Layout Thrashing
useLayoutEffect(() => {
const element = elementRef.current;
const { offsetWidth, offsetHeight } = element; // Read dimensions
element.style.width = `${offsetWidth}px`; // Write dimensions
}, []);
4. Use Debouncing and Throttling
5. Profile Performance
6. Test Across Different Scenarios
By applying these debugging and optimization strategies, you can effectively manage useLayoutEffect in your React components, leading to a smoother user experience and more efficient performance.
Let’s walk through a hands-on example of using useLayoutEffect in a React component. We’ll create a simple component that measures the dimensions of a div element and adjusts its style based on these dimensions. This example demonstrates how useLayoutEffect can be used for precise DOM measurements and updates.
In this example, we’ll create a component that adjusts the width of a div based on its height, ensuring that the width is always 50% of the height. We’ll use the useLayoutEffect to perform the measurement and style updates synchronously before the browser paints.
import React, { useLayoutEffect, useRef, useState } from 'react';
function ResponsiveBox() {
const [dimensions, setDimensions] = useState({ width: 0, height: 0 });
const boxRef = useRef(null);
useLayoutEffect(() => {
// Function to update dimensions
const updateDimensions = () => {
if (boxRef.current) {
const height = boxRef.current.offsetHeight;
const width = height * 0.5; // Set width to 50% of height
setDimensions({ width, height });
}
};
// Update dimensions initially and on resize
updateDimensions();
window.addEventListener('resize', updateDimensions);
// Cleanup function to remove event listener
return () => {
window.removeEventListener('resize', updateDimensions);
};
}, []); // Empty dependency array means this runs once after the initial render
return (
<div
ref={boxRef}
style={{
width: `${dimensions.width}px`,
height: `${dimensions.height}px`,
backgroundColor: 'lightcoral',
transition: 'width 0.3s, height 0.3s'
}}
>
Responsive Box
</div>
);
}
export default ResponsiveBox;
1. State and Ref Setup:
2. useLayoutEffect:
3. Cleanup:
4. Styles:
useLayoutEffect is a vital React hook for managing side effects that require precise timing, particularly for tasks involving direct DOM interactions and layout adjustments. Unlike useEffect, which runs asynchronously after the browser has painted, useLayoutEffect executes synchronously after DOM updates but before the browser renders those changes, ensuring that any DOM measurements or manipulations are accurately reflected in the user’s view. This synchronous behavior makes useLayoutEffect ideal for tasks such as calculating element dimensions or synchronizing with third-party libraries.
However, it should be used judiciously to avoid blocking the browser’s paint process, which can impact performance. Proper use involves careful management of dependencies, avoiding layout thrashing, and always including cleanup functions to prevent memory leaks. By understanding these aspects and applying best practices, you can effectively use useLayoutEffect to create responsive, performant, and visually consistent React components, ultimately enhancing the user experience.
Copy and paste below code to page Head section
useLayoutEffect and useEffect are both hooks for managing side effects in React, but they differ in timing. useLayoutEffect runs synchronously after the DOM has been updated but before the browser paints the updates. This allows you to make changes or measurements to the DOM before it is visible to the user. In contrast, useEffect runs asynchronously after the DOM updates and the browser has painted, making it suitable for side effects that do not need to be immediately visible or require layout measurements.
Use useLayoutEffect when you need to perform operations that require immediate access to the DOM or need to measure layout changes before the browser paints. This is particularly useful for tasks such as calculating element dimensions, synchronizing with external libraries, or making layout adjustments that should be visible without flickers. For most other side effects, such as data fetching or subscriptions, useEffect is typically sufficient and more performant.
Yes, useLayoutEffect can impact performance if not used carefully. Since it runs synchronously, it can block the browser’s rendering process, leading to potential delays or visual issues. To mitigate this, use useLayoutEffect only when necessary and ensure that heavy computations are avoided. For side effects that don’t require precise timing, useEffect is a better choice.
The dependency array of useLayoutEffect should include all variables and props that the effect depends on. This ensures that the effect runs whenever any of these dependencies change. Be cautious not to include functions or objects that are recreated on each render unless necessary, as this can lead to unnecessary re-renders.
useLayoutEffect does not run on the server during server-side rendering (SSR) because it is designed to run after the DOM is updated and the browser paint occurs. For server-side rendering, where there is no DOM to interact with, useEffect is typically used. React will automatically handle the difference between useEffect and useLayoutEffect when rendering on the client side.
To debug useLayoutEffect, use console.log statements to track when the effect runs and verify if DOM measurements or manipulations are correct. Check the browser’s developer tools to inspect the DOM and ensure that changes are applied as expected. Additionally, monitor performance using React’s profiling tools and ensure dependencies are correctly specified to avoid infinite loops.