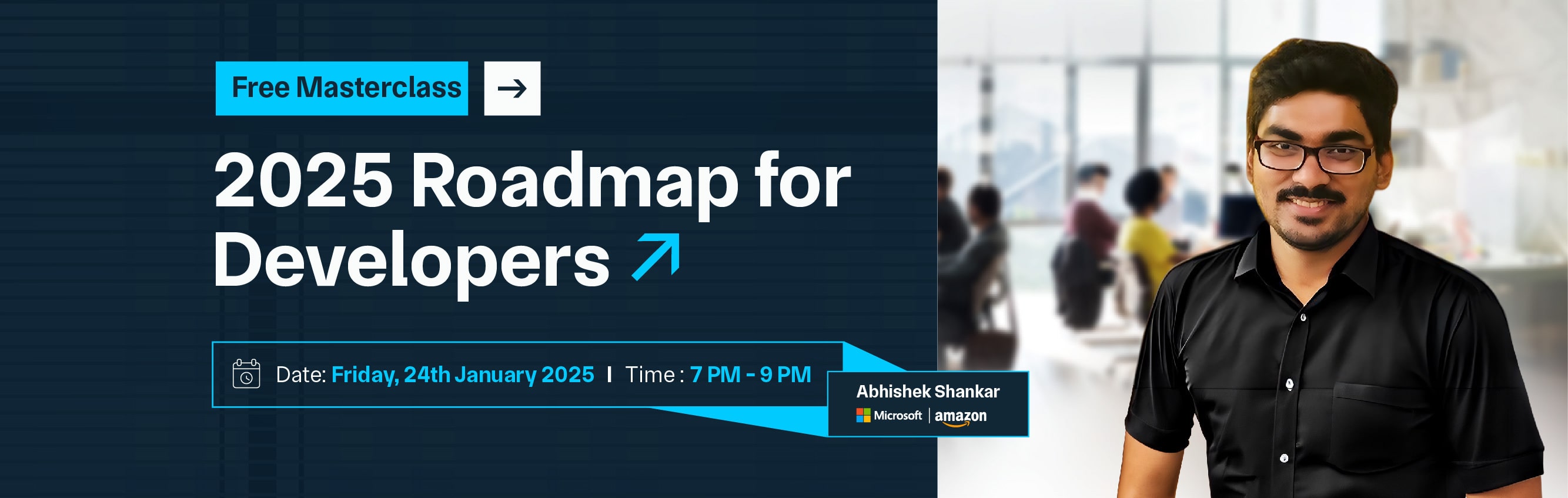

The React useEffect hook is a powerful tool for managing side effects in functional components. Introduced in React 16.8, it allows developers to perform operations like data fetching, subscriptions, or manually changing the DOM after rendering. The hook accepts two arguments: a callback function that contains the side effect logic and an optional dependency array.
The callback runs after every render by default, but by specifying dependencies, you can control when the effect should be re-run. For example, passing an empty array makes the effect run only once, similar to componentDidMount while providing specific values causes it to re-run when those values change. This capability helps to optimize performance by preventing unnecessary operations.
Additionally, useEffect can return a cleanup function, which is executed before the effect runs again or when the component unmounts, making it useful for cleaning up resources like subscriptions or timers. Understanding useEffect is crucial for managing lifecycle events in React applications, enabling developers to create responsive and efficient user interfaces that react to changes in state or props seamlessly.
The useEffect hook in React is a built-in hook that allows you to manage side effects in functional components. Side effects are operations that can affect other components or interact with the outside world, such as data fetching, subscriptions, or manual DOM manipulations.
Here’s a quick overview of how useEffect works:
Syntax: It takes two parameters:
Execution: By default, the effect runs after every render. However, if you provide an empty array as the second argument, the effect will run only once after the initial render, mimicking the behavior of componentDidMount. If you include variables in the array, the effect will be re-run whenever those variables change.
Cleanup: You can return a cleanup function from the effect. This function runs before the component unmounts or before the effect re-runs, allowing you to clean up resources, such as canceling subscriptions or clearing timers.
Overall, useEffect helps manage side effects in a declarative way, making it easier to handle asynchronous operations and maintain clean component logic.
The useEffect hook in React is a powerful tool for managing side effects in functional components. Here are some reasons to choose useEffect:
Using useEffect leads to cleaner, more predictable code in functional components, aligning well with React's declarative nature.
The useEffect hook works by allowing you to run side effects in your functional components. Here’s a breakdown of how it functions:
useEffect(() => {
// Your side effect code here
return () => {
// Cleanup code (optional)
};
}, [dependencies]);
Importing useEffect hook
To use the useEffect hook in a React component, you need to import it from the React library. Here’s how you can do it:
import React, { useEffect } from 'react';
const MyComponent = () => {
useEffect(() => {
// Your side effect logic here
return () => {
// Cleanup logic here (optional)
};
}, []); // Dependency array
return (
<div>
{/* Your component JSX here */}
</div>
);
};
export default MyComponent;
In this example, useEffect is used to handle side effects, such as data fetching or subscriptions. The second argument, an empty array ([]), ensures that the effect runs only once when the component mounts. You can adjust the dependencies in the array to control when the effect runs.
In this example, useEffect is used to handle side effects, such as data fetching or subscriptions. The second argument, an empty array ([]), ensures that the effect runs only once when the component mounts. You can adjust the dependencies in the array to control when the effect runs.
The useEffect hook has a specific structure and syntax that you should follow. Here’s a breakdown:
Basic Structure
useEffect(() => {
// Effect logic (side effects)
return () => {
// Cleanup logic (optional)
};
}, [dependencies]);
Components of the Structure
1. Effect Function:
2. Cleanup Function:
3. Dependency Array:
The second argument is an array of dependencies. This tells React when to re-run the effect:
Example
Here's a more detailed example:
import React, { useEffect, useState } from 'react';
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
// Side effect: fetch data
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
};
fetchData();
// Cleanup function (if needed)
return () => {
// Cleanup code here (e.g., aborting fetch, clearing timers, etc.)
};
}, []); // Runs once on mount
return (
<div>
{data? <div>{JSON.stringify(data)}</div> : <p>Loading...</p>}
</div>
);
};
export default MyComponent;
The useEffect hook allows you to run code that has side effects in your React components. Side effects can include things like fetching data, setting up subscriptions, or manually changing the DOM.
If you want something to happen only when your component first loads (like fetching data), use an empty dependency array:
import React, { useEffect, useState } from 'react';
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data from an API
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
};
fetchData();
}, []); // This runs only once when the component mounts
return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>;
};
Wrapping effects in custom hooks is a great way to encapsulate logic that can be reused across multiple components. Custom hooks allow you to share stateful logic without changing the component structure. Here’s how you can create and use custom hooks with useEffect.
Let’s create a custom hook that fetches data from an API.
import { useState, useEffect } from 'react';
const useFetch = (url) => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(url);
if (!response. ok) {
throw new Error('Network response was not ok');
}
const result = await response.json();
setData(result);
} catch (err) {
setError(err.message);
} finally {
setLoading(false);
}
};
fetchData();
}, [url]); // Re-run effect if URL changes
return { data, loading, error };
};
Now, you can use this custom hook in any component to fetch data easily.
Import React from 'react';
import useFetch from './useFetch'; // Adjust the path as needed
const DataDisplay = () => {
const { data, loading, error } = useFetch('https://api.example.com/data');
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error}</p>;
return (
<div>
<h1>Data</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
};
export default data display;
You can create a custom hook for managing the state with local storage:
import { useState, useEffect } from 'react';
const use local storage = (key, initialValue) => {
const [storedValue, setStoredValue] = useState(() => {
try {
const item = window.localStorage.getItem(key);
Return item? JSON.parse(item) : initialValue;
} catch (error) {
console.error(error);
return initialValue;
}
});
useEffect(() => {
try {
window.localStorage.setItem(key, JSON.stringify(storedValue));
} catch (error) {
console.error(error);
}
}, [key, storedValue]);
return [storedValue, setStoredValue];
};
You can use this hook in your components like this:
const App = () => {
const [name, setName] = useLocalStorage('name', 'John Doe');
return (
<div>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<p>Your name is: {name}</p>
</div>
);
};
Controlling a non-React widget (like a third-party library or a custom UI component) from a React component involves integrating the widget into the React lifecycle. Here’s how you can do that effectively using hooks, particularly useEffect, to manage the widget's lifecycle and state.
Let’s say we want to integrate a non-React charting library (like Chart.js) into a React component.
You can install Chart.js using npm:
npm install chart.js
import React, { useEffect, useRef } from 'react';
import { Chart } from 'chart.js';
const ChartComponent = ({ data, options }) => {
const canvasRef = useRef(null); // Create a ref for the canvas element
useEffect(() => {
// Initialize the chart when the component mounts
const ctx = canvasRef.current.getContext('2d');
const chartInstance = new Chart(ctx, {
Type: 'bar,'// or 'line,' 'pie,' etc.
data: data,
options: options,
});
// Cleanup function to destroy the chart instance
return () => {
chartInstance.destroy();
};
}, [data, options]); // Re-run if data or options change
return <canvas ref={canvasRef} />;
};
export default ChartComponent;
Now you can use your chart component in another component:
Import React from 'react';
import ChartComponent from './ChartComponent'; // Adjust path as needed
const App = () => {
const data = {
labels: ['Red,' 'Blue,' 'Yellow'],
datasets: [
{
label: 'Votes',
data: [12, 19, 3],
backgroundColor: ['rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)'],
borderColor: ['rgba(255, 99, 132, 1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)'],
borderWidth: 1,
},
],
};
const options = {
scales: {
y: {
beginAtZero: true,
},
},
};
return (
<div>
<h1>My Chart</h1>
<ChartComponent data={data} options={options} />
</div>
);
};
export default App;
Fetching data with the useEffect hook in React is a common pattern for loading data from an API or other sources when a component mounts or when certain dependencies change. Here’s a step-by-step guide on how to effectively fetch data using useEffect.
Let’s create a simple component that fetches data from a public API.
import React, { useEffect, useState } from 'react';
const DataFetcher = () => {
const [data, setData] = useState(null); // State for data
const [loading, setLoading] = useState(true); // State for loading
const [error, setError] = useState(null); // State for errors
useEffect(() => {
// Function to fetch data
const fetchData = async () => {
try {
setLoading(true); // Set loading to true before fetching
const response = await fetch('https://api.example.com/data'); // Replace with your API
if (!response. ok) {
throw new Error('Network response was not ok'); // Handle non-200 responses
}
const result = await response.json(); // Parse JSON response
setData(result); // Set the fetched data
} catch (err) {
setError(err.message); // Set error if fetching fails
} finally {
setLoading(false); // Set loading to false after fetching
}
};
fetchData(); // Call the fetch function
}, []); // Empty dependency array to run once on mount
// Render loading, error, or data states
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error}</p>;
return (
<div>
<h1>Fetched Data</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
};
export default DataFetcher;
In React, when you use the useEffect hook, specifying reactive dependencies correctly is crucial for controlling when your effects run. This allows you to optimize performance, prevent unnecessary re-renders, and ensure that your component behaves as expected. Here’s how to do it effectively.
The dependency array in useEffect determines when the effect should run. Here are the main scenarios:
If you want an effect to run only once when the component mounts (similar to componentDidMount), use an empty array:
import React, { useEffect } from 'react';
const Component = () => {
useEffect(() => {
console.log('Component mounted');
// You could fetch data here
return () => {
console.log('Cleanup on unmount');
};
}, []); // Empty array means this runs once
return <div>Hello World</div>;
};
If you want the effect to run whenever a specific state variable changes, include that variable in the dependency array:
import React, { useEffect, useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
useEffect(() => {
console.log(`Count updated: ${count}`);
}, [count]); // This runs every time `count` changes
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
If you have multiple pieces of state or props that should trigger the effect when they change, list them all:
import React, { useEffect, useState } from 'react';
const UserProfile = ({ userId }) => {
const [userData, setUserData] = useState(null);
const [refresh, setRefresh] = useState(false);
useEffect(() => {
const fetchUserData = async () => {
const response = await fetch(`https://api.example.com/users/${userId}`);
const data = await response.json();
setUserData(data);
};
fetchUserData();
}, [userId, refresh]); // Runs when `userId` or `refresh` changes
return (
<div>
<h1>User Profile</h1>
<button onClick={() => setRefresh(prev => !prev)}>Refresh</button>
{userData && <div>{JSON.stringify(userData)}</div>}
</div>
);
};
Updating the state based on the previous state within an effect in React can be tricky. Still, it’s essential to ensure that your state updates correctly, especially when dealing with asynchronous operations or when state changes are dependent on previous values. Here's how to do it effectively.
When you want to update a state based on its previous value, it’s recommended to use the functional form of the state setter function. This ensures you have the most recent state, preventing stale closures.
Let’s create an example where we increment a counter based on its previous state when an effect runs. This is common in situations like fetching data that might trigger a series of updates.
import React, { useEffect, useState } from 'react';
const CounterWithEffect = () => {
const [count, setCount] = useState(0);
useEffect(() => {
const timer = setTimeout(() => {
// Update the count based on the previous state
setCount(prevCount => prevCount + 1); // Use functional update
}, 1000); // Increment count every second
// Cleanup function to clear the timeout
return () => clearTimeout(timer);
}, []); // Run this effect only once when the component mounts
return (
<div>
<p>Count: {count}</p>
</div>
);
};
export default CounterWithEffect;
1. State Initialization:
2. Effect Hook:
3. Functional State Update:
4. Cleanup:
In cases where the effect depends on a state that might change, you can still use the functional form:
const NumberDoubler = () => {
const [number, setNumber] = useState(1);
useEffect(() => {
const interval = setInterval(() => {
setNumber(prevNumber => prevNumber * 2); // Double the previous number
}, 2000); // Double every 2 seconds
return () => clearInterval(interval);
}, []); // Run once on mount
return (
<div>
<p>Number: {number}</p>
</div>
);
};
When using the useEffect hook in React, it's crucial to manage dependencies carefully to avoid unnecessary re-renders and performance issues. One common pitfall is including objects or arrays directly in the dependency array, which can lead to unexpected behavior due to how JavaScript handles object reference equality. Here’s how to effectively manage dependencies and avoid unnecessary updates.
When you include an object in the dependency array of useEffect, React checks for reference equality. This means that even if the contents of the object haven’t changed if a new object is created on each render, the effect will run again.
Consider the following example where an object is used as a dependency:
import React, { useEffect, useState } from 'react';
const ExampleComponent = () => {
const [count, setCount] = useState(0);
const options = { threshold: 10 }; // Example object
useEffect(() => {
console.log('Effect ran:', options);
}, [options]); // This will run on every render
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default ExampleComponent;
In this example, the effect will run every time the component renders, even if options haven't changed, because a new object is created on each render.
Memoizing Objects with useMemo: Use useMemo to ensure that the object reference stays the same unless its dependencies change.
import React, { useEffect, useState, useMemo } from 'react';
const ExampleComponent = () => {
const [count, setCount] = useState(0);
const options = useMemo(() => ({ threshold: 10 }), []); // Memoized object
useEffect(() => {
console.log('Effect ran:', options);
}, [options]); // This will now only run once
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default ExampleComponent;
Using State to Manage Complex Objects: If the object changes based on user interactions or API responses, you can use useState to manage it.
import React, { useEffect, useState } from 'react';
const ExampleComponent = () => {
const [count, setCount] = useState(0);
const [options, setOptions] = useState({ threshold: 10 });
useEffect(() => {
console.log('Effect ran:', options);
}, [options]); // Will only run when options change
const updateOptions = () => {
setOptions(prevOptions => ({ ...prevOptions, threshold: prevOptions.threshold + 1 }));
};
return (
<div>
<p>Count: {count}</p>
<p>Threshold: {options.threshold}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={updateOptions}>Update Options</button>
</div>
);
};
export default ExampleComponent;
Flattening State: If you have nested objects, consider flattening your state to avoid complex dependencies.
Reading the latest props and states from within a useEffect hook in React is important to ensure that your effect behaves correctly and responds to the most recent values. However, there are specific practices to follow to avoid issues like stale closures, especially when dealing with asynchronous operations or effects that depend on props and state.
In React, when you define a function (like the one in useEffect), it "captures" the values of variables from the surrounding scope at that time. If those variables change after the effect is defined, the effect won’t see the updated values unless you handle it correctly.
Here’s an example where the effect depends on props and state:
import React, { useEffect, useState } from 'react';
const TimerComponent = ({ interval }) => {
const [count, setCount] = useState(0);
useEffect(() => {
const timer = setInterval(() => {
setCount(prevCount => prevCount + 1); // Using previous state
}, interval); // Runs the timer based on the latest interval prop
return () => clearInterval(timer); // Cleanup on unmount or interval change
}, [interval]); // Re-run effect when interval changes
return <div>Count: {count}</div>;
};
export default TimerComponent;
In situations where you have asynchronous code and need to ensure you're using the latest values, a ref can help:
import React, { useEffect, useState, useRef } from 'react';
const FetchDataComponent = ({ userId }) => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(false);
const latestUserId = useRef(userId); // Create a ref to hold the latest userId
// Update ref whenever userId changes
useEffect(() => {
latestUserId.current = userId;
}, [userId]);
useEffect(() => {
const fetchData = async () => {
setLoading(true);
try {
const response = await fetch(`https://api.example.com/users/${latestUserId.current}`);
const result = await response.json();
setData(result);
} catch (error) {
console.error(error);
} finally {
setLoading(false);
}
};
fetchData();
}, []); // This effect runs once on mount
if (loading) return <p>Loading...</p>;
return <div>{data ? JSON.stringify(data) : 'No data'}</div>;
};
export default FetchDataComponent;
When working with the useEffect hook and managing state in React, you may encounter various issues. Here are some common troubleshooting strategies and tips to help you diagnose and resolve problems effectively.
1. Effect Not Running as Expected
useEffect(() => {
console.log('Effect ran', count);
}, [count]); // Ensure `count` is included if you want it to trigger the effect
2. Stale Closures
3. Infinite Loops
useEffect(() => {
if (condition) {
setState(newValue);
}
}, [dependency]); // Ensure condition prevents infinite loop
4. Memory Leaks
useEffect(() => {
const timer = setInterval(() => {
// some logic
}, 1000);
return () => clearInterval(timer); // Cleanup on unmount
}, []);
5. Incorrect State Updates
setCount(prevCount => prevCount + 1); // Correct way to update based on previous state
The useEffect hook is essential for managing side effects in React functional components. It allows for efficient handling of tasks like data fetching and subscriptions while ensuring performance through proper dependency management. Mastering useEffect leads to cleaner, more maintainable code, enhancing overall application responsiveness and reliability.
Copy and paste below code to page Head section
useEffect is a React hook that allows you to perform side effects in functional components, such as data fetching, subscriptions, or manually updating the DOM.
It runs after every render by default, but you can control its execution by providing a dependency array. It will only run when the values in that array change.
The dependency array determines when the effect should run. If you pass an empty array ([]), the effect runs only once after the initial render. If you include a specific state or props, it runs when those values change.
You can return a cleanup function from within useEffect. This function will run before the effect is re-executed or when the component unmounts, helping to prevent memory leaks.
Yes, you can use multiple useEffect hooks in a single component to handle different side effects separately, making your code more organized and manageable.
A common issue is stale closures, where the effect captures outdated values of state or props. To avoid this, use the functional form of the state setter or manage the latest values with useRef.