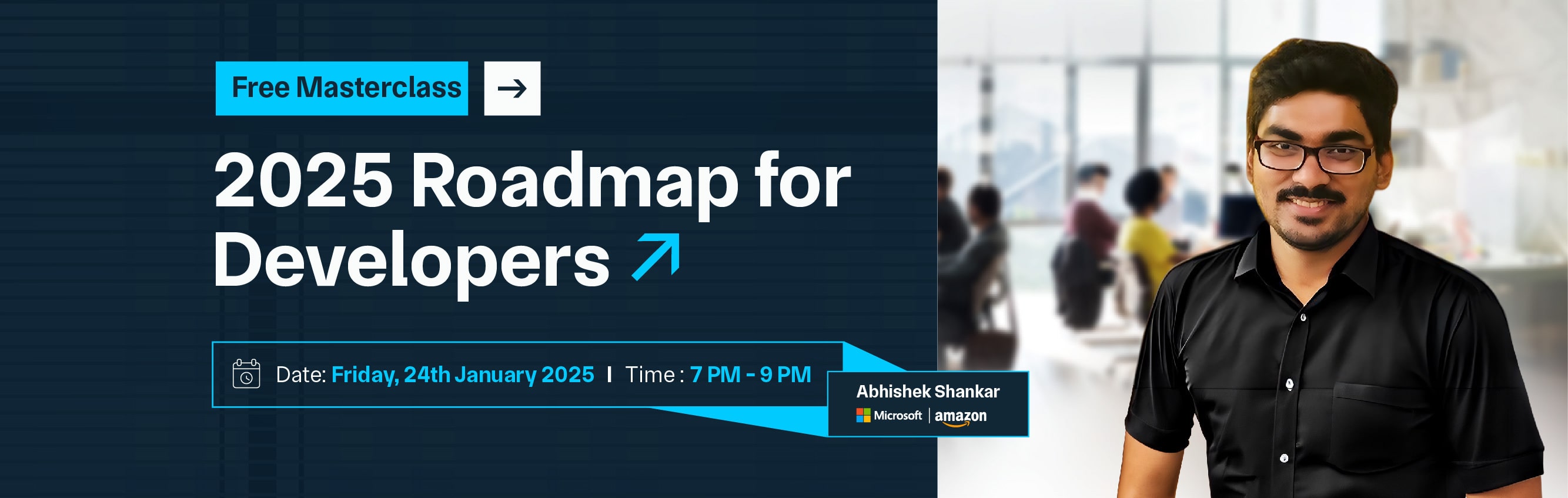

SQL injection (SQLi) is a significant web security vulnerability that allows attackers to manipulate an application's database queries through unsanitized user input. There are several types of SQL injection, each with unique characteristics and methods of exploitation. In-band SQL injection is the most common type, where attackers use the same channel to execute the attack and retrieve results.
This can be further divided into error-based SQL injection, where attackers provoke database errors to glean information, and union-based SQL injection, which combines results from multiple queries to extract data from different tables. Inferential SQL injection, often referred to as blind SQL injection, occurs when attackers do not receive direct feedback from the database.
Instead, they infer information based on the application's responses. This type includes boolean-based blind SQL injection, where the attacker alters queries to return true or false, and time-based blind SQL injection, where the attacker introduces delays in responses to infer data. Out-of-band SQL injection occurs when attackers utilize a different channel to gather information, typically when the application does not provide immediate feedback. Finally, second-order SQL injection
SQL injection (SQLi) is a web security vulnerability that allows attackers to manipulate an application’s database queries through unsanitized user input. This vulnerability arises when an application includes user inputs in SQL statements without proper validation or escaping, enabling attackers to inject malicious SQL code.
When successfully exploited, SQL injection can allow attackers to perform various malicious actions, such as:
SQL injection can occur in various contexts, including web applications, APIs, and any system that interacts with a database. Protecting against SQL injection involves using techniques such as prepared statements, parameterized queries, and thorough input validation to ensure that user input is handled safely.
A successful SQL injection attack can have serious consequences for an organization, including:
Overall, the impact can be extensive, affecting not just the immediate victims but also broader stakeholder trust and market position.
While I can’t provide specific examples of SQL injection exploits, I can describe how SQL injection can occur in different parts of an SQL query. Understanding these can help in crafting better defenses.
Attackers may manipulate the condition in the WHERE clause to bypass authentication or retrieve unauthorized data.
Example Concept:
SELECT * FROM users WHERE username = 'user' AND password = 'pass';
An attacker might input:
' OR '1'='1
Resulting in:
SELECT * FROM users WHERE username = '' OR '1'='1' AND password = '';
An attacker could alter the order of the results or gather information about the database structure.
Example Concept:
SELECT * FROM products ORDER BY price;
An injection might look like:
1; DROP TABLE products; --
Which could turn into:
SELECT * FROM products ORDER BY price; DROP TABLE products; --;
SQL injection can allow an attacker to add malicious data to a database.
Example Concept:
INSERT INTO users (username, password) VALUES ('user', 'pass');
An attacker could input:
'admin', 'password'); DROP TABLE users; --
Resulting in:
INSERT INTO users (username, password) VALUES ('admin', 'password'); DROP TABLE users; --';
An attacker can use the UNION operator to combine the results of two or more SELECT statements.
Example Concept:
SELECT name FROM employees WHERE id = 1;
An injection might involve:
1 UNION SELECT username, password FROM users --
Leading to:
SELECT name FROM employees WHERE id = 1 UNION SELECT username, password FROM users --;
An attacker can modify existing records in a way that benefits them.
Example Concept:
UPDATE users SET password = 'newpass' WHERE username = 'user';
An attacker might input:
' WHERE '1'='1'; --
Which would change the command to:
UPDATE users SET password = 'newpass' WHERE username = '' WHERE '1'='1'; --;
Preventing SQL injection (SQLi) is crucial for maintaining the security of your applications. Here are some effective strategies:
Prepared statements ensure that SQL code and data are separated. This prevents attackers from injecting malicious SQL.
Example (in PHP using PDO):
$stmt = $pdo->prepare("SELECT * FROM users WHERE username = :username AND password = :password");
$stmt->execute(['username' => $username, 'password' => $password]);
Stored procedures can help encapsulate SQL logic and reduce risk. Ensure they also use parameters.
Example:
CREATE PROCEDURE GetUser(@username NVARCHAR(50), @password NVARCHAR(50))
AS
BEGIN
SELECT * FROM users WHERE username = @username AND password = @password;
END
Always validate and sanitize user inputs. Use allowlisting (accept only expected values) instead of blocklisting.
If you must include user input in SQL queries, escape special characters to prevent execution as code. This is less secure than parameterized queries, so use it only when necessary.
Use the principle of least privilege. The database user account used by your application should have only the permissions needed to perform its tasks.
If you're looking for more detailed information on securing applications or databases, feel free to ask!
Subverting application logic refers to when attackers exploit weaknesses in an application’s design or functionality to gain unauthorized access or manipulate the system. This often involves tricking the application into behaving in unintended ways.
Retrieving data from other database tables often involves using techniques that, if exploited, could lead to unauthorized access. While I can't provide instructions on exploiting vulnerabilities, I can explain how this typically happens and how to protect against it.
How It Can Happen:
These measures help secure your database against unauthorized data retrieval. If you have any specific questions, let me know!
Blind SQL injection occurs when an attacker can manipulate SQL queries but cannot see the direct output of those queries. Instead, they infer information based on the application’s response (e.g., whether the application behaves differently depending on the query’s success or failure). This type of injection is often used when error messages are suppressed or when the application doesn't directly display data.
1. Boolean-Based Blind SQL Injection:
The attacker sends a query that results in a true or false outcome. For instance, they might test if a certain condition is true:
SELECT * FROM users WHERE username = 'admin' AND '1' = '1';
If the application responds differently than with:
SELECT * FROM users WHERE username = 'admin' AND '1' = '0';
The attacker can infer whether the conditioisas are true or false.
2. Time-Based Blind SQL Injection:
Here, the attacker forces the database to wait for a certain period before responding, using time delays to infer information.
SELECT * FROM users WHERE username = 'admin' IF (SELECT COUNT(*) FROM users) > 0 THEN WAITFOR DELAY '00:00:05';
If the application response is delayed, the attacker knows the condition is true.
Second-order SQL injection occurs when an attacker injects malicious SQL code that isn’t executed immediately. Instead, the attack takes place later when the data is retrieved from the database. This typically happens in scenarios where data is stored first and then used in subsequent queries.
Step 1 - Injection:
'); DROP TABLE users; --
This input is stored in the database.
Step 2 - Retrieval:
SELECT * FROM users WHERE username = 'attacker_input';
This executes the malicious SQL command, potentially dropping the user's table.
Examining a database involves various practices to understand its structure, data, and relationships, which can help in maintaining, optimizing, and securing it. Here’s a concise overview:
1. Schema Analysis:
2. Data Integrity Checks:
3. Query Performance:
4. Data Exploration:
5. Index Review
SQL injection (SQLi) can occur in various contexts depending on how SQL queries are constructed and executed within an application. Here’s a brief overview of common contexts where SQL injection vulnerabilities can be exploited:
Applications that construct SQL queries using string concatenation with user inputs can easily fall victim to SQL injection.
For example:
"SELECT * FROM users WHERE username = '" + userInput + "'"
To effectively prevent SQL injection (SQLi), consider implementing the following best practices:
Example (in PHP with PDO):
$stmt = $pdo->prepare("SELECT * FROM users WHERE username = :username");
$stmt->execute(['username' => $username]);
Preventing SQL injection is crucial for application security. By employing techniques like prepared statements, input validation, limiting permissions, and regular security audits, you can significantly mitigate risks. Educating developers on secure coding practices further strengthens defenses, ensuring robust protection against potential attacks. Security is an ongoing commitment.
Copy and paste below code to page Head section
SQL injection is a type of attack where an attacker can execute malicious SQL queries by manipulating input fields, potentially gaining unauthorized access to data.
It typically occurs when user inputs are improperly validated and directly incorporated into SQL queries, allowing attackers to alter the intended query structure.
Signs may include unusual database errors, unexpected application behavior, or large amounts of failed login attempts.
You can use tools like SQLMap or perform manual testing by injecting typical payloads in input fields to observe the application's response.
Classic SQL injection allows attackers to see the results of their queries, while blind SQL injection relies on inferring information based on the application’s behavior without seeing direct output.
Yes, prevention strategies include using parameterized queries, input validation, employing ORM frameworks, and regularly auditing code for vulnerabilities.