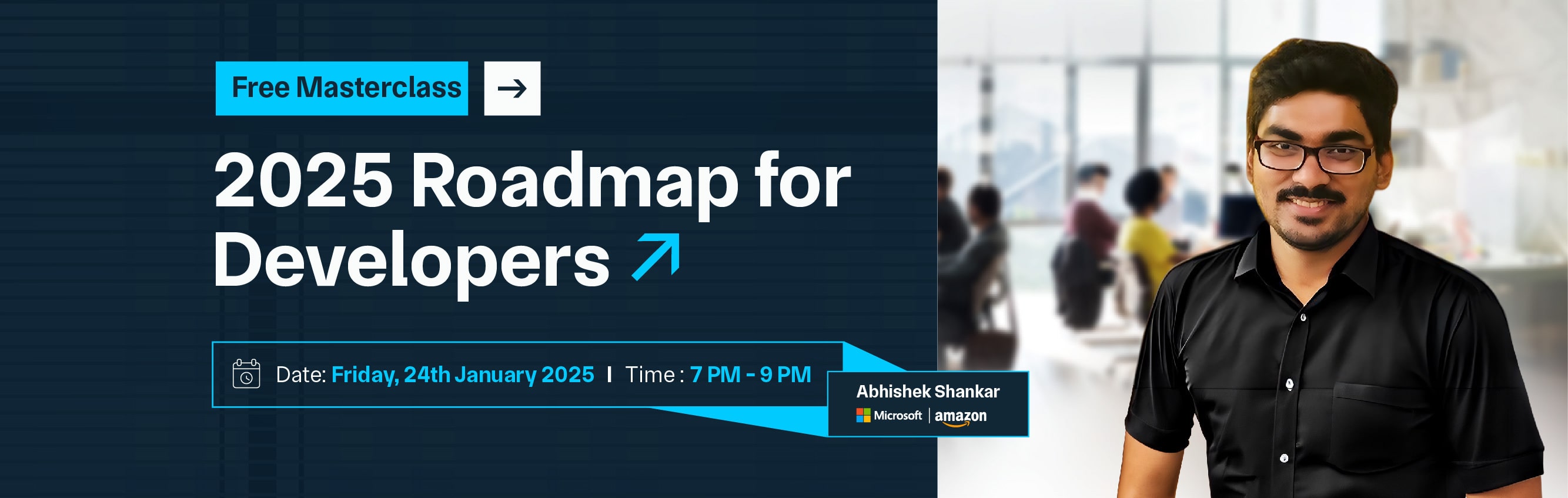

In Python, sys. Args is a list that contains the command-line arguments passed to a script when it is executed. It is part of the sys module, which must be imported to access this functionality. The first element of sys.argv (sys.argv[0]) is always the name of the script being run, and the following elements (sys.argv[1:]) contain any additional arguments passed by the user.
This allows Python scripts to accept dynamic inputs from the command line, such as file paths, configuration settings, or flags, which can alter the behavior of the script. For example, if you run a script with the command python myscript.py input.txt output.txt, sys.argv will hold the list ['myscript.py,' 'input.txt,' 'output.txt']. The script can then access these arguments to perform operations, such as reading from input.txt and writing to output.txt.
While sys.argv is simple to use; it only provides basic functionality for handling arguments. For more advanced parsing, such as handling optional arguments, type conversion, or displaying help messages, Python's argparse module is often a better choice. It provides a more user-friendly way to manage command-line arguments and improve error handling.
To use sys.argv in Python, you must first import the sys module, as it provides access to command-line arguments passed to a script. The sys.argv list holds all the arguments, with sys.argv[0] being the script name and sys.argv[1:] containing the arguments provided by the user when running the script. Here's a step-by-step guide on how to use sys.argv:
Import the sys module:
import sys
Access command-line arguments: The command-line arguments are stored in sys.argv, which is a list. The first element is the script name, and subsequent elements are the arguments passed to the script.
print(sys.argv)
If you run the script python myscript.py arg1 arg2, the output will be:
['myscript.py', 'arg1', 'arg2']
Use arguments in your script: You can access the command-line arguments directly from sys.argv and use them to control the flow of your program.
For example, to print the arguments:
import sys
if len(sys.argv) > 1:
print(f"Script name: {sys.argv[0]}")
print(f"First argument: {sys.argv[1]}")
print(f"Second argument: {sys.argv[2]}")
Else:
print("No arguments provided.")
Handle errors: If you expect a certain number of arguments, you should check if sys.argv has the required length before accessing the elements to avoid IndexError.
Example with error handling:
import sys
if len(sys.argv) < 3:
print("Please provide two arguments.")
Else:
print(f"First argument: {sys.argv[1]}")
print(f"Second argument: {sys.argv[2]}")
Let's say you want to create a script that accepts two arguments — an input file and an output file. Here's a basic example of how to use sys.argv:
import sys
# Check if the correct number of arguments are passed
if len(sys.argv) != 3:
print("Usage: python script.py <input_file> <output_file>")
sys.exit(1)
input_file = sys.argv[1]
output_file = sys.argv[2]
# Open the files (assuming text files for simplicity)
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
content = infile.read()
outfile.write(content)
print(f"Content from {input_file} has been written to {output_file}")
If you run the above script from the command line like this:
python script.py input.txt output.txt
It will read the content from input.txt and write it to output.txt.
Sys.argv in Python is a list that contains the command-line arguments passed to a script when it is executed. It is part of the sys module, which provides access to variables and functions that interact with the Python runtime environment. The argv stands for "argument vector" and allows you to pass arguments to your Python script from the command line, which can be used to influence the behavior of the script during execution.
When you run a Python script from the command line, you can pass additional arguments after the script name. These arguments are captured in sys.argv as strings, where the first element (sys.argv[0]) is always the name of the script, and the following elements (sys.argv[1:]) the user provides the arguments.
For example, if you run a Python script like this:
python myscript.py arg1 arg2 arg3
The value of sys.argv would be:
['myscript.py', 'arg1', 'arg2', 'arg3']
You can access these arguments in your script to make decisions, configure settings, or perform tasks dynamically. Sys.argv is commonly used for basic command-line argument parsing, such as reading input files or adjusting script parameters. However, for more complex argument parsing, the argparse module is often preferred.
sys.argv in Python allows you to pass and access command-line arguments, providing a simple way to make scripts dynamic. By using the sys.argv list, you can access the script name and any arguments provided, enabling customizable behavior based on user input during script execution.
Copy and paste below code to page Head section
Sys.argv is a list in Python that contains command-line arguments passed to a script when it is executed. The first element (sys.argv[0]) is the script name, and subsequent elements (sys.argv[1:]) does the user pass the arguments.
You can access command-line arguments by importing the sys module and using sys.argv. The script name is always sys.argv[0], and the arguments follow in sys.argv[1], sys.argv[2], etc.
Here, arg1 and arg2 are the arguments passed to the script.
You can check the length of sys.argv to ensure the required number of arguments are passed. If not, you can print an error message and exit the script. if len(sys.argv) < 3: print("Please provide at least two arguments.") sys.exit(1)
Sys.argv is a simple way to capture command-line arguments, but it lacks advanced features like argument type validation, help messages and default values. For more complex argument parsing, Python's argparse module is recommended as it provides a more robust solution.
No, sys.argv does not support optional arguments directly. To handle optional arguments or flags, you would need to implement custom logic or use the argparse module, which is designed for more complex command-line argument parsing.