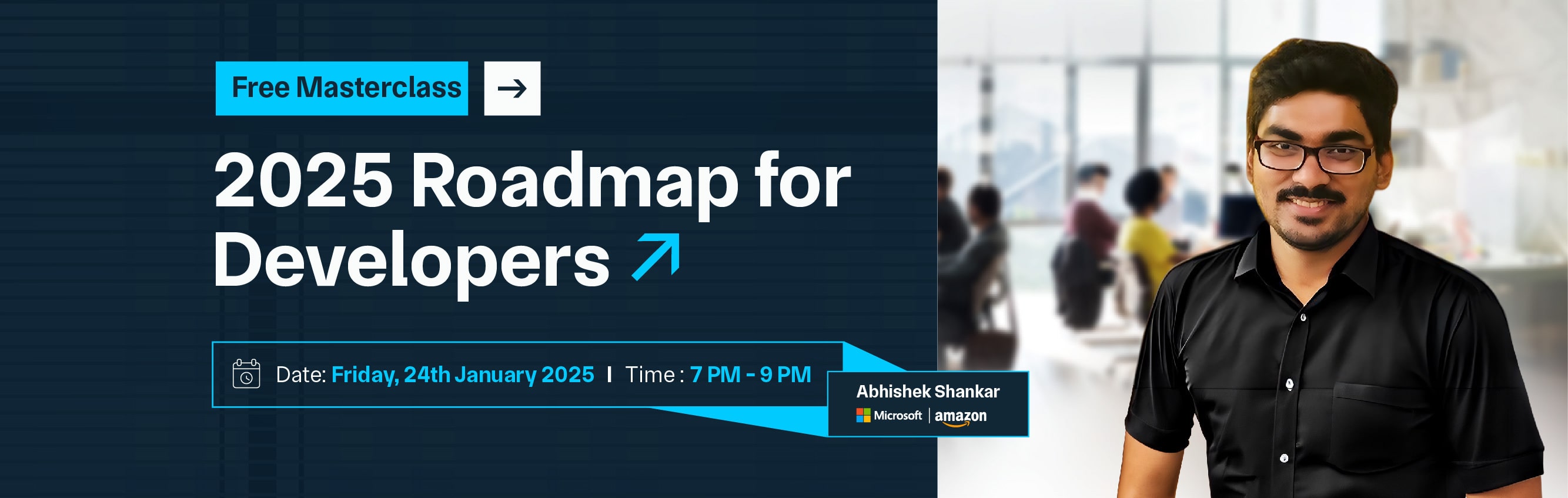

String operations are fundamental in data structures, as strings are a primary data type used in programming and algorithms. Key operations include creation, manipulation, searching, and comparison. Creating strings involves allocating memory and initializing values, while manipulation encompasses tasks like concatenation, substring extraction, and replacement. These operations often leverage data structures such as arrays or linked lists to manage character sequences efficiently.
Searching for specific patterns within strings can be performed using algorithms like the Knuth-Morris-Pratt or Boyer-Moore, which enhance performance over naive methods. Comparing strings is another critical operation, often implemented using lexicographical order, which is vital for tasks such as sorting and organizing data. String length and traversal are also essential, enabling access to individual characters and facilitating operations like reversing or trimming.
Moreover, the choice of data structure can significantly impact the efficiency of string operations. For instance, using tries for prefix searching can provide faster lookup times than traditional arrays. As strings are prevalent in applications ranging from text processing to databases, understanding these operations is crucial for effective programming and algorithm design, ensuring optimal performance and resource management in software development.
A string is a data structure used to represent a sequence of characters. It is commonly used in programming to handle text and can include letters, numbers, symbols, and whitespace. Here are some key points about string data structures:
1. Immutability vs. Mutability:
2. Character Encoding: Strings are encoded using various character encoding schemes, with UTF-8 and ASCII being the most common. This affects how characters are represented in memory.
3. Operations: Common operations on strings include:
4. Memory Allocation: Strings can be allocated statically (fixed size) or dynamically (variable size), depending on the programming language and implementation.
5. Use Cases: Strings are widely used for text processing, user input, file handling, and in data formats like JSON or XML.
Overall, strings are a fundamental data structure in computer science and programming, essential for manipulating and representing textual data.
Strings are a fundamental data type in programming and are often manipulated using various operations in data structures. Here are some common operations and concepts related to strings:
Strings can be created in various ways:
Literals: Directly using quotes.
s1 = "Hello, World!"
Constructors: Using a constructor in some languages (e.g., String in Java).
java
Copy code
String s2 = new String("Hello");
Concatenation is the operation of joining two or more strings.
Using the + operator:
s1 = "Hello, "
s2 = "World!"
result = s1 + s2 # "Hello, World!"
Using join() method:
result = ''.join([s1, s2]) # "Hello, World!"
You can extract parts of a string using slicing.
Basic slicing:
s = "Hello, World!"
sub = s[7:12] # "World"
Negative indexing:
sub = s[-6:] # "World!" (from the end)
Finding the number of characters in a string.
Using len() function:
length = len(s) # 13 for "Hello, World!"
Finding the position of a substring.
Using find():
index = s.find("World") # 7
Using in keyword:
exists = "World" in s # True
A binary string is a sequence of characters consisting only of the digits 0 and 1. This format is foundational in computing because it represents binary data, which is the fundamental language of computers.
Binary strings are exclusively made up of the characters 0 and 1. Each character represents a bit, the smallest unit of data in a computer.
The length of a binary string can vary depending on how much data it needs to represent. For example, the string 1101 has a length of 4.
Binary strings can represent various types of data:
Binary strings, which are sequences of bits (0s and 1s), have various uses across computer science, data processing, and telecommunications. Here are some key applications and uses of binary strings:
Binary strings are fundamental for representing various types of data in computing. Numbers, both integers and floating-point values, are commonly stored as binary strings, enabling efficient processing by computers. For example, the binary string 1010 corresponds to the decimal number 10.
Additionally, characters in text are encoded using binary representations through schemes like ASCII or UTF-8, where each character is mapped to a specific binary string. This encoding allows computers to store, manipulate, and display text effectively.
In the realm of data storage and transmission, binary strings play a critical role in data compression algorithms. These algorithms, such as Huffman coding, utilize binary strings to reduce the size of data files by encoding frequently occurring patterns with shorter binary representations.
This efficient use of binary strings minimizes the amount of space required for storage. It enhances the speed of data transmission over networks, making them essential in applications where bandwidth is a concern.
Binary strings are crucial in ensuring data integrity during transmission through the use of error detection and correction codes. Techniques like checksums and Hamming codes employ binary strings to identify and correct errors that may occur during data transfer.
By adding extra bits to the original binary string, these methods enable the detection of discrepancies and, in many cases, allow for the reconstruction of the original data, thus enhancing reliability in communication systems.
In the field of cryptography, binary strings represent keys, encrypted messages, and hash values. Cryptographic algorithms, both symmetric and asymmetric, operate on these binary strings to secure data from unauthorized access.
The binary representation allows for complex mathematical operations, such as bitwise manipulations, which are fundamental to creating secure encryption schemes. This use of binary strings is critical for safeguarding sensitive information in digital communications.
Binary strings are the backbone of data transmission in network protocols such as TCP/IP. These protocols use binary strings to structure headers, payloads, and control information, enabling reliable communication between devices over the internet.
The binary representation of data ensures that it can be efficiently processed by networking hardware and software, facilitating the transfer of information across different network layers.
In machine learning and artificial intelligence, binary strings can represent features or attributes in binary classification tasks. For example, a binary string may encode the presence (1) or absence (0) of specific traits or characteristics relevant to the model being trained.
This binary representation allows algorithms to efficiently process and analyze large datasets, contributing to the development of predictive models and decision-making systems.
Here are some common problems and challenges related to palindrome strings, along with explanations and examples:
Problem: Determine whether a given string is a palindrome, meaning it reads the same backward as forward.
Input: "racecar"
Output: True
def is_palindrome(s):
return s == s[::-1]
print(is_palindrome("racecar")) # Output: True
Problem: Given a string, find the longest substring that is a palindrome.
Input: "bad"
Output: "bab" or "aba" (both are valid)
def longest_palindrome(s):
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
Return right - left - 1
start, end = 0, 0
for i in range(len(s)):
len1 = expand_around_center(i, i) # Odd length
len2 = expand_around_center(i, i + 1) # Even length
length = max(len1, len2)
If length > end - start:
start = i - (length - 1) // 2
end = i + length // 2
return s[start:end + 1]
print(longest_palindrome("babad")) # Output: "bab" or "aba"
Problem: Count how many substrings of a given string are palindromes.
Input: "ABC"
Output: 3 (The palindromic substrings are: "a", "b", "c")
def count_palindromic_substrings(s):
count = 0
def expand_around_center(left, right):
nonlocal count
while left >= 0 and right < len(s) and s[left] == s[right]:
count += 1
left -= 1
right += 1
for i in range(len(s)):
expand_around_center(i, i) # Odd length palindromes
expand_around_center(i, i + 1) # Even length palindromes
return count
print(count_palindromic_substrings("abc")) # Output: 3
Problem: Given a string containing parentheses and lowercase letters, remove the minimum number of invalid parentheses to make the string a palindrome.
Input: "(a)b(c)(c)a"
Output: "(a)b(c)a" or similar valid palindromic formations.
Problem: Given a string, partition it such that every substring is a palindrome. Return all possible palindrome partitioning.
Input: "aab"
Output: [["a," "a," "b"], ["aa," "b"]]
def is_palindrome(s):
return s == s[::-1]
def backtrack(start, path):
if start == len(s):
result.append(path)
return
for end in range(start + 1, len(s) + 1):
substring = s[start:end]
if is_palindrome(substring):
backtrack(end, path + [substring])
result = []
s = "aab"
backtrack(0, [])
print(result) # Output: [["a," "a," "b"], ["aa," "b"]]
Strings are represented in memory in various ways, depending on the programming language and the string's characteristics. Here’s a detailed overview of how strings are stored and managed in memory:
Strings are stored as sequences of characters, each represented by a specific encoding scheme. Common encoding schemes include:
Strings are typically stored in contiguous blocks of memory. The representation can vary based on mutability:
Example: In languages like Java and Python, strings are immutable. Once a string is created, it cannot be modified.
Memory Representation:
Memory Representation:
In languages like C, strings are represented as arrays of characters terminated by a null character (\0). This indicates the end of the string.
char str[] = "Hello"; // Stored as ['H,' 'e,' 'l,' 'l,' 'o', '\0']
Some languages (like Java) use a concept called string interning, which stores only one copy of each distinct string value in memory. If two strings have the same value, they reference the same memory location.
In addition to storing the characters, some string implementations also store the length of the string for quick access. This helps optimize operations like slicing and concatenation.
Python:
Java:
C:
Here’s how to declare strings in various programming languages:
In Python, strings can be declared using single quotes, double quotes, or triple quotes for multi-line strings.
# Single quotes
s1 = 'Hello, World!'
# Double quotes
s2 = "Hello, World!"
# Triple quotes (multi-line)
s3 = """ This is a multi-line string.
It can span multiple lines."""
In Java, strings are declared using double quotes. The String class is used for string manipulation.
String s1 = "Hello, World!";
In JavaScript, strings can be declared using single quotes, double quotes, or backticks for template literals.
// Single quotes
let s1 = 'Hello, World!';
// Double quotes
let s2 = "Hello, World!";
// Template literals (allows interpolation and multi-line)
let s3 = `Hello,
World!`;
In C++, strings can be declared using the std::string class or as C-style strings using character arrays.
#include <string>
// Using std::string
std::string s1 = "Hello, World!";
// C-style string
char s2[] = "Hello, World!";
In C#, strings are declared using double quotes, similar to Java.
string s1 = "Hello, World!";
In Ruby, strings can be declared using single quotes or double quotes.
# Single quotes
s1 = 'Hello, World!'
# Double quotes
s2 = "Hello, World!"
In PHP, strings can be declared using single quotes or double quotes. Double quotes allow for variable interpolation.
// Single quotes
$s1 = 'Hello, World!';
// Double quotes
$s2 = "Hello, World!";
In Swift, strings are declared using double quotes.
var s1 = "Hello, World!"
In Go, strings can be declared using double quotes for regular strings or backticks for raw strings (which can include line breaks).
// Regular string
s1 := "Hello, World!"
// Raw string
s2 := `Hello,
World!`
In Kotlin, strings are declared using double quotes, similar to Java.
val s1 = "Hello, World!"
Here’s a detailed overview of common string functions across various programming languages, including their purpose and examples.
Length: len()
s = "Hello"
length = len(s) # Output: 5
Concatenation: +
s1 = "Hello"
s2 = "World"
result = s1 + " " + s2 # Output: "Hello World"
Substring: Slicing
substring = s[1:4] # Output: "ell"
Uppercase: upper()
upper_s = s.upper() # Output: "HELLO"
Lowercase: lower()
lower_s = s.lower() # Output: "hello"
Find: find()
index = s.find("l") # Output: 2
Replace: replace()
new_s = s.replace("l", "p") # Output: "Heppo"
Split: split()
words = s.split(" ") # Output: ['Hello']
Join: join()
words = ['Hello', 'World']
joined = " ".join(words) # Output: "Hello World"
Length: length()
String s = "Hello";
int length = s.length(); // Output: 5
Concatenation: +
String s1 = "Hello";
String s2 = "World";
String result = s1 + " " + s2; // Output: "Hello World"
Substring: substring()
String sub = s.substring(1, 4); // Output: "ell"
Uppercase: toUpperCase()
String upper_s = s.toUpperCase(); // Output: "HELLO"
Lowercase: toLowerCase()
String lower_s = s.toLowerCase(); // Output: "hello"
Find: indexOf()
int index = s.indexOf("l"); // Output: 2
Replace: replace()
String new_s = s.replace("l", "p"); // Output: "Heppo"
Split: split()
String[] words = s.split(" "); // Output: ["Hello"]
Join String. join()
String[] words = {"Hello", "World"};
String joined = String.join(" ", words); // Output: "Hello World"
Length: .length
let s = "Hello";
let length = s.length; // Output: 5
Concatenation: +
let s1 = "Hello";
let s2 = "World";
let result = s1 + " " + s2; // Output: "Hello World"
Substring: substring()
let sub = s.substring(1, 4); // Output: "ell"
Uppercase: toUpperCase()
let upper_s = s.toUpperCase(); // Output: "HELLO"
Lowercase: toLowerCase()
let lower_s = s.toLowerCase(); // Output: "hello"
Find: indexOf()
let index = s.indexOf("l"); // Output: 2
Replace: replace()
let new_s = s.replace("l", "p"); // Output: "Heppo"
Split: split()
let words = s.split(" "); // Output: ["Hello"]
Join: join()
Let words = ["Hello," "World"];
let joined = words.join(" "); // Output: "Hello World"
Length: .length() (for std::string)
std::string s = "Hello";
int length = s.length(); // Output: 5
Concatenation: +
std::string s1 = "Hello";
std::string s2 = "World";
std::string result = s1 + " " + s2; // Output: "Hello World"
Substring: substr()
std::string sub = s.substr(1, 3); // Output: "ell"
Uppercase: transform()
std::string upper_s = s;
std::transform(upper_s.begin(), upper_s.end(), upper_s.begin(), ::toupper); // Output: "HELLO"
Lowercase: Similar to uppercase using transform().
Find: find()
size_t index = s.find("l"); // Output: 2
Replace: replace()
std::string new_s = s;
std::replace(new_s.begin(), new_s.end(), 'l', 'p'); // Output: "Heppo"
Length: Length
string s = "Hello";
int length = s.Length; // Output: 5
Concatenation: +
string s1 = "Hello";
string s2 = "World";
string result = s1 + " " + s2; // Output: "Hello World"
Substring: Substring()
string sub = s.Substring(1, 3); // Output: "ell"
Uppercase: ToUpper()
string upper_s = s.ToUpper(); // Output: "HELLO"
Lowercase: ToLower()
string lower_s = s.ToLower(); // Output: "hello"
Find: IndexOf()
int index = s.IndexOf("l"); // Output: 2
Replace: Replace()
string new_s = s.Replace("l", "p"); // Output: "Heppo"
Split: Split()
string[] words = s.Split(' '); // Output: ["Hello"]
Strings are fundamental data types that support various operations. Here are some common operations that can be performed on strings:
Concatenation is the process of joining two or more strings together to form a single string. This operation is often used to combine user inputs or to build dynamic messages.
Example: In Python, you can concatenate strings using the + operator:
greeting = "Hello" + " " + "World" # Results in "Hello World"
Slicing allows you to extract a portion of a string. This is useful for retrieving specific characters or sequences from a string.
Example: In Python:
s = "Hello, World!"
sub = s[7:12] # Extracts "World"
Searching involves finding the position of a substring within a string. This can help determine if a string contains specific text.
Example: Using the find() method in Python:
Index = s.find("World") # Returns 7, the starting index of "World"
Replacing substitute occurrences of a substring with another substring. This is useful for modifying text or correcting errors.
Example: In Python:
new_s = s.replace("World", "Python") # Results in "Hello, Python!"
Converting strings to uppercase or lowercase is commonly used for case-insensitive comparisons or formatting.
Example: In Python:
upper_s = s.upper() # Results in "HELLO, WORLD!"
lower_s = s.lower() # Results in "Hello, world!"
Trimming removes whitespace or specified characters from the beginning and end of a string. This helps clean user inputs or data from external sources.
Example: In Python:
trimmed = " Hello ".strip() # Results in "Hello"
Splitting divides a string into a list or array of substrings based on a specified delimiter. This is useful for processing comma-separated values or space-separated words.
Example: In Python:
words = "a,b,c".split(",") # Results in ['a', 'b', 'c']
Joining combines a list or array of strings into a single string with a specified delimiter. This is useful for formatting output or preparing data for display.
Example: In Python:
joined = " ".join(['Hello', 'World']) # Results in "Hello World"
Determining the length of a string is often necessary for validation or iteration purposes.
Example: In Python:
length = len("Hello") # Results in 5
Reversing a string creates a new string that is the reverse of the original. This can be useful in certain algorithms or puzzles.
Example: In Python:
reversed_s = s[::-1] # Results in "!dlroW, college"
Strings are used in a wide variety of applications across different fields. Here are some common applications:
Strings are essential for handling and manipulating textual data in applications like word processors, text editors, and online platforms where text input and formatting are crucial.
Strings are often used to serialize data for storage or transmission, particularly in formats like JSON and XML, which are widely used in APIs and data exchange between systems.
In user interfaces, strings capture and process user input. Form fields on websites and applications typically accept string inputs, requiring validation and processing.
Strings are vital for writing code, displaying messages, and including comments. They are used extensively in scripting and programming for variable names and output.
Strings are used to read from and write to files, handling file names, paths, and contents. This is crucial for data storage and manipulation in many applications.
In networking applications, strings are used to represent URLs, headers, and data packets, facilitating communication between servers and clients.
Strings are integral to formulating SQL queries and interacting with databases, enabling data retrieval, updates, and management.
In NLP applications, strings are fundamental for analyzing, processing, and generating human language, playing a key role in machine learning and AI technologies.
Strings are involved in cryptographic algorithms for securing data, including hashing and encryption processes that protect sensitive information.
String operations are essential in search algorithms, used in search engines and database functionalities to perform pattern matching and retrieval of information.
Strings are a fundamental data type in programming, and they offer several advantages that make them essential for various applications. Here are some key benefits of using strings:
Strings are easily readable and understandable by humans, making them ideal for displaying messages, prompts, and outputs. This readability is crucial in user interfaces and documentation.
Strings can store a wide range of data types, including letters, numbers, and symbols. This versatility allows developers to use strings for various purposes, from simple text to complex structured data.
Most programming languages provide a rich set of functions and methods for manipulating strings, such as concatenation, slicing, searching, and replacing. This makes it easy to perform common operations without complex algorithms.
Strings are commonly used for data interchange between systems, especially in formats like JSON and XML. Their ability to represent structured data as text makes them suitable for APIs and web services.
Strings allow for dynamic content generation, enabling developers to create messages, labels, and notifications based on user input or system state. This is essential for building interactive applications.
While strings are a fundamental and versatile data type, they also come with several disadvantages that developers should be aware of. Here are some key drawbacks of using strings:
In languages like Java and Python, strings are immutable, meaning that any modification (like concatenation or replacement) creates a new string rather than altering the original. This can lead to performance issues and increased memory usage, especially when manipulating large strings.
Strings can consume a significant amount of memory, particularly if they are long or if many temporary strings are created during manipulation. This overhead can affect performance in memory-constrained environments.
Operations such as concatenation in immutable string languages can be inefficient. Each operation creates a new string, leading to increased time complexity (e.g., O(n) for each concatenation), which can slow down applications that perform numerous string manipulations.
Strings can only represent textual data, which may require conversion or additional parsing for numerical or binary data. This limitation can complicate data handling in applications that need to process various data types.
Strings used in SQL queries or command-line executions can expose applications to injection attacks (e.g., SQL injection) if not properly sanitized. This poses security risks, requiring developers to implement additional measures for data validation and escaping.
Strings are a fundamental data structure widely used in programming and computer science. Their versatility allows for the representation and manipulation of textual data, making them essential for various applications, from user interfaces to data processing and storage.
Copy and paste below code to page Head section
A string is a sequence of characters used to represent text. It can include letters, numbers, symbols, and whitespace. Strings are a fundamental data type in most programming languages.
Strings are typically stored in memory as arrays of characters. Depending on the language, strings may be immutable (like in Java and Python) or mutable (like in C and C++), affecting how they are manipulated.
Common operations include: Concatenation Substring extraction Searching for substrings Replacing characters or substrings Converting case (uppercase/lowercase) Trimming whitespace Splitting into an array of substrings Joining an array into a single string
Advantages include: Readability and ease of understanding Flexibility to represent various data Rich built-in functions for manipulation Compatibility with data interchange formats like JSON and XML
Disadvantages include: Memory overhead, especially with large strings Performance issues due to immutability in some languages Vulnerability to injection attacks if not properly handled Complexity in encoding and validation
In languages where strings are immutable, consider using specific functions or classes designed for efficient concatenation (e.g., StringBuilder in Java or StringIO in Python). This reduces performance overhead associated with creating multiple intermediate strings.