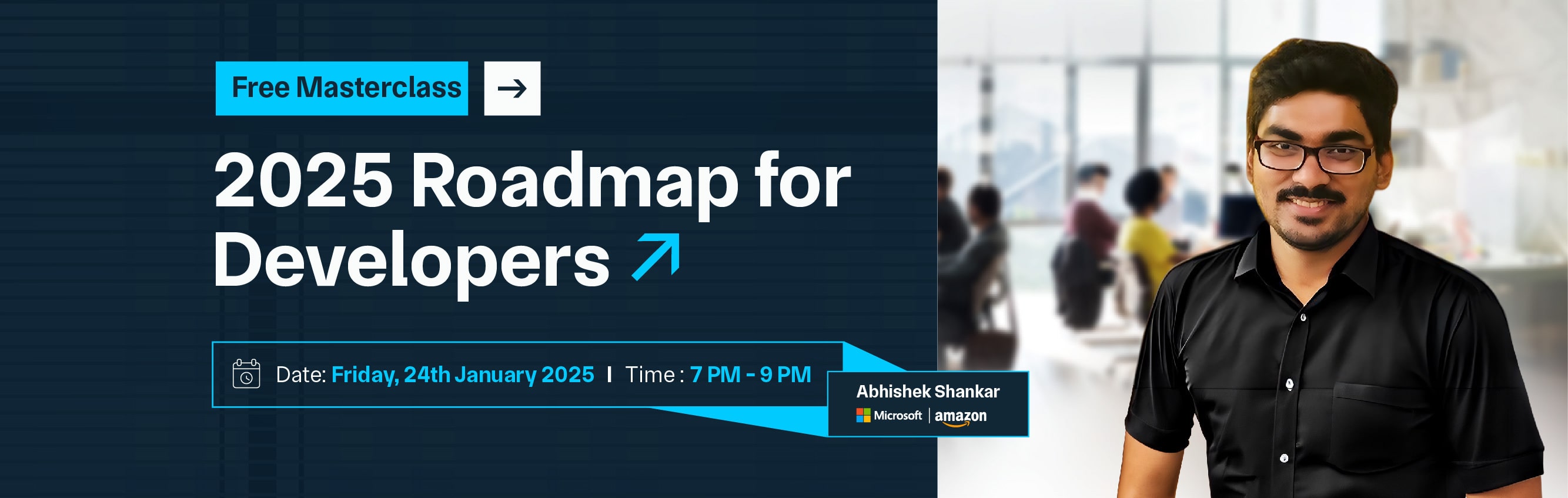

A Software Engineering Project with source code provides developers with practical, hands-on experience to enhance their skills and knowledge in real-world scenarios. These projects typically span various domains, from web development and mobile apps to machine learning and artificial intelligence. With source code included, developers can study the underlying architecture, design patterns, and implementation strategies used in each project.
For beginners, projects might focus on simple applications like a to-do list app, calculator, or a basic website, helping developers understand fundamental programming concepts. Advanced projects, such as content management systems (CMS), e-commerce platforms, or machine learning models, offer more complex challenges that involve problem-solving, algorithm optimization, and working with different technologies.
By exploring these projects, developers not only learn how to code but also how to manage the software development life cycle, including planning, design, coding, testing, and deployment. The availability of source code also enables modification, customization, and integration with other tools, fostering creativity and innovation. Additionally, contributing to open-source projects or building personal portfolios from these examples can be a great way to showcase skills to potential employers or collaborators. Ultimately, software engineering projects with source code are valuable resources for learning, practicing, and mastering the craft of software development.
A Software Engineering Project is a practical, structured initiative aimed at designing, developing, testing, and maintaining software applications or systems. It involves applying engineering principles to create reliable, efficient, and scalable software solutions that meet specific user needs or solve real-world problems. These projects follow a systematic approach that typically includes stages like planning, design, coding, testing, deployment, and maintenance. Software engineering projects can vary in complexity and scope, from small applications to large-scale enterprise systems.
They can be developed for various platforms, including web, mobile, desktop, or cloud. The goal of these projects is not only to write functional code but to do so in a way that ensures software quality, usability, and performance. A typical software engineering project follows methodologies like Agile, Waterfall, or DevOps, which guide the development process, project management, and team collaboration.
These projects often require a combination of programming skills, problem-solving abilities, and an understanding of system architecture, databases, and user interfaces. Overall, a software engineering project can be a solo effort or a collaborative team effort, often involving multiple stages and roles, including project managers, developers, testers, and designers. The outcome is a well-structured software product or system that fulfills its intended purpose and meets the desired quality standards.
1. To-Do List Application
2. Calculator App
3. Personal Blog Website
4. Number Guessing Game
5. Simple Weather App
6. Expense Tracker
7. Basic Chat Application
8. Simple Contact Manager
9. Quiz Application
10. Countdown Timer
11. Basic Portfolio Website
12. Flashcard App
13. To-Do List with Reminders
14. Recipe Finder
15. Movie Database App
16. Unit Converter
17. Tic-Tac-Toe Game
18. Pomodoro Timer
19. Expense Splitter
20. Flashlight App
21. Note-Taking App
22. Password Manager
23. Personal Finance Tracker
24. Music Player
25. Weather Forecasting App
26. Online Store (E-commerce)
27. Job Search Application
28. Currency Converter
29. Flashcards with Progress Tracking
30. Social Media Dashboard
31. Booking System (Hotel or Event)
32. Markdown Editor
33. Multi-User Blog
34. Online Polling System
35. Travel Planning App
Beginner projects are great for building a solid foundation in software development. These projects typically focus on basic programming concepts such as variables, loops, conditionals, functions, and simple data structures.
They help you get comfortable with coding, debugging, and working with user interfaces. Completing these projects will allow you to develop a portfolio and gain confidence before tackling more complex applications.
A To-Do List Application is a simple app where users can add tasks, mark them as completed, and delete them. The app stores tasks locally (or in a database for more complex systems). It teaches essential concepts such as CRUD operations (Create, Read, Update, Delete), handling user input, and storing data persistently.
You can add features like due dates, priorities, or categories. It’s a great project to practice JavaScript and front-end development or even back-end if using a server and database.
Source Code: Click Here
A Calculator App is a basic application that allows users to perform arithmetic operations, such as addition, subtraction, multiplication, and division. This project will help you learn how to handle user input, create buttons, and implement arithmetic logic.
It's also an excellent opportunity to practice using event listeners and manipulating the Document Object Model (DOM) to display results. You can extend the app with more complex operations like square roots, exponents, or even graphing capabilities, making it a versatile learning tool.
Source Code: Click Here
A Personal Blog Website is a static website where users can read blog posts. You can start by designing a layout with HTML and CSS and later add JavaScript for interactivity, such as comment sections or dynamic content.
If you add a backend (e.g., Node.js, PHP), the site could allow users to submit posts and comments. This project helps you get familiar with web development, basic design principles, and user experience. It’s also a good starting point for building a personal portfolio or content management system.
Source Code: Click Here
In a Number Guessing Game, the computer selects a random number, and the user tries to guess it. The app provides feedback on whether the guess is too high or too low. It’s an excellent beginner project for learning about random number generation, loops, conditionals, and user input handling.
You can add features like a limited number of guesses, a scoreboard, or different difficulty levels, which would make the game more engaging and provide additional challenges for you to solve.
Source Code: Click Here
A Simple Weather App allows users to enter a location and view the current weather conditions. It fetches data from a public API, such as OpenWeatherMap, and displays temperature, humidity, wind speed, and other relevant data.
This project introduces you to working with APIs, making asynchronous HTTP requests (using fetch() or Axios), and processing JSON data. Additionally, you will practice dynamically updating the user interface and handling errors (like invalid city names), which are crucial skills in full-stack development.
Source Code: Click Here
An Expense Tracker is an app that helps users track their income and expenses. It allows users to categorize their expenses, set budgets, and view reports to analyze their spending habits. You can store data using local storage or databases and implement CRUD operations.
This project will teach you how to design forms, validate user input, calculate totals, and store information persistently. If you add a user authentication feature, it could be a great way to learn backend development and integrate APIs for currency conversion.
Source Code: Click Here
A Basic Chat Application allows users to send and receive messages in real time. You can create a simple chat room where users can join and interact with each other. This project introduces you to client-server communication, working with WebSockets or libraries like Socket.io, and real-time data synchronization.
You will practice handling events, updating the UI dynamically, and using back-end technologies to store messages and user data. If you implement features like private messaging or file sharing, it will provide additional challenges to solve.
Source Code: Click Here
A Simple Contact Manager allows users to add, edit, view, and delete contacts. Each contact typically has information like name, phone number, and email. This app is an excellent way to practice CRUD operations, form handling, and data management.
You can start with local storage for data persistence or integrate a database for scalability. Additional features include search functionality, contact categorization, or a way to import/export contacts. It's an ideal project to hone skills in user interface design and data organization.
Source Code: Click Here
A Quiz Application allows users to answer multiple-choice questions and calculates a score at the end. You can store questions and answers in arrays or objects and use conditionals to check whether the user’s response is correct.
This project helps you practice working with data structures like arrays and objects, using loops to iterate over questions, and managing user input. Advanced features could include a timer, a scoring system, question categories, and a leaderboard. It’s a fun and interactive way to improve JavaScript skills.
Source Code: Click Here
A Countdown Timer app allows users to set a timer for a specific duration, and the timer counts down to zero. Once the time is up, it can alert the user with a sound or notification.
This project is an excellent way to learn how to handle date and time in JavaScript, work with setInterval or setTimeout, and dynamically update the UI. You can enhance this app by adding features like recurring timers, alarm sounds, or the ability to pause and resume the countdown.
Source Code: Click Here
A Basic Portfolio Website is a personal website that showcases your skills, projects, and achievements. It’s essential for building an online presence, especially if you’re a developer or designer. Using HTML, CSS, and JavaScript, you can create a responsive, visually appealing site.
This project will help you refine your design and web development skills. You can also add interactive elements like modals or a contact form. Later, you might extend the site to include dynamic content or a backend (e.g., to manage project details).
Source Code: Click Here
A Flashcard App allows users to create, view, and study flashcards, typically with questions on one side and answers on the other. This is a simple but effective way to learn about handling data structures, user input, and creating dynamic content.
You could store flashcards in an array or a database and implement features like shuffling cards, reviewing wrong answers, or tracking progress. It’s a great app to practice JavaScript and explore concepts like local storage or even spaced repetition algorithms for more advanced learning features.
Source Code: Click Here
A To-Do List with Reminders is an enhanced version of a basic to-do list app, allowing users to set reminders for each task. The app will notify the user when a task is due. It’s an excellent project to practice handling dates and times, setting notifications, and integrating with APIs for push notifications or alarms.
You can extend the app by adding recurring tasks, categorization, and filtering options. This project combines front-end UI development with more complex logic around reminders and time management.
Source Code: Click Here
A Recipe Finder app allows users to input ingredients they have, and it provides recipes they can make with those ingredients. This app fetches recipe data from an API (like Spoonacular) and displays the results.
The project introduces API integration, dynamic data rendering, and user input handling. You’ll learn how to make HTTP requests, process JSON responses, and display filtered data based on user input. Advanced features might include saving favorite recipes, creating a shopping list, or adding user-generated recipes.
Source Code: Click Here
A Movie Database App uses a public API (such as OMDB or TMDB) to fetch information about movies, including titles, genres, ratings, and descriptions. It allows users to search for movies, view details, and even create a watchlist.
This project teaches API integration, asynchronous programming, and working with JSON data. You’ll practice creating an intuitive user interface, displaying dynamic content, and handling user inputs. Enhancements can include pagination, sorting, and filtering results based on user preferences.
Source Code: Click Here
A Unit Converter app helps users convert units between different measurement systems (e.g., miles to kilometers, Celsius to Fahrenheit). This project helps you practice handling numbers, basic math operations, and designing interactive forms.
The app can include various conversion categories such as length, weight, temperature, or volume. It teaches you about user input validation and error handling. Adding extra functionality like saving frequent conversions or integrating a currency conversion API could further enhance the app and make it more useful.
Source Code: Click Here
Tic-Tac-Toe is a two-player game where players take turns marking spaces on a 3x3 grid, and the first to get three in a row wins. This game helps you practice game logic conditionals and update the UI dynamically.
You’ll learn how to check for a winner, manage player turns, and implement a reset feature. You can further extend this project by implementing an AI opponent using basic algorithms or adding a scoring system to keep track of wins and losses.
Source Code: Click Here
A Pomodoro Timer is a productivity tool based on the Pomodoro Technique, which divides work into intervals (usually 25 minutes) separated by short breaks. The app shows a countdown timer for work and break periods, helping users stay focused.
This project is great for learning how to handle time-based events, such as using setInterval and setTimeout. You’ll also practice building a user interface with interactive elements like start, stop, and reset buttons. Additional features might include customizable work/break intervals and notification alerts.
Source Code: Click Here
An Expense Splitter helps groups of people divide shared costs evenly. For example, after a dinner or a trip, the app calculates how much each person owes. It also helps manage individual contributions and displays a summary of the total amount.
This project involves practicing math operations, handling user input, and working with arrays or objects to store people’s names and amounts. You can add features like tracking who paid for what and supporting multiple currencies, making it more complex and practical.
Source Code: Click Here
A Flashlight App turns on and off the device’s flashlight. This project uses mobile hardware (for mobile apps) or JavaScript libraries (for web apps) to access the device’s flashlight feature. You’ll learn how to interact with a device’s native hardware and create a simple UI with buttons to control the flashlight.
While simple, this project introduces important concepts in mobile or hardware interaction. It’s especially useful for developers working with mobile apps and native device features, like cameras or sensors.
Source Code: Click Here
Intermediate projects are designed to bridge the gap between beginner and expert-level applications. These projects require a solid understanding of basic programming concepts but introduce more complexity through features like databases, APIs, authentication, and multi-component systems.
They often involve real-world problems that require you to integrate both front-end and back-end technologies, making them ideal for developers aiming to strengthen their full-stack development skills.
A Note-Taking App allows users to create, edit, delete, and organize notes in a user-friendly interface. It typically supports text formatting and may include features like categorization or tagging for better organization.
This app introduces concepts like local storage (for saving notes), CRUD operations (Create, Read, Update, Delete), and managing large datasets. You can further enhance it by adding a search feature, data encryption for security, or syncing across multiple devices using a backend.
Source Code: Click Here
A Password Manager securely stores and retrieves passwords for websites and services. The app typically encrypts passwords to protect user data, making it ideal for learning about security, encryption algorithms (like AES or RSA), and hashing techniques (such as crypt).
Features include the ability to auto-fill login details, generate secure passwords, and safely store them in a database or local storage. This project is an excellent way to dive deeper into handling sensitive data and understanding data security practices.
Source Code: Click Here
A Personal Finance Tracker helps users manage their income, expenses, savings, and investments. It allows for categorizing transactions, generating reports, and visualizing financial data in charts.
This project introduces working with numbers, managing data, and integrating APIs for real-time financial data (like stock market prices or exchange rates). Advanced features include setting financial goals, creating a budget, and tracking progress toward savings targets.
Source Code: Click Here
A Music Player app lets users play music files, create playlists, and control playback features like play, pause, next, previous, volume, and shuffle. It requires working with multimedia APIs, handling file input/output, and building a responsive user interface.
You can also add additional features like an equalizer, a music discovery feature (e.g., integration with a music API like Spotify), or a feature to download music. It’s a good project for learning about media controls and designing rich, interactive UI elements.
Source Code: Click Here
A Weather Forecasting App provides users with the weather forecast for the next few days. It fetches weather data from an external API like OpenWeatherMap or AccuWeather, displaying current weather, future predictions, humidity, temperature, and wind speed.
The project introduces you to working with APIs, parsing JSON data, and dynamically updating the UI. You can further enhance it by adding location-based features, maps, or different themes based on weather conditions (e.g., dark mode for stormy weather).
Source Code: Click Here
An Online Store or E-commerce platform allows users to browse products, add them to a cart, and complete purchases. It includes features like user authentication (sign-up/login), a product catalog, a shopping cart, and order processing.
You’ll work with back-end technologies like databases for managing products and orders, and integrate payment gateways like Stripe or PayPal. This project helps you learn how to build complex user interfaces, handle transactions, and manage a database effectively, making it perfect for anyone wanting to learn full-stack development.
Source Code: Click Here
A Job Search Application lets users search for job listings, apply for jobs, and save job searches. The app fetches job data from APIs like LinkedIn or Indeed, allowing users to filter and search listings by location, industry, or salary range.
It introduces working with external APIs, handling search filters, and creating dynamic content. The app could be extended to allow users to upload resumes or manage job applications, making it a practical project for learning about job portals or career-related applications.
Source Code: Click Here
A Currency Converter allows users to convert money between different currencies based on real-time exchange rates. The app can use an API like Open Exchange Rates or XE to get the latest exchange rates and perform conversions.
This project teaches you how to work with APIs, manipulate numbers, and build simple but useful tools. You can enhance the app by adding historical data for exchange rates, converting multiple currencies at once, or even supporting cryptocurrency conversions.
Source Code: Click Here
A Flashcards app helps users memorize information (such as vocabulary or formulas) by displaying cards with questions on one side and answers on the other. The app tracks progress, helping users focus on areas they need to improve.
It can include features like spaced repetition, which is an algorithm that schedules flashcards based on how well the user remembers them. This project teaches you about data management, tracking user progress, and implementing algorithms for learning reinforcement.
Source Code: Click Here
A Social Media Dashboard aggregates posts, analytics, and insights from multiple social media platforms (e.g., Facebook, Twitter, Instagram) into a single interface. It allows users to track engagement metrics, manage posts, and analyze trends.
This project will help you work with social media APIs, authentication (OAuth), and building real-time dashboards. Features could include scheduling posts, analyzing user interactions, or generating detailed reports based on user activity, which makes it a good project for building complex, data-driven applications.
Source Code: Click Here
A Booking System allows users to book hotel rooms, event tickets, or other types of reservations. The app manages available dates, payment processing, user authentication, and booking history.
It involves working with databases to manage reservations and availability, integrating payment gateways, and creating user-friendly interfaces. Advanced features could include a calendar view for bookings, email notifications for reminders, or user-specific pricing. It’s a great way to learn about booking systems and multi-user management in a real-world context.
Source Code: Click Here
A Markdown Editor allows users to write and preview content written in Markdown, which is a lightweight markup language. The app typically features a live preview, where users can see how their Markdown will appear as formatted text.
This project teaches text parsing, dynamic rendering, and creating a user-friendly editor. You can also add features like saving files, exporting to PDF, or integrating with cloud services like Google Drive for file management.
Source Code: Click Here
A Multi-User Blog platform enables multiple users to write, edit, and publish blog posts. Features typically include user registration/login, role-based access (e.g., admin, editor, reader), and commenting. This project introduces you to user authentication, content management systems (CMS), and role-based permissions.
It’s also a good introduction to building full-stack web applications, as it typically involves a front-end interface, a back-end server (for authentication and managing posts), and a database (for storing user and post data).
Source Code: Click Here
Expert Software Engineering Projects are complex, high-level applications that require advanced knowledge of programming, algorithms, design patterns, and system architecture. These projects typically involve multiple technologies, frameworks, and tools and often focus on real-world challenges such as scalability, performance, security, and integration with external systems.
Expert projects push the boundaries of your skills, involving complex problem-solving, advanced database management, and, often, collaboration in team-based development environments. Completing these projects can significantly enhance your portfolio and demonstrate your ability to build sophisticated, production-ready software solutions.
An Online Polling System allows users to create polls, vote on them, and view results in real time. It typically includes features like multiple-choice questions, a voting mechanism, and real-time result updates. This project introduces concepts such as user authentication (if required), handling user input, dynamically updating results, and managing vote data.
It may require back-end technologies to store votes and manage poll data, making it ideal for learning full-stack development. You can further extend it by adding features like anonymous voting, limiting votes per user, or scheduling polls.
Source Code: Click Here
A Travel Planning App helps users organize their trips by offering features like creating itineraries, booking accommodations, and finding activities to do. The app can pull data from APIs for hotel bookings, flight information, weather forecasts, and tourist attractions. This project involves integrating various external APIs, handling dates, and organizing data in a structured way.
You could add features like a travel budget tracker, collaborative trip planning (for groups), or integration with maps for navigation. It's a great project for learning about managing dynamic content and building interactive, data-driven applications.
Source Code: Click Here
Programming Side Projects are personal, independent coding initiatives that developers work on outside of their primary job or academic commitments. These projects can be anything from simple scripts or applications to more complex software solutions. Side projects are usually self-directed and allow developers to experiment with new technologies, practice skills, or solve real-world problems they are passionate about.
Project-based learning (PBL) is considered one of the most effective methods of learning, especially in fields like software engineering, design, and technical disciplines. It focuses on applying theoretical knowledge to practical, real-world scenarios through the creation of projects. This hands-on approach offers several key benefits that contribute to deeper understanding, better retention, and skill development.
Technical skills acquired through software development projects are critical for building proficiency in coding, problem-solving, and developing robust, scalable applications. Working on real-world projects helps you gain a deeper understanding of concepts and technologies used in software development. Below are key technical skills you acquire through such projects:
Proficiency in programming languages such as Python, Java, JavaScript, C++, or Ruby is the foundation of software development. Working on projects allows you to strengthen your understanding of syntax, logic, and structure in these languages.
Familiarity with popular frameworks like Django (Python), React (JavaScript), Spring (Java), or Ruby on Rails helps developers speed up development while ensuring better code structure and maintainability.
Building software often involves solving algorithmic problems, optimizing performance, and improving the efficiency of code. You'll sharpen your skills in algorithm design, data structures (e.g., trees, graphs, linked lists), and complexity analysis (Big O notation).
Learning Git is essential for collaborative development. Version control systems like Git allow you to track changes, manage codebases, and collaborate effectively with other developers. GitHub or GitLab are often used for project hosting and collaboration.
Relational databases (e.g., MySQL, PostgreSQL) and NoSQL databases (e.g., MongoDB) are key components in most software projects. You'll learn SQL queries, data modeling, and database design while interacting with databases to store and retrieve data.
For web applications, you'll gain expertise in front-end technologies like HTML, CSS, JavaScript, and front-end frameworks like Angular or React, as well as back-end technologies like Node.js, Django, or Flask. API development (RESTful APIs, GraphQL) becomes crucial for communication between the front-end and back-end.
Writing unit tests, integration tests, and performing manual testing will deepen your understanding of quality assurance (QA). Tools like JUnit, Mocha, or Selenium can help automate testing, ensuring your software works as expected.
Debugging is a critical skill that helps you identify and fix issues in your code. You’ll learn how to use debugging tools like Chrome DevTools, Python’s pdb, or IDE debuggers to trace errors and optimize code performance.
Learning to design and architect software involves understanding design patterns (e.g., MVC, Singleton, Factory) and best practices. You'll also delve into principles like modularity, scalability, and maintainability when building large-scale applications.
Software development projects teach you how to integrate security features into your code. You’ll work with authentication and authorization (OAuth, JWT), data encryption (AES), and secure coding practices to protect applications from common vulnerabilities (e.g., SQL injection, XSS).
Knowledge of cloud platforms (AWS, Azure, Google Cloud) is vital for deploying and scaling applications. You’ll learn about containerization (Docker), continuous integration/continuous deployment (CI/CD), and automation tools like Jenkins or GitLab CI for seamless deployment.
For mobile projects, you'll acquire expertise in developing applications using native technologies (Swift for iOS, Kotlin for Android) or cross-platform frameworks (Flutter, React Native), including managing user interfaces, navigation, and device integration.
Many software projects require understanding networking concepts such as HTTP/HTTPS, TCP/IP, WebSockets, and RESTful API protocols. You may also work with web servers like Apache and Nginx or deploy to cloud servers (e.g., EC2 instances).
Working on software projects often involves using Agile development methodologies, such as Scrum or Kanban, to manage tasks, prioritize features, and deliver software incrementally. Tools like Jira, Trello, and Asana are commonly used for task management in teams.
With DevOps tools like Docker, Kubernetes, and Terraform, you’ll learn to automate infrastructure management, streamline deployment processes, and maintain code in production environments. Knowledge of containerization and orchestration is critical for modern software deployment.
While developing software, especially front-end or mobile apps, you’ll understand the importance of UI/UX principles. Skills like wireframing, prototyping, and using design tools (e.g., Figma, Sketch) will help you deliver visually appealing and user-friendly applications.
Optimizing code for speed, efficiency, and resource management is vital in software development. You’ll learn techniques such as caching, load balancing, memory management, and profiling tools to ensure high-performance applications.
Software development projects are essential for both budding developers and experienced professionals for several key reasons. They serve as the practical application of theoretical concepts, helping developers to solidify their understanding and improve their problem-solving abilities.
These projects allow developers to face real-world challenges and refine their skills in a collaborative and creative environment. Here are the main reasons why software development projects are important:
Software development projects provide the hands-on experience necessary to apply theoretical knowledge in real-world scenarios. Whether you are learning a new programming language, mastering a framework, or developing a full-fledged system, projects offer an opportunity to put your learning to the test. Without practical experience, it’s difficult to grasp complex concepts fully.
Every software project involves solving problems, from designing an intuitive user interface to optimizing performance. Working through these problems improves your critical thinking, logical reasoning, and debugging skills, which are fundamental in software development. Through trial and error, developers learn to identify, analyze, and resolve issues effectively.
A well-rounded portfolio of software projects showcases your skills to potential employers, clients, or collaborators. Demonstrating real, tangible results in your portfolio (such as apps, websites, or tools you've built) is often more impactful than a resume alone. It shows that you not only know the theory but can also execute ideas and deliver products.
Projects allow developers to bridge the gap between theoretical knowledge and real-world applications. The design, development, testing, and deployment of software in real-world conditions often involve unexpected challenges that require creative solutions. This provides valuable insight into the process of software development that cannot be fully simulated in textbooks or classroom environments.
Working on projects introduces you to a variety of tools, technologies, and best practices. As you build more projects, you are forced to learn and adapt to new technologies and methodologies, improving your technical stack. Whether it’s learning a new database system, mastering a framework, or experimenting with new design patterns, software development projects allow you to expand your skill set continuously.
Many software development projects, especially larger ones, require collaboration. Developers learn how to work in teams, communicate effectively, and share code using version control systems like Git. This enhances soft skills like communication, teamwork, and collaboration, which are critical in real-world software development, where developers often work with designers, project managers, and other stakeholders.
Through software projects, developers gain an understanding of the entire Software Development Lifecycle (SDLC): planning, design, development, testing, deployment, and maintenance. Each phase involves different techniques, tools, and best practices. Having practical experience in each of these stages ensures that developers are well-prepared to manage and contribute to the software development process from start to finish.
Software projects often involve multiple tasks that need to be completed within deadlines. Developers learn time management and project planning skills, such as breaking down a project into smaller tasks, setting milestones, and prioritizing. These skills are transferable to other professional areas, helping developers to become more efficient and organized in their work.
While software projects often involve solving specific problems, they also encourage creativity and innovation. Developers get the opportunity to design unique solutions, experiment with new technologies, and come up with fresh ideas. This fosters creativity, which is crucial not only for software development but also for staying ahead of the curve in a rapidly evolving field.
Building software projects keeps developers aligned with industry trends and emerging technologies. For example, by working on projects involving cloud computing, AI, or machine learning, developers gain experience with cutting-edge technologies that are in high demand. This relevance to current market needs makes developers more competitive and adaptable in the job market.
Completing a project boosts a developer's confidence and reinforces the idea that they can tackle challenges on their own. The satisfaction of seeing a project through to completion is a huge motivator. It provides a sense of accomplishment and encourages developers to continue learning and improving their skills.
Working on projects allows for feedback from peers, mentors, or even users. This feedback loop is valuable for identifying areas of improvement, refining code, and learning new techniques. Through iterative development, developers constantly improve their problem-solving abilities and coding practices.
Successful software projects can help propel a developer’s career forward. Whether it’s impressing a hiring manager with an innovative project, gaining recognition in the developer community by contributing to open-source projects, or simply demonstrating technical competence, project-based learning can accelerate career development.
Many software projects require developers to design solutions based on user needs and feedback. This helps developers understand the importance of user-centered design, usability, and user experience (UX). Understanding how to build products that are intuitive and meet the needs of the user is a crucial skill for any developer.
Developing a software project starts with selecting the right project topic. Choosing a relevant and engaging topic is crucial because it determines the scope, complexity, and learning opportunities associated with the project. Here’s a step-by-step guide on how to develop a software project topic:
Selecting a software project topic that aligns with your interests and existing strengths is crucial for staying motivated throughout the project. If you're passionate about a particular domain, such as education, gaming, or finance, you're more likely to remain engaged and invested in the outcome.
At the same time, consider your current skillset. If you're new to programming, choose a project that allows you to practice fundamental concepts without overwhelming yourself. For example, building a simple to-do list app might be perfect for beginners, while more advanced developers can take on full-stack web development projects or machine learning applications. This combination of interest and skill will make your project both enjoyable and an opportunity for growth.
A great software project often addresses real-world problems or improves existing solutions. Start by identifying pain points in everyday life or specific industries. For example, is there a manual task at work that could be automated with a small application? Is there a niche in the market where current software solutions could be improved?
By focusing on a real-world problem, your project gains immediate value and relevance. Research existing solutions to see if they meet user needs or if there’s room for innovation. This research phase is vital to identify gaps in current technology, ensuring your project solves a problem effectively and efficiently.
The scope and complexity of a project are crucial factors in determining its feasibility. It's important to choose a project that matches your current skill level and can be completed within your available time frame. Beginners should start with simple projects that focus on one or two key features, such as a basic to-do list or a weather app.
As you gain more experience, you can gradually increase the complexity of your projects. For instance, a beginner might build a basic blog, while an intermediate developer could add user authentication, dynamic content, or integration with APIs. A well-defined project with a reasonable scope ensures that you don't feel overwhelmed and that you're able to complete it with the knowledge you have.
Software projects can be categorized into several areas, each offering unique challenges and learning opportunities. Web development, for example, can include everything from building simple static websites to complex, data-driven web applications. Mobile app development, on the other hand, involves learning how to design and develop apps for Android or iOS, which requires knowledge of native languages or cross-platform frameworks like Flutter or React Native.
Other categories include game development, machine learning, data science, software tools, and cybersecurity projects. Choosing a category that interests you allows you to dive deeper into that domain while mastering related technologies. It's important to explore different areas and decide which category aligns best with your career goals or personal passion.
To make your project more relevant and future-proof, consider leveraging current and emerging technologies. Areas like artificial intelligence, blockchain, cloud computing, Internet of Things (IoT), and augmented/virtual reality (AR/VR) are rapidly growing and offer exciting project opportunities. Working with these technologies not only enhances your learning experience but also keeps your project aligned with industry trends.
For instance, building a blockchain-based application or an AI-powered recommendation system can provide valuable experience and make your portfolio stand out to potential employers. Keeping an eye on trends ensures that you are working on a project that is not only technically challenging but also in demand.
Understanding the tools and frameworks available for your project is essential for efficient development. Many modern software projects are built with the help of frameworks, libraries, and tools that streamline the development process. For example, web development projects often use frameworks like Django, Flask, React, or Angular, while mobile apps might be built using React Native or Flutter.
Additionally, tools like Docker for containerization or Git for version control can make the development process smoother and more manageable. Researching available tools also allows you to select the most appropriate technologies for your project, ensuring that you can implement features quickly and effectively without reinventing the wheel.
Brainstorming is a key step in generating software project ideas. Start by listing any problems you've encountered or tasks you'd like to automate. These can serve as the basis for your projects. Try to think outside the box and come up with unique, creative ideas that interest you. For example, you might have an idea for a personal finance app that automates budgeting based on user spending patterns.
Once you've compiled a list of ideas, evaluate each one in terms of feasibility, scalability, and how much you can learn from it. Consider factors such as the technologies involved, the time required to complete the project, and your level of expertise. Prioritize the projects that excite you the most and offer the greatest learning opportunities.
Once you’ve brainstormed several ideas, it's essential to narrow them down to a specific problem that is worth solving. A focused project will help ensure clarity and avoid scope creep. For instance, instead of trying to build a complete e-commerce platform from scratch, you might decide to focus on one component, such as the shopping cart or user authentication system.
Narrowing the focus ensures that you stay within a manageable scope while still developing a meaningful solution. This allows you to concentrate your efforts on solving one problem really well, which is often more valuable than tackling too many features at once.
Software development offers a wide range of domains that programmers can explore based on their interests, expertise, and career goals. Each domain has its own set of challenges, tools, technologies, and learning opportunities. Here’s a breakdown of some popular domains of software development that programmers can explore:
Web development involves creating websites and web applications that run on browsers. This domain is highly versatile and offers opportunities to specialize in various areas. Front-end development focuses on the visual and interactive aspects of a website, working with HTML, CSS, JavaScript, and frameworks like React or Angular.
Back-end development involves server-side programming, databases, and APIs using languages like Node.js, Django, or Ruby on Rails. Full-stack development combines both front-end and back-end skills, allowing developers to build both client and server-side applications. Web development is constantly evolving, with frameworks and tools being updated regularly, offering developers a wealth of opportunities to expand their skill set.
Mobile app development focuses on creating software applications for smartphones and tablets. It is a rapidly growing domain driven by the global use of mobile devices. Native mobile development involves writing platform-specific code, such as Swift for iOS or Kotlin for Android. Cross-platform development, on the other hand, uses frameworks like React Native or Flutter to write one codebase that runs on both iOS and Android.
Mobile developers also create mobile games, which requires knowledge of game engines like Unity or Unreal Engine. Given the increasing reliance on smartphones, this field offers vast career opportunities and creative challenges.
Game development is the art and science of designing and creating interactive video games. It requires a deep understanding of both programming and creative design. Game developers often work with specialized game engines like Unity (using C#) or Unreal Engine (using C++), which provide tools to build complex 3D environments, physics simulations, and interactive gameplay.
Artificial intelligence (AI) is often used to design non-player characters (NPCs) that respond to players’ actions. Game development also involves 3D modeling and animation, creating the visual elements of the game, and implementing sound and music. It’s a highly dynamic and rewarding field, combining technical skill with creativity.
Data Science and Machine Learning (ML) are fields that deal with extracting knowledge from large datasets and building algorithms that allow systems to learn from data. Data science involves statistical analysis, data visualization, and using libraries like Pandas and NumPy for data manipulation and Matplotlib or Seaborn for data visualization.
Machine learning focuses on creating algorithms that can make predictions or decisions based on past data, using tools like scikit-learn, TensorFlow, and Keras. Deep learning, a subfield of ML, uses neural networks to work with large-scale data, such as in image recognition or natural language processing (NLP). As businesses increasingly rely on data-driven decisions, these skills are in high demand.
AI and robotics aim to replicate human intelligence and automate tasks. Artificial intelligence (AI) includes technologies like machine learning, computer vision, and natural language processing. AI applications range from chatbots to self-driving cars. Robotics involves designing and programming machines capable of performing tasks autonomously or with minimal human input.
This field typically requires knowledge of embedded systems, sensor integration, and robotic control algorithms. Tools like ROS (Robot Operating System) are frequently used to simplify robot development. The combination of AI and robotics is revolutionizing industries such as manufacturing, healthcare, and logistics.
Cloud computing enables the delivery of computing services—such as storage, databases, networking, and software—over the internet rather than from local servers. Developers working in cloud computing typically work with cloud platforms like AWS (Amazon Web Services), Google Cloud, and Microsoft Azure to build scalable, flexible, and cost-effective systems.
They also use serverless computing, where the cloud provider manages the infrastructure, and developers write functions that automatically scale. Infrastructure as Code (IaC) tools like Terraform and Ansible help automate the deployment and management of cloud infrastructure. As more companies migrate to the cloud, cloud computing expertise is becoming increasingly essential for developers.
Cybersecurity is critical to protecting computer systems and networks from security breaches, cyberattacks, and unauthorized access. Developers in this field focus on creating software that enhances security, including firewalls, antivirus software, and encryption algorithms. Ethical hacking, or penetration testing, involves simulating attacks to identify vulnerabilities before malicious hackers exploit them.
Network security aims to safeguard data as it travels across networks, using methods like virtual private networks (VPNs) and intrusion detection systems (IDS). As cyber threats continue to evolve, the demand for cybersecurity professionals grows, making it an essential domain for developers to explore.
Blockchain is a decentralized and secure way to store and transfer data, and it is the foundation of cryptocurrencies like Bitcoin and Ethereum. Blockchain developers build smart contracts—self-executing contracts where the terms are directly written into lines of code—and work with distributed ledger technologies like Hyperledger and Corda.
Blockchain also has applications beyond cryptocurrency, such as supply chain management, secure voting systems, and digital identity verification. Developers can also work on creating decentralized applications (dApps) that run on blockchain networks, making this an exciting and innovative field with significant potential for impact.
Embedded systems are specialized computers designed to perform specific tasks, and they form the backbone of many modern devices. Embedded system development requires knowledge of hardware programming, typically using languages like C or C++, to interact directly with devices like sensors, motors, and microcontrollers.
The Internet of Things (IoT) connects these devices to the Internet, allowing them to collect and exchange data. For example, smart home devices like thermostats and wearables are part of the IoT. Developers working in this field often use Raspberry Pi, Arduino, or ESP32 for prototyping IoT applications, combining sensor integration, real-time systems, and wireless communication.
DevOps is a set of practices aimed at combining software development and IT operations to streamline the development lifecycle and improve deployment efficiency. Developers in this domain often work with CI/CD (Continuous Integration/Continuous Deployment) pipelines, automating the process of building, testing, and deploying code.
Containerization tools like Docker and Kubernetes are used to create consistent environments that can scale and run applications reliably across different systems. Infrastructure as Code (IaC) tools like Terraform and Ansible automate infrastructure management. DevOps aims to reduce development time, increase deployment speed, and ensure continuous delivery of high-quality software.
Software testing and quality assurance (QA) are critical for ensuring that software is reliable, functional, and free of defects. Manual testing involves testing software manually to identify bugs or issues in functionality, while automated testing uses scripts to perform repetitive tests, improving efficiency and accuracy automatically.
Developers in QA may also work on performance testing to ensure that applications run smoothly under load and security testing to identify vulnerabilities. With the rise of continuous testing in CI/CD pipelines, developers are increasingly integrating automated tests throughout the development process to catch issues early and ensure quality at every stage.
Software architecture and design is the foundational phase of building software applications. This domain focuses on creating a blueprint for the entire system that defines its structure, components, and interactions. Developers working in architecture design systems that are scalable, maintainable, and efficient. They often use design patterns like Model-View-Controller (MVC), Observer, and Singleton to solve common architectural problems.
A key part of software architecture is ensuring that applications can handle growth without compromising performance, which is why microservices architecture has become popular. Developers working on software architecture must think about both high-level system design and low-level code implementation to ensure that software meets user needs while remaining efficient.
Engaging in software engineering projects is one of the most effective ways to enhance your skills and build a strong portfolio. The 35 projects listed—ranging from beginner to expert levels—offer opportunities to work on real-world problems and gain hands-on experience with various technologies. Whether you’re building a To-Do List Application, a Personal Finance Tracker, or diving into more complex projects like Machine Learning Algorithms or Blockchain Applications, each project helps sharpen your understanding of key software development concepts.
By working on these projects, you not only learn different programming languages and frameworks but also understand the software development lifecycle, debugging techniques, and best practices for code optimization. Each project has the potential to teach new skills, whether it's web development, mobile app creation, game development, or data science. Additionally, these projects allow you to demonstrate your proficiency to potential employers or collaborators by showcasing a portfolio of well-documented, functional software.
Copy and paste below code to page Head section
Software engineering projects involve the application of engineering principles to software development. These projects typically focus on designing, developing, testing, and maintaining software systems. They range from simple applications (like a To-Do list app) to complex systems (like machine learning models or cloud-based applications), helping developers apply theoretical knowledge in a real-world context.
Working on software engineering projects helps you build practical skills, enhance your problem-solving abilities, and demonstrate your knowledge to potential employers. These projects also allow you to understand the complete software development lifecycle, including requirements gathering, design, coding, testing, and deployment.
To start your first project, choose a beginner-friendly idea like a To-Do List Application or a Simple Calculator App. Make sure you pick a project that aligns with the skills you're currently learning (like HTML/CSS for web development or Python for basic apps). Break the project into smaller tasks and tackle them one by one to build your confidence.
While working alone is a great way to learn and build your skills, collaborating with others can also be beneficial. Working in teams simulates real-world development environments, where you’ll need to collaborate, communicate, and share responsibilities. Platforms like GitHub can help you manage collaborative projects, even if you're working remotely.
To ensure that you're working at the right level, pick projects that align with your current knowledge but also push you to learn something new. For beginners, projects like To-Do List Applications or Simple Weather Apps are great starters. As you gain experience, you can attempt more challenging projects like E-commerce Websites, Social Media Dashboards, or Machine Learning Models.
A portfolio of completed software projects demonstrates your practical abilities to potential employers. It showcases your problem-solving skills, your ability to deliver working applications and your knowledge of various technologies. A well-maintained portfolio can set you apart from other candidates in competitive job markets.