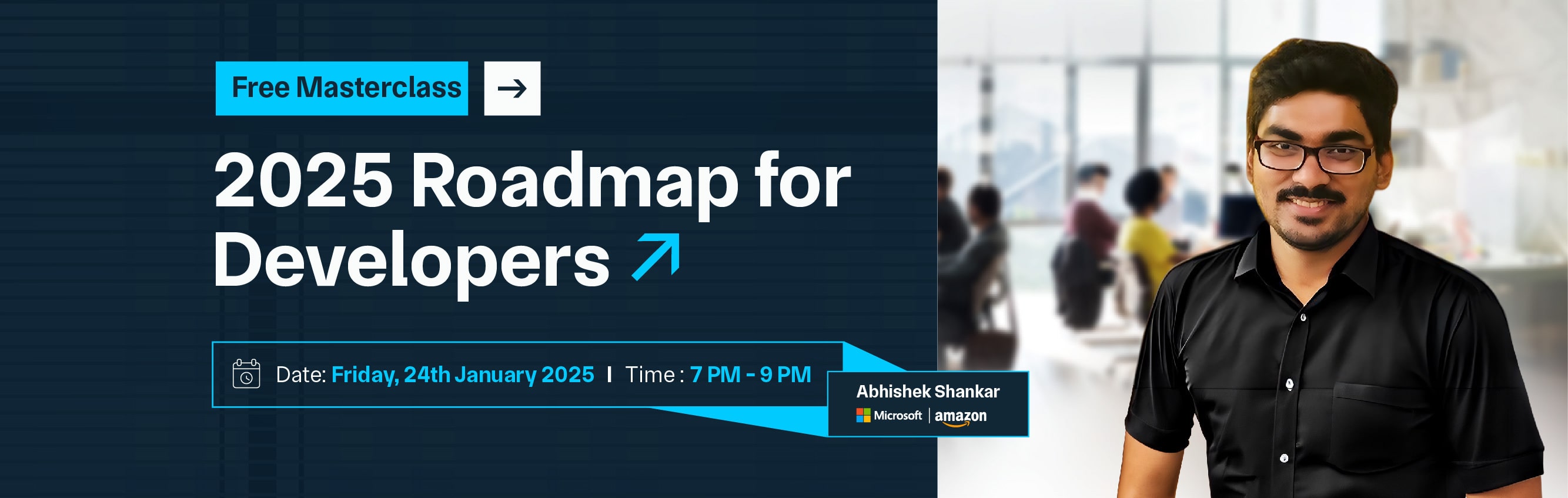

In Python, the self keyword is a crucial element in defining instance methods within a class. It serves as a reference to the current instance of the class, allowing access to its attributes and methods. When you define a method in a class, the first parameter is typically named self, although you could technically use any name; using self is a convention that enhances readability and understanding.
For example, within a class, you might have a method defined as def method name(self). When you call this method on an instance of the class, Python automatically passes the instance itself as the first argument, enabling the method to access instance-specific data. This is essential for maintaining the state of each object created from the class.
Without self, methods would not know which instance's data they should operate on, leading to confusion and errors in object-oriented programming. Additionally, self differentiates between instance attributes and local variables, ensuring that changes to an attribute affect the instance and not just a temporary variable. Thus, understanding and properly using self is fundamental for effective object-oriented programming in Python.
In Python, self is a reference to the current instance of a class. It is used within instance methods to access attributes and methods associated with that specific object. When you define a method inside a class, the first parameter is conventionally named self, allowing the method to operate on the instance’s data. For example, when you create an object of a class and call a method on it, Python automatically passes the object itself as the first argument.
This means you can access instance variables and call other methods using self. It helps differentiate between instance attributes and local variables, ensuring that modifications affect the object's state.
Using self is crucial for maintaining clarity in object-oriented programming, as it makes it clear which instance's attributes you are referring to. While self is a convention, you could technically use another name, but doing so would make your code less readable and understandable. Overall, self is fundamental to defining and manipulating the properties and behaviors of objects in Python.
The self keyword in Python is essential for working with class instances, providing a way to access instance variables and methods. Here’s a detailed explanation of how self works:
When a method is called on an instance of a class, Python automatically passes the instance itself as the first argument to the method. This is where self comes into play. By convention, the first parameter of instance methods is named self, allowing you to refer to the instance within the method.
Using self, you can access and modify instance variables. When you assign values to instance attributes (typically defined in the __init__ method), you use self to distinguish them from local variables. For example:
class Dog:
def __init__(self, name):
self.name = name # self.name is an instance variable
def bark(self):
print(f"{self.name} says woof!")
Here, self.name refers to the instance variable name, allowing each Dog instance to have its unique name.
You can also call other methods within the class using self. This helps maintain the context of the current instance. For example:
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return f"{self.name} says woof!"
def greet(self):
print(self.bark()) # Calls the bark method using self
self helps distinguish instance attributes from local variables within methods. If a method has a local variable with the same name as an instance variable, using self clarifies which one you are referring to:
class Dog:
def __init__(self, name):
self.name = name
def set_name(self, name): # Local variable with the same name
self.name = name # Refers to the instance variable
While self is primarily used in instance methods, it’s important to note that class methods use cls as the first parameter to refer to the class itself instead of an instance.
The self keyword in Python can appear in various contexts, primarily within classes, but its usage can differ based on the type of method being defined. Here’s a detailed look at how self functions in different contexts:
In instance methods, self refers to the current instance of the class. This is the most common usage of self and allows you to access instance attributes and other methods.
class Cat:
def __init__(self, name):
self.name = name
def meow(self):
print(f"{self.name} says meow!")
# Example usage
my_cat = Cat("Whiskers")
my_cat.meow() # Output: Whiskers says meow!
In class methods, the first parameter is conventionally named cls, not self. Class methods operate on the class itself rather than on instances. They are defined using the @classmethod decorator.
class Dog:
species = "Canine"
@classmethod
def get_species(cls):
return cls.species
# Example usage
print(Dog.get_species()) # Output: Canine
Static methods do not use self or cls as parameters. They do not depend on class or instance attributes and are defined using the @staticmethod decorator. This makes them suitable for utility functions that might belong to a class but don’t need access to class or instance data.
class MathUtils:
@staticmethod
def add(x, y):
return x + y
# Example usage
print(MathUtils.add(3, 5)) # Output: 8
When defining properties with the @property decorator, self is used to get or set values, allowing you to control access to instance attributes.
class Person:
def __init__(self, age):
self._age = age
@property
def age(self):
return self._age
@age.setter
def age(self, value):
if value < 0:
raise ValueError("Age cannot be negative.")
self._age = value
# Example usage
person = Person(30)
print(person.age) # Output: 30
person.age = 35
In subclasses, self still refers to the instance of the subclass. When overriding methods from a parent class, you can use self to call the parent class’s methods, maintaining access to the subclass instance.
class Animal:
def speak(self):
print("Animal speaks.")
class Dog(Animal):
def speak(self):
super().speak() # Calls the parent class method
print("Dog barks.")
# Example usage
dog = Dog()
dog.speak()
# Output:
# Animal speaks.
# Dog barks.
The syntax of self in Python is straightforward, but its correct usage is essential for defining instance methods and accessing instance variables. Here’s a breakdown of how self is typically used in various contexts:
When defining a class, the self parameter is included in the __init__ method to initialize instance variables.
class ClassName:
def __init__(self, parameter1, parameter2):
self.attribute1 = parameter1
self.attribute2 = parameter2
class Dog:
def __init__(self, name):
self.name = name # self.name refers to the instance variable
Instance methods also take self as their first parameter to allow access to instance attributes and other methods.
class ClassName:
def method_name(self, parameters):
# Access instance variables and methods
self.attribute = parameters
class Dog:
def bark(self):
print(f"{self.name} says woof!")
Within methods, you can access instance attributes using self.
class ClassName:
def method_name(self):
print(self.attribute1) # Accessing instance variable
class Cat:
def meow(self):
print(f"{self.name} says meow!")
You can call other instance methods using self within the same class.
class ClassName:
def method1(self):
self.method2() # Calling another instance method
def method2(self):
print("Method 2 called")
class Cat:
def greet(self):
self.meow() # Calls the meow method
def meow(self):
print("Meow!")
When overriding methods in subclasses, self still refers to the subclass instance, allowing access to both parent and subclass attributes and methods.
class ParentClass:
def method(self):
print("Parent method")
class ChildClass(ParentClass):
def method(self):
super().method() # Calls the parent class method
print("Child method")
The self keyword is essential in Python classes for several key reasons:
self allows methods to reference the instance of the class they belong to. This is crucial for maintaining the state of each object. Without self, methods would have no way to access or modify instance-specific data.
Using self, you can access instance variables and methods from within other methods. This enables the encapsulation of behavior and state, allowing an object to manage its own data and functionality.
self helps differentiate between instance attributes and local variables. This clarity is vital when variables have the same name. For example, when you assign a value to self. attribute, it explicitly indicates that you're modifying an instance attribute rather than a temporary local variable.
self is foundational for implementing object-oriented programming (OOP) principles such as encapsulation and inheritance. It allows you to build classes that encapsulate data and behavior, promoting code reusability and organization.
In subclasses, self maintains a reference to the subclass instance, which allows overridden methods to operate correctly on the subclass's data and functionality. This is essential for achieving polymorphism in OOP.
By using self, code becomes more readable and maintainable. Developers can easily identify which variables and methods belong to the instance, making it clearer how objects interact with their data.
The explicit definition of self in Python serves several important purposes that align with the language's design philosophy and principles of object-oriented programming:
By requiring self to be explicitly defined as the first parameter in instance methods, Python enhances code readability. It makes it clear when a method is accessing instance variables or calling other instance methods, helping both the original developer and others who read the code to understand its structure and flow.
Python follows the principle of "explicit is better than implicit." By explicitly declaring self, the language emphasizes that methods belong to instances, not the class itself. This reduces ambiguity, as it's immediately clear which data is being accessed or modified.
Explicitly using self allows each instance of a class to maintain its state. This is crucial in object-oriented programming, where each object may have different attributes. Without an explicit reference to self, managing multiple instances with distinct data would be more complex and less intuitive.
The explicit nature of self allows developers to choose any name for it if they wish (though it’s strongly advised to stick with self for clarity). This flexibility can be useful in specific contexts, such as when defining decorators or meta classes, providing a way to adapt to various programming needs.
When calling methods, using self helps differentiate between instance methods and class methods or static methods. It makes it clear that the method is meant to operate on the instance’s state rather than the class or its static properties.
By making the instance reference explicit, Python encourages a more functional approach to programming, where the state of an object is clearly defined and manipulated. This can lead to cleaner, more maintainable code.
The self keyword is fundamental in Python classes, as it allows access to instance attributes and methods. Here are some examples demonstrating its usage:
class Car:
def __init__(self, make, model):
self.make = make # Assigning instance variable
self.model = model
def display_info(self):
print(f"Car make: {self.make}, Model: {self.model}")
# Creating an instance of Car
my_car = Car("Toyota", "Corolla")
my_car.display_info() # Output: Car make: Toyota, Model: Corolla
In this example, self is used in the __init__ method to initialize the instance variables make and model, which are specific to each Car object. The display_info method uses self to access these variables.
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return f"{self.name} says woof!"
def greet(self):
print(self.bark()) # Calls the bark method using self
# Creating an instance of Dog
my_dog = Dog("Buddy")
my_dog.greet() # Output: Buddy says woof!
Here, the greet method calls the bark method using self, demonstrating how self allows one method to access another within the same class.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def celebrate_birthday(self):
self.age += 1 # Modifies the instance variable age
print(f"Happy Birthday, {self.name}! You are now {self.age} years old.")
# Creating an instance of Person
john = Person("John", 30)
john.celebrate_birthday() # Output: Happy Birthday, John! You are now 31 years old.
In this case, self is used to modify the instance variable age when the celebrate birthday method is called.
Definition: Instance methods are functions defined within a class that operate on an instance of that class. They take self as their first parameter, which refers to the specific object calling the method.
Characteristics:
Example:
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self): # Instance method
return self.width * self.height
# Creating an instance of Rectangle
rect = Rectangle(4, 5)
print(rect.area()) # Output: 20
Definition: Class methods are functions defined within a class that operate on the class itself rather than on instances. They take cls (by convention) as their first parameter, which refers to the class.
Characteristics:
Example:
class Circle:
pi = 3.14 # Class variable
@classmethod
def calculate_area(cls, radius): # Class method
return cls.pi * (radius ** 2)
# Calling the class method
print(Circle.calculate_area(5)) # Output: 78.5
Using self as the first parameter of instance methods in Python is crucial for several reasons:
The primary purpose of self is to provide a clear and explicit reference to the instance of the class that is calling the method. This helps maintain the context, allowing the method to access and manipulate the instance’s attributes and other methods.
By convention, using self makes the code more readable. It indicates to anyone reading the code that the method is dealing with instance-specific data. This explicitness helps differentiate between instance variables and local variables within the method.
Having self as the first parameter creates a consistent structure for instance methods. This consistency makes it easier for developers to understand and predict how methods will behave, leading to better code maintenance and collaboration.
Using self aligns with object-oriented programming principles by clearly linking methods to the objects they operate on. This helps encapsulate behavior and state, which is fundamental to designing robust classes.
While self is the conventional name, it is not a keyword in Python, meaning you could technically use a different name. However, sticking with self ensures that your code remains consistent with community standards and is easily understood by other Python developers.
By explicitly defining self, you avoid potential ambiguities in method calls. It’s clear when you are accessing an instance variable (e.g., self.attribute) versus a local variable or a parameter passed to the method.
In Python, the term self is often associated with instance methods and attributes, but it doesn't refer specifically to a "self constructor." Instead, it typically comes into play within the constructor method, which is defined by the __init__ function. Here’s a detailed explanation of how self is used in constructors and the purpose of the constructor itself:
A constructor in Python is a special method called when an instance of a class is created. The __init__ method serves as the constructor and is automatically invoked upon object instantiation. It is used to initialize the instance's attributes and perform any setup required for the object.
1. First Parameter: The __init__ method takes self as its first parameter, allowing it to reference the newly created instance. This is essential for setting instance-specific attributes.
2. Initializing Attributes: Within the constructor, you can use self to assign values to instance attributes, enabling each object to maintain its own state.
Here’s a simple example to illustrate how self is used in a Python class constructor:
class Person:
def __init__(self, name, age): # Constructor method
self.name = name # Initializing instance variable
self.age = age # Initializing instance variable
def introduce(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
# Creating an instance of Person
person1 = Person("Alice", 30)
person1.introduce() # Output: Hello, my name is Alice and I am 30 years old.
In Python, you do not explicitly pass self when calling an instance method; the Python interpreter automatically handles it. Here’s a detailed explanation:
When you call an instance method on an object, Python automatically passes the instance as the first argument to the method. This means that you do not need to include self in the call.
class Dog:
def bark(self):
print("Woof!")
# Create an instance of Dog
my_dog = Dog()
my_dog.bark() # Python automatically passes my_dog as self
In this example, when my_dog.bark() is called, Python internally translates it to Dog.bark(my_dog), where my_dog is passed as self.
While you do not pass self during the method call, you must include it as the first parameter in the method definition. This is necessary for the method to know which instance it is operating on.
class Cat:
def __init__(self, name):
self.name = name
def greet(self):
print(f"{self.name} says meow!")
# Create an instance of Cat
my_cat = Cat("Whiskers")
my_cat.greet() # No need to pass self explicitly
It’s also important to distinguish between instance methods and class methods:
class Example:
@classmethod
def show_class_name(cls):
print(cls.__name__)
Example.show_class_name() # No need to pass cls explicitly
In Python, you cannot completely skip using self in instance methods if you want to reference instance attributes or methods. However, there are some scenarios where you might not need to explicitly define or use self. Here are some approaches and techniques:
Static methods do not require self because they do not operate on an instance of the class. Instead, they can be called on the class itself and do not have access to instance-specific data.
Example:
class MathUtils:
@staticmethod
def add(a, b):
return a + b
# Calling the static method
result = MathUtils.add(5, 3) # Output: 8
print(result)
Class methods use cls instead of self. They operate on the class itself rather than on instances. You define them using the @classmethod decorator.
Example:
class Example:
class_variable = 10
@classmethod
def display_class_variable(cls):
print(cls.class_variable)
# Calling the class method
Example.display_class_variable() # Output: 10
If you define functions outside of a class, you won’t need self at all. However, these functions won't have access to instance-specific data.
Example:
def add(a, b):
return a + b
# Calling the function
result = add(5, 3) # Output: 8
print(result)
You can use lambda functions, which also do not require self. These can be defined within or outside a class.
Example:
class Example:
def __init__(self, x):
self.x = x
self.double = lambda: self.x * 2 # Using lambda without self in the signature
# Creating an instance
obj = Example(5)
print(obj.double()) # Output: 10
In Python, self and __init__ are fundamental concepts in object-oriented programming. While self serves as a reference to the current instance of a class, enabling access to instance attributes and methods, __init__ is a special method that acts as a constructor, initializing an object's state upon creation. The following table highlights the key differences between self and __init__, providing a clear understanding of their roles and functionalities within a class.
In Python, self is a crucial convention used in object-oriented programming, but it is not a reserved keyword. It serves as a reference to the current instance of a class, allowing access to instance attributes and methods.
While Python permits the use of any valid identifier in place of self, adhering to this naming convention enhances code readability and consistency. This introduction will explore the nature of self, its purpose, and best practices for its use in class definitions.
In Python, self is not a reserved keyword like def or class. Instead, it is a naming convention widely accepted by Python developers to represent the instance of a class within its methods.
The choice of the name self is not enforced by the language, which means you could technically use any valid identifier in its place. However, using a consistent name like self helps maintain code readability and aligns with community standards.
The primary purpose of self is to serve as a reference to the current instance of a class. When you define methods in a class, the first parameter is typically self, which allows you to access instance variables and methods from within those methods.
This reference is crucial for managing the state of the object and enabling the behavior associated with that particular instance. It helps differentiate between instance attributes and local variables, making your code clearer.
Although it is common practice to use self, Python allows you to use any name for the first parameter of instance methods. For instance, you could use my_instance, this, or any other valid variable name.
While this flexibility exists, it is generally discouraged because it can lead to confusion and make your code less readable. By using self, you adhere to the conventions of Python, making it easier for other developers (and your future self) to understand the code.
To promote clarity and consistency in your code, it is best practice to use self as the first parameter in instance methods. This naming convention helps convey the intent of the code clearly and aligns with Python's design philosophy of simplicity and readability.
Sticking to established conventions not only enhances your code's maintainability but also makes it easier for other developers to collaborate on your projects, as they will be familiar with the use of self in object-oriented programming.
The self keyword is a fundamental aspect of Python's object-oriented programming, serving as a reference to the current instance of a class. Understanding how to use self effectively can greatly enhance your coding practices and improve the readability and maintainability of your code.
This guide provides essential tips for leveraging self in your Python programs, ensuring that you adhere to best practices and conventions in class design. Here are some useful tips about using the self keyword in Python:
Always use self as the first parameter in instance methods. This is the widely accepted convention in Python, and it enhances code readability and maintainability. Following this standard makes your code easier for others (and yourself) to understand.
Use self to clearly distinguish between instance attributes and local variables. When you assign a value to self. attribute, it is clear that you are modifying an instance variable, whereas a local variable would not use self.
If you choose to use self, maintain consistency throughout your codebase. Avoid mixing different names for the instance reference in the same class, as this can lead to confusion and decrease the readability of your code.
Remember to include self in all instance methods, including constructors and any method that accesses or modifies instance attributes. This ensures that you can access the instance’s state correctly.
When calling instance methods, you do not need to pass self explicitly; Python does this for you. This keeps method calls clean and simple. For example, simply call object.method() instead of Class.method(object).
Know that self is only available within instance methods. In class methods (defined with @classmethod), you should use cls instead, and in static methods (defined with @staticmethod), you do not use self at all.
Leverage self when working with properties or decorators. In property methods, self allows you to access the instance’s attributes, making it easy to create getter and setter methods.
While self is standard, ensure that your method names are descriptive. This complements the use of self, making it clear what the method is doing in relation to the instance.
the self keyword is a vital component of Python's object-oriented programming paradigm, providing a clear reference to instance attributes and methods. By adhering to the conventions and best practices outlined, you can enhance the readability, maintainability, and overall quality of your code.
Understanding and effectively utilizing self not only simplifies interactions with object instances but also fosters collaboration and understanding within the Python community. Embracing these practices will lead to cleaner and more efficient code in your Python projects.
Copy and paste below code to page Head section
No, self is not a reserved keyword. It is a convention used to refer to the instance of a class within its methods. You can technically use any valid name, but it’s best to stick with self for clarity and consistency.
self allows instance methods to access instance attributes and other methods. It differentiates between instance variables and local variables, ensuring that the correct data is manipulated.
Yes, you can use any valid identifier in place of self. However, using a different name may confuse readers of your code, so it’s advisable to adhere to the convention.
No, you do not need to pass self explicitly when calling an instance method. Python automatically passes the instance as the first argument.
self is used in instance methods to refer to the specific instance of a class, while cls is used in class methods (defined with @classmethod) to refer to the class itself.
No, self is not used in static methods (defined with @staticmethod) because they do not operate on an instance of the class and do not have access to instance attributes.