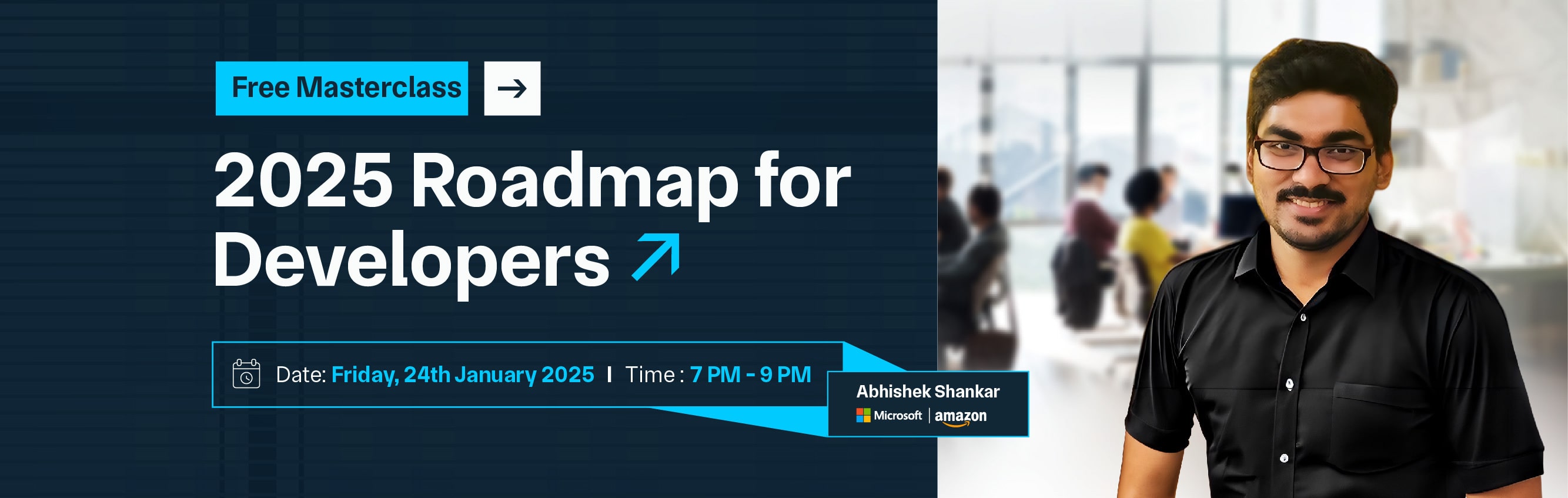

Redux is a state management library used with React and React Native to handle complex state interactions in applications. It centralizes the application state into a single store, ensuring consistent and predictable state management across the app. Redux operates on the principles of a single immutable state tree, actions to describe state changes, and reducers to handle those changes by producing a new state. To integrate Redux into a React Native app, start by installing Redux and React-Redux.
Define actions and reducers to manage state changes, configure a Redux store to maintain state, and use the Provider component from React-Redux to make the store available throughout the app. Finally, connect components to the store using connect to access and update state, facilitating a more manageable and scalable application architecture. This structured approach helps in maintaining a consistent state, improving the predictability and maintainability of the application.
Redux is a robust state management tool for React and React Native applications, designed to handle complex state interactions with ease. It centralizes the application’s state into a single store, offering a predictable way to manage and update the state through actions and reducers. Actions describe state changes, while reducers define how these changes are applied.
Redux is a state management library commonly used with React and React Native to handle complex state logic predictably. It centralizes the state of an application into a single store, allowing components to access and update the state through actions and reducers. This approach simplifies state management, especially in large applications with intricate state interactions.
Redux operates on three core principles that help manage the application state in a predictable and organized manner:
By adhering to these principles, Redux provides a structured and predictable way to manage state in applications, leading to more maintainable and scalable code.
Implementing Redux in a React Native application involves several steps to set up state management efficiently. Here’s a comprehensive guide to get you started:
First, you need to install Redux and React-Redux, which integrates Redux with React Native.
npm install redux react-redux
Actions are plain JavaScript objects that describe changes to the state. Define action types and action creators to specify what kind of actions can be dispatched.
actions.js:
// Define action types
export const INCREMENT = 'INCREMENT';
export const DECREMENT = 'DECREMENT';
// Action creators
export const increment = () => ({
type: INCREMENT,
});
export const decrement = () => ({
type: DECREMENT,
});
Reducers specify how the application's state changes in response to actions. They are pure functions that take the current state and an action and return a new state.
reducer.js:
import { INCREMENT, DECREMENT } from './actions';
// Initial state
const initialState = {
count: 0,
};
// Reducer function
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case INCREMENT:
return { count: state.count + 1 };
case DECREMENT:
return { count: state.count - 1 };
default:
return state;
}
};
export default counterReducer;
The store holds the state of your application. Configure the store with the reducer(s) you’ve created.
store.js:
import { createStore } from 'redux';
import counterReducer from './reducer';
// Create the Redux store
const store = createStore(counterReducer);
export default store;
Use the Provider component from react-redux to make the Redux store available to all components in your app.
App.js:
import React from 'react';
import { Provider } from 'react-redux';
import store from './store';
import Counter from './Counter'; // Your connected component
const App = () => (
<Provider store={store}>
<Counter />
</Provider>
);
export default App;
Use the connect function from react-redux to connect your React Native components to the Redux store, allowing them to access the state and dispatch actions.
Counter.js:
import React from 'react';
import { connect } from 'react-redux';
import { View, Text, Button } from 'react-native';
import { increment, decrement } from './actions';
// Define the component
const Counter = ({ count, increment, decrement }) => (
<View>
<Text>Count: {count}</Text>
<Button title="Increment" onPress={increment} />
<Button title="Decrement" onPress={decrement} />
</View>
);
// Map state and dispatch to props
const mapStateToProps = state => ({
count: state.count,
});
const mapDispatchToProps = {
increment,
decrement,
};
// Connect the component to the Redux store
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
Redux is a powerful state management library that can help you manage complex states in your React Native applications. Here’s a step-by-step guide to setting up Redux in your React Native app:
First, you need to install Redux and React-Redux, which will help integrate Redux with your React Native app.
npm install redux react-redux
Actions are payloads of information that send data from your application to your Redux store. They are plain JavaScript objects that have a type property and can include additional data.
actions.js:
// Define action types
export const INCREMENT = 'INCREMENT';
export const DECREMENT = 'DECREMENT';
// Action creators
export const increment = () => ({
type: INCREMENT,
});
export const decrement = () => ({
type: DECREMENT,
});
Reducers are pure functions that take the current state and an action and return a new state. They specify how the state changes in response to actions.
reducer.js:
import { INCREMENT, DECREMENT } from './actions';
// Initial state
const initialState = {
count: 0,
};
// Reducer function
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case INCREMENT:
return { count: state.count + 1 };
case DECREMENT:
return { count: state.count - 1 };
default:
return state;
}
};
export default counterReducer;
The store is the object that holds the state of your application. Create a Redux store by passing your reducer to createStore.
store.js:
import { createStore } from 'redux';
import counterReducer from './reducer';
// Create the Redux store
const store = createStore(counterReducer);
export default store;
Wrap your application with the Provider component from react-redux to make the Redux store available to all components.
App.js:
import React from 'react';
import { Provider } from 'react-redux';
import store from './store';
import Counter from './Counter'; // Your connected component
const App = () => (
<Provider store={store}>
<Counter />
</Provider>
);
export default App;
Use the connect function from react-redux to connect your React Native components to the Redux store. This function maps state and dispatch to the props of the component.
Counter.js:
import React from 'react';
import { connect } from 'react-redux';
import { View, Text, Button } from 'react-native';
import { increment, decrement } from './actions';
// Define the component
const Counter = ({ count, increment, decrement }) => (
<View>
<Text>Count: {count}</Text>
<Button title="Increment" onPress={increment} />
<Button title="Decrement" onPress={decrement} />
</View>
);
// Map state and dispatch to props
const mapStateToProps = state => ({
count: state.count,
});
const mapDispatchToProps = {
increment,
decrement,
};
// Connect the component to the Redux store
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
Actions are the payloads of information that send data from your application to your Redux store. They play a crucial role in the Redux architecture by describing what happened in your application. Here’s a step-by-step guide on how to create actions in Redux:
Action types are constants that represent the types of actions your application can perform. It’s a good practice to define these as constants to avoid typos and ensure consistency.
actionTypes.js:
// Define action types as constants
export const ADD_TODO = 'ADD_TODO';
export const REMOVE_TODO = 'REMOVE_TODO';
export const TOGGLE_TODO = 'TOGGLE_TODO';
Action creators are functions that return action objects. They encapsulate the creation of actions, making your code cleaner and easier to maintain.
actions.js:
import { ADD_TODO, REMOVE_TODO, TOGGLE_TODO } from './actionTypes';
// Action creator for adding a todo
export const addTodo = (text) => ({
type: ADD_TODO,
payload: {
text,
completed: false,
},
});
// Action creator for removing a todo
export const removeTodo = (id) => ({
type: REMOVE_TODO,
payload: id,
});
// Action creator for toggling a todo's completion status
export const toggleTodo = (id) => ({
type: TOGGLE_TODO,
payload: id,
});
You can dispatch actions using these action creators from your components or other parts of your application.
Example Component Using Action Creators:
import React from 'react';
import { connect } from 'react-redux';
import { addTodo, removeTodo, toggleTodo } from './actions';
import { View, Text, Button, TextInput } from 'react-native';
const TodoApp = ({ todos, addTodo, removeTodo, toggleTodo }) => {
const [text, setText] = React.useState('');
return (
<View>
<TextInput
placeholder="Add a new todo"
value={text}
onChangeText={setText}
/>
<Button
title="Add Todo"
onPress={() => {
addTodo(text);
setText('');
}}
/>
{todos.map(todo => (
<View key={todo.id}>
<Text
style={{ textDecorationLine: todo.completed ? 'line-through' : 'none' }}
onPress={() => toggleTodo(todo.id)}
>
{todo.text}
</Text>
<Button
title="Remove"
onPress={() => removeTodo(todo.id)}
/>
</View>
))}
</View>
);
};
// Map state and dispatch to props
const mapStateToProps = state => ({
todos: state.todos,
});
const mapDispatchToProps = {
addTodo,
removeTodo,
toggleTodo,
};
export default connect(mapStateToProps, mapDispatchToProps)(TodoApp);
Reducers are pure functions that specify how the state of your application changes in response to actions. They receive the current state and an action, and return a new state. Here’s how to create and manage reducers in Redux:
Start by defining the initial state of your application. This state represents the default values before any actions have been applied.
initialState.js:
// Initial state for the todos feature
const initialState = {
todos: [],
};
export default initialState;
Reducers are functions that handle state changes based on the action types they receive. Each reducer function takes two arguments: the current state and the action object.
reducer.js:
import { ADD_TODO, REMOVE_TODO, TOGGLE_TODO } from './actionTypes';
import initialState from './initialState';
// Reducer function
const todoReducer = (state = initialState.todos, action) => {
switch (action.type) {
case ADD_TODO:
// Add a new todo to the list
return [
...state,
{
id: Date.now(), // Unique ID for each todo
text: action.payload.text,
completed: action.payload.completed,
},
];
case REMOVE_TODO:
// Remove a todo by filtering out the one with the specified ID
return state.filter(todo => todo.id !== action.payload);
case TOGGLE_TODO:
// Toggle the completion status of a todo
return state.map(todo =>
todo.id === action.payload
? { ...todo, completed: !todo.completed }
: todo
);
default:
return state;
}
};
export default todoReducer;
If your application has multiple reducers, use combineReducers from Redux to combine them into a single root reducer.
rootReducer.js:
import { combineReducers } from 'redux';
import todoReducer from './reducer';
const rootReducer = combineReducers({
todos: todoReducer,
});
export default rootReducer;
To set up a Redux store in a React Native application:
Install Redux:
npm install redux react-redux
Define Reducers: Create reducers to handle state changes based on actions.
// counterReducer.js
const counterReducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
export default counterReducer;
Combine Reducers (if needed):
// rootReducer.js
import { combineReducers } from 'redux';
import counterReducer from './counterReducer';
const rootReducer = combineReducers({
counter: counterReducer,
});
export default rootReducer;
Create the Store:
// store.js
import { createStore } from 'redux';
import rootReducer from './rootReducer';
const store = createStore(rootReducer);
export default store;
Integrate with React Native: Wrap your app with the Provider from react-redux.
// App.js
import React from 'react';
import { Provider } from 'react-redux';
import store from './store';
import Counter from './Counter'; // Your component
const App = () => (
<Provider store={store}>
<Counter />
</Provider>
);
export default App;
This setup centralizes state management and makes it accessible across your React Native app.
Integrating Redux with a React Native application involves connecting your app's components to the Redux store to manage and access global state. Here’s a concise guide to get you started:
First, install the necessary libraries:
npm install redux react-redux
Define actions to describe state changes.
actions.js:
// Action types
export const ADD_TODO = 'ADD_TODO';
// Action creator
export const addTodo = (text) => ({
type: ADD_TODO,
payload: { text, completed: false }
});
Write reducers to handle state changes based on actions.
reducer.js:
import { ADD_TODO } from './actions';
const initialState = { todos: [] };
const todoReducer = (state = initialState, action) => {
switch (action.type) {
case ADD_TODO:
return { todos: [...state.todos, action.payload] };
default:
return state;
}
};
export default todoReducer;
Set up the Redux store with the reducer.
store.js:
import { createStore } from 'redux';
import todoReducer from './reducer';
const store = createStore(todoReducer);
export default store;
Use Provider from react-redux to make the store available to your components.
App.js:
import React from 'react';
import { Provider } from 'react-redux';
import store from './store';
import TodoApp from './TodoApp'; // Your main component
const App = () => (
<Provider store={store}>
<TodoApp />
</Provider>
);
export default App;
In Redux, handling asynchronous actions requires additional setup because Redux itself only manages synchronous state changes. Middleware like redux-thunk or redux-saga is used to handle asynchronous operations such as API calls. Here’s a guide to handling asynchronous actions using redux-thunk, a popular middleware for managing async logic in Redux.
First, install redux-thunk:
npm install redux-thunk
Set up Redux-Thunk in your store configuration. This middleware allows you to write action creators that return functions instead of plain action objects.
store.js:
import { createStore, applyMiddleware } from 'redux';
import thunk from 'redux-thunk';
import rootReducer from './rootReducer'; // Combine your reducers if needed
const store = createStore(
rootReducer,
applyMiddleware(thunk) // Apply thunk middleware
);
export default store;
Define action creators that return a function (thanks to redux-thunk), which can perform async operations and then dispatch actions based on the results.
actions.js:
import { FETCH_TODOS_REQUEST, FETCH_TODOS_SUCCESS, FETCH_TODOS_FAILURE } from './actionTypes';
// Action creator for fetching todos
export const fetchTodos = () => {
return async (dispatch) => {
dispatch({ type: FETCH_TODOS_REQUEST });
try {
const response = await fetch('https://api.example.com/todos');
const data = await response.json();
dispatch({ type: FETCH_TODOS_SUCCESS, payload: data });
} catch (error) {
dispatch({ type: FETCH_TODOS_FAILURE, error });
}
};
};
When working with Redux in a React Native application, you might encounter various issues related to state management, actions, or component connections. Here’s a guide to troubleshoot and debug common Redux issues:
1. Check Action Types and Action Creators
Ensure that action types and action creators are correctly defined and imported. Incorrect action types or mismatched action creators can cause unexpected behavior.
2. Validate Reducer Logic
Reducers should handle actions and update state correctly. Ensure your reducer logic is correctly implemented:
Example Issue: If the state isn’t updating, check if the reducer is returning a new state object.
3. Use Redux DevTools
Redux DevTools can be extremely helpful for debugging. It provides a UI to inspect state changes, dispatched actions, and more.
Example Configuration:
import { createStore } from 'redux';
import rootReducer from './rootReducer';
import { composeWithDevTools } from 'redux-devtools-extension';
const store = createStore(
rootReducer,
composeWithDevTools() // Enhances store with DevTools
);
export default store;
4. Check Middleware Setup
Middleware like redux-thunk or redux-saga should be correctly applied to handle asynchronous actions. Ensure middleware is added correctly when creating the store.
5. Inspect Component Connections
Ensure that components are properly connected to the Redux store using connect from react-redux.
Example Check: If the component doesn’t render as expected, check if the props are correctly passed and updated.
6. Verify Store Integration
Make sure the Redux store is correctly integrated with your React Native app using the Provider component from react-redux.
7. Debug Asynchronous Actions
When dealing with asynchronous actions, check:
Example: Use console.log or breakpoints inside async actions to check if they are executing and dispatching actions as expected.
8. Use Console Logs and Breakpoints
Add console.log statements or use breakpoints to debug issues:
To set up Redux in a React Native application, you need to install a few essential packages. Here’s a step-by-step guide:
Redux is the core library for state management. Install it using npm or yarn:
npm install redux
or
yarn add redux
React-Redux is the binding library that connects Redux with React. It provides the Provider component to pass the Redux store to your React components and the connect function to connect components to the store.
npm install react-redux
or
yarn add react-redux
Redux Thunk is a middleware that allows you to write action creators that return functions instead of plain objects. This is useful for handling asynchronous actions.
npm install redux-thunk
or
yarn add redux-thunk
Redux DevTools helps in debugging by providing a graphical interface to inspect the Redux store. You can use it in your browser or configure it in your React Native app.
For Browser: Install the Redux DevTools extension from your browser's extension store.
For React Native: You can use redux-devtools-extension:
npm install redux-devtools-extension
or
yarn add redux-devtools-extension
After installing the packages, configure Redux in your React Native project:
Example Store Setup:
import { createStore, applyMiddleware } from 'redux';
import thunk from 'redux-thunk'; // Import thunk middleware
import rootReducer from './reducers'; // Your combined reducers
import { composeWithDevTools } from 'redux-devtools-extension'; // For Redux DevTools
const store = createStore(
rootReducer,
composeWithDevTools(applyMiddleware(thunk)) // Apply middleware and DevTools
);
export default store;
Example App Integration:
import React from 'react';
import { Provider } from 'react-redux';
import store from './store'; // Import the configured store
import App from './App'; // Your main app component
const Root = () => (
<Provider store={store}>
<App />
</Provider>
);
export default Root;
Redux is a powerful state management library that offers several benefits for React Native applications. Here are some key advantages:
Redux enforces a strict unidirectional data flow, which helps maintain a predictable state throughout the application. Actions are dispatched to reducers that return new state objects, making it easier to trace state changes and debug issues.
Redux centralizes the application's state in a single store, which simplifies the management of state across different components. This centralized state management ensures that all parts of the application have access to the same state, avoiding inconsistencies and simplifying data sharing.
With Redux, debugging becomes more manageable thanks to tools like Redux DevTools. These tools allow you to inspect state changes, view action history, and time-travel through state changes, making it easier to identify and fix issues.
Redux’s structured approach to state management makes it scalable for large applications. By separating concerns into actions, reducers, and middleware, Redux supports complex state interactions and asynchronous operations, making it well-suited for applications with growing complexity.
Redux's middleware capability allows you to extend its functionality. Middleware like redux-thunk or redux-saga facilitates handling asynchronous actions, logging, and other side effects, enhancing the flexibility of state management.
By following Redux's principles and patterns, your codebase becomes more organized and modular. Actions, reducers, and selectors are separated, making the code easier to maintain and refactor as the application evolves.
Redux ensures that data flow is consistent across the application. Components can subscribe to specific pieces of state and dispatch actions, leading to a uniform approach to managing and updating the application state.
Redux has a large and active community, providing a wealth of resources, middleware, and extensions. The strong community support ensures that there are plenty of best practices, libraries, and tools available to enhance your Redux setup.
When working with Redux in a React Native application, seeking help and engaging in discussions can significantly improve your understanding and resolve issues more efficiently. Here’s how to effectively seek help and participate in discussions:
When seeking help, be clear and specific:
Redux is a popular state management library, but whether you should use it depends on the specific needs and complexity of your React Native application. Here are factors to consider when deciding if Redux is right for your project:
Use Redux if:
Avoid Redux if:
Use Redux if:
Avoid Redux if:
Use Redux if:
Avoid Redux if:
Use Redux if:
Avoid Redux if:
Use Redux if:
Avoid Redux if:
Deciding whether to use Redux in your React Native application hinges on the specific needs of your project. Redux excels in scenarios where you have complex state management requirements, need a predictable state container, or are working on a large-scale application that demands sophisticated debugging and middleware capabilities. It provides a structured approach to managing state, handling asynchronous actions, and integrating with various tools and libraries, making it ideal for complex and scalable projects. However, for simpler applications or projects where the state management needs are modest, React’s built-in state management solutions, such as useState, useReducer, or the Context API, might be more appropriate.
These alternatives offer a more lightweight approach and can often handle state management without the additional complexity that comes with Redux. Ultimately, the choice to use Redux should be guided by your project's complexity, the anticipated scale, and your team's familiarity with Redux's concepts. Weigh the benefits of Redux against the potential overhead and complexity it introduces, and choose the solution that best aligns with your development goals and application requirements.
Copy and paste below code to page Head section
Redux is a predictable state management library for JavaScript applications. It centralizes the state of your application into a single store, making it easier to manage and debug state changes across your components.
Redux is useful in React Native applications for managing complex states, handling global states, and managing asynchronous actions. It helps in maintaining a predictable state and makes debugging easier with tools like Redux DevTools.
To set up Redux, install redux, react-redux, and optionally redux-thunk for handling asynchronous actions. Create a store with createStore(), wrap your app with Provider to pass the store to your components, and define actions and reducers to manage state changes.
Redux-thunk is a middleware for Redux that allows action creators to return functions instead of plain objects. This is useful for handling asynchronous operations such as API calls within Redux.
Redux itself does not handle asynchronous actions. Middleware like redux-thunk or redux-saga is used to manage async operations. These middlewares allow you to dispatch functions or handle side effects in a more organized manner.
Redux DevTools is a tool that provides a graphical interface for inspecting Redux state changes, dispatched actions, and state history. To use it, integrate the Redux DevTools extension with your Redux store setup, allowing you to debug and track state changes easily.