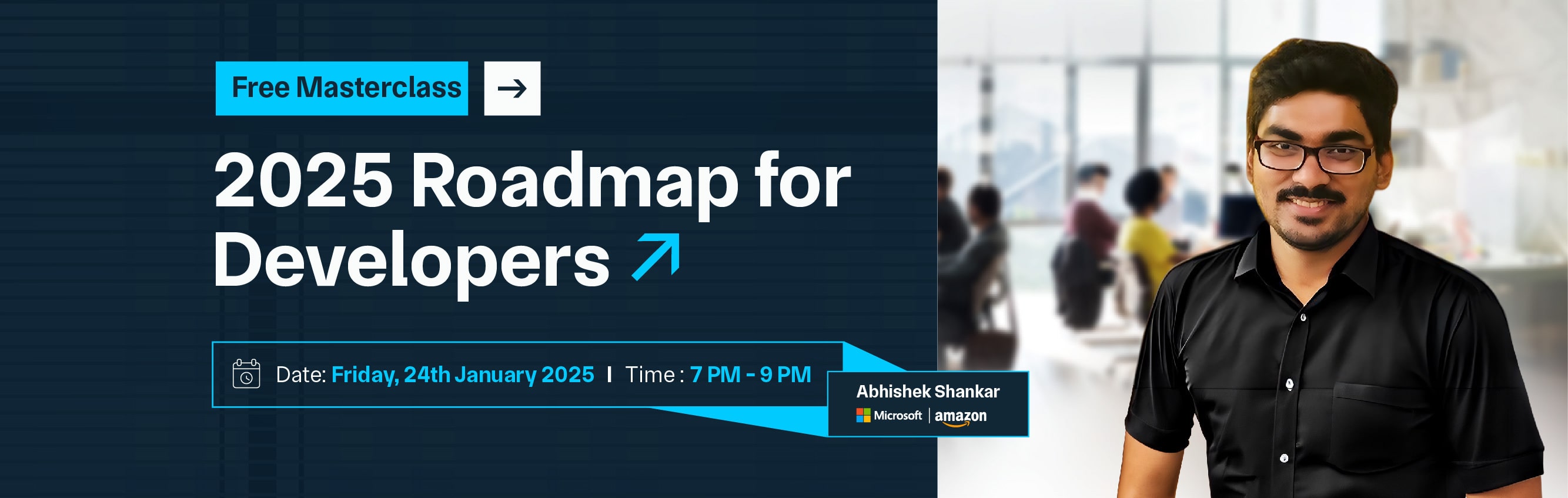

Simply called a constructor is a method that helps in the creation of objects. The constructor is no different in React. This can connect event handlers to the component and initialize the component’s local state. Before the component is mounted, the constructor() function is shot, and, like most things in React, it has a few rules that you can follow when using them. The concept of a constructor is the same in React.
The constructor in a React component is called before the component is mounted. When you implement the constructor for a React component, you need to call the super(props) method before any other statement. Suppose you do not call the super(props) method, this.
Props will be undefined in the constructor and can lead to bugs. If there is a constructor() function in your component, this function will be called when the component gets initiated. The constructor function is where you initiate the component’s properties.in react, component properties should be kept in an object called state.
Constructor is the method that is called automatically when we create the object from that class. In the context of React, it's essential for setting up the initial component state and for the binding method.
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props); // Call the parent class's constructor
// Initialize state
this.state = {
count: 0,
};
// Bind methods if needed
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<button onClick={this.handleClick}>Count: {this.state.count}</button>
</div>
);
}
}
export default MyComponent;
Before using this. props in a constructor is important to call its important to call super(props).If you want to implement the constructor for a React component, call the super(props) method before any other statement. Otherwise, this. props will be undefined in the constructor and create bugs. console.log(this.props) will safely access this.props.
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props); // You must call super(props) first!
console.log(this.props); // Now you can safely access this.props
this.state = {
count: 0,
};
}
render() {
return (
<div>
<p>{this.props.message}</p> {/* Accessing props here is fine */}
<p>Count: {this.state.count}</p>
</div>
);
}
}
export default MyComponent;
Don't call setState after the component is created. It's not meant for updates. You should never call setState() inside the constructor() in React.
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
// Don't call setState here! It's not meant for updates.
this.setState({ count: 0 }); // This will lead to an error or unexpected behavior
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
</div>
);
}
}
export default MyComponent;
This is necessary because, by default, event handler methods in JavaScript do not automatically bind to the class instance (this), which is why you use .bind(this).
constructor(props) {
super(props); // Call parent constructor
this.state = {
count: 0,
};
// Binding event handlers in one place (the constructor)
this.onClick = this.onClick.bind(this);
this.onKeyUp = this.onKeyUp.bind(this);
}
One issue is that we can not test it properly until an API call is made and we get a response. It encourages the developer not to assign a value directly from the props object to the state of a component.
Props are for Passing Data: props are used to pass data from the parent component to the child component. The data in the state can change the component itself. Hence, props cannot modified inside components.
State Should Be Independent: when we assign props to a state, it may couple between parent and child components. It may cause issues.Its better to props remain static and state data through dynamic.
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = {
data: null,
loading: true,
};
}
// Make the API call after the component is mounted
componentDidMount() {
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((data) => {
this.setState({
data: data,
loading: false,
});
})
.catch((error) => {
console.error('Error fetching data:', error);
this.setState({ loading: false });
});
}
render() {
const { data, loading } = this.state;
if (loading) {
return <div>Loading...</div>;
}
return <div>{data ? JSON.stringify(data) : 'No data available'}</div>;
}
}
export default MyComponent;
They play an important role and help us in initializing components, and states, and binding event handlers. A better understanding of the React component constructors helps in better creating and managing our React application and components.
Copy and paste below code to page Head section
Because of the advantages of a constructor in a react component, it is a best practice always to try to use one.
The constructor is a method used to initialize an object's state in a class. It is automatically called during the creation of an object in a class. The concept of a constructor is the same in React. The constructor in a React component is called before the component is mounted.
Typically, in React, constructors are only used for two purposes: Initializing the local state by assigning an object to this.state. Binding event handler methods to an instance.
Redux is a state management library commonly used with React, although it can also be used with other JavaScript frameworks. It helps manage the state of your application. It was inspired by Flux, another state management architecture developed by Facebook for building client-side web applications.
The use of super(props) becomes mandatory to invoke the constructor from React. Component before you access this in a constructor of React class components. The constructor gains accessibility to this. Props through utilizing the super(props) method. The use of super(props) is vital because it prevents errors that result from uninitialized.
Hooks exist exclusively for functional components and functionally cannot be incorporated into class components. The constructor of class components helps state initialization while binding event handlers to objects. When planning to implement hooks, you need to convert your class component to a functional component.