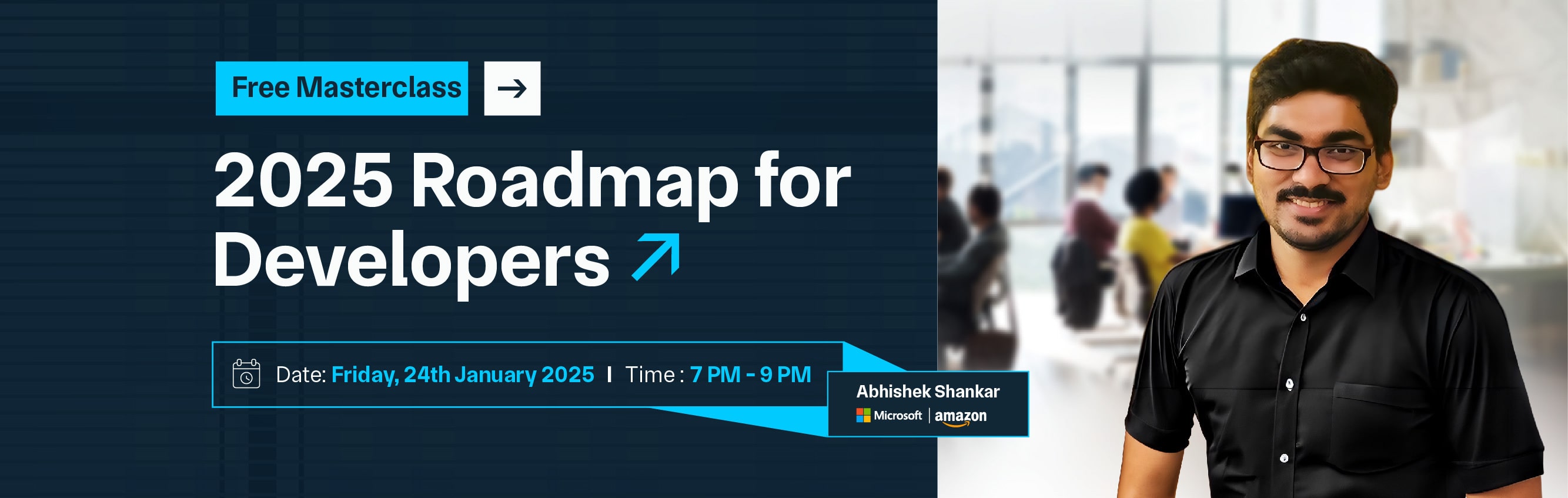

In Angular, Promises are a fundamental tool for handling asynchronous operations, allowing you to manage operations that are complete in the future, such as HTTP requests or time-based actions. A Promise represents a value that is not yet available but will be resolved at some point. It has three states: pending, resolved (fulfilled), or rejected.
When using Promises in Angular, you can perform actions after the asynchronous operation completes using the .then() method for success and .catch() for error handling. For example, when fetching data from a server, you might use the HttpClient service to return an observable, which can then be converted to a Promise using the toPromise() method. This approach allows you to handle the response data once it’s available or manage errors if something goes wrong.
Promises are useful for chaining multiple asynchronous operations or for integrating with APIs that return Promises. Although Angular’s HttpClient uses observables by default, understanding Promises is crucial for integrating with libraries or legacy code that relies on them, ensuring that you can manage asynchronous data flow efficiently within your applications.
In Angular, Promises are a way to handle asynchronous operations, such as fetching data from a server or performing a time-based task. A Promise represents an operation that hasn't been completed yet but is expected to do so in the future. It essentially acts as a placeholder for a value that will be available once the operation finishes.
A Promise has three key states:
Promises in Angular are often used with the HttpClient service for making HTTP requests. When an HTTP request is made, it returns an Observable by default, but it can be converted to a Promise using the toPromise() method. This allows developers to use Promises if they prefer their syntax or need to integrate with code that uses Promises.
To handle the result of a Promise, you use the .then() method to define what should happen when the Promise resolves and the .catch() method to handle any errors that occur. Promises provide a way to write cleaner, more manageable asynchronous code by allowing you to chain operations and handle errors in a structured manner.
In AngularJS, Promises are a core concept for managing asynchronous operations, such as HTTP requests, timers, or any other actions that take time to complete. Promises provide a way to handle these asynchronous operations in a more manageable and predictable manner.
A Promise in AngularJS represents an operation that will be completed in the future, and it has three possible states:
In AngularJS, the $q service is used to create and manage Promises. The $q service provides a promise-based API for handling asynchronous tasks. You can use $q to create a new Promise and manage its resolution or rejection.
For example, when making an HTTP request with AngularJS’s $http service, it returns a promise by default. You can use the .then() method to handle the successful response and .catch() to handle any errors. This allows you to write cleaner, more readable code for asynchronous operations by chaining .then() calls and handling errors in a consistent manner.
Here’s a brief example of how you might use Promises in AngularJS:
$http.get('/api/data')
.then(function(response) {
// Success callback
console.log('Data:', response.data);
})
.catch(function(error) {
// Error callback
console.error('Error:', error);
});
By using Promises, AngularJS makes it easier to work with asynchronous data, handle errors, and ensure that your code is executed in the proper sequence.
In Angular (specifically AngularJS), Promises are used for managing asynchronous operations and are typically handled using the $q service. The $q service provides a way to create and manage promises. Below is a breakdown of the syntax for using Promises with $q and $http in AngularJS.
To create a new promise using $q, you use the $q constructor, which provides a defer method. The defer method returns an object with promise, resolve, and reject methods. Here’s how you can use it:
javascript
var deferred = $q.defer();
var promise = deferred.promise;
promise
.then(function(result) {
// Handle success
console.log('Success:', result);
})
.catch(function(error) {
// Handle error
console.error('Error:', error);
});
// To resolve or reject the promise
deferred.resolve('Data has been resolved');
deferred.reject('An error occurred');
When using $http for making HTTP requests, it automatically returns a promise. You can handle the result of the promise with .then() and .catch() methods:
$http.get('/api/data')
.then(function(response) {
// Handle success
console.log('Data:', response.data);
})
.catch(function(error) {
// Handle error
console.error('Error:', error);
});
Promises can be chained to handle a sequence of asynchronous operations. Each .then() call returns a new promise:
$http.get('/api/data')
.then(function(response) {
// Process the response and return another promise
return $http.get('/api/other-data');
})
.then(function(otherResponse) {
// Handle the second response
console.log('Other Data:', otherResponse.data);
})
.catch(function(error) {
// Handle any error from the chain
console.error('Error:', error);
});
You can also use $q.all() to handle multiple promises simultaneously:
$q.all([
$http.get('/api/data1'),
$http.get('/api/data2')
])
.then(function(results) {
// results is an array of responses
console.log('Data1:', results[0].data);
console.log('Data2:', results[1].data);
})
.catch(function(error) {
// Handle any error from the promises
console.error('Error:', error);
});
AngularJS’s $q service and $http service make it straightforward to work with asynchronous operations using Promises, allowing you to handle success and error scenarios cleanly and effectively.
In AngularJS, as well as in JavaScript Promises in general, there are three fundamental states that a Promise can be in. These states help in managing and handling asynchronous operations effectively:
Pending: This is the initial state of a Promise. When a Promise is first created, it is in the "pending" state, meaning that the asynchronous operation has not yet completed. The Promise is waiting for the operation to either resolve successfully or reject with an error.
Fulfilled: Once the asynchronous operation completes successfully, the Promise transitions to the "fulfilled" state. This means that the operation was successful and the Promise now has a resolved value. The .then() method is used to handle the result of a fulfilled Promise.
For example:
promise.then(function(result) {
console.log('Success:', result);
});
Rejected: If the asynchronous operation fails for some reason, the Promise transitions to the "rejected" state. This indicates that there was an error or problem with the operation, and the Promise has an associated error value. The .catch() method is used to handle errors from a rejected Promise.
For example:
promise.catch(function(error) {
console.error('Error:', error);
});
Suppose you have an AngularJS application where you need to fetch user data from an API. You can use the $http service to perform this operation, which returns a Promise. Here’s how you can handle the data and errors using Promises.
angular.module('myApp', [])
.controller('MyController', ['$scope', '$http', function($scope, $http) {
// Function to fetch user data
$scope.fetchUserData = function() {
$http.get('https://api.example.com/users')
.then(function(response) {
// This is called when the Promise is fulfilled (success)
$scope.users = response.data;
console.log('Data fetched successfully:', $scope.users);
})
.catch(function(error) {
// This is called when the Promise is rejected (error)
console.error('Error fetching data:', error);
});
};
// Call the function to fetch data
$scope.fetchUserData();
}]);
If you need to create and handle Promises manually, you can use the $q service to create a Promise and resolve or reject it. Here’s a basic example:
angular.module('myApp', [])
.controller('MyController', ['$scope', '$q', function($scope, $q) {
// Function to simulate an asynchronous operation
$scope.simulateAsyncOperation = function() {
var deferred = $q.defer();
// Simulate async operation
setTimeout(function() {
var success = true; // Simulate success or failure
if (success) {
deferred.resolve('Operation completed successfully');
} else {
deferred.reject('Operation failed');
}
}, 1000);
// Handle the promise
deferred.promise
.then(function(result) {
// Success callback
console.log(result);
})
.catch(function(error) {
// Error callback
console.error(error);
});
};
// Call the function to simulate the async operation
$scope.simulateAsyncOperation();
}]);
Chaining Promises in AngularJS is a powerful technique for managing a sequence of asynchronous operations. By chaining Promises, you can ensure that operations are performed in a specific order, with each step depending on the result of the previous one.
This is particularly useful when you need to perform multiple async tasks in sequence, such as making a series of HTTP requests or processing data sequentially.Here’s a detailed example to illustrate how to chain Promises in AngularJS:
Suppose you need to perform two HTTP requests in sequence. The second request should only be made after the first request is successfully completed.
angular.module('myApp', [])
.controller('MyController', ['$scope', '$http', function($scope, $http) {
// Function to fetch user data and then fetch additional details
$scope.fetchDataInSequence = function() {
// First HTTP request to get user data
$http.get('https://api.example.com/users')
.then(function(response) {
// Process the first response
$scope.users = response.data;
console.log('Users fetched:', $scope.users);
// Return the second HTTP request, which will be chained
return $http.get('https://api.example.com/user-details');
})
.then(function(response) {
// Process the second response
$scope.userDetails = response.data;
console.log('User details fetched:', $scope.userDetails);
})
.catch(function(error) {
// Handle any error from either request
console.error('Error occurred:', error);
});
};
// Call the function to fetch data
$scope.fetchDataInSequence();
}]);
1. First HTTP Request:
2. Chaining the Second Request:
3. Handling the Second Response:
4. Error Handling:
Defining your own Promises in AngularJS involves using the $q service to create and manage Promises manually. This can be useful for scenarios where you need more control over the asynchronous operations, such as custom asynchronous functions, processing data, or integrating with libraries that use Promises. Here’s a step-by-step guide on how to define and use your own Promises in AngularJS:
First, ensure that the $q service is injected into your AngularJS component (e.g., a controller or service).
angular.module('myApp', [])
.controller('MyController', ['$scope', '$q', function($scope, $q) {
// Define and use Promises here
}]);
To create a new Promise, use the $q.defer() method, which returns an object containing promise, resolve, and reject methods.
angular.module('myApp', [])
.controller('MyController', ['$scope', '$q', function($scope, $q) {
// Function that returns a custom Promise
$scope.customAsyncOperation = function() {
// Create a deferred object
var deferred = $q.defer();
// Simulate an asynchronous operation (e.g., an API call or timeout)
setTimeout(function() {
var success = true; // Simulate success or failure
if (success) {
deferred.resolve('Operation succeeded');
} else {
deferred.reject('Operation failed');
}
}, 1000); // 1 second delay
// Return the promise object
return deferred.promise;
};
// Call the function and handle the promise
$scope.customAsyncOperation()
.then(function(result) {
// Success callback
console.log('Success:', result);
})
.catch(function(error) {
// Error callback
console.error('Error:', error);
});
}]);
1. Creating the Deferred Object:s
2. Simulating Asynchronous Operation:
3. Returning the Promise:
4. Handling the Promise:
Suppose you want to create a custom service to handle asynchronous data fetching with Promises. Here’s how you might define such a service:
angular.module('myApp', [])
.service('MyService', ['$q', '$http', function($q, $http) {
this.getData = function() {
var deferred = $q.defer();
$http.get('https://api.example.com/data')
.then(function(response) {
// Resolve the deferred object with the response data
deferred.resolve(response.data);
})
.catch(function(error) {
// Reject the deferred object with the error
deferred.reject(error);
});
return deferred.promise;
};
}]);
1. Service Definition:
2. HTTP Request:
3. Returning the Promise:
By defining your own Promises in AngularJS using $q, you gain control over asynchronous operations and can integrate them seamlessly into your applications.
Here is a comparison table outlining the key differences between Promises and Observables in the context of AngularJS (and Angular):
Integrating Promises with Angular components involves using Promises to handle asynchronous operations within the component's lifecycle. This can be useful for scenarios like fetching data from an API, handling form submissions, or any task that involves waiting for a result before proceeding. Here’s a step-by-step guide on how to integrate Promises with Angular components:
First, ensure that you inject necessary services such as $http or $q into your Angular component or service.
angular.module('myApp', [])
.controller('MyController', ['$scope', '$http', '$q', function($scope, $http, $q) {
// Define your component logic here
}]);
Create a function that performs an asynchronous operation and returns a Promise. This function can be part of the component or a service.
angular.module('myApp', [])
.controller('MyController', ['$scope', '$http', '$q', function($scope, $http, $q) {
// Function to fetch data using $http
$scope.fetchData = function() {
var deferred = $q.defer();
$http.get('https://api.example.com/data')
.then(function(response) {
deferred.resolve(response.data);
})
.catch(function(error) {
deferred.reject('Error fetching data: ' + error.statusText);
});
return deferred.promise;
};
// Call the function and handle the promise
$scope.fetchData()
.then(function(data) {
$scope.data = data;
console.log('Data fetched successfully:', $scope.data);
})
.catch(function(error) {
console.error(error);
});
}]);
Use the .then() method to handle the result when the Promise is fulfilled, and .catch() to handle any errors.
angular.module('myApp', [])
.controller('MyController', ['$scope', '$http', '$q', function($scope, $http, $q) {
// Function to fetch data and update the component
$scope.loadData = function() {
$scope.fetchData()
.then(function(data) {
$scope.data = data; // Update scope with the fetched data
})
.catch(function(error) {
$scope.error = error; // Update scope with the error message
});
};
// Initialize data loading
$scope.loadData();
}]);
You can also use Promises for other asynchronous operations, such as submitting forms or performing background tasks.
angular.module('myApp', [])
.controller('FormController', ['$scope', '$http', '$q', function($scope, $http, $q) {
// Function to submit form data
$scope.submitForm = function() {
var deferred = $q.defer();
$http.post('https://api.example.com/submit', $scope.formData)
.then(function(response) {
deferred.resolve(response.data);
})
.catch(function(error) {
deferred.reject('Error submitting form: ' + error.statusText);
});
return deferred.promise;
};
// Handle form submission
$scope.handleSubmit = function() {
$scope.submitForm()
.then(function(result) {
$scope.submitSuccess = 'Form submitted successfully!';
})
.catch(function(error) {
$scope.submitError = error;
});
};
}]);
Transitioning to async/await from Promises can greatly simplify your asynchronous code, making it more readable and easier to maintain. The async/await syntax, introduced in ECMAScript 2017 (ES8), allows you to write asynchronous code in a way that looks synchronous, using async functions and the await keyword. Here’s a guide on how to transition from Promises to async/await in AngularJS (and Angular):
Here’s how you might convert a function that returns a Promise into an async function:
angular.module('myApp', [])
.controller('MyController', ['$scope', '$http', '$q', function($scope, $http, $q) {
// Function returning a Promise
$scope.fetchData = function() {
var deferred = $q.defer();
$http.get('https://api.example.com/data')
.then(function(response) {
deferred.resolve(response.data);
})
.catch(function(error) {
deferred.reject('Error fetching data: ' + error.statusText);
});
return deferred.promise;
};
// Handling the Promise
$scope.fetchData()
.then(function(data) {
$scope.data = data;
})
.catch(function(error) {
console.error(error);
});
}]);
angular.module('myApp', [])
.controller('MyController', ['$scope', '$http', function($scope, $http) {
// Define an async function
$scope.fetchData = async function() {
try {
const response = await $http.get('https://api.example.com/data');
return response.data; // Automatically wrapped in a Promise
} catch (error) {
throw new Error('Error fetching data: ' + error.statusText);
}
};
// Handling the async function
$scope.loadData = async function() {
try {
$scope.data = await $scope.fetchData();
} catch (error) {
console.error(error);
}
};
// Call the loadData function
$scope.loadData();
}]);
Using async/await allows for simpler and more intuitive error handling with try/catch blocks.
$scope.loadData = async function() {
try {
$scope.data = await $scope.fetchData();
} catch (error) {
$scope.error = error.message; // Handle errors
console.error(error);
}
};
You can also use async/await with other asynchronous tasks like form submissions or custom operations:
angular.module('myApp', [])
.controller('FormController', ['$scope', '$http', function($scope, $http) {
// Define an async function for form submission
$scope.submitForm = async function() {
try {
const response = await $http.post('https://api.example.com/submit', $scope.formData);
$scope.submitSuccess = 'Form submitted successfully!';
} catch (error) {
$scope.submitError = 'Error submitting form: ' + error.statusText;
}
};
}]);
The future of Promises in Angular, particularly in Angular (version 2+), is intertwined with the evolution of JavaScript and the adoption of newer technologies like async/await. Here’s an overview of the future of Promises in Angular and how they fit into the broader context of modern web development:
In modern Angular development, handling asynchronous operations is essential for creating responsive applications. Promises, a staple of asynchronous JavaScript, offer a straightforward approach to manage single asynchronous tasks through .then() and .catch(). While they remain relevant, especially for legacy systems and simpler scenarios, the async/await syntax has emerged as a powerful enhancement, simplifying the process of working with Promises by making asynchronous code appear more synchronous and readable.
In addition to Promises, Observables, provided by RxJS, offer advanced capabilities for managing complex or continuous data streams, making them preferable for handling real-time updates and intricate asynchronous workflows in Angular applications. As the ecosystem evolves, integrating async/await with Promises and leveraging Observables for advanced use cases reflects modern best practices, ensuring developers can efficiently handle asynchronous operations while maintaining code clarity and scalability.
Copy and paste below code to page Head section
Promises in Angular represent a single asynchronous operation that will eventually complete with a result or an error. They are used to handle asynchronous tasks such as HTTP requests, file operations, or any operation that is not complete immediately.
In AngularJS (Angular 1.x), Promises are managed using the $q service. This service provides the defer() and promise methods to create and handle asynchronous operations. Promises can be chained with .then() and .catch() methods to handle successful results and errors, respectively.
Promises handle a single asynchronous event and are resolved once with a result or rejected with an error. Observables, on the other hand, handle multiple asynchronous events over time and offer advanced features such as operators for transformation, filtering, and combining streams of data. Observables are integral to Angular's reactive programming model and are used extensively with RxJS.
To convert Promises to async/await, declare the function as async and use the await keyword to pause execution until the Promise is resolved or rejected. This approach simplifies handling asynchronous operations and improves code readability.
Use Promises for simpler scenarios where you need to handle a single asynchronous result or error. Use Observables for more complex scenarios involving multiple asynchronous events, real-time data streams, or when you need advanced operations like filtering and combining data streams.
async/await is built on top of Promises, so there are no compatibility issues. However, for older AngularJS applications that use $q for Promises, transitioning to async/await may involve refactoring to use modern JavaScript syntax. Angular (version 2+) and newer frameworks support both Promises and async/await, allowing for smooth integration.