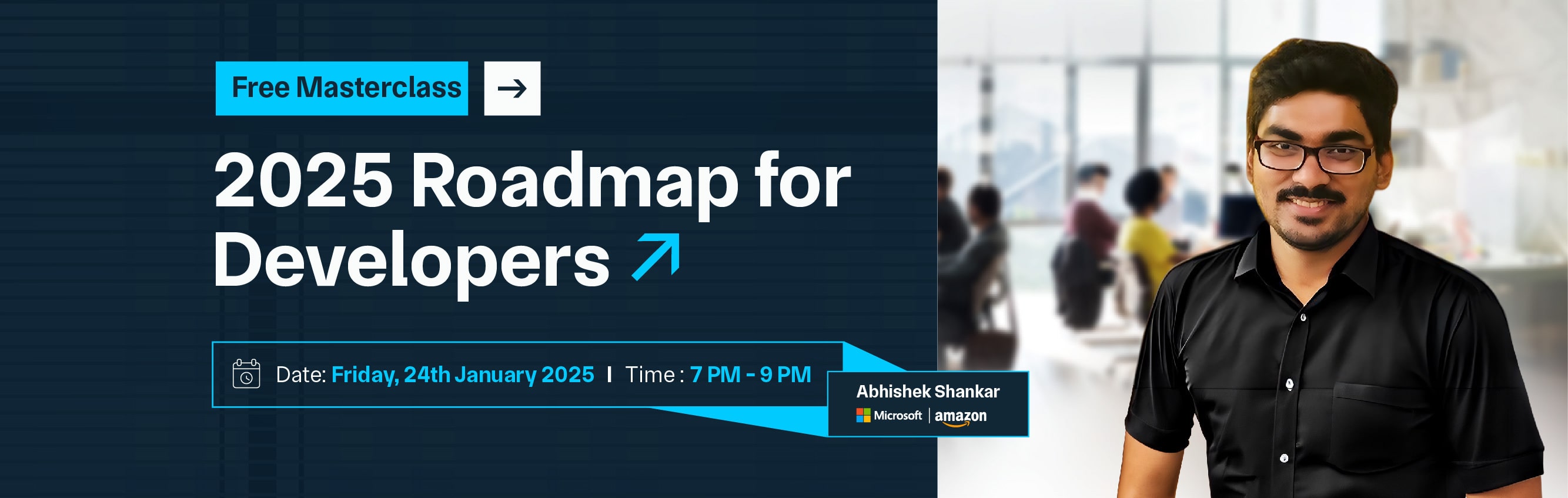

Checking your Node.js version is crucial for maintaining a stable development environment and ensuring compatibility with various packages and tools. Node.js, a popular JavaScript runtime, evolves rapidly with frequent updates that can introduce new features, fix bugs, or deprecate older functionalities. Knowing your Node.js version helps you make informed decisions about updating your codebase or dependencies. To check your Node.js version, open a terminal or command prompt and type node -v or node --version.
This command will display the currently installed version of Node.js, such as v16.13.0. It's a straightforward process, but knowing how to do it is essential for developers working with Node.js applications. Regularly updating Node.js can improve performance, security, and compatibility with modern libraries. However, it's crucial to review the release notes and test your applications after an upgrade to avoid breaking changes.
Checking your Node.js version is a simple yet vital task that helps ensure your development environment is up-to-date and compatible with your project's requirements. Additionally, different projects may require specific Node.js versions due to varying dependencies. Tools like nvm (Node Version Manager) can be extremely helpful for managing multiple Node.js versions on the same machine, allowing you to switch between versions as needed. This flexibility helps you maintain compatibility and streamline your development workflow.
Node.js is an open-source, cross-platform runtime environment that allows developers to execute JavaScript code server-side. Built on Chrome's V8 JavaScript engine, Node.js is designed for building scalable network applications. It uses an event-driven, non-blocking I/O model, making it lightweight and efficient for handling concurrent operations.
One of Node.js's key features is its package manager, npm, which provides access to a vast repository of open-source libraries and tools, simplifying the development process. Node.js is particularly popular for building real-time applications like chat services, APIs, and single-page applications due to its asynchronous capabilities.
Its single-threaded event loop handles multiple connections simultaneously, allowing for high performance and responsiveness. Node.js is well-suited for scenarios where high throughput and low latency are critical, making it a popular choice among developers for modern web applications and services.
Knowing your Node.js version is vital for several reasons:
Staying informed about your Node.js version is crucial for maintaining compatibility, security, and performance in your development projects.
Knowing your Node.js version is essential for ensuring that your development environment runs smoothly and securely. Node.js, a popular runtime for server-side JavaScript, frequently updates with new features, security patches, and performance enhancements.
By checking your version, you can ensure compatibility with libraries and tools, stay protected from vulnerabilities, and take advantage of the latest improvements. Regularly verifying your Node.js version helps maintain an optimal and reliable development experience. Checking your Node.js version is an important practice for several key reasons:
In essence, checking your Node.js version helps maintain compatibility, security, performance, and overall effectiveness in your development environment.
Checking your Node.js version through the command line is a quick and straightforward process. Here’s how you can do it:
1. Open Your Command Line Interface: This can be Terminal on macOS and Linux or Command Prompt/PowerShell on Windows.
Enter the Version Command: Type the following command and press Enter:
node -v
or
node --version
2. View the Output: The command will return the currently installed Node.js version in a format like v16.13.0. This output tells you the exact version of Node.js that your system is using.
This simple command helps you quickly determine if your Node.js version meets the requirements for your projects or if an update is necessary.
To check your Node.js version on a Windows system, follow these steps:
1. Open Command Prompt or PowerShell:
2. Run the Version Command: Type the following command and press Enter:
node -v
or
node --version
3. Check the Output: The command will display the installed Node.js version, such as v18.15.0. This output confirms which version of Node.js is currently in use on your system.
This process allows you to quickly verify your Node.js version, ensuring compatibility with your projects and confirming if any updates are needed.
To check your Node.js version on macOS or Linux, follow these steps:
1. Open Terminal: You can find Terminal in your Applications folder under Utilities on macOS, or you can search for it in the Applications menu on Linux.
Run the Version Command: Type the following command and press Enter:
node -v
or
node --version
2. View the Output: The command will display the installed Node.js version in a format such as v18.15.0. This tells you the exact version currently running on your system.
Checking your Node.js version using these commands ensures that your environment is set up correctly and helps you manage compatibility with various projects and dependencies.
When you check your Node.js version using the command node -v or node --version, the output provides essential information about your Node.js installation. Here’s how to understand it:
v18.15.0
1. Version Number: The string v18.15.0 represents the version of Node.js installed on your system. It follows a format that indicates:
2. Pre-release or Build Metadata (if any): Sometimes, the version might include additional identifiers such as -alpha, -beta, or build metadata. For example, v18.15.0-alpha.1 indicates an alpha release, which may not be fully stable.
By understanding the output, you can effectively manage your development environment and make informed decisions about updates and compatibility.
Upgrading Node.js ensures you benefit from the latest features, performance improvements, and security patches. Here’s a guide to upgrading Node.js on various systems:
1. Using the Node.js Installer:
2. Using Node Version Manager (nvm-windows):
Open Command Prompt or PowerShell and run:
nvm install <version>
nvm use <version>
Using Node Version Manager (nvm):
Install nvm if you haven’t already. Follow instructions from the nvm GitHub repository.
To install the latest version of Node.js, open Terminal, and run:
nvm install node # Installs the latest version
nvm use node # Switches to the latest version
To install a specific version, use:
nvm install <version>
nvm use <version>
Using Homebrew (macOS only):
If you use Homebrew, you can update Node.js by running:
brew update
brew upgrade node
Using NodeSource (Linux):
Add the NodeSource repository and install the latest version using:
curl -fsSL https://deb.nodesource.com/setup_18.x | sudo -E bash -
sudo apt-get install -y nodejs
After upgrading, verify the new version by running:
node -v
This command should now display the updated version number.
Upgrading Node.js helps ensure your development environment remains secure, efficient, and compatible with the latest tools and libraries.
Automating Node.js version checks can streamline your workflow and help ensure that your development environment is always using the correct version. Here are a few methods to automate version checks and manage Node.js updates:
You can add a script to your package.json file to check the Node.js version and ensure it meets the required criteria. This script can be run manually or integrated into your build process.
Add a Script: In your package.json, add a script like this:
"scripts": {
"check-node-version": "node -e 'if (process.version !== \"v14.17.0\") { console.error(`Expected Node.js v14.17.0 but found ${process.version}`); process.exit(1); }'"
}
Run the Script: Execute the script with:
npm run check-node-version
Incorporate Node.js version checks into your Continuous Integration/Continuous Deployment (CI/CD) pipelines. This ensures that the correct Node.js version is used during automated builds and deployments.
Add a Node.js Version Check Step: In your CI/CD configuration file (e.g., .github/workflows/main.yml for GitHub Actions or .gitlab-ci.yml for GitLab), add a step to check the Node.js version.
Example for GitHub Actions:
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: '14.x'
- name: Check Node.js version
run: node -v
If you use version managers like nvm or n, you can automate version switching and ensure the correct version is used for different projects.
Using .nvmrc or .node-version: Create a .nvmrc or .node-version file in your project’s root directory with the desired version: 14.17.0
Automate Version Switching: Configure your shell profile to automatically switch to the version specified in .nvmrc or .node-version upon entering the project directory. For nvm, add this to your .bashrc, .zshrc, or other shell configuration files:
cd() {
builtin cd "$@"
if [ -f .nvmrc ]; then
nvm use
fi
}
Use tools to monitor and notify you of Node.js updates. Tools like Node.js Update Monitor can check for the latest Node.js versions and notify you when an upgrade is available.
By implementing these automation techniques, you can ensure that your Node.js environment is consistently up to date and compliant with project requirements, reducing manual checks and potential compatibility issues.
Troubleshooting Node.js version issues can help you resolve common problems related to compatibility, updates, and environment configurations. Here’s a guide to addressing some typical issues:
Issue: Your application or dependencies may require a different version of Node.js.
Solution:
Use nvm or nvm-windows: Switch to the required version using Node Version Manager.
nvm install <version>
nvm use <version>
Update Version: If you're using a specific version, make sure it's installed correctly. For example, with nvm:
nvm install 18.15.0
nvm use 18.15.0
Issue: Node.js version remains the same after attempting an update.
Solution:
Issue: Encountering permission errors when installing or upgrading Node.js.
Solution:
Use sudo (Linux/macOS): If you’re facing permission issues, try using sudo to grant administrative privileges:
sudo npm install -g <package>
Reconfigure Permissions: Adjust permissions for Node.js directories:
sudo chown -R $(whoami) /usr/local/lib/node_modules
Issue: Global npm packages are not working after an upgrade.
Solution:
Reinstall Global Packages: After upgrading Node.js, you might need to reinstall global packages.
npm install -g <package>
Issue: Node Version Manager (nvm) commands are not recognized.
Solution:
Reload Shell Configuration: After installing or updating nvm, reload your shell configuration:
source ~/.bashrc
or
source ~/.zshrc
Issue: Your project does not use the expected Node.js version.
Solution:
Manually Set Version: Use nvm or n to manually set the version in your terminal:
nvm use
Issue: Your application throws errors or behaves unexpectedly after a Node.js upgrade.
Solution:
By following these troubleshooting steps, you can resolve common issues related to Node.js versions and ensure a smooth development experience.
Managing your Node.js version effectively is crucial for maintaining a stable, secure, and efficient development environment. By regularly checking your Node.js version, you ensure compatibility with your projects and dependencies, leverage the latest features and performance improvements, and stay protected against security vulnerabilities. Upgrading Node.js, whether through direct installation or version managers like nvm, helps keep your environment current with the latest advancements.
Automating version checks and updates through scripts and CI/CD pipelines can streamline your workflow and reduce manual oversight. When encountering issues, such as version incompatibility or update failures, following troubleshooting steps can resolve common problems and restore functionality. Ultimately, staying informed and proactive about Node.js version management helps optimize your development process, enhance application performance, and maintain a secure and reliable coding environment.
Copy and paste below code to page Head section
To check your current Node.js version, open your command line interface (Command Prompt on Windows, Terminal on macOS/Linux) and run: Node -v or node --version
Node Version Manager (nvm) is a tool that allows you to manage multiple versions of Node.js on the same machine. It simplifies switching between different versions and ensures compatibility with various projects.
If your application breaks after an upgrade, check the release notes for breaking changes and deprecated features. Test your application thoroughly and update any dependencies that may be incompatible with the new Node.js version.
If you need to revert to a previous version, use nvm: nvm install <old-version> nvm use <old-version>
If Node.js is not updating, ensure that: Your PATH environment variable is correctly configured. The update process was completed successfully without errors. Any version managers or package managers are up-to-date and configured correctly.
You can use multiple Node.js versions simultaneously with version managers like nvm or n. These tools allow you to switch between versions as needed for different projects.