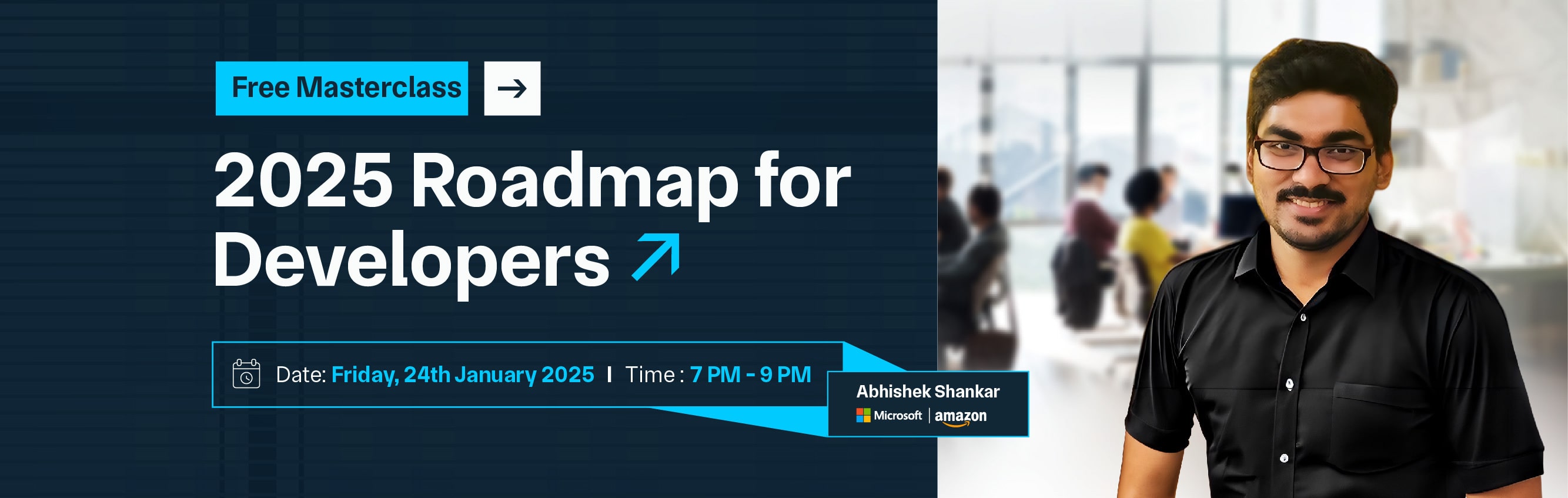

Node Sass is a popular library that provides a fast and efficient way to compile Sass (Syntactically Awesome Style Sheets) into CSS within a Node.js environment. Leveraging the LibSass engine, Node Sass offers developers the ability to write stylesheets using advanced features like variables, nesting, mixins, and more, which enhance the maintainability and scalability of styles. This library integrates seamlessly with various build tools such as Webpack, Gulp, and Grunt, making it a versatile choice for modern web development workflows.
Setting up Node Sass is straightforward, requiring just a few installation commands via npm. Once installed, developers can easily compile their Sass files into CSS, allowing for real-time updates during the development process. One of the standout benefits of Node Sass is its speed, as it compiles Sass much faster than traditional Ruby-based implementations.
However, it's worth noting that while Node Sass is highly efficient, it may only support some of the latest features available in Dart Sass, which is the primary implementation of Sass moving forward. Despite this, Node Sass remains a reliable choice for developers looking to harness the power of Sass in their Node.js applications, streamlining their styling processes and improving overall productivity.
Node Sass is a library that allows developers to use Sass (Syntactically Awesome Style Sheets) within a Node.js environment. It provides a fast and efficient way to compile Sass files into standard CSS, leveraging the LibSass engine, which is a C++ implementation of Sass. By using Node Sass, developers can take advantage of Sass features such as variables, nested rules, mixins, and functions, which enhance the modularity and maintainability of stylesheets.
This library is particularly useful in modern web development workflows as it integrates seamlessly with various build tools like Webpack, Gulp, and Grunt, making it easy to incorporate Sass compilation into automated processes. Node Sass is known for its speed and performance, offering quicker compilation times compared to traditional Ruby-based Sass implementations.
However, it’s important to note that while Node Sass is efficient, it may not support all the latest features introduced in Dart Sass, the primary and recommended implementation moving forward. Despite this limitation, Node Sass remains a popular choice for developers seeking to leverage the power of Sass in their Node.js applications.
Node Sass offers several key features that make it a valuable tool for developers working with Sass in a Node.js environment. Here are some of its main features:
Node Sass utilizes the LibSass engine, which is designed for high performance. This results in faster compilation times compared to traditional Ruby Sass implementations, making it ideal for large projects.
It supports a wide range of Sass features, including:
Node Sass integrates seamlessly with popular build tools and task runners such as Webpack, Gulp, and Grunt. This makes it easy to incorporate Sass compilation into automated workflows.
With the right setup, Node Sass can be configured for real-time compilation, allowing developers to see changes immediately as they edit Sass files. This improves workflow efficiency during development.
Node Sass works across various operating systems, including Windows, macOS, and Linux, providing a consistent experience for developers regardless of their environment.
Node Sass supports source maps, enabling better debugging by allowing developers to trace compiled CSS back to the original Sass files. This feature is particularly useful for identifying issues in styles.
Developers can create custom importers to handle specific file types or implement unique import logic, offering flexibility in how stylesheets are structured and managed.
Node Sass benefits from a strong community and comprehensive documentation, making it easier for developers to find support and resources when working with the library.
These features collectively make Node Sass a powerful choice for developers looking to utilize Sass in their Node.js projects, enhancing both productivity and the quality of the final stylesheets.
Setting up Node Sass is a straightforward process that involves a few simple steps. Here’s a guide to help you get started:
Before installing Node Sass, ensure that you have the following installed on your machine:
If you don't have a project set up yet, you can create a new directory and initialize a new Node.js project:
mkdir my-sass-project
cd my-sass-project
npm init -y
You can install Node Sass via npm by running the following command in your project directory:
npm install node-sass --save-dev
This command adds Node Sass as a development dependency in your project.
Create a new .scss file in your project directory. For example, create a file named styles. scss and add some sample Sass code:
$ primary color: blue;
body {
background-color: $primary-color;
h1 {
color: white;
}
}
You can compile your Sass file to CSS using Node Sass. You can do this from the command line with the following command:
npx node-sass styles.scss styles.css
This command compiles styles.scss into styles.css.
To automatically compile your Sass file whenever you make changes, you can use the --watch flag:
npx node-sass styles.scss styles.css --watch
Now, any changes you make to styles.scss will automatically update styles.css.
If you’re using build tools like Webpack, Gulp, or Grunt, you can configure them to use Node Sass for automatic compilation as part of your build process. Each tool has specific plugins or configurations for this purpose.
Using Node Sass allows developers to compile Sass files into CSS efficiently, taking advantage of Sass's powerful features. Here’s a guide on how to effectively use Node Sass in your projects:
After setting up Node Sass, you can compile your Sass files into CSS using the command line. Here’s the basic syntax:
npx node-sass input.scss output.css
For example, to compile a file named styles.scss into styles.css:
npx node-sass styles.scss styles.css
To continuously monitor your Sass files for changes and automatically compile them, use the --watch flag:
npx node-sass styles.scss styles.css --watch
This command will keep running, compiling your Sass file every time it’s saved.
Node Sass supports various Sass features that enhance your stylesheets:
Variables: Define reusable values for colors, fonts, etc.
$primary-color: #3498db;
body {
background-color: $primary-color;
}
Nesting: Organize your CSS in a hierarchical structure.
.nav {
ul {
list-style: none;
}
li {
display: inline-block;
}
}
Mixins: Create reusable style blocks.
@mixin border-radius($radius) {
border-radius: $radius;
}
.box {
@include border-radius(10px);
}
Functions: Define custom functions for more complex calculations.
@function calculate-rem($pixels) {
@return $pixels / 16 * 1rem;
}
.text {
font-size: calculate-rem(24px);
}
To make debugging easier, enable source maps, which link your compiled CSS back to your original Sass files:
npx node-sass --source-map styles.scss styles.css
This generates a source map file that browsers can use to trace CSS styles back to their Sass origins.
Node Sass allows for custom importers, enabling you to customize how Sass files are imported. This can be useful for managing assets or integrating with other file types.
When you compile Sass, errors can occur if there are issues in your Sass code. Node Sass will output error messages in the console, allowing you to identify and fix problems quickly.
For larger projects, integrate Node Sass with build tools like Webpack, Gulp, or Grunt to automate the compilation process as part of your overall build workflow. This setup can improve efficiency and reduce manual tasks.
Using Node Sass comes with several advantages that make it a popular choice among developers working with Sass in Node.js environments. Here are some key benefits:
Node Sass leverages the LibSass engine, which is known for its speed. This results in faster compilation times compared to traditional Ruby Sass implementations, especially for larger projects with complex stylesheets.
Node Sass integrates easily with various build tools and task runners, such as Webpack, Gulp, and Grunt. This allows developers to incorporate Sass compilation into their automated workflows without hassle.
Node Sass supports a wide range of Sass features, including variables, nesting, mixins, and functions. This allows developers to write more modular and maintainable CSS, enhancing overall code quality.
With the watch mode feature, Node Sass can automatically recompile Sass files whenever changes are made. This real-time feedback helps streamline the development process and improves productivity.
Node Sass works across multiple operating systems, including Windows, macOS, and Linux. This consistency ensures that developers can use Node Sass in various environments without compatibility issues.
While Node Sass offers many advantages, there are also challenges and considerations that developers should be aware of when using it. Here are some key points to keep in mind:
Node Sass, being based on the LibSass engine, does not support all the latest features of Sass introduced in Dart Sass. This includes some advanced functionalities and newer syntax. Developers need to check compatibility if they want to use cutting-edge features.
Node Sass has been deprecated in favor of Dart Sass, which is the primary implementation of Sass moving forward. While Node Sass still works, relying on it may pose risks for future support and updates.
While Node Sass works well in many environments, there can be occasional compatibility issues, especially with certain Node.js versions or specific operating systems. Developers should ensure they are using compatible versions to avoid unexpected behavior.
Although Node Sass provides error messages during compilation, these can sometimes be less informative than desired. Identifying issues in complex Sass files may require detective work, especially for beginners.
Node Sass's performance and features are tied to LibSass. If LibSass encounters bugs or limitations, Node Sass may inherit these issues. This reliance can be a drawback if you encounter problems that still need to be solved upstream.
While the basic setup is straightforward, using advanced features like custom importers or integrating with build tools may require additional configuration and knowledge, which can be a barrier for some developers.
If you're considering alternatives to Node Sass, there are several options available that cater to different needs and preferences. Here are some popular alternatives:
Dart Sass is the primary and officially recommended implementation of Sass, designed to support the latest features and syntax of the language. As an actively maintained project, Dart Sass ensures compatibility with ongoing updates and new Sass functionalities, making it a future-proof choice for developers. It offers a range of features, including support for modules, which enables better organization of stylesheets.
Dart Sass can be easily installed via npm, allowing developers to incorporate it into their workflows seamlessly. With its efficient real-time compilation capabilities, Dart Sass is well-suited for modern web development, ensuring that developers can harness the full power of Sass without worrying about deprecation issues.
Ruby Sass was the original implementation of Sass, widely used before the introduction of Dart Sass. However, it has since been deprecated, which means it no longer receives updates or support for newer features. While some legacy projects may still utilize Ruby Sass, it is not recommended for new projects due to its limitations and lack of ongoing maintenance.
Developers are encouraged to transition to Dart Sass or other modern alternatives to take advantage of the latest enhancements in the Sass ecosystem. Ruby Sass may be relevant in environments that heavily rely on Ruby, but its use should be approached with caution.
PostCSS is a versatile tool that transforms CSS using JavaScript plugins, offering a highly customizable approach to CSS preprocessing. By utilizing the PostCSS-Sass plugin, developers can write in Sass syntax while benefiting from the extensive PostCSS ecosystem. This setup allows for a flexible workflow where various CSS transformations can be applied alongside traditional Sass features.
PostCSS also enables developers to use plugins for auto prefixing, minification, and other optimizations. It is a powerful alternative for those looking to enhance their styling processes without being locked into a specific preprocessor.
Less is another popular CSS preprocessor that shares similarities with Sass in terms of functionality. It provides features such as variables, nesting, and mixins, allowing developers to write more maintainable and reusable stylesheets. Less is often favored by developers who prefer its straightforward syntax and find it easier to learn, particularly for those coming from a JavaScript background.
It integrates seamlessly with various build tools like Gulp and Webpack, making it a flexible choice for different project setups. While it doesn’t offer all the features of Sass, Less is a solid alternative for those looking for a simpler approach to CSS preprocessing.
Stylus is a CSS preprocessor that emphasizes flexibility and minimalism in its syntax. It allows developers to choose between an indentation-based syntax or a more traditional bracketed style, catering to individual preferences. Stylus provides dynamic features such as conditionals and loops, enabling more complex styling scenarios.
Its versatility makes it suitable for a variety of projects, especially for developers who appreciate a less opinionated approach to writing styles. Stylus can also be easily integrated into build systems, providing an efficient workflow for managing styles in larger applications.
Node Sass serves as a powerful and efficient tool for compiling Sass within the Node.js ecosystem, offering developers a robust way to harness the advanced features of Sass, such as variables, nesting, and mixins. Its fast compilation speed, seamless integration with various build tools, and support for real-time updates enhance productivity and streamline the web development process. However, developers should be mindful of its limitations, particularly regarding the support of newer Sass features and the deprecation of Node Sass in favor of Dart Sass.
As the web development landscape continues to evolve, transitioning to Dart Sass may be beneficial for those looking to utilize the latest advancements in the Sass ecosystem. By understanding the strengths and challenges associated with Node Sass, developers can make informed decisions about their styling workflows and select the best tools for their projects. Ultimately, Node Sass remains a valuable option for many, but staying updated with the latest practices and technologies will ensure that developers are well-equipped to create modern, maintainable, and efficient stylesheets.
Copy and paste below code to page Head section
Node Sass is a library that allows developers to compile Sass (Syntactically Awesome Style Sheets) into CSS in a Node.js environment. It uses the LibSass engine for fast and efficient compilation.
You can install Node Sass via npm by running the command: npm install node-sass --save-dev This adds Node Sass as a development dependency in your project.
To compile a Sass file, use the command: npx node-sass input.scss output.css Replace input. scss and output.css with your actual file names.
Yes! You can use the --watch flag to recompile your Sass files when changes are made automatically: npx node-sass styles.scss styles.css --watch
Node Sass supports many Sass features, including variables, nesting, mixins, and functions. However, it may not support the latest features available in Dart Sass.
Node Sass has been deprecated in favor of Dart Sass, which is actively maintained and supports the latest Sass features. It is recommended to use Dart Sass for new projects.