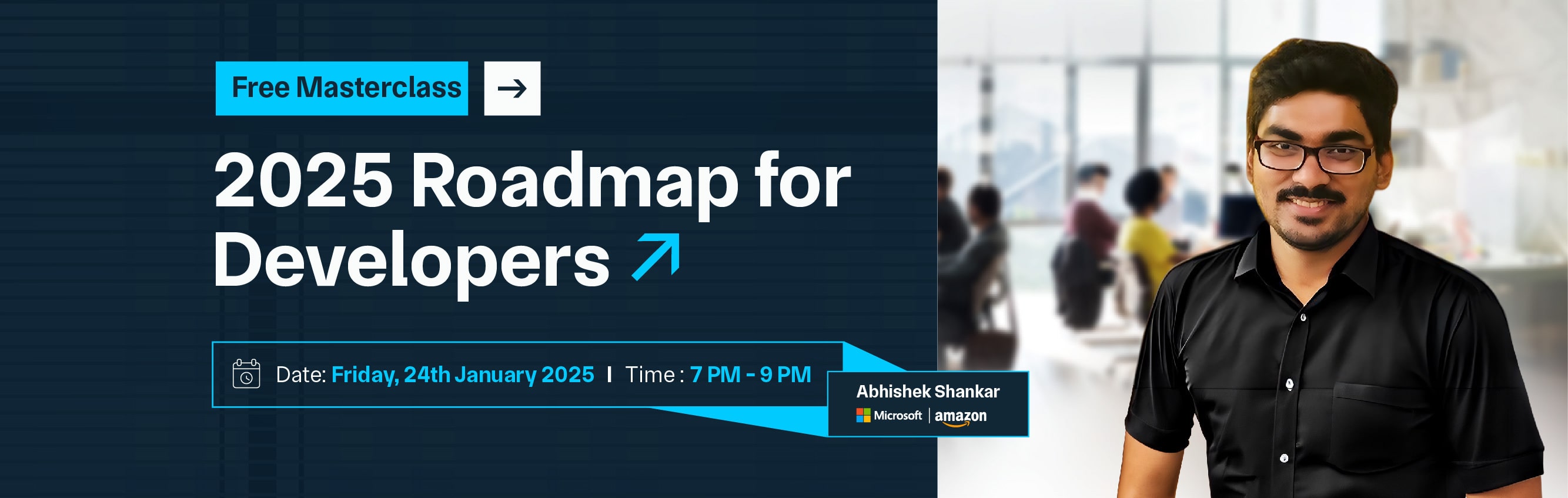

In Node.js, the callback concept is essential for handling asynchronous operations, a core feature of the platform. Since Node.js operates on a non-blocking I/O model, it processes requests without waiting for previous ones to finish, making callbacks crucial for managing the flow of asynchronous tasks. A callback is a function passed as an argument to another function and is executed once the operation completes.
For instance, when reading a file or making a database query, Node.js doesn’t wait for the operation to finish; instead, it continues executing other tasks and invokes the callback once the operation is done. This allows Node.js to efficiently handle multiple tasks concurrently without freezing the application. However, overusing nested callbacks can lead to callback hell, where code becomes hard to read and maintain due to deeply indented and tangled callback functions.
To overcome this, developers can modularize their code or use newer features like Promises and async/await, which help avoid nested structures and make asynchronous code more readable and manageable. Despite these alternatives, understanding and properly using callbacks remains fundamental to working effectively with Node.js, particularly in handling tasks such as file I/O, HTTP requests, and database interactions.
A callback is a function that is passed as an argument to another function and is executed once a certain task or operation is completed. The primary purpose of a callback is to allow the program to continue executing other tasks while waiting for a specific task to finish, making the code asynchronous.
In simpler terms, a callback function is "called back" once the function it's passed to finishes its execution. Callbacks are commonly used in JavaScript, especially in asynchronous operations like file reading, web requests, or database queries. For example, in Node.js, when you read a file asynchronously, you pass a callback function to handle the file content once it's successfully read:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading the file', err);
return;
}
console.log('File content:', data);
});
In this example:
Callbacks are essential for handling asynchronous operations and are fundamental in JavaScript and Node.js development.
In Node.js, callbacks are used to handle asynchronous operations. Node.js is designed to be non-blocking, which means it doesn't wait for an operation to complete before moving on to the next one. Instead, it delegates tasks (like reading files, querying a database, or making HTTP requests) to background processes and continues with other tasks in parallel.
When the background task finishes, a callback function is called to handle the result. This approach improves performance and allows Node.js to handle multiple operations efficiently.
const fs = require('fs');
// Asynchronous file read operation with a callback
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File content:', data);
});
In this example, fs.readFile is asynchronous. It takes three arguments:
The callback function is invoked after the file is read. If there is an error (e.g., the file doesn't exist), the callback will handle it using the first argument err. If the file is read successfully, the data is passed as the second argument, and it's logged to the console.
Why Use Callbacks?: The callback approach allows Node.js to efficiently handle I/O operations without blocking the event loop. This enables it to scale and manage many operations concurrently, making it particularly useful for real-time applications like web servers, APIs, or systems that require high performance and low latency.
Callbacks are integral to Node.js, especially because Node.js uses an asynchronous, non-blocking I/O model. Below are some common use cases of callbacks in Node.js:
Node.js provides the fs (file system) module to work with files. Operations like reading, writing, and modifying files are asynchronous by default, and callbacks are used to handle the results.
Example:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.log('Error reading file:', err);
return;
}
console.log('File content:', data);
});
In this case, the callback handles the file content once the file is successfully read, or it handles the error if the file doesn't exist or can't be read.
Database interactions in Node.js are typically asynchronous. When querying a database, a callback is used to handle the query result (success or failure). For example, libraries like MongoDB or MySQL use callbacks for their asynchronous methods.
Example (using MongoDB):
const MongoClient = require('mongodb').MongoClient;
MongoClient.connect('mongodb://localhost:27017/mydb', (err, db) => {
if (err) {
console.log('Error connecting to database:', err);
return;
}
const collection = db.collection('users');
collection.findOne({ name: 'John' }, (err, user) => {
if (err) {
console.log('Error querying database:', err);
return;
}
console.log('User data:', user);
});
});
In this example, callbacks handle the database connection and query result.
In web development, HTTP requests are often asynchronous. For both client-side and server-side HTTP operations, callbacks are used to handle the response once the request is completed.
Example (HTTP request in Node.js server):
const http = require('HTTP);
const server = http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, World!\n');
});
server.listen(8080, '127.0.0.1', () => {
console.log('Server is running at http://127.0.0.1:8080/');
});
Here, the callback is inside the server.l isten() is invoked when the server starts listening on the specified port.
Node.js uses an event-driven architecture. Event listeners in Node.js rely on callbacks to handle events asynchronously. For example, the events module allows you to create custom events and register callbacks to handle them when they are emitted.
Example:
const EventEmitter = require('events');
const myEmitter = new EventEmitter();
myEmitter.on('event', () => {
console.log('An event occurred!');
});
myEmitter.emit('event');
In this case, the callback function is triggered when the 'event' is emitted.
Node.js provides functions like setTimeout() and setInterval() for delayed or repeated actions, which require callbacks to handle the action when the timer expires or the interval fires.
Example (using setTimeout):
setTimeout(() => {
console.log('This message is displayed after 2 seconds');
}, 2000);
The callback is executed after the specified delay (2000 ms in this example).
Node.js allows spawning child processes to execute commands asynchronously. The child_process module uses callbacks to handle results when the child process completes.
Example:
const { exec } = require('child_process');
Exec ('l,' (err, stdout, stderr) => {
if (err) {
console.error('Error executing command:', err);
return;
}
console.log('Output:', stdout);
});
In this example, the callback handles the output of the ls command.
Streams in Node.js (such as reading from a file or writing data to a server) are asynchronous and use callbacks to process the data as it flows.
Example (using a readable stream):
const fs = require('fs');
const readableStream = fs.createReadStream('largefile.txt', 'utf8');
readableStream.on('data', (chunk) => {
console.log('Received chunk:', chunk);
});
readableStream.on('end', () => {
console.log('File read completed.');
});
The callback inside the data event handles each chunk of data as it's read from the file.
In web applications, callbacks are often used to handle authentication and authorization tasks. For example, after a user submits login credentials, a callback function might process the response and determine whether the credentials are valid.
Example:
function authenticate(username, password, callback) {
// Simulate checking credentials asynchronously
setTimeout(() => {
if (username === 'admin' && password === 'password123') {
callback(null, 'Authentication successful');
} else {
callback('Invalid credentials', null);
}
}, 1000);
}
authenticate('admin', 'password123', (err, message) => {
if (err) {
console.log(err);
} else {
console.log(message);
}
});
Callback Hell (also known as Pyramid of Doom) refers to a situation where multiple nested callbacks are used in asynchronous operations. As a result, the code becomes increasingly difficult to read, understand, and maintain due to excessive indentation and complex nesting of functions. This often happens when asynchronous tasks rely on each other, requiring a callback within a callback for each step of the process.
In JavaScript (and particularly in Node.js), asynchronous operations like file reading, database queries, and HTTP requests often require callbacks to handle the results. When these operations are chained together, and each one includes a new callback, the code can quickly become deep and convoluted, leading to callback hell.
fs.readFile('file1.txt', 'utf8', (err, data1) => {
if (err) {
console.error('Error reading file1:', err);
return;
}
fs.readFile('file2.txt', 'utf8', (err, data2) => {
if (err) {
console.error('Error reading file2:', err);
return;
}
fs.readFile('file3.txt', 'utf8', (err, data3) => {
if (err) {
console.error('Error reading file3:', err);
return;
}
console.log('Data from file3:', data3);
});
});
});
In this example, there are multiple levels of nested callbacks. If more operations are added, the code becomes easier to manage and maintain.
There are several techniques to prevent callback hell and make asynchronous code more readable and manageable.
Instead of nesting callbacks inside each other, break the code into smaller, reusable functions. This reduces complexity and increases readability.
Example:
function readFile(fileName, callback) {
fs.readFile(fileName, 'utf8', (err, data) => {
if (err) {
callback(err);
return;
}
callback(null, data);
});
}
readFile('file1.txt', (err, data1) => {
if (err) {
console.error('Error:', err);
return;
}
readFile('file2.txt', (err, data2) => {
if (err) {
console.error('Error:', err);
return;
}
console.log('Data from file2:', data2);
});
});
This modular approach reduces nesting by moving repeated logic into separate functions.
Promises provide a cleaner way to handle asynchronous operations by allowing you to chain .then() and .catch() blocks. This improves readability and error handling.
Example:
const readFile = (fileName) => {
return new Promise((resolve, reject) => {
fs.readFile(fileName, 'utf8', (err, data) => {
if (err) {
reject(err);
} else {
resolve(data);
}
});
});
};
readFile('file1.txt')
.then((data1) => {
console.log('File1 content:', data1);
return readFile('file2.txt');
})
.then((data2) => {
console.log('File2 content:', data2);
})
.catch((err) => {
console.error('Error reading file:', err);
});
By using promises, the flow of asynchronous operations is much clearer, and errors are handled more effectively.
Async/await is a modern feature in JavaScript that simplifies working with asynchronous code. It allows you to write asynchronous code that looks and behaves like synchronous code, making it easier to read and debug.
Example:
const fs = require('fs').promises;
async function readFiles() {
try {
const data1 = await fs.readFile('file1.txt', 'utf8');
console.log('File1 content:', data1);
const data2 = await fs.readFile('file2.txt', 'utf8');
console.log('File2 content:', data2);
} catch (err) {
console.error('Error:', err);
}
}
readFiles();
The await keyword pauses execution until the promise resolves, resulting in code that looks like it's synchronous but is still non-blocking.
There are several third-party libraries, such as Async.js, that help manage complex asynchronous workflows by providing functions for controlling flow, handling parallel tasks, and managing nested operations.
Example using Async.js:
const async = require('async');
async.series([
(callback) => {
fs.readFile('file1.txt', 'utf8', callback);
},
(callback) => {
fs.readFile('file2.txt', 'utf8', callback);
}
], (err, results) => {
if (err) {
console.error('Error:', err);
} else {
console.log('Results:', results);
}
});
Async.js offers a range of methods to run asynchronous tasks in parallel, series, or with other control flow options, helping to reduce callback hell.
Error handling is crucial when working with callbacks in Node.js, especially because Node.js uses asynchronous operations that might fail due to various reasons, such as a missing file, a failed network request, or an incorrect database query. Ensuring that errors are properly handled prevents your application from crashing and allows you to respond to unexpected issues.
In Node.js, error handling in callbacks follows a common pattern known as the Error-First Callback convention. This pattern ensures that the first argument passed to the callback is reserved for an error (if one occurs), and the subsequent arguments are used for the results.
The standard way to handle errors in callbacks is always to pass an error object as the first parameter. If no error occurs, this parameter should be null or undefined. The subsequent parameters represent the result of the operation.
Example:
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
Return; // Early return if there is an error
}
console.log('File content:', data);
});
In Node.js, callbacks are widely used for handling asynchronous operations. However, callbacks can lead to issues like callback hell (nested callbacks becoming difficult to manage). To overcome these issues, alternatives to callbacks have been introduced that make asynchronous programming more readable and maintainable.
Here are some of the most common alternatives to callbacks in Node.js:
Promises are a cleaner alternative to callbacks and allow you to handle asynchronous operations in a more structured way. A promise represents a value that may not be available yet but will be resolved at some point in the future. Promises make it easier to chain operations and handle errors.
const fs = require('fs').promises;
fs.readFile('file.txt', 'utf8')
.then((data) => {
console.log('File content:', data);
})
.catch((err) => {
console.error('Error reading file:', err);
});
Promises help avoid nested callbacks and can chain multiple asynchronous operations in a linear, readable manner.
Async/await is a syntactic sugar built on top of promises that makes asynchronous code look and behave like synchronous code. It allows you to use await to pause the execution of an asynchronous operation and handle the result in a more readable manner. Async functions always return a promise.
const fs = require('fs').promises;
async function readFileContent() {
try {
const data = await fs.readFile('file.txt', 'utf8');
console.log('File content:', data);
} catch (err) {
console.error('Error reading file:', err);
}
}
readFileContent();
Async/await makes code look more synchronous, reduces indentation, and improves readability compared to callbacks and promises.
The EventEmitter class is a part of Node.js's core events module. It allows you to handle events and their associated listeners, providing an alternative to callbacks, especially when you need to handle multiple listeners for specific events.
const EventEmitter = require('events');
const myEmitter = new EventEmitter();
// Listen for an event
myEmitter.on('event', () => {
console.log('An event occurred!');
});
// Emit an event
myEmitter.emit('event');
In this example, an event listener is added for the event, and a callback function is invoked when the event is emitted. This pattern is useful when you need to handle multiple events or work with an event-driven architecture.
Streams are a powerful feature in Node.js that handles asynchronous data processing. Streams allow data to be read or written in chunks, and they are highly efficient for handling large amounts of data. Streams are a good alternative to callbacks when processing large files, HTTP requests, or other data-intensive tasks.
const fs = require('fs');
const readableStream = fs.createReadStream('largefile.txt', 'utf8');
// Reading data from a file stream
readableStream.on('data', (chunk) => {
console.log('Received chunk:', chunk);
});
readableStream.on('end', () => {
console.log('File read completed.');
});
Streams allow you to handle large data asynchronously without blocking the execution of the program, and they help avoid callback nesting.
RxJS is a library for reactive programming that allows you to compose asynchronous and event-based programs using observable sequences and operators. It provides a more functional approach to handling asynchronous code, and you can use operators like map, filter, mergeMap, and concatMap to manipulate streams of data.
const { of } = require('rxjs');
const { map } = require('rxjs/operators');
of(1, 2, 3, 4)
.pipe(map(x => x * 2))
.subscribe(console.log); // Output: 2, 4, 6, 8
RxJS enables functional handling of asynchronous data and offers powerful operators to manage complex async workflows. It’s particularly useful when working with multiple asynchronous events and streams of data.
For CPU-bound tasks, Node.js provides worker threads that allow you to run JavaScript code in parallel threads, avoiding blocking the event loop. This is useful when you need to perform complex computations asynchronously.
const { Worker, isMainThread, parentPort } = require('worker_threads');
if (isMainThread) {
const worker = new Worker(__filename);
worker.on('message', (message) => {
console.log('Received from worker:', message);
});
worker.postMessage('Hello, worker!');
} else {
parentPort.on('message', (message) => {
console.log('Received in worker:', message);
parentPort.postMessage('Hello, main thread!');
});
}
Worker threads provide a way to offload CPU-intensive tasks to separate threads, improving the performance of your application.
In some cases, you may find it useful to combine callbacks with promises, especially when dealing with legacy code. Some Node.js libraries still rely on callbacks, but you can easily convert them into promises using Promise constructors or libraries like util. Promiscuity.
const util = require('util');
const fs = require('fs');
const readFilePromise = util.promisify(fs.readFile);
readFilePromise('file.txt', 'utf8')
.then((data) => {
console.log('File content:', data);
})
.catch((err) => {
console.error('Error reading file:', err);
});
Here, we convert the fs.readFile callback function into a promise-based function using util. Promiscuity, making it easier to chain and handle asynchronously.
Callbacks are integral to Node.js, especially because Node.js uses an asynchronous, non-blocking I/O model. Below are some common use cases of callbacks in Node.js:
Node.js provides the fs (file system) module to work with files. Operations like reading, writing, and modifying files are asynchronous by default, and callbacks are used to handle the results.
Example:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.log('Error reading file:', err);
return;
}
console.log('File content:', data);
});
In this case, the callback handles the file content once the file is successfully read, or it handles the error if the file doesn't exist or can't be read.
Database interactions in Node.js are typically asynchronous. When querying a database, a callback is used to handle the query result (success or failure). For example, libraries like MongoDB or MySQL use callbacks for their asynchronous methods.
Example (using MongoDB):
const MongoClient = require('mongodb').MongoClient;
MongoClient.connect('mongodb://localhost:27017/mydb', (err, db) => {
if (err) {
console.log('Error connecting to database:', err);
return;
}
const collection = db.collection('users');
collection.findOne({ name: 'John' }, (err, user) => {
if (err) {
console.log('Error querying database:', err);
return;
}
console.log('User data:', user);
});
});
In this example, callbacks handle the database connection and query result.
In web development, HTTP requests are often asynchronous. For both client-side and server-side HTTP operations, callbacks are used to handle the response once the request is completed.
Example (HTTP request in Node.js server):
const http = require('HTTP);
const server = http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, World!\n');
});
server.listen(8080, '127.0.0.1', () => {
console.log('Server is running at http://127.0.0.1:8080/');
});
Here, the callback is inside the server.l isten() is invoked when the server starts listening on the specified port.
Node.js uses an event-driven architecture. Event listeners in Node.js rely on callbacks to handle events asynchronously. For example, the events module allows you to create custom events and register callbacks to handle them when they are emitted.
Example:
const EventEmitter = require('events');
const myEmitter = new EventEmitter();
myEmitter.on('event', () => {
console.log('An event occurred!');
});
myEmitter.emit('event');
In this case, the callback function is triggered when the 'event' is emitted.
Node.js provides functions like setTimeout() and setInterval() for delayed or repeated actions, which require callbacks to handle the action when the timer expires or the interval fires.
Example (using setTimeout):
setTimeout(() => {
console.log('This message is displayed after 2 seconds');
}, 2000);
The callback is executed after the specified delay (2000 ms in this example).
Node.js allows spawning child processes to execute commands asynchronously. The child_process module uses callbacks to handle results when the child process completes.
Example:
const { exec } = require('child_process');
Exec ('l,' (err, stdout, stderr) => {
if (err) {
console.error('Error executing command:', err);
return;
}
console.log('Output:', stdout);
});
In this example, the callback handles the output of the ls command.
Streams in Node.js (such as reading from a file or writing data to a server) are asynchronous and use callbacks to process the data as it flows.
Example (using a readable stream):
const fs = require('fs');
const readableStream = fs.createReadStream('largefile.txt', 'utf8');
readableStream.on('data', (chunk) => {
console.log('Received chunk:', chunk);
});
readableStream.on('end', () => {
console.log('File read completed.');
});
The callback inside the data event handles each chunk of data as it's read from the file.
In web applications, callbacks are often used to handle authentication and authorization tasks. For example, after a user submits login credentials, a callback function might process the response and determine whether the credentials are valid.
Example:
function authenticate(username, password, callback) {
// Simulate checking credentials asynchronously
setTimeout(() => {
if (username === 'admin' && password === 'password123') {
callback(null, 'Authentication successful');
} else {
callback('Invalid credentials', null);
}
}, 1000);
}
authenticate('admin', 'password123', (err, message) => {
if (err) {
console.log(err);
} else {
console.log(message);
}
});
Callbacks are a fundamental concept in Node.js that enable asynchronous programming, allowing the application to handle multiple operations concurrently without blocking the execution of other tasks. By passing functions as arguments to other functions, callbacks provide a way to execute code after an asynchronous operation completes.
However, the callback pattern, while powerful, comes with its challenges. Callback hell or pyramid of doom can make the code harder to maintain and read, especially when callbacks are deeply nested. To mitigate these issues, alternatives like Promises and async/await have become popular in modern JavaScript, offering more manageable and readable ways to handle asynchronous flows.
Copy and paste below code to page Head section
A callback in Node.js is a function that is passed as an argument to another function, which is then invoked once an asynchronous operation is complete. It helps manage the asynchronous nature of Node.js by enabling the execution of code after a task finishes without blocking other operations.
Callbacks are essential in Node.js because they handle asynchronous operations. Since Node.js operates on a single-threaded event loop, callbacks ensure that the application can perform multiple tasks concurrently without waiting for each operation to complete sequentially, thus improving performance.
Callback hell, also known as the pyramid of doom, occurs when callbacks are nested inside one another, making the code hard to read, understand, and maintain. It can be avoided by: Modularizing code into smaller functions. Using Promises or async/await to flatten nested callbacks.
The error-first callback pattern is a convention where the first argument of a callback is reserved for an error, and the subsequent arguments are reserved for the result. This pattern makes error handling easier and ensures that errors are handled before any results are processed.
Yes, callbacks can be used in combination with Promises in Node.js. You can use Promises to avoid deep nesting of callbacks and still handle asynchronous tasks. The Node.js core API, such as fs.readFile, can also be provisioned to return promises instead of using callbacks.
Callback Hell: When callbacks are deeply nested, the code becomes harder to maintain. Error handling: If error handling is not managed properly, it may lead to unexpected crashes or bugs. Inconsistent API design: Some libraries still use callbacks while others use Promises, leading to inconsistency in the codebase.