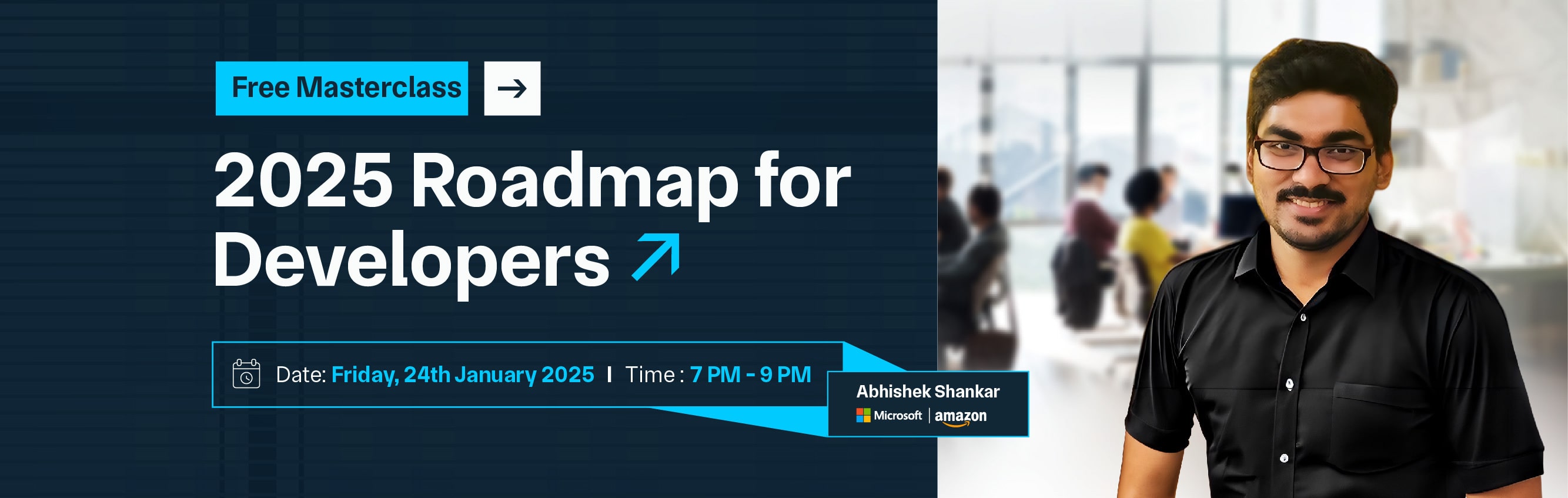

In MongoDB, projection refers to the process of selecting specific fields from documents in a query rather than retrieving the entire document. This helps optimize performance by reducing the amount of data transferred over the network, which is particularly useful for large datasets. Projection is commonly used to retrieve only the necessary fields, minimizing the load on both the database and the application.
To use projection, you include a second argument in your query, specifying which fields to include or exclude. For example, to retrieve only the name and age fields from a collection of user documents, you would use a query like db. Users.find({}, {name: 1, age: 1}). Setting a field to 1 means "include" it, while 0 means "exclude" it. By default, the _id field is included unless explicitly excluded.
Projection also supports advanced operations, such as computed fields or array slicing, allowing for even more flexible queries. However, it's important to note that MongoDB does not allow both including and excluding fields in the same projection (except for the _id field). Effective use of projection is key to improving query performance and resource efficiency in MongoDB applications.
MongoDB Projection is the process of specifying which fields to include or exclude in the documents returned by a query. Instead of retrieving the entire document, which may contain unnecessary data, projection allows you to retrieve only the fields that are relevant to your application.
This reduces the amount of data transferred from the database and can significantly improve query performance, especially when working with large datasets. In MongoDB, projection is specified in the second argument of the find() query. You can include or exclude fields by setting them to 1 (include) or 0 (exclude). For example:
Projection can also be used for more advanced features, such as limiting array elements or applying mathematical operations to fields, allowing for flexible and efficient querying of MongoDB collections.
MongoDB Projection works by specifying which fields you want to include or exclude when querying documents from a collection. By default, MongoDB returns all fields of a document, but using projection, you can refine the data returned to improve performance, reduce network overhead, and make queries more efficient.
Including Fields: To include specific fields in the result, you use 1 in the projection object. For example, to return only the name and age fields of each document, you would write:
Db. collection.find({}, { name: 1, age: 1 })
1. This tells MongoDB to include the name and age fields in the result while excluding all others. Note that by default, the _id field is included unless explicitly excluded.
Excluding Fields: If you want to exclude certain fields, you use 0 in the projection object. For instance, to exclude the _id field, you would write:
Db. collection.find({}, { _id: 0 })
2. You can exclude any other fields the same way, but MongoDB does not allow excluding both some fields and the _id field at the same time.
3. Limitations:
4. Advanced Projection: MongoDB projection also supports advanced use cases:
Array Slicing: You can limit the number of elements returned from an array in a document using array slicing. For example:
Db. collection.find({}, { items: { $slice: 3 } })
Example:
Consider a collection of users with documents that look like this:
{ "_id": 1, "name": "Alice", "age": 30, "email": "alice@example.com" }
{ "_id": 2, "name": "Bob", "age": 25, "email": "bob@example.com" }
Projection to include only name and age:
Db.users.find({}, { name: 1, age: 1 })
This will return:
{ "_id": 1, "name": "Alice", "age": 30 }
{ "_id": 2, "name": "Bob", "age": 25 }
Projection to exclude email:
Db. users.find({}, { email: 0 })
This will return:
{ "_id": 1, "name": "Alice", "age": 30 }
{ "_id": 2, "name": "Bob", "age": 25 }
MongoDB Projection allows you to fine-tune the data returned by your queries, improving both performance and efficiency by only returning the fields that are necessary for your application.
MongoDB offers several projection operators to provide advanced capabilities for selecting and transforming data in query results. These operators allow you to manipulate how fields are returned, slice arrays, and perform computations or transformations on specific fields. Here’s an overview of key MongoDB projection operators:
1. $elemMatch
Example:
Db. orders.find({}, { items: { $elemMatch: { quantity: { $gte: 10 } } } })
2. $slice
Example:
Db.users.find({}, { posts: { $slice: 5 } })
This would return only the first five elements from the posts array in each document.
You can also specify a starting point:
Db. users.find({}, { posts: { $slice: [2, 5] } })
3. $ (Positional Operator)
Example:
Db. orders.find({}, { "items.$": 1 })
4. Aggregation Operators in Projection (Using Aggregation Framework)
MongoDB’s aggregation framework can also be used to project and transform data in powerful ways. Some of these operators include:
Example:
Db.orders.aggregate([
{ $project: { totalPrice: { $multiply: ["$price", "$quantity"] } } }
])
This adds a computed total price field by multiplying price and quantity.
5. $type
Example:
Db.users.find({}, { "age": { $type: "int" } })
6. $cond (Conditional Operator)
Example:
Db.users.aggregate([
{
$project: {
status: {
$cond: { if: { $gte: ["$age," 18] }, then: "Adult," else: "Minor" }
}
}
}
])
7. $concat (String Concatenation)
Example:
Db. users.aggregate([
{
$project: {
fullName: { $concat: ["$firstName", " ", "$lastName"] }
}
}
])
8. $regex (Regular Expression)
Example:
Db.users.find({}, { email: { $regex: "@gmail.com$" } })
9. $literal
Example:
Db.orders.aggregate([
{
$project: {
status: { $literal: "Completed" }
}
}
])
10. $meta (Text Search Score)
Example:
Db. posts.find(
{ $text: { $search: "mongodb" } },
{ score: { $meta: "textScore" } }
)
MongoDB Projection Queries are used to specify which fields you want to include or exclude when retrieving documents. Projection helps in reducing the amount of data returned by a query, making it more efficient. Below are examples of various projection queries in MongoDB:
You can include specific fields in the result using 1 in the projection object.
Example: Retrieve only the name and age fields from a user's collection.
Db. users.find({}, { name: 1, age: 1 })
This returns:
{ "_id": 1, "name": "Alice", "age": 30 }
{ "_id": 2, "name": "Bob", "age": 25 }
You can exclude specific fields using 0 in the projection object.
Example: Retrieve all fields except the email field from a user's collection.
db.users.find({}, { email: 0 })
This returns:
{ "_id": 1, "name": "Alice", "age": 30 }
{ "_id": 2, "name": "Bob", "age": 25 }
By default, the _id field is included in query results. If you want to exclude it, explicitly set it to 0.
Example: Retrieve name and age but exclude _id.
Db. users.find({}, { _id: 0, name: 1, age: 1 })
This returns:
{ "name": "Alice", "age": 30 }
{ "name": "Bob", "age": 25 }
You can include some fields while excluding others (except _id, which can be excluded by default).
Example: Retrieve name and exclude email but include all other fields.
Db. users.find({}, { name: 1, email: 0 })
This returns:
{ "_id": 1, "name": "Alice", "age": 30 }
{ "_id": 2, "name": "Bob", "age": 25 }
The $slice operator is used to limit the number of elements returned from an array in a document.
Example: Retrieve the first three elements of the items array from each document in the orders collection.
db.orders.find({}, { items: { $slice: 3 } })
This returns:
{ "_id": 1, "items": [ { "product": "item1", "quantity": 5 }, { "product": "item2", "quantity": 10 }, { "product": "item3", "quantity": 3 }] }
{ "_id": 2, "items": [ { "product": "item4", "quantity": 7 }, { "product": "item5", "quantity": 12 }, { "product": "item6", "quantity": 2 }] }
The $elemMatch operator returns the first array element that matches the specified query condition.
Example: Retrieve documents where the items array contains at least one element with a quantity greater than or equal to 10.
db.orders.find({}, { items: { $elemMatch: { quantity: { $gte: 10 } } } })
This returns:
{ "_id": 1, "items": [ { "product": "item2", "quantity": 10 } ] }
{ "_id": 2, "items": [ { "product": "item5", "quantity": 12 } ] }
In the aggregation framework, $project is used to reshape documents by adding new fields, modifying existing ones, or excluding fields.
Example: Use $project to calculate a totalPrice field from price and quantity in an orders collection.
Db. orders.aggregate([
{
$project: {
totalPrice: { $multiply: ["$price", "$quantity"] }
}
}
])
This returns:
{ "_id": 1, "totalPrice": 100 }
{ "_id": 2, "totalPrice": 250 }
The $cond operator is used for conditional logic during projection, allowing you to modify fields conditionally.
Example: Create a new field status based on the age field, setting it to "Adult" if age >= 18 and "Minor" otherwise.
Db. users.aggregate([
{
$project: {
status: {
$cond: {
if: { $gte: ["$age", 18] },
then: "Adult,"
else: "Minor"
}
}
}
}
])
This returns:
{ "_id": 1, "status": "Adult" }
{ "_id": 2, "status": "Minor" }
The $concat operator is used to combine string fields into a single field during projection.
Example: Concatenate firstName and lastName into a new field fullName.
Db. users.aggregate([
{
$project: {
fullName: { $concat: ["$firstName", " ", "$lastName"] }
}
}
])
This returns:
{ "_id": 1, "fullName": "Alice Johnson" }
{ "_id": 2, "fullName": "Bob Smith" }
The $meta operator is used to return the score of a text search query.
Example: Perform a text search and return documents with the text search score.
Db. posts.find(
{ $text: { $search: "mongodb" } },
{ score: { $meta: "textScore" } }
)
This returns:
{ "_id": 1, "title": "MongoDB Basics", "score": 1.5 }
{ "_id": 2, "title": "Learning MongoDB", "score": 1.2 }
MongoDB Projection is a powerful feature that allows you to control which fields are included or excluded in the results of a query. By specifying which fields to return, projection helps optimize performance, reduces data transfer, and improves the efficiency of database operations. Whether you're working with simple queries or complex aggregation pipelines, projection gives you fine-grained control over the data you retrieve.
Copy and paste below code to page Head section
MongoDB Projection is the process of selecting specific fields to include or exclude in the documents returned by a query. It allows you to control the shape of the data, improving query performance and reducing the amount of data transferred between the server and client.
In MongoDB, projection is specified in the second argument of the find() query. To include a field, set it to 1, and to exclude it, set it to 0. Include specific fields: db.collection.find({}, { name: 1, age: 1 }) Exclude specific fields: db.collection.find({}, { email: 0 })
es, you can exclude the _id field by explicitly setting it to 0 in the projection: Db. collection.find({}, { _id: 0 })
No, MongoDB does not allow you to include and exclude fields at the same time, except for the _id field. Suppose you want to exclude the _id while including other fields; you must explicitly set _id: 0.
The $slice operator is used to limit the number of elements returned from an array. For example, if you want to return only the first three elements of an array, you can use $slice: Db.collection.find({}, { items: { $slice: 3 } })
The $elemMatch operator is used to return a single element from an array that matches a specific condition. This allows you to filter array elements based on the criteria: Db.orders.find({}, { items: { $elemMatch: { quantity: { $gte: 10 } } } })