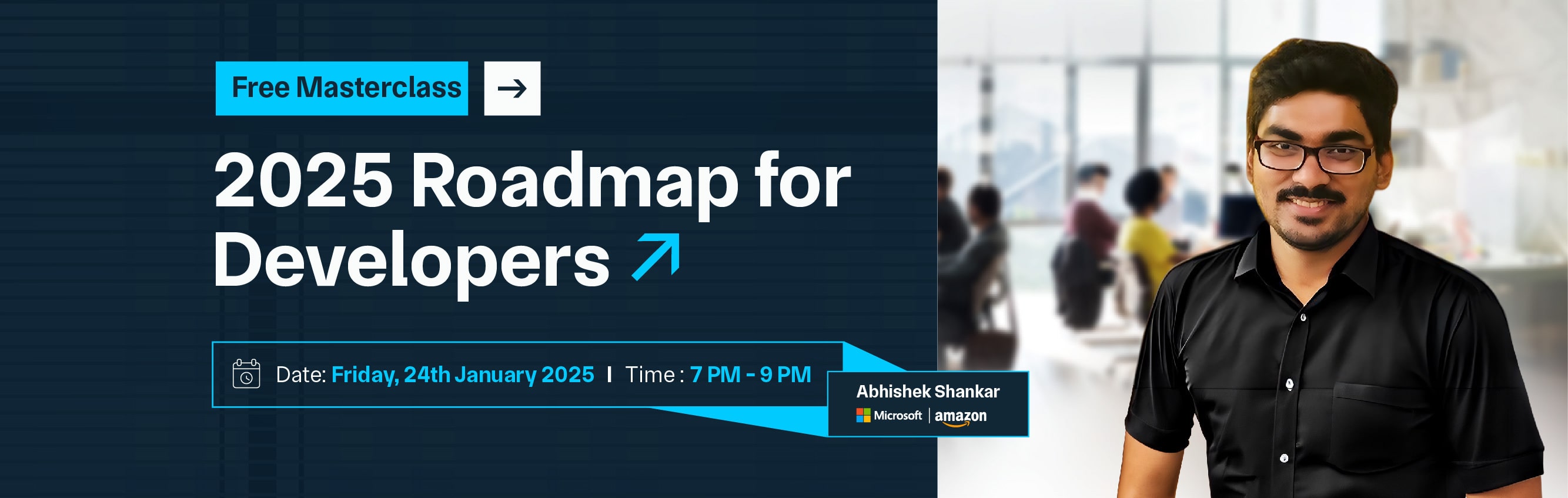

Preparing for a Java developer interview requires a combination of technical expertise, problem-solving skills, and effective communication. With Java being one of the most widely used programming languages, employers seek candidates who not only possess strong coding abilities but also understand the ecosystem around Java. Knowing how to articulate your knowledge and showcase your skills can make a significant difference during the interview process.
Start by mastering the core concepts of Java, including object-oriented programming, data structures, algorithms, and multithreading. Employers often test candidates on these fundamental areas, so brushing up on these topics is essential. Additionally, familiarize yourself with frameworks like Spring and Hibernate, as well as tools such as Maven and Jenkins. A hands-on understanding of these tools can demonstrate your readiness to handle real-world projects effectively.
Beyond technical skills, practice coding problems on platforms like LeetCode and HackerRank to improve problem-solving abilities. Simultaneously, prepare for behavioral questions that assess your teamwork, adaptability, and conflict-resolution skills. Mock interviews can help you refine your answers and build confidence. Remember, success in a Java developer interview is about balancing technical expertise with the ability to communicate your ideas clearly and collaborate effectively.
Preparing for a Java interview requires a thorough understanding of Java fundamentals, advanced concepts, and practical implementation skills. Employers look for candidates who can demonstrate proficiency in object-oriented programming, problem-solving, and familiarity with the latest Java tools and frameworks. A strong grasp of both theoretical knowledge and hands-on coding abilities is essential to stand out in the competitive hiring process.
To ace your Java interview, focus on a wide range of topics that showcase your technical expertise and understanding of industry practices. These include foundational concepts like core Java and multithreading, as well as modern frameworks and tools like Spring Boot and Maven. Covering these areas ensures you are well-prepared to answer both theoretical and practical questions. Below is a list of the top 25 topics to prepare for Java interviews.
Core Java Basics provides the essential building blocks for understanding and working with Java. These include fundamental concepts such as syntax, data types, operators, and control flow structures like loops and conditionals. Grasping these concepts ensures a solid foundation for writing efficient and error-free programs. Additionally, familiarity with methods, arrays, and string manipulation is crucial for solving real-world problems effectively. A deep understanding of these basics not only helps in coding but also builds confidence for tackling more advanced topics.
Beyond just syntax, Core Java Basics introduces you to the object-oriented nature of Java, providing the groundwork for understanding complex concepts like inheritance and polymorphism. They also cover Java's platform-independent characteristics enabled by the JVM. Exploring these aspects early on ensures you comprehend Java's architecture and how it facilitates cross-platform development. Interviewers often test these foundational skills through problem-solving tasks to evaluate your readiness for higher-level Java challenges.
Object-oriented programming is the core paradigm that makes Java a versatile and powerful language. It revolves around principles like encapsulation, inheritance, polymorphism, and abstraction. Encapsulation ensures that data is protected and only accessible through defined methods, while inheritance allows the creation of new classes based on existing ones, promoting reusability. Polymorphism enables the implementation of multiple forms of a method or object, enhancing flexibility and scalability in programming.
Abstraction helps in focusing on essential features while hiding unnecessary details, simplifying the development process. Mastering OOP concepts is crucial as they form the foundation of Java application development. Most real-world applications rely on these principles for modularity and maintainability. Interviewers often evaluate candidates' ability to apply OOP principles through scenario-based questions and coding tasks, testing their understanding of designing effective, reusable, and scalable solutions. Proficiency in OOP not only enhances your problem-solving skills but also ensures you can handle complex programming challenges in Java.
Data Structures and Algorithms (DSA) are vital for building efficient and optimized Java applications. Data structures like arrays, linked lists, stacks, queues, and trees help in organizing and managing data systematically. Algorithms such as sorting, searching, and dynamic programming provide the logic for solving computational problems. A strong grasp of DSA is crucial for writing code that is not only correct but also efficient in terms of time and space complexity.
Employers frequently test candidates on DSA to assess their problem-solving abilities. Proficiency in these topics demonstrates your ability to handle large datasets, optimize performance, and troubleshoot issues in applications. Mastery of DSA also aids in better understanding advanced frameworks and libraries in Java, as they are built upon these core principles. Regular practice on coding platforms can help you sharpen your DSA skills and build confidence for Java interviews.
The Java Collections Framework is an essential part of Java programming that provides a set of classes and interfaces to handle collections of objects. It offers various data structures, such as lists, sets, maps, and queues, which help simplify the storage, retrieval, and manipulation of data. Classes like ArrayList, HashSet, and HashMap offer efficient ways to manage large volumes of data, allowing for optimal performance and scalability. By utilizing these pre-built data structures, developers can focus more on business logic rather than the complexities of implementing data structures from scratch.
In interviews, candidates are often asked to explain how the different collections work, their time and space complexity, and how they differ from one another. For instance, understanding how a HashMap resolves collisions or the performance differences between an ArrayList and a LinkedList is essential. Proficiency in the Java Collections Framework helps developers design dynamic, scalable applications and is key to answering both theoretical and practical questions in Java interviews.
Exception Handling in Java is crucial for building robust applications. It enables developers to manage runtime errors, such as incorrect user input or system failures, in a controlled way. Key constructs like try-catch blocks, finally statements, and custom exceptions allow developers to handle unexpected situations without crashing the program. Exception handling ensures that errors are properly logged, resources are freed up, and the application can continue functioning or fail gracefully.
During interviews, candidates are tested on their ability to create custom exceptions, implement proper error-handling strategies, and write clean, maintainable code. Interviewers often ask about specific scenarios like handling input validation errors or managing resource failures effectively. Mastery in exception handling demonstrates your ability to write resilient, user-friendly applications that anticipate potential issues, thus making your code more robust and reliable.
Multithreading and concurrency in Java enable efficient execution of multiple threads at the same time, optimizing performance in multi-core processors. Concepts such as thread creation, synchronization, locks, and thread-safe collections are vital for solving complex concurrency issues like race conditions, deadlocks, and thread safety. Java provides robust tools like the Executor framework, synchronized blocks, and Java. Util. Concurrent package to simplify thread management and improve performance.
Interviewers often focus on the candidate’s ability to handle issues like thread lifecycle, synchronization, and the prevention of deadlocks. Understanding how to design and implement multithreaded applications that are efficient and thread-safe is crucial. Mastery of multithreading and concurrency shows that you can build high-performing, scalable Java applications, which is particularly important in enterprise-level or performance-critical systems.
Introduced in Java 8, the Stream API allows developers to process data in a functional programming style, enabling more concise and readable code. It offers methods like filter(), map(), and reduce() to perform complex operations on collections, making code more expressive and easier to maintain. Streams allow for both sequential and parallel processing, optimizing performance by taking advantage of multi-core processors. Lazy evaluation ensures that computations are only performed when necessary, reducing overhead.
In interviews, candidates are often asked to demonstrate their knowledge of transforming data using the Stream API, such as filtering collections or aggregating results. They may also be asked about the performance differences between sequential and parallel streams, as well as their use cases. A strong understanding of the Stream API highlights your ability to write modern, efficient Java code that leverages functional programming principles for cleaner, more maintainable applications.
Lambda expressions, introduced in Java 8, allow developers to write more compact and readable code by representing anonymous functions. They simplify operations on collections, particularly when combined with the Stream API. A lambda expression consists of a parameter list, the arrow token (->), and a body, which can contain a single or multiple statements. Lambda expressions are commonly used with functional interfaces like Predicate, Function, and Consumer.
In interviews, candidates are frequently asked to demonstrate their understanding of lambda expressions by implementing functional-style code. You might be asked about their syntax, usage in collections, or their interaction with streams. Mastery of lambda expressions indicates your ability to write modern, concise Java code that reduces boilerplate, making your applications cleaner and more efficient, which is highly valued in contemporary software development.
Generics provide a way to ensure type safety in Java, enabling classes, methods, and interfaces to work with different data types while preventing runtime errors caused by type casting. By using generics, you can write reusable and flexible code that operates on objects of various types. Key features of generics include bounded type parameters, wildcard arguments, and generic methods, which add significant flexibility and safety to your code.
Interviewers often test candidates on their understanding of generics, particularly in the context of collections and custom classes. You may be asked to write generic methods or explain how to use generics to ensure type safety and prevent common issues like ClassCastException. Proficiency in generics demonstrates your ability to write robust, reusable, and type-safe Java code, which is essential for building scalable applications.
The Java Virtual Machine (JVM) is responsible for executing Java bytecode and ensuring platform independence. It abstracts the underlying operating system and hardware, allowing Java programs to run on any platform that has a JVM installed. The JVM handles memory management, garbage collection, and the execution of Java bytecode, enabling developers to focus on writing code without worrying about platform-specific issues.
In interviews, candidates are often asked to explain the architecture of the JVM, including components like the class loader, execution engine, and memory areas (e.g., heap, stack, method area). Understanding how the JVM works and its impact on application performance, such as the effects of garbage collection or memory allocation, is vital. Expertise in JVM internals helps you optimize Java applications, troubleshoot performance issues, and ensure your code runs efficiently across different platforms.
Memory Management in Java plays a pivotal role in ensuring efficient application performance. The JVM handles memory through automatic garbage collection, which reclaims unused objects to optimize memory usage. Java developers need to understand memory areas such as the heap, stack, and method area to design applications that minimize memory leaks and optimize resource utilization. Properly managing memory is especially important in large-scale applications where performance can be significantly impacted by inefficient memory handling.
In Java, memory management involves understanding the life cycle of objects, the allocation of memory during runtime, and the role of garbage collectors in reclaiming memory that is no longer in use. Developers can fine-tune memory management by configuring JVM parameters and using tools like profilers and heap dumps to identify memory consumption patterns. Proficiency in this area helps in building high-performance, scalable systems and is frequently tested in interviews through questions that explore memory leak detection and optimization techniques.
Serialization and Deserialization are processes in Java that involve converting objects into a byte stream and vice versa. Serialization is crucial for tasks such as saving object states or transmitting objects over a network. Deserialization reverses the process of reconstructing objects from the byte stream. The Serializable interface in Java facilitates serialization, allowing classes to convert objects into a format that can be easily stored or transferred.
Understanding the nuances of Serialization and Deserialization is essential for handling Java objects efficiently in distributed systems, databases, and file I/O operations. Interview questions on this topic may involve implementing custom serialization logic, dealing with versioning issues, and ensuring security during deserialization. Java developers are often asked to explain how to maintain compatibility across different versions of serialized objects, a critical aspect of evolving systems. Knowledge of best practices for managing the serialization process ensures that your applications are secure, maintainable, and performant.
File Handling in Java is an essential skill for developers working with file-based data storage. Java provides robust APIs for reading from and writing to files, such as the File, FileReader, FileWriter, BufferedReader, and BufferedWriter classes. These APIs allow developers to manage files effectively, whether handling text, binary, or serialized objects. File handling in Java also involves understanding file system paths, directories, and basic file operations like creation, deletion, and modification.
In interviews, developers are often asked to demonstrate their ability to manipulate files using Java’s I/O API. Questions might focus on efficiently reading large files, handling file exceptions, or performing operations on multiple files simultaneously. Proficiency in file handling is crucial for building applications that interact with external data, such as processing log files, importing/exporting data, or building file-based data systems. Mastery of this topic showcases your ability to handle real-world scenarios that require effective data management.
Java 8 introduced several powerful features that significantly enhanced the language, including the Stream API, Lambda expressions, and Java. Time package for date and time operations. These features enable developers to write more concise, efficient, and functional-style code. The Optional class helps prevent NullPointerException and improve readability, while new APIs like the CompletableFuture class facilitate easier handling of asynchronous tasks.
In interviews, employers often assess candidates' knowledge of Java 8 features, as they represent the modern Java programming style. Developers are typically asked how to use these features to simplify code and improve performance, as well as how to handle challenges like backward compatibility. Mastering Java 8 features not only demonstrates your ability to use modern Java effectively but also shows that you can write cleaner, more maintainable code that aligns with industry best practices.
Design Patterns in Java provide proven solutions to common design problems encountered during software development. Some well-known patterns include Singleton, Factory, Observer, and Strategy. These patterns help developers create flexible, reusable, and maintainable code. The correct use of design patterns can drastically improve code quality, making it easier to extend, maintain, and understand.
In interviews, candidates are often asked to explain and implement various design patterns in Java, testing their understanding of software architecture and design principles. Interviewers may present scenario-based questions that require applying a design pattern to solve a specific problem, assessing the candidate’s ability to choose the most appropriate pattern based on the context. Proficiency in design patterns demonstrates that you can create software systems that are not only functional but also scalable and maintainable over time.
Annotations in Java provide metadata that can be used to influence the behavior of code at runtime or during compilation. Java offers several built-in annotations, such as @Override, @Deprecated, and @SuppressWarnings, to help with code maintenance and readability. Custom annotations can also be created for more specialized use cases, such as generating documentation or defining custom behavior in frameworks.
Interview questions on annotations may involve explaining how and why to use specific annotations, as well as how to create custom ones for various purposes. Understanding annotations is especially important for developers working with frameworks like Spring, Hibernate, or JUnit, which heavily rely on them. Mastery of annotations shows that you can leverage the full potential of Java’s reflection capabilities and streamline development processes, such as automatic dependency injection or unit testing.
The Reflection API in Java allows developers to inspect and modify the properties of classes, methods, fields, and constructors during runtime. This powerful feature makes it possible to access information about classes that are not known at compile time, enabling flexible and dynamic programming. Reflection is often used in frameworks like Spring and Hibernate for operations such as dependency injection and object mapping.
In interviews, candidates may be asked to explain the practical uses of reflection and how it works under the hood. Understanding reflection’s impact on performance and security is also essential, as it can lead to issues if not used correctly. Proficiency in the Reflection API indicates that you can build dynamic applications and frameworks that require runtime flexibility, making it an important skill for advanced Java development.
Java Database Connectivity (JDBC) is an API that allows Java applications to interact with databases. It provides a standard interface for connecting to relational databases, executing SQL queries, and retrieving results. JDBC supports all major relational databases such as MySQL, Oracle, and PostgreSQL. Through JDBC, developers can manage database connections, execute queries, and update records in a structured and efficient manner.
In interviews, candidates are often asked to demonstrate their ability to set up a database connection, execute queries, and handle SQL exceptions. Understanding JDBC transactions, connection pooling, and batch processing is essential for optimizing database operations. Additionally, knowledge of the difference between statement types like PreparedStatement and Statement is important, as these impact performance and security. Mastery of JDBC ensures that you can develop robust, database-driven applications that can handle large volumes of data and integrate smoothly with relational databases.
RESTful APIs are a crucial aspect of modern web services, and Spring Boot simplifies the process of building them in Java. By leveraging Spring Boot, developers can create scalable, maintainable, and efficient RESTful APIs with minimal configuration. Spring Boot automates many aspects of the setup process, allowing developers to focus on application logic and business functionality. Through annotations like @RestController and @RequestMapping, developers can easily map HTTP requests to Java methods.
In interviews, you may be asked to explain how to design and implement RESTful services using Spring Boot. Questions cover topics such as request mapping, JSON response handling, authentication, and exception management. Understanding how to design RESTful APIs for scalability, security, and performance optimization is essential. Proficiency in Spring Boot and REST APIs indicates that you can create efficient web services that form the backbone of modern enterprise applications.
Hibernate is a powerful Object-Relational Mapping (ORM) framework that allows Java developers to map Java objects to database tables and vice versa. It abstracts the complexities of direct database interactions and simplifies the development process by handling the conversion of data between the Java application and relational databases. Hibernate provides features like automatic dirty checking, caching, and connection pooling, which are critical for developing high-performance database-driven applications.
In interviews, developers are often asked to demonstrate their knowledge of Hibernate’s configuration, mapping annotations, and how to perform CRUD operations. Candidates may be asked to explain how Hibernate handles relationships between entities (e.g., one-to-many, many-to-many) and how it optimizes database queries through lazy loading and caching. Mastery of Hibernate helps developers create more maintainable, efficient, and scalable data access layers in Java applications and is frequently tested in Java-related interviews.
Microservices Architecture is a design approach where an application is broken down into smaller, independent services that communicate over a network. Each service is focused on a specific business capability, and they can be developed, deployed, and scaled independently. Microservices enhance the maintainability, scalability, and flexibility of applications and are often used in cloud-based systems. Developers must design APIs for inter-service communication and ensure that services are loosely coupled and can function autonomously.
In interviews, candidates may be asked about their experience in designing and implementing microservices using Java technologies like Spring Boot and Docker. Key topics include service discovery, API gateway, fault tolerance, and distributed transaction management. Developers should also be able to explain how microservices are deployed in containerized environments, how to handle inter-service communication with protocols like REST and messaging queues, and how to monitor and scale microservices efficiently. Proficiency in a microservices architecture is a valuable skill for developers building modern, scalable, cloud-based systems.
Build tools like Maven and Gradle are essential for automating the build, testing, and deployment processes of Java applications. These tools allow developers to manage dependencies, configure project structure, and automate repetitive tasks such as compiling code, running tests, and packaging applications. Maven uses XML-based configuration files (pom.xml), while Gradle is based on Groovy and allows for more flexibility with its Domain-Specific Language (DSL).
In interviews, you may be asked about your experience with these build tools, including how to set up dependencies, create build profiles, and integrate testing frameworks. Understanding the differences between Maven and Gradle, as well as when to use each, is crucial. Additionally, interviewers might ask about advanced features such as continuous integration (CI) pipelines, artifact repositories, and multi-module project setups. Proficiency with Maven and Gradle helps developers streamline development workflows, ensuring faster and more reliable software delivery.
Testing is a critical component of software development, and frameworks like JUnit and Mockito play a key role in ensuring the reliability of Java applications. JUnit is a widely used testing framework that helps developers write repeatable tests for unit testing. At the same time, Mockito is a powerful mocking framework that allows developers to simulate dependencies in tests, making it easier to test individual components in isolation. Both frameworks are integral for ensuring that the code functions as expected and can be easily maintained.
In interviews, you may be asked to write unit tests using JUnit or Mockito, mock dependencies, and explain concepts like test-driven development (TDD), mock objects, and assertions. Understanding how to structure unit tests, how to mock external systems, and how to ensure that tests are reliable and easy to maintain is essential. Familiarity with testing frameworks shows that you can ensure the quality and reliability of your code, a key aspect of professional software development.
Garbage Collection in Java refers to the process of automatically reclaiming memory by identifying and deleting objects that are no longer needed. The Java Virtual Machine (JVM) manages memory through garbage collectors that run in the background, preventing memory leaks and improving application performance. Developers should have a clear understanding of how garbage collection works, including different garbage collection algorithms like G1, Parallel, and CMS.
In interviews, candidates are often asked to explain the role of garbage collection in Java, how to monitor and optimize garbage collection behavior, and how to troubleshoot memory-related issues like high latency or memory leaks. Understanding JVM flags for garbage collection tuning and how to configure the garbage collector for different use cases is crucial. Mastery of garbage collection techniques ensures that Java applications can scale efficiently and perform optimally without memory bottlenecks.
Security is a critical consideration for any Java application, especially when dealing with sensitive data or operating in a networked environment. Java provides a comprehensive set of APIs and libraries for implementing security features such as encryption, authentication, and authorization. Java Security Manager, Java Cryptography Architecture (JCA), and Java Secure Socket Extension (JSSE) are some of the tools used to protect data and applications from unauthorized access and malicious activities.
In interviews, candidates may be asked to demonstrate their understanding of securing Java applications using encryption algorithms, SSL/TLS, and secure coding practices. Key topics may include handling user authentication, securing data in transit and at rest, and preventing common vulnerabilities such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Mastery of security best practices is essential for building robust, secure applications that can protect sensitive data and maintain user trust in production environments.
When preparing for a Java interview, it's important to focus on a wide range of questions that cover both foundational concepts and advanced topics. Java interviews typically test a candidate’s understanding of core Java, object-oriented principles, data structures, algorithms, and the language’s unique features. Being well-versed in these areas allows you to demonstrate your technical expertise and problem-solving skills.
In addition to technical knowledge, Java interviews often include questions related to real-world scenarios, code optimization, and system design. Having a strong grasp of these areas will allow you to handle questions confidently and efficiently. Below are the top 10 common Java interview questions, each designed to test your proficiency and readiness for a Java development role.
This question is a common one in Java interviews, as it tests your understanding of Java's development environment and runtime execution. The Java Development Kit (JDK) is a complete package that includes tools needed for developing Java applications, such as compilers, debuggers, and libraries. The Java Runtime Environment (JRE) is a subset of the JDK and provides the necessary environment for running Java applications, including the JVM.
The Java Virtual Machine (JVM), a part of the JRE, is responsible for executing Java bytecode. Understanding these differences is important because each plays a specific role in the Java development and execution process. The JDK is used during development, the JRE is used for running applications, and the JVM is the engine that interprets and executes the bytecode. Being able to clearly explain these distinctions shows your solid foundation in Java fundamentals, an essential aspect of any Java developer role.
Object-oriented programming (OOP) is a fundamental concept in Java, and understanding its core principles is crucial for any Java developer. The four main principles of OOP are Encapsulation, Abstraction, Inheritance, and Polymorphism. Encapsulation involves bundling the data and methods that operate on that data into a single unit, typically a class, and restricting access to some of the object’s components. Abstraction simplifies complex systems by hiding unnecessary implementation details and exposing only essential functionalities.
Inheritance allows one class to inherit the properties and behaviors of another class, promoting code reuse and reducing redundancy. Polymorphism enables objects of different classes to be treated as objects of a common superclass, allowing for dynamic method binding. Mastery of these OOP principles is crucial for designing and implementing maintainable, scalable, and reusable Java applications. Interviewers may ask you to demonstrate these principles in action through practical coding questions or conceptual discussions.
Both abstract classes and interfaces are used to achieve abstraction in Java, but they serve different purposes and have distinct characteristics. An abstract class can have both abstract methods (without implementation) and concrete methods (with implementation), and it can also maintain state (fields). In contrast, an interface only allows abstract methods (until Java 8, when default and static methods were introduced), and it cannot have any state or instance variables.
Another key difference is that a class can extend only one abstract class but can implement multiple interfaces, making interfaces more flexible in certain scenarios. Understanding when to use an abstract class versus an interface is important for designing efficient and modular systems. For example, interfaces are ideal for defining common behaviors across unrelated classes, while abstract classes are suitable for sharing common functionality between closely related classes. This question tests your ability to apply the right abstraction technique in real-world scenarios.
In Java, the final keyword is used to define constants, prevent method overriding, and prevent inheritance. When applied to a variable, final ensures that its value cannot be changed after initialization, essentially making it a constant. If applied to a method, the final prevents the method from being overridden by subclasses, ensuring its behavior remains unchanged. Additionally, when used with a class, the final prevents the class from being subclassed, making it immutable.
The final keyword is an essential tool for enforcing security and maintaining control over your codebase. It is often used in situations where you want to ensure the integrity of a variable, method, or class. Understanding the final keyword's applications and the scenarios in which it should be used is crucial for writing reliable and maintainable code, and interviewers often ask about it to assess your familiarity with Java's best practices.
This keyword in Java is a reference to the current object within an instance method or constructor. It is used to differentiate between instance variables and parameters that have the same name. For example, if a constructor or method parameter has the same name as an instance variable, this keyword can be used to refer to the instance variable. Additionally, this can be used to invoke other constructors in the same class (known as constructor chaining).
It can also be used to pass the current object as an argument to other methods or constructors. This keyword is commonly used to improve code clarity and avoid ambiguity in situations where parameters and instance variables have the same name. Understanding the proper usage of this is a fundamental aspect of Java programming.
Memory management in Java is primarily handled by the JVM (Java Virtual Machine) through automatic garbage collection. The JVM divides memory into different areas, such as the heap, stack, and method area. Objects are created in the heap, and when they are no longer referenced, they become eligible for garbage collection. The garbage collector (GC) automatically frees memory by removing unused objects, thus reducing the burden on developers to manage memory manually.
Java’s memory management system helps prevent memory leaks, as the garbage collector ensures that objects that are no longer in use are cleared. However, developers are still responsible for managing stack memory by using local variables efficiently and minimizing object creation. An understanding of Java’s memory management is critical for optimizing performance and avoiding memory-related issues in large-scale applications.
In Java, == and .equals() are both used for comparison, but they differ in how they work. The == operator compares the memory addresses (reference equality) of two objects, meaning it checks whether two variables point to the same object in memory. On the other hand, the .equals() method is meant for logical equality. It checks whether two objects are meaningfully equal based on the values of their fields, not just their memory references.
Understanding this difference is crucial, especially when dealing with objects. For example, when comparing strings in Java, it’s important to use .equals() because == will only return true if the two string variables point to the same memory location, which may not always be the case. Knowing when to use each method ensures that comparisons in your code are done correctly.
Method overloading and method overriding are both used to achieve polymorphism in Java, but they work in different ways. Method overloading occurs when a class has multiple methods with the same name but different parameter lists (either in number, type, or both). This allows developers to perform similar actions with different inputs. The method signature (name and parameters) must differ for overloading to work.
Method overriding, on the other hand, involves defining a method in a subclass that has the same name and parameters as a method in the superclass. It allows the subclass to provide a specific implementation for the method that was already defined in the superclass. The @Override annotation is typically used to indicate that a method is being overridden. Understanding the differences between overloading and overriding is essential for implementing flexible and maintainable code.
A singleton class in Java is a class that allows only one instance of itself to be created and provides a global point of access to that instance. Singleton classes are often used for scenarios where only a single instance of a class is needed, such as in logging, thread pools, or configuration management. To implement a singleton class, the constructor is made private, and a static method is provided to return the single instance.
The singleton pattern ensures that a class has only one instance, which helps conserve memory and ensures that shared resources are used consistently. Understanding how to implement a singleton class and the potential pitfalls, such as thread safety and performance issues, is important for designing efficient, scalable systems. Interviewers often test your ability to implement a singleton pattern correctly in multi-threaded environments.
The super keyword in Java is used to refer to the superclass (parent class) of the current object. It can be used in several contexts: to call a constructor of the parent class, to access a method or variable of the superclass, or to override a method in the child class. Using super helps differentiate between a parent class’s members and those of the current class when there is a naming conflict.
In constructors, super() is used to invoke the parent class’s constructor. If no constructor is explicitly called, the default constructor of the parent class is invoked. The super keyword allows child classes to inherit and extend the functionality of their parent classes while maintaining clear code readability. Understanding the proper use of super is key for inheritance and method overriding in Java.
A Java coding interview assesses your problem-solving skills, programming knowledge, and ability to write clean, efficient code. It typically involves algorithmic challenges, data structure problems, and questions that test your understanding of Java’s core concepts. To succeed in a Java coding interview, candidates need to be proficient in writing code that is both functional and optimal.
Interviewers expect you to demonstrate your logical thinking, debugging skills, and ability to work under pressure. Familiarity with common interview problems, such as those involving arrays, strings, trees, and dynamic programming, is crucial for performing well. Being able to explain your thought process and justify your code choices also plays a key role in the evaluation.
Preparing for a Java interview requires more than just technical knowledge; it involves presenting yourself as a competent, problem-solving candidate. Success in Java interviews comes from practicing coding problems, mastering Java concepts, and understanding how to approach and solve problems methodically. To stand out, you should also practice good communication skills, explain your thought process clearly, and demonstrate confidence in your abilities.
Moreover, preparing for behavioral questions and having a strategy to handle challenges during the interview is essential. A systematic approach to preparation and staying calm under pressure will greatly improve your chances of success.
Problem-solving is a critical skill in any Java interview, and mastering the right techniques can significantly improve your chances of success. During the interview, you will be tasked with solving complex problems under time constraints, and how you approach these problems matters as much as finding the correct solution.
It’s important to break down the problem, choose the right approach, and communicate your thought process clearly. Practicing different problem-solving techniques will help you stay calm under pressure and solve coding challenges more effectively.
Preparing for a Java interview requires not only understanding key concepts but also having access to the right resources. Using comprehensive study materials, such as books, online tutorials, and practice platforms, will help you gain a deeper understanding of Java and refine your problem-solving skills.
These resources provide valuable insight into both theoretical concepts and hands-on coding practice, enabling you to tackle interview questions confidently. By utilizing these study tools, you can structure your preparation effectively and improve your chances of success.
Mastering advanced Java concepts is crucial for developers looking to deepen their expertise and advance in their careers. These topics not only enhance your understanding of the Java language but also provide the foundation for developing scalable, efficient, and maintainable applications.
Focusing on advanced areas like concurrency, memory management, Java’s internal architecture, and design patterns will equip you with the skills needed to tackle complex projects and succeed in technical interviews. In addition to core concepts, familiarity with frameworks and tools used in the Java ecosystem will help you build high-performance applications and handle real-world development challenges effectively.
Gaining proficiency in Java frameworks and libraries is essential for developers aiming to build scalable, efficient, and maintainable applications. Frameworks and libraries provide pre-built components that simplify common tasks like database interaction, web development, and security implementation.
By mastering popular Java frameworks such as Spring, Hibernate, and JavaFX, developers can significantly reduce development time and improve the quality of their applications. Familiarity with these tools also enhances your ability to work on enterprise-level projects and understand industry best practices.
Preparing for behavioral questions in a Java interview is crucial, as these questions assess how you handle various situations, demonstrate problem-solving abilities, and work within a team. Employers use these questions to understand your personality, communication skills, and cultural fit within the organization. Behavioral interviews focus on your past experiences, typically framed around specific challenges, achievements, or conflicts you've faced in previous roles.
In Java interviews, behavioral questions may center around how you handled coding problems, team collaborations, or dealt with project deadlines. Preparing thoughtful answers with real-life examples can make a significant impact. Employers look for candidates who can demonstrate both technical proficiency and interpersonal skills, as both are necessary for success in development roles.
This question assesses your problem-solving abilities and how you approach complex issues. Employers want to hear about specific situations where you encountered a tough coding problem and the steps you took to resolve it. In your response, highlight your thought process, the tools and methods you used, and how you were able to identify the core issue. Discuss any challenges or obstacles you faced during the problem-solving process and how you overcame them.
Mention any relevant technologies or techniques, such as debugging, optimizing code, or collaborating with others, that helped you find the best solution. Your ability to think critically and work through challenges is key here, as it showcases not only your technical skills but also your determination and perseverance when facing difficulties in coding tasks. Be sure to focus on a real-life example that illustrates your approach to solving tough problems efficiently and effectively.
Employers want to gauge your ability to stay focused and manage stress during high-pressure situations. In response, discuss a scenario where you had to deliver a project or task under tight deadlines. Explain how you managed your time and prioritized tasks to ensure that you met the deadline without compromising the quality of your work. Share the methods you use to stay organized, such as breaking tasks into smaller milestones, using project management tools, or collaborating with team members to distribute workload.
Emphasize your ability to remain calm under pressure, make quick decisions, and maintain high productivity. In addition, highlight how you communicated with stakeholders to manage expectations and ensure everyone was aligned on the project's progress. Employers look for candidates who can thrive in fast-paced environments and continue to deliver results, even when faced with challenging deadlines and unexpected obstacles. This shows that you can contribute effectively to projects in high-pressure situations.
Collaboration is a key component of any development project, and this question helps employers assess your interpersonal skills and ability to work with diverse personalities. Describe an instance where you worked with a team member who was challenging to collaborate with. Focus on how you handled the situation professionally, even when there were disagreements or communication issues. Share the steps you took to resolve conflicts, such as initiating open conversations, finding common ground, or seeking input from other team members.
Highlight how you maintained a positive attitude, kept the project on track, and remained focused on achieving the shared goal despite the interpersonal challenges. Show that you understand the importance of communication, empathy, and conflict resolution in team dynamics and how you used these skills to ensure smooth collaboration. Employers value candidates who can work well with diverse teams, manage conflicts effectively, and maintain a collaborative atmosphere even in difficult circumstances.
Conflicting requirements are common in software development, especially when multiple stakeholders are involved. Employers want to understand how you manage such situations effectively. In your response, provide an example where you encountered conflicting priorities or requirements from different stakeholders. Explain how you gathered all the necessary information, weighed the different perspectives, and worked toward a solution that met the most critical needs of the project.
Discuss how you communicated with stakeholders to clarify priorities and ensure alignment. Additionally, mention how you may have had to make compromises or offer alternatives to satisfy both parties while keeping the project on track. Show that you can navigate ambiguity, negotiate effectively, and make decisions that benefit the project as a whole. This will demonstrate your ability to manage complex situations and ensure that you deliver quality results even in the face of competing demands.
This question helps employers assess your proactive approach to improving workflows and problem-solving abilities. In your response, share a specific example where you identified inefficiencies in a process or system and took steps to enhance it. Discuss how you analyzed the existing system, identified pain points, and proposed or implemented changes to streamline operations. Be sure to highlight the positive outcomes that resulted, such as improved performance, time savings, or reduced errors.
Whether it was optimizing code, automating repetitive tasks, or improving team communication, explain the specific actions you took and the impact they had. This showcases your ability to identify opportunities for improvement and contribute to the overall success of a project or team. Employers value candidates who are not only capable of completing tasks but also take the initiative to innovate and find better, more efficient ways of doing things.
In the ever-evolving field of software development, employers want to know how you stay up to date with new technologies and programming languages. Describe a scenario where you had to learn a new language, framework, or tool quickly. Discuss the resources you utilized, such as online courses, documentation, or hands-on practice, and explain how you applied the knowledge to real-world projects. Highlight your enthusiasm for continuous learning and how you ensure that you are always expanding your skill set.
Share how you manage your learning process, whether through self-study, team collaboration, or attending conferences. Emphasize your ability to adapt to new technologies and your commitment to staying current in the industry. Employers value candidates who are lifelong learners and can quickly pick up new skills to stay ahead in a fast-paced, ever-changing environment. This shows that you can contribute to innovative projects and remain competitive in the field.
Receiving constructive criticism is an essential part of personal and professional growth. Employers want to know how you respond to feedback and whether you can use it to improve your performance. Share an experience where you received feedback, either from a colleague or manager or during a code review. Discuss how you received the feedback, acknowledged areas for improvement, and took steps to make changes. Be sure to emphasize how you remained open-minded and took the opportunity to learn from the criticism.
Highlight how you applied the feedback to your work and the positive results that followed. This demonstrates that you are receptive to feedback, committed to self-improvement, and capable of adapting to suggestions that enhance your work. Employers look for candidates who are humble, willing to learn, and continuously striving to improve themselves, both technically and personally.
Software developers often need to manage multiple tasks or projects simultaneously, so this question evaluates your time management and multitasking abilities. Discuss a time when you successfully juggled multiple projects or responsibilities. Explain how you prioritized tasks, organized your schedule, and kept track of progress to ensure that deadlines were met. Highlight any tools or techniques you use to stay organized, such as to-do lists, task management apps, or setting clear milestones.
Discuss how you stay focused and avoid burnout by breaking tasks into manageable parts and adjusting your workload when necessary. Employers want to see that you can handle multiple competing priorities and maintain productivity without sacrificing quality. Show that you can manage time effectively and stay focused on delivering results, even when dealing with several tasks simultaneously.
Unclear or ambiguous requirements are common in software development, and employers want to know how you navigate such situations. In your answer, describe a time when you had to work on a project with vague or incomplete requirements. Explain how you approached the problem by proactively seeking clarification from stakeholders, asking the right questions, and gathering additional information to define the project's scope.
Discuss how you worked with your team or clients to refine the requirements and ensure that everyone was aligned. Highlight your ability to adapt to uncertainty and find solutions even when the project details are not well-defined. Employers value candidates who can take the initiative, communicate effectively, and produce results even when faced with unclear or evolving requirements. This shows your problem-solving ability and adaptability in the face of ambiguity.
Mentoring or helping junior developers is an important part of being a senior developer or team leader. Share a time when you provided guidance or support to a less experienced developer. Discuss the challenges they were facing, how you identified areas where they needed help, and the steps you took to support their growth. Whether it was through code reviews, pair programming, or teaching them new technologies, explain how you helped them improve their skills.
Highlight the positive outcome of your mentorship, such as the junior developer's progress or the success of the project. This demonstrates your leadership abilities, willingness to collaborate, and commitment to fostering growth within your team. Employers value candidates who can guide and support their colleagues while maintaining a positive, collaborative team environment.
Researching the company before a Java interview is essential for understanding its culture, values, products, and the technical challenges it faces. It allows you to tailor your responses, align your skills with the company’s needs, and demonstrate genuine interest. Employers appreciate candidates who show initiative and have a good understanding of the company’s goals. Conducting thorough research gives you the opportunity to prepare meaningful questions, which can leave a positive impression.
Additionally, it enables you to assess if the company is the right fit for your career aspirations, providing insights into potential growth opportunities and work environment. By researching the company, you also gain insight into its industry, competitors, and technological direction, which can help you tailor your answers to show how your Java skills can contribute to its goals. Being prepared shows that you are proactive, motivated, and genuinely interested in the opportunity.
Mock interviews are a valuable tool for Java interview preparation, providing candidates with the opportunity to practice their responses to common interview questions in a simulated environment. These practice sessions help you improve your communication skills, refine your answers, and boost your confidence. By participating in mock interviews, you can identify areas where you need improvement and receive constructive feedback that can enhance your performance.
Mock interviews allow you to familiarize yourself with the interview format and experience real-time pressure, which can be beneficial during the actual interview. Engaging in mock interviews also helps you manage your nerves, perfect your body language, and practice answering technical and behavioral questions smoothly. Overall, mock interviews are an essential preparation step, offering insights into your strengths and weaknesses and enabling you to make a strong impression when facing the real interview.
Preparing thoughtful questions for your interviewer is a crucial aspect of Java interview preparation. Asking questions not only shows your interest in the company and the role but also demonstrates your proactive approach and analytical thinking. By researching the company and the position, you can formulate relevant and insightful questions that help you understand the workplace culture, expectations, and technical challenges you may face.
Moreover, asking the right questions can help you assess whether the company’s values align with yours. Interviewers often appreciate candidates who ask insightful questions, as it reflects a genuine interest in the role and a forward-thinking attitude. Preparing for the interview with a list of questions shows that you’re taking the opportunity seriously and that you're ready to contribute meaningfully to the team. Thoughtful questions can set you apart from other candidates and leave a positive impression on the interviewer.
Understanding the technical challenges the team is dealing with gives you valuable insight into the current state of the company’s projects and technical landscape. By asking this question, you can gauge the scope of the problems you'll be expected to solve and determine whether these align with your skill set.
It also demonstrates your eagerness to contribute to resolving these challenges, making you appear proactive and solution-oriented. Additionally, this question can help you assess whether the challenges are exciting and manageable, providing you with a better understanding of the job expectations.
Asking about the development tools and technologies used by the team is essential to understanding the work environment and the tools you'll need to become proficient with. Whether it’s Java frameworks, version control systems, or deployment tools, this question reveals the technological stack you’ll be working with on a day-to-day basis.
It also helps you evaluate if you're already familiar with these tools or need additional training. Moreover, understanding the tools in use can help you make informed decisions about your professional growth within the company.
Inquiring about the team’s code review and collaboration process gives you insights into the team culture and workflow. Code reviews are an essential part of a developer’s life, and knowing how the team handles them can help you assess the level of collaboration, quality control, and feedback you can expect.
Whether the focus is on code quality, knowledge sharing, or best practices, understanding this process helps you gauge the team’s focus on continuous improvement. It also helps you prepare for how your work will be evaluated and how you'll be interacting with colleagues.
Asking about professional development opportunities shows that you’re committed to growing your skills and advancing your career. This question helps you learn whether the company offers training programs, mentorship, conferences, or other resources to support your growth.
It also provides insight into how the company values continuous learning and the support provided for career progression. By asking this question, you show that you are looking for a long-term commitment to both the company and your personal development.
Understanding how success is defined and measured in the role is crucial for aligning your efforts with the company’s expectations. This question helps you clarify the performance metrics, such as the quality of code, the ability to meet deadlines, or contributing to team goals.
Knowing how success is evaluated helps you prioritize your tasks effectively and focus on what matters most to the company. It also ensures that you’re clear on the expectations, which is important for setting your own career goals.
Agile and Scrum methodologies are widely used in software development. Asking about how the team implements these methodologies helps you understand the development process and whether the team follows best practices for iterative development, sprint planning, and progress tracking.
It also gives you a glimpse of the team dynamics, how tasks are assigned, and how collaboration happens during sprints. This is especially important if you're someone who thrives in Agile environments, as it can help you determine if you will be a good fit for the team.
Company culture is an important factor to consider when evaluating a job offer. By asking about the work culture and work-life balance, you get a sense of how employees interact, the overall environment, and whether it aligns with your values.
This question shows that you care about the well-being of employees, and it can help you determine whether the company offers flexible hours, remote work options, or other benefits that promote a healthy work-life balance. A company with a supportive culture can lead to higher job satisfaction.
Asking about growth and advancement opportunities helps you understand the potential for career progression within the company. It gives you an idea of whether there are clear pathways to promotion or if the company encourages lateral movement between teams to gain experience.
It also helps you assess if the company invests in its employees’ long-term success. This question highlights your commitment to your career and your interest in growing within the organization, signaling to the interviewer that you are looking for more than just a short-term role.
Diversity and inclusion are important aspects of modern work environments. By asking about the company’s initiatives in this area, you show that you value a diverse and inclusive work culture. This question allows you to understand the company’s commitment to creating an environment where all employees feel welcome and respected.
It also helps you assess whether the company prioritizes diversity in hiring, offers training on inclusion, or has specific policies in place to ensure that all employees are supported.
Asking about the next steps in the interview process shows that you are organized and eager to move forward. This question helps you understand the timeline and what to expect, whether it’s another round of interviews, a coding test, or an offer.
It provides clarity on the process and allows you to better prepare for what comes next. It also demonstrates your interest in the role and helps you follow up effectively after the interview.
Practicing coding challenges is one of the most effective ways to prepare for Java interviews. By solving problems regularly, you not only improve your problem-solving skills but also get better at writing efficient, clean code under time constraints. Coding challenges allow you to work on a wide range of topics, from data structures to algorithms, which are frequently tested in interviews. They help you identify your strengths and weaknesses and offer a structured way to improve.
Moreover, consistent practice helps you develop a faster thought process, an essential skill when solving problems during an interview. It’s important to tackle a variety of problems to familiarize yourself with different patterns and techniques. As you practice, you’ll become more comfortable with common interview questions and gain the confidence to face coding challenges in real interviews. Here are some key tips to improve your performance in coding challenges.
The hiring process for a Java developer typically consists of multiple stages to assess both technical and soft skills thoroughly. Employers seek candidates with strong knowledge of core Java concepts, relevant frameworks, and practical coding experience. The process usually starts with resume screening, followed by a technical interview, coding challenges, and in-depth discussions on software engineering principles.
Throughout the interview, both technical prowess and cultural fit are evaluated to ensure the candidate can collaborate effectively within the development team. Success in this process often depends on a solid understanding of Java and the ability to solve complex problems in real-world scenarios.
Preparing for a Java developer interview requires a combination of technical knowledge, problem-solving skills, and a deep understanding of Java concepts. Focus on mastering core Java topics like OOP, collections, and multithreading while also gaining proficiency in advanced concepts such as JVM internals, garbage collection, and design patterns. Consistent practice with coding challenges, along with a solid understanding of frameworks like Spring and Hibernate, will give you an edge. Additionally, preparing for behavioral questions and researching the company will help you stand out as a well-rounded candidate.
Remember that success in interviews isn't just about solving problems it's also about demonstrating your ability to think critically, communicate clearly, and work efficiently under pressure. Mock interviews can help you simulate real interview scenarios and refine your approach. By practicing regularly, learning from your mistakes, and staying up to date with Java advancements, you’ll be well-equipped to tackle Java developer interviews with confidence.
Copy and paste below code to page Head section
To prepare for a Java developer interview, focus on mastering core Java concepts such as OOP, data structures, algorithms, and exception handling. Practice coding problems, study commonly asked questions, and learn about the Java ecosystem. Additionally, be ready for technical and behavioral questions and brush up on Java frameworks like Spring and Hibernate.
Key topics to focus on include core Java fundamentals, object-oriented programming (OOP), data structures and algorithms, multithreading, exception handling, and Java collections. Additionally, learn about Java 8 features, JVM internals, memory management, and frameworks like Spring Boot and Hibernate, as these are often discussed in interviews with Java developers.
Improve problem-solving skills by practicing coding challenges on platforms like LeetCode, HackerRank, and CodeSignal. Focus on solving problems related to data structures, algorithms, and system design. Understanding the time and space complexity of your solutions is essential, and revisiting concepts that you find difficult can help solidify your understanding.
Both coding challenges and theoretical knowledge are crucial. While coding challenges demonstrate your ability to implement solutions efficiently, theoretical knowledge of Java concepts, such as OOP principles, JVM, and multithreading, ensures that you understand the underlying mechanisms of the language. Balance both to perform well in interviews.
Common questions include topics such as explaining OOP concepts, differences between ArrayList and LinkedList, handling exceptions in Java, and explaining Java’s memory model. Additionally, expect questions about Java frameworks like Spring Boot and Hibernate, and understanding design patterns, threading, and multithreading challenges.
Understanding Java frameworks, such as Spring, Hibernate, and Java EE, is important as they are widely used in Java development. Interviewers expect candidates to be familiar with these frameworks and their core concepts, such as dependency injection in Spring or ORM in Hibernate, as they are crucial for building modern Java applications.