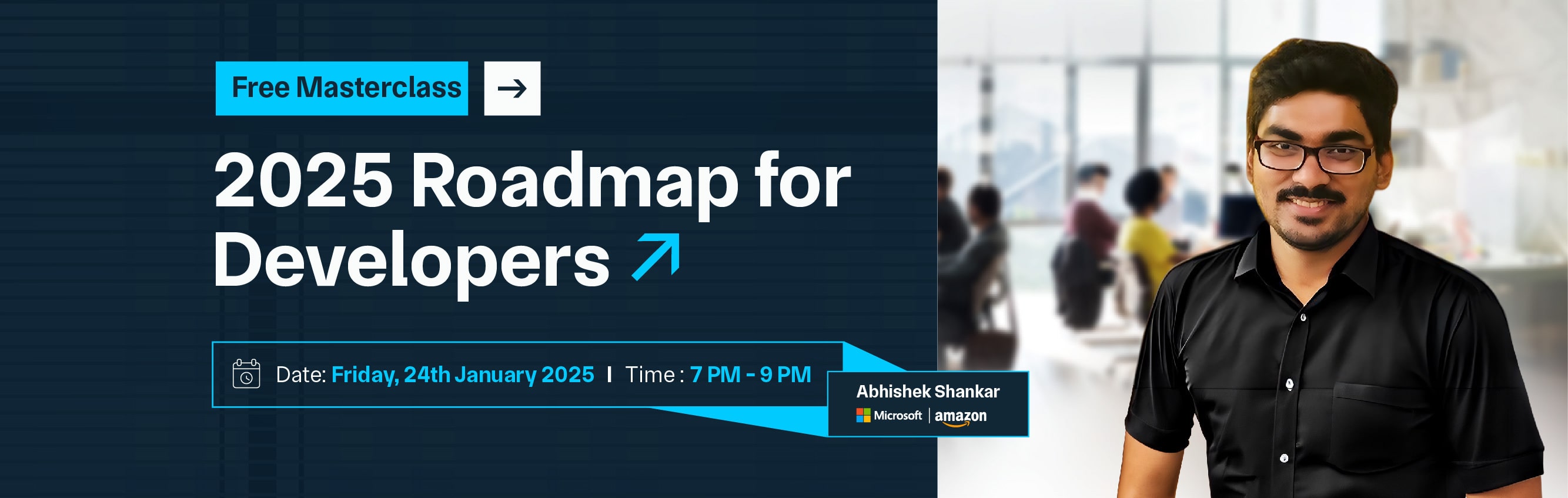

In Java, interfaces are used to define a contract for what a class should do without specifying how it should do it. This abstraction allows developers to design flexible and reusable code. Interfaces enable multiple classes to implement the same set of methods, ensuring that they all follow the same structure, yet each class can implement the methods in its way. This feature promotes loose coupling, meaning that classes can interact with each other without being tightly bound to one another’s implementations.
Additionally, interfaces allow Java to support multiple inheritance, something that is not possible with classes. A class can implement multiple interfaces, thereby inheriting behaviors from various sources. Interfaces are key to achieving polymorphism, where objects of different classes that implement the same interface can be treated interchangeably.
They are widely used in defining APIs, event-driven programming, and in design patterns like Strategy and Observer. Moreover, interfaces enhance testability by allowing developers to create mock implementations for unit testing. With Java 8, interfaces also support default methods, enabling methods with a body, further increasing their flexibility. Overall, interfaces are essential for writing clean, maintainable, and scalable code in Java.
An interface in Java is a reference type, similar to a class, that is used to define a contract for what a class can do without specifying how it does it. It can contain abstract methods (methods without a body) and constants (static final variables). Any class that implements an interface must provide concrete implementations for all of the abstract methods defined in that interface.
In Java, an interface is declared using the interface keyword. Unlike a class, an interface cannot have instance variables or constructors, and all the methods declared in an interface are implicitly abstract (unless they are default or static methods). Interfaces allow Java to achieve abstraction, ensuring that only the method signatures are exposed while the actual logic remains hidden in the implementing classes.
An important feature of interfaces is that a class can implement multiple interfaces, allowing for multiple inheritance of behavior, which Java does not support with classes. This is one of the key reasons interfaces are widely used in Java for building flexible and modular applications. Additionally, interfaces are used to define APIs and support polymorphism, as objects of different classes implementing the same interface can be treated uniformly.
interface {
// declare constant fields
// declare methods that abstract
// by default.
}
Interfaces in Java are used for several important reasons that enhance the flexibility, scalability, and maintainability of code. Here are some of the key reasons why interfaces are widely used in Java:
In Java, interfaces allow you to define abstract methods—methods without implementations—that must be implemented by any class that chooses to implement the interface.
This provides a way to establish a contract that specifies what methods a class must have without dictating how they should work. By doing so, interfaces support abstraction, enabling you to focus on what actions an object can perform rather than how these actions are implemented.
Java does not support multiple inheritance for classes (i.e., a class cannot inherit from more than one class), but it allows a class to implement multiple interfaces.
This provides a way for a class to inherit behavior from multiple sources. Through interfaces, a class can implement several different functionalities from different interfaces, promoting code reuse and flexibility.
Interfaces help in reducing the dependency between classes by providing a level of loose coupling. When a class interacts with another class, it can do so through an interface rather than directly accessing a specific class.
This means that changes made to the implementing class will not directly affect other parts of the code that rely on the interface, promoting easier maintenance and flexibility in the system.
Interfaces enable polymorphism, a core principle of object-oriented programming. Polymorphism allows objects of different classes to be treated as objects of a common interface type.
For example, you can have different classes that implement the same interface, and you can call the methods of these classes without knowing the specific type of the object as long as it implements the interface. This makes the code more flexible and extensible.
Interfaces can be used to define a callback mechanism, where one object calls methods in another object via the interface. This is common in event-driven programming, where a component (e.g., a user interface) needs to notify other components (e.g., event listeners) when certain actions occur, like button clicks or user input. The implementing class can define how the callback methods work, but the interface guarantees that these methods will exist.
Interfaces provide a way to define common behavior across different, unrelated classes. For example, an interface like Serializable allows different classes to be serialized without having to share a common parent class. Any class that implements this interface can be serialized, providing consistent behavior across various class types, even if they don’t share a common superclass.
When writing unit tests, using interfaces makes it easier to mock dependencies. For example, if a class depends on a specific interface, you can create a mock implementation of that interface for testing purposes. This allows you to isolate the class being tested and simulate interactions with its dependencies, making tests more focused and reliable.
By using interfaces, you can separate concerns in your application. For instance, the interface can define the contract or high-level behavior, while the implementation handles the specific details. This separation allows you to develop, test, and maintain parts of your system independently, which is a key principle in designing scalable and maintainable applications.
Interfaces help make your code more flexible for future changes. When you define behavior through interfaces, you can change or extend the implementation details without affecting the rest of the system that relies on the interface. This reduces the need to refactor code extensively when new functionality is added or when existing functionality is modified.
One of the key advantages of interfaces is that they allow different classes to provide their implementations of the same set of methods. This is useful when you have multiple ways of achieving the same goal. For instance, if you have a payment system, you might define an interface PaymentMethod with methods like processPayment(). Different classes like CreditCardPayment or PayPalPayment can then provide their specific implementations of this method, offering flexibility and extensibility.
Interfaces in Java promote code modularity, maintainability, and flexibility. They allow classes to define behavior without dictating implementation, enable multiple inheritance of behavior, and facilitate loose coupling and polymorphism. Through interfaces, Java offers a way to structure code that is both extensible and easy to maintain, making it a fundamental tool for developing large-scale applications.
In Java, an interface is defined using the interface keyword. Below is the basic syntax for declaring and implementing an interface:
interface InterfaceName {
// Abstract methods (by default, all methods are abstract)
returnType methodName1(parameters);
returnType methodName2(parameters);
}
interface Animal {
void sound(); // Abstract method
void eat(); // Abstract method
}
A class can implement an interface using the implements keyword. The class must provide concrete implementations for all the abstract methods in the interface.
class Dog implements Animal {
@Override
public void sound() {
System.out.println("Bark");
}
@Override
public void eat() {
System.out.println("Eating dog food");
}
}
Java 8 introduced default methods in interfaces. A default method provides a method body in the interface itself.
interface Animal {
void sound(); // Abstract method
// Default method
default void sleep() {
System.out.println("Animal is sleeping");
}
}
In this case, a class implementing the Animal interface is not required to implement the sleep() method, as it has a default implementation.
Interfaces can also have static methods. Static methods in interfaces must have a body, and they can be called without creating an instance of the interface.
interface Animal {
static void description() {
System.out.println("Animals are living beings.");
}
}
Here’s a simple example of how to use an interface in Java:
Let's create an interface called Animal that defines two methods: sound() and eat().
// Interface definition
interface Animal {
// Abstract methods (no body)
void sound();
void eat();
// Default method (Java 8 and later)
default void sleep() {
System.out.println("The animal is sleeping.");
}
}
Now, let's create a class Dog that implements the Animal interface and provides concrete implementations for the sound() and eat() methods.
// Class implementing the Animal interface
class Dog implements Animal {
// Implementing sound() method
@Override
public void sound() {
System.out.println("Bark");
}
// Implementing eat() method
@Override
public void eat() {
System.out.println("The dog is eating.");
}
// Optionally, you can override the default method 'sleep()' if needed
@Override
public void sleep() {
System.out.println("The dog is sleeping in its bed.");
}
}
Finally, we’ll write a Main class to create an instance of Dog and invoke its methods.
1. Interface Definition (Animal):
2. Implementation (Dog):
3. Using the Interface:
Bark
The dog is eating.
The dog is sleeping in its bed.
In Java, interfaces can be nested within other classes or even within other interfaces. A nested interface is simply an interface defined within the scope of a class or another interface. Nested interfaces behave like top-level interfaces, but they are logically grouped with the class or interface they are nested in, making the design cleaner and more encapsulated
.
There are two types of nested interfaces in Java:
Let’s look at examples for both types.
A static nested interface is an interface that is declared static inside a class. Static nested interfaces can be accessed without an instance of the enclosing class.
// Outer class with a static nested interface
class OuterClass {
// Static nested interface
public static interface NestedInterface {
void display();
}
}
// Class implementing the nested interface
class InnerClass implements OuterClass.NestedInterface {
@Override
public void display() {
System.out.println("Hello from the nested interface!");
}
}
public class Main {
public static void main(String[] args) {
// Creating an instance of InnerClass which implements the nested interface
InnerClass obj = new InnerClass();
obj.display(); // Output: Hello from the nested interface!
}
}
A non-static nested interface is defined without the static keyword and requires an instance of the outer class to be used.
// Outer class with a non-static nested interface
class OuterClass {
// Non-static nested interface (Inner interface)
public interface NestedInterface {
void display();
}
}
// Class implementing the non-static nested interface
class InnerClass implements OuterClass.NestedInterface {
@Override
public void display() {
System.out.println("Hello from the non-static nested interface!");
}
}
public class Main {
public static void main(String[] args) {
// Creating an instance of OuterClass
OuterClass outer = new OuterClass();
// Creating an instance of InnerClass, which implements the nested interface
InnerClass obj = new InnerClass();
obj.display(); // Output: Hello from the non-static nested interface!
}
}
Explanation:
In Java, interfaces and classes are two fundamental concepts, but they serve different purposes. A class defines the structure and behavior of objects, whereas an interface defines a contract that classes must follow, specifying what methods a class should implement without dictating how. Below is a comparison table highlighting the key differences between interface and class.
These features make interfaces a powerful tool in Java for defining abstract behaviors and achieving flexible, reusable, and modular code.
Interfaces in Java are widely used in real-world applications due to their ability to define contracts and enable polymorphism. Here are some practical use cases where interfaces are commonly employed:
interface Animal {
void makeSound();
}
class Dog implements Animal {
public void makeSound() {
System.out.println("Bark");
}
}
class Cat implements Animal {
public void makeSound() {
System.out.println("Meow");
}
}
public class Main {
public static void main(String[] args) {
Animal dog = new Dog();
dog.makeSound(); // Output: Bark
Animal cat = new Cat();
cat.makeSound(); // Output: Meow
}
}
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import javax.swing.JButton;
import javax.swing.JFrame;
public class ButtonExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Button Example");
JButton button = new JButton("Click Me");
// Implementing the ActionListener interface
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked!");
}
});
frame.add(button);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Interfaces in Java offer several key advantages that enhance the flexibility, maintainability, and extensibility of code. Here are some of the major benefits of using interfaces:
Interfaces provide a way to achieve abstraction in Java. By defining abstract methods in an interface, you specify the required behavior of a class without dictating how it should be implemented. This allows developers to focus on the overall design and structure of the system rather than getting bogged down with specific implementation details.
Abstraction also enhances code readability and maintainability because developers can work with the interface to understand the behavior without delving into the underlying logic. This separation between interface and implementation helps to keep the code clean and organized, improving its scalability and flexibility over time.
Unlike classes, Java does not support multiple inheritance, where a class can inherit from more than one class. However, interfaces provide a way to bypass this limitation. A class in Java can implement multiple interfaces, allowing it to inherit behaviors from more than one source.
This feature is useful when a class needs to adopt multiple functionalities without being constrained by the rigid structure of a single inheritance. For instance, a class can implement both Serializable and Cloneable interfaces to gain the respective behaviors, making the class more versatile and adaptable.
Loose coupling refers to the concept of reducing the dependencies between components in a system. Interfaces promote this by allowing classes to interact with each other through a common interface rather than relying on direct class dependencies. This means that the implementation of one class can be changed without affecting others as long as the interface remains the same.
For example, a PaymentProcessor class can work with any class that implements the PaymentService interface, regardless of the actual payment method (e.g., PayPal, CreditCard, etc.). This decoupling enhances maintainability, scalability, and flexibility, as changes to one part of the system do not require widespread changes across other components.
Polymorphism is a key concept in object-oriented programming that allows objects of different types to be treated as instances of a common type. Interfaces play a crucial role in enabling polymorphism in Java. Since a class can implement multiple interfaces, you can interact with objects of different classes that implement the same interface uniformly.
For example, objects of the Cat and Dog classes, both implementing an Animal interface, can be treated as Animal objects and passed to a method that expects an Animal type, regardless of the concrete class type. This promotes flexibility in the code and allows for dynamic behavior, as the specific implementation is resolved at runtime.
Loose binding, or dynamic binding, refers to the ability to decide at runtime which method or implementation to call. Interfaces are instrumental in enabling this behavior, as they allow you to define method signatures without specifying the implementation details. The actual method execution is resolved when an object of a concrete class is created, and the method is invoked.
For example, when a class implements an interface, the specific method to be invoked depends on the object instance, not the class type. This feature helps in creating flexible systems where the behavior of an object can be determined dynamically, allowing for more extensible and adaptable code.
Interfaces play a vital role in making code easier to maintain and extend. Since interfaces separate the definition of behavior from its implementation, developers can make changes to the implementation of a class without affecting other parts of the system. If an interface remains unchanged, all implementing classes continue to work as expected, even if the implementation details evolve.
This separation helps to reduce the risk of introducing bugs during code changes and makes it easier to add new features. Additionally, because the functionality is defined in interfaces, developers can easily identify and modify specific behaviors in the code without affecting unrelated areas of the application.
In Java, interfaces are a powerful tool for achieving abstraction, polymorphism, and loose coupling. However, they come with certain limitations that developers must be aware of to use them effectively.
In Java, interfaces are not allowed to have instance variables, which are fields that store state specific to an object instance. Instead, interfaces can only have constants, which are implicitly public, static, and final. This limitation means that interfaces cannot manage object-level states directly. While classes can maintain instance variables to store and manipulate data, interfaces are meant to define a contract for behavior rather than hold a specific state.
As a result, any shared state management must be handled within the implementing classes, which can sometimes lead to more complex designs when consistent state management across multiple implementations is needed.
Interfaces cannot have constructors because they cannot be instantiated directly. The primary role of an interface is to define a set of abstract methods that concrete classes must implement. Since an interface doesn’t create instances of objects, there is no need for constructors, which are used to initialize new objects.
This can be a limitation when there’s a need to encapsulate specific initialization logic for the interface itself. For initialization, developers must rely on constructors in the implementing classes or use other design patterns like Factory methods or Dependency Injection to handle object creation and setup.
Traditional interfaces in Java are used solely to declare method signatures and do not provide any implementation. This means that any class that implements the interface must provide its implementation for those methods. Although Java 8 introduced default and static methods, allowing interfaces to provide method implementations, they have a limited scope.
Default methods allow common behavior to be shared across implementations, but they are not a complete replacement for a full implementation that a class would provide. This restriction can lead to situations where code duplication occurs, as each implementing class may need to repeat certain logic that could be centrally managed in a class.
All methods in an interface are implicitly public. This means that you cannot declare methods as private, protected, or package-private within an interface. The rationale behind this is that interfaces are meant to define public contracts that any implementing class can access. However, this can limit flexibility, especially when you want to restrict access to certain methods.
For example, if you want a method to be accessible only within a certain package or to certain classes, you cannot enforce that restriction in the interface itself. Instead, such restrictions need to be enforced in the implementing class, which may lead to inconsistencies and a more complex design.
While classes can inherit both method signatures and implementations from parent classes, interfaces can only define method signatures and cannot provide full method implementations (except through default methods). This means interfaces cannot reuse code in the same way classes can through inheritance.
In some cases, multiple interfaces can be implemented by a class, which allows the class to inherit behaviors from multiple sources. However, this does not eliminate the need for each class to implement the behavior defined by the interface. Developers often have to implement the common functionality in each class, which could lead to code duplication or the need for additional design patterns.
Java 8 introduced default methods, which allow interfaces to provide a default implementation for methods. While this feature is useful, it can also create conflicts if a class implements multiple interfaces that provide different default implementations for the same method.
In such cases, the class is forced to explicitly resolve the conflict by overriding the method. This can make the design more complicated and require additional logic in the class to handle such conflicts. It also introduces a level of uncertainty, as developers may not be immediately aware of which default implementation will be used unless the conflict is resolved explicitly.
While interfaces in Java offer significant advantages, such as promoting abstraction, flexibility, and loose coupling, they also have limitations that developers must consider. These limitations, including the inability to store instance variables, lack of constructor support, and potential conflicts with default methods, can introduce complexity into the design.
Despite these challenges, interfaces remain a crucial feature of object-oriented programming in Java. By understanding these limitations, developers can make informed decisions and utilize interfaces effectively, ensuring that their code remains clean, maintainable, and adaptable to future changes.
Copy and paste below code to page Head section
An interface in Java is a reference type, similar to a class, that can contain only constants, method signatures, default methods, static methods, and nested types. It defines a contract that classes must follow by implementing the abstract methods declared in the interface. Interfaces enable abstraction and allow classes to implement multiple behaviors.
The key difference is that an abstract class can have both abstract (without implementation) and concrete methods (with implementation). In contrast, an interface can only have abstract methods (except for default and static methods introduced in Java 8). Additionally, a class can implement multiple interfaces but can inherit from only one abstract class, making interfaces more flexible for multiple inheritance.
No, interfaces cannot have instance variables. They can only contain constants, which are implicitly public, static, and final. Instance variables are meant to hold object-specific data, which is not the purpose of interfaces.
No, interfaces cannot have constructors because they cannot be instantiated directly. Interfaces serve only as a blueprint for the classes that implement them.
Default methods, introduced in Java 8, allow interfaces to provide method implementations. These methods have a body, and classes implementing the interface can use or override them. Default methods enable interfaces to evolve without breaking existing implementations.
Yes, a class in Java can implement multiple interfaces. This allows a class to inherit behaviors from several interfaces, overcoming the limitation of Java's single inheritance model.