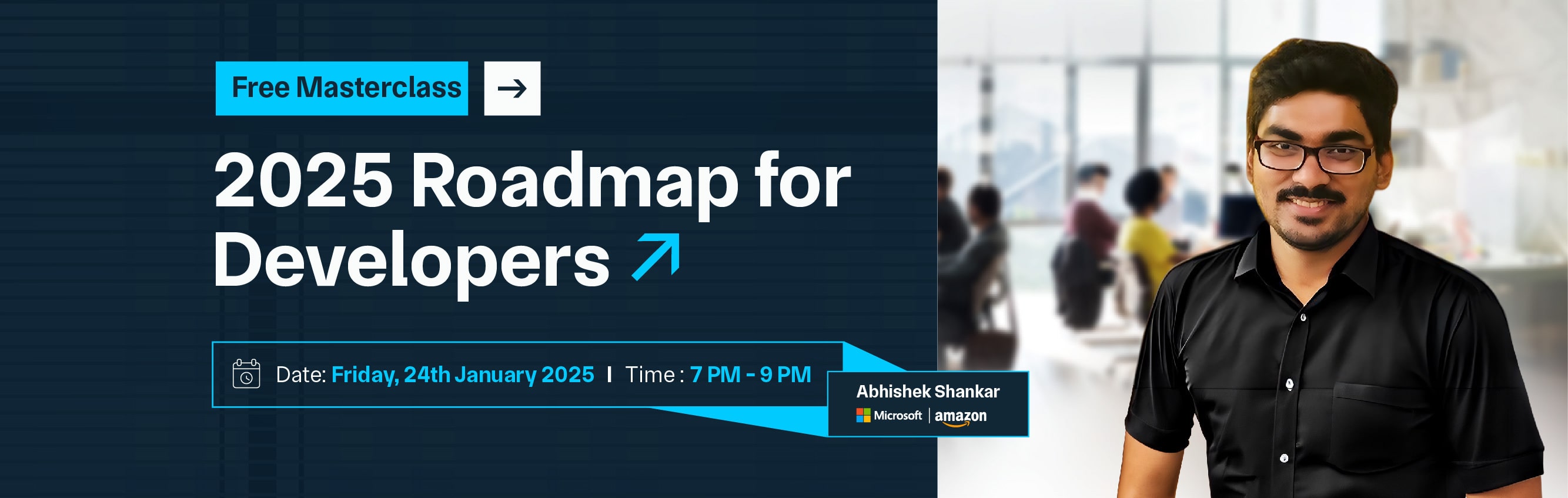

Installing Django on Ubuntu is a straightforward process that can be completed in a few simple steps. First, ensure that your Ubuntu system is up to date by running sudo apt update && sudo apt upgrade in the terminal. Then, install Python3 and pip (Python’s package manager). If they aren’t already installed with the command sudo apt, install python3 python3-pip. Next, it’s recommended to set up a virtual environment to isolate your Django project from system dependencies.
You can install the virtual environment package with sudo apt install python3-venv, create a virtual environment using python3 -m venv myenv, and activate it with source myenv/bin/activate. Once the virtual environment is active, install Django by running pip install django. After installation, verify it by typing django-admin --version, which will show the installed version of Django.
To create a new Django project, run django-admin startproject projectname, then navigate into the project directory with cd projectname. Finally, start the development server by executing python3 manage.py runserver and visit http://127.0.0.1:8000/ in your web browser to see the Django welcome page. By following these steps, you'll have Django up and running on Ubuntu and can start building web applications.
Updating system packages on Ubuntu is an essential step to ensure that your system has the latest software versions and security patches. To update the packages, follow these steps:
1. Open the Terminal: Press Ctrl + Alt + T to open the terminal on your Ubuntu system.
Update the Package List: Run the following command to update the list of available packages and their versions:
sudo apt update
2. This command fetches the most recent package information from the repositories, ensuring that you are installing the latest versions.
Upgrade the Installed Packages: To upgrade the outdated packages to their latest versions, use the following command:
sudo apt upgrade
3. You will be prompted to confirm the upgrade. Press Y to proceed.
Clean Up Unused Packages: After upgrading, you can remove unnecessary packages and dependencies with:
sudo apt autoremove
4. This helps free up disk space by removing packages that are no longer needed.
Optional - Full Upgrade: To upgrade all packages, including those that may require removing older versions or installing new dependencies, you can run:
sudo apt full-upgrade
Updating system packages regularly ensures your Ubuntu system remains secure and runs smoothly.
To install Python and pip on Ubuntu, follow these steps:
Most recent versions of Ubuntu come with Python pre-installed. However, to make sure Python is installed or to install a specific version, use the following steps:
Check if Python is already installed by running:
python3 --version
If Python is not installed or if you want to install a specific version, run:
sudo apt update
sudo apt install python3
This installs the latest version of Python 3 available in the official Ubuntu repositories.
pip is the package manager for Python, and it's used to install Python packages. To install pip for Python 3, run the following command:
Install pip by running:
sudo apt install python3-pip
After installation, verify that the pip was successfully installed:
pip3 --version
This will display the installed version of pip.
To ensure you have the latest version of pip, you can upgrade it by running:
pip3 install --upgrade pip
Now, you have Python and pip installed and ready to use on your Ubuntu system. You can use pip to install Python libraries and packages.
To install and use a virtual environment on Ubuntu, follow these steps. Using a virtual environment is highly recommended as it helps isolate your project’s dependencies, preventing conflicts with system-wide packages.
The first step is to install the python3-venv package, which provides the necessary tools to create virtual environments.
Open a terminal and run:
sudo apt update
sudo apt install python3-venv
Once the python3-venv package is installed, you can create a virtual environment in your project directory.
Navigate to your project directory (or create one) where you want the virtual environment:
mkdir myproject
cd myproject
Create the virtual environment using the python3 -m venv command. You can name your virtual environment folder anything you like.
For example:
python3 -m venv myenv
This command creates a new directory called myenv (or your chosen name) that contains a fresh Python environment isolated from the system environment.
To start using the virtual environment, activate it with the following command:
source myenv/bin/activate
After activation, your terminal prompt will change to show the name of the virtual environment, indicating that it is active.
Example:
(myenv) user@ubuntu:~/myproject$
While the virtual environment is activated, you can install Python packages using pip and they will only be available within that environment.
For example, to install Django:
pip install django
When you're done working in the virtual environment, you can deactivate it by running:
deactivate
Your terminal prompt will return to the system’s default Python environment.
To install Django on Ubuntu, follow these steps:
If you're using a virtual environment (which is recommended to avoid conflicts with system-wide packages), make sure it’s activated first. If you haven’t set up a virtual environment yet, follow the steps in the previous section. To activate the virtual environment, run:
source myenv/bin/activate
This will activate the virtual environment, and you’ll see the environment name in the terminal prompt, such as (myenv).
With your virtual environment activated (or directly on the system if not using one), install Django by running the following command:
pip install django
This command will download and install the latest version of Django from the Python Package Index (PyPI).
After the installation completes, verify that Django was successfully installed by checking its version.
Run:
django-admin --version
This will display the installed version of Django. If Django is installed correctly, you’ll see an output like 4.1.0 (depending on the version you installed).
To create a new Django project, run the following command:
django-admin startproject myproject
Replace myproject with the desired name of your project. This will create a new directory with the project structure.
Navigate into your project directory:
cd myproject
Start the development server:
python3 manage.py runserver
This will start Django’s built-in development server. You can now visit http://127.0.0.1:8000/ in your web browser, where you should see the Django welcome page.
When you’re done, you can deactivate the virtual environment by running:
deactivate
Now you have Django installed, and your first Django project up and running on Ubuntu!
Creating a Django project is a simple process. Below are the detailed steps to create your first Django project after installing Django on Ubuntu.
Before you create a Django project, navigate to the directory where you want to store your project files. You can create a new directory or use an existing one.
For example, to create a new directory and navigate to it:
mkdir myproject
cd myproject
Once you're in your desired directory, use the django-admin command to create a new project.
Run the following command:
django-admin startproject myproject
Replace myproject with your preferred project name. This command creates a new directory with the project structure, including files like manage.py and the folder for your project settings.
The structure will look like this:
myproject/
manage.py
myproject/
__init__.py
settings.py
urls.py
wsgi.py
After creating the project, navigate to the project directory:
cd myproject
Now, you're inside the Django project, where you'll run management commands.
Once the project is created, you can start the Django development server to verify everything is set up correctly.
Run the following command:
python3 manage.py runserver
This will start the Django development server at http://127.0.0.1:8000/. Open your web browser and go to this address. You should see the Django welcome page indicating that the project was created successfully.
A Django project can have multiple "apps." An app is a module or component that encapsulates specific functionality within the project. You can create a Django app inside your project like this:
python3 manage.py startapp myapp
This creates a new directory called myapp/ containing the basic structure for a Django app.
If you are using a virtual environment, deactivate it when you're done:
deactivate
Running the development server in Django is a simple process. The Django development server allows you to preview your web application locally on your computer before deploying it to a production environment. Here's how to run the server:
First, ensure that you're in the directory where your Django project is located (the same directory as the manage.py file). If you are not already there, navigate to it using the cd command:
cd /path/to/your/project/myproject
Replace /path/to/your/project/myproject with the actual path to your project directory.
Once you're inside the project directory, you can start the development server by running the following command:
python3 manage.py runserver
This will start the development server on the default address http://127.0.0.1:8000/. You will see an output in the terminal similar to this:
Performing system checks...
System check identified no issues (0 silenced).
December 20, 2024 - 12:00:00
Django version 4.x.x, using settings 'myproject.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CONTROL-C.
After the server starts, open your web browser and go to the following URL:
http://127.0.0.1:8000/
You should see the Django welcome page, which confirms that the development server is running properly. The page will look something like this:
The install worked successfully! Congratulations!
By default, Django runs on 127.0.0.1:8000. If you want to run the server on a different port or make it accessible on your local network, you can specify a different port or IP address:
To run the server on a different port (e.g., port 8080):
python3 manage.py runserver 8080
To make the server accessible on your local network (useful for testing on mobile devices or other computers), run:
python3 manage.py runserver 0.0.0.0:8000
This will allow access to the server from any device on the same network using your machine's local IP address.
To stop the server, go back to your terminal and press Ctrl + C. This will halt the server, and you will return to the command prompt.
Accessing the Django Admin interface is an important step in managing and interacting with the data in your Django application. The Django admin is a built-in feature that allows you to easily manage models, users, and other aspects of your site. Here's how you can access it:
Before you can access the Django Admin interface, you need to create a superuser (admin user) with administrative privileges. The superuser will allow you to log in and manage the admin interface. To create a superuser, follow these steps:
Step 1: Make sure your development server is running:
python3 manage.py runserver
Step 2: Open a new terminal window (or keep the current one) and run the following command to create a superuser:
python3 manage.py createsuperuser
Step 3: You'll be prompted to provide a username, email address, and password for the superuser. Ensure that the password is strong enough, as Django will require it to meet certain security criteria (e.g., a mix of letters, numbers, and special characters).
Example prompts:
Username (leave blank to use 'myusername'): admin
Email address: admin@example.com
Password: ********
Password (again): ********
After successfully creating the superuser, you’ll see a message confirming the creation.
Once the superuser is created, you can access the Django admin interface through your web browser.
Step 1: Make sure the Django development server is running:
python3 manage.py runserver
Step 2: Open your web browser and go to the following URL:
http://127.0.0.1:8000/admin/
Step 3: This URL will take you to the login page for the Django admin interface.
Step 4: Enter the superuser credentials (username and password) that you created earlier.
Step 5: After logging in, you will be directed to the Django Admin dashboard, where you can:
You can customize the Django Admin interface to fit your project’s needs. For example, you can register your models so that they appear in the admin panel:
Register your models:
from django.contrib import admin
from .models import MyModel
admin.site.register(MyModel)
This will display MyModel in the admin interface, allowing you to manage it from there.
Additional configuration in Django allows you to customize your project further to suit your needs. Below are some optional configurations that can help you set up your Django project more effectively, such as database settings, static file management, and custom error pages.
By default, Django uses SQLite as the database for development. If you'd like to use a different database (such as PostgreSQL, MySQL, or others), you can configure it in the settings.py file.
1. Install PostgreSQL and psycopg2: First, install PostgreSQL and the psycopg2 package (which is a PostgreSQL adapter for Python) using the following commands:
sudo apt install postgresql postgresql-contrib
pip install psycopg2
2. Configure Database in settings.py: In your settings.py file, find the DATABASES setting and configure it for PostgreSQL:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': 'mydatabase',
'USER': 'myuser',
'PASSWORD': 'mypassword',
'HOST': 'localhost',
'PORT': '5432',
}
}
Replace 'mydatabase', 'myuser', and 'mypassword' with your PostgreSQL database credentials.
3. Migrate Database: After updating the database configuration, run the migration commands to set up the tables in your database:
python3 manage.py migrate
In production, you’ll need to configure static files (CSS, JavaScript, images) to be served separately from your Django app. Here’s how you can set up static files in the development environment:
Configure Static File Settings: Open the settings.py file and locate the STATIC_URL and STATICFILES_DIRS settings:
STATIC_URL = '/static/'
# Add additional directories where your static files are located
STATICFILES_DIRS = [
BASE_DIR / "static",
]
Collect Static Files: Run the following command to collect all static files into one directory:
python3 manage.py collectstatic
This is typically required in production to ensure all static files are served efficiently.
Django uses the urls.py file to route requests to the appropriate views. You can add custom URL patterns or redirect URLs to control how users access different parts of your site.
Define URLs in urls.py: Open your myproject/urls.py and add custom paths. For example, if you have an app called blog, you can include its URLs like this:
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('blog/', include('blog.urls')),
]
Create URL Patterns in the App: In your app’s urls.py file (e.g., blog/urls.py), define URL patterns specific to the app:
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
]
Custom error pages can improve the user experience. Django comes with default error pages, but you can create your own for common HTTP errors (404 Not Found, 500 Internal Server Error, etc.).
1. Create Custom Error Templates: Create custom HTML templates for error pages inside your app’s templates/ directory:
For example, in the templates folder:
myproject/
templates/
404.html
500.html
2. Configure DEBUG Setting: In your settings.py, set DEBUG to False for production:
DEBUG = False
This will tell Django to use the custom error templates when an error occurs.
In a production environment, Django requires you to specify which host/domain names the site can serve. This is a security measure to prevent HTTP Host header attacks.
Set ALLOWED_HOSTS in settings.py: Add your domain name or IP address to the ALLOWED_HOSTS list:
ALLOWED_HOSTS = ['yourdomain.com', 'www.yourdomain.com', '127.0.0.1']
This ensures that your site only responds to requests from these hosts.
Django has built-in support for sending emails. To configure email functionality, you can set up email settings in settings.py.
Configure Email Backend: For development, you can use Django's console backend to print emails to the console:
EMAIL_BACKEND = 'django.core.mail.backends.console.EmailBackend'
For production, configure it to use an SMTP server (e.g., Gmail, SendGrid, etc.):
EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend'
EMAIL_HOST = 'smtp.gmail.com'
EMAIL_PORT = 587
EMAIL_USE_TLS = True
EMAIL_HOST_USER = 'youremail@gmail.com'
EMAIL_HOST_PASSWORD = 'yourpassword'
Make sure to use appropriate values for your email provider.
Django provides several security settings that are important for production deployments.
1. Enable HTTPS (SSL/TLS): In production, use HTTPS to encrypt communication.
Set SECURE_SSL_REDIRECT to True:
SECURE_SSL_REDIRECT = True
2. Set a Secret Key: Django uses the SECRET_KEY for cryptographic signing. Ensure it’s unique and kept secure. Do not share it publicly.
SECRET_KEY = 'your_secret_key_here'
3. Set CSRF_COOKIE_SECURE and SESSION_COOKIE_SECURE: These settings help protect against Cross-Site Request Forgery (CSRF) and ensure session cookies are only sent over HTTPS:
CSRF_COOKIE_SECURE = True
SESSION_COOKIE_SECURE = True
Django allows you to configure logging to track errors, warnings, and other events. You can configure logging in settings.py like this:
LOGGING = {
'version': 1,
'disable_existing_loggers': False,
'handlers': {
'file': {
'level': 'ERROR',
'class': 'logging.FileHandler',
'filename': 'errors.log',
},
},
'loggers': {
'django': {
'handlers': ['file'],
'level': 'ERROR',
'propagate': True,
},
},
}
Installing Django on Ubuntu is a straightforward process that involves preparing your system, installing the necessary dependencies, setting up a virtual environment, and finally installing Django itself. With these steps completed, you'll be ready to start building powerful web applications using Django.
Using a virtual environment ensures that your project dependencies are isolated and manageable, while Django provides a robust framework for developing scalable and maintainable applications. Whether you're working on a small personal project or a large-scale production application, Django offers all the tools you need to create dynamic websites efficiently.
Copy and paste below code to page Head section
Django is a high-level Python web framework that allows you to build secure and maintainable web applications quickly. It emphasizes rapid development, clean design, and the "don't repeat yourself" (DRY) principle.
A virtual environment helps isolate your project dependencies from the system’s global Python environment. This ensures that your project uses the required versions of packages without conflicts with other projects or the system Python.
Yes, Django supports several databases like PostgreSQL, MySQL, and Oracle. You can configure the database of your choice by modifying the DATABASES setting in your settings.py file.
Yes, Django can be deployed on a production server using web servers like Nginx or Apache, with WSGI servers such as Gunicorn. You'll also need to configure a database, static files, and security settings for a production environment.
To uninstall Django, you can use the following command if you installed it via pip: pip uninstall django. If you use a virtual environment, make sure to deactivate it before uninstalling.
If you face permission errors while installing Django or other dependencies, try using sudo for system-wide installations: sudo pip install django.