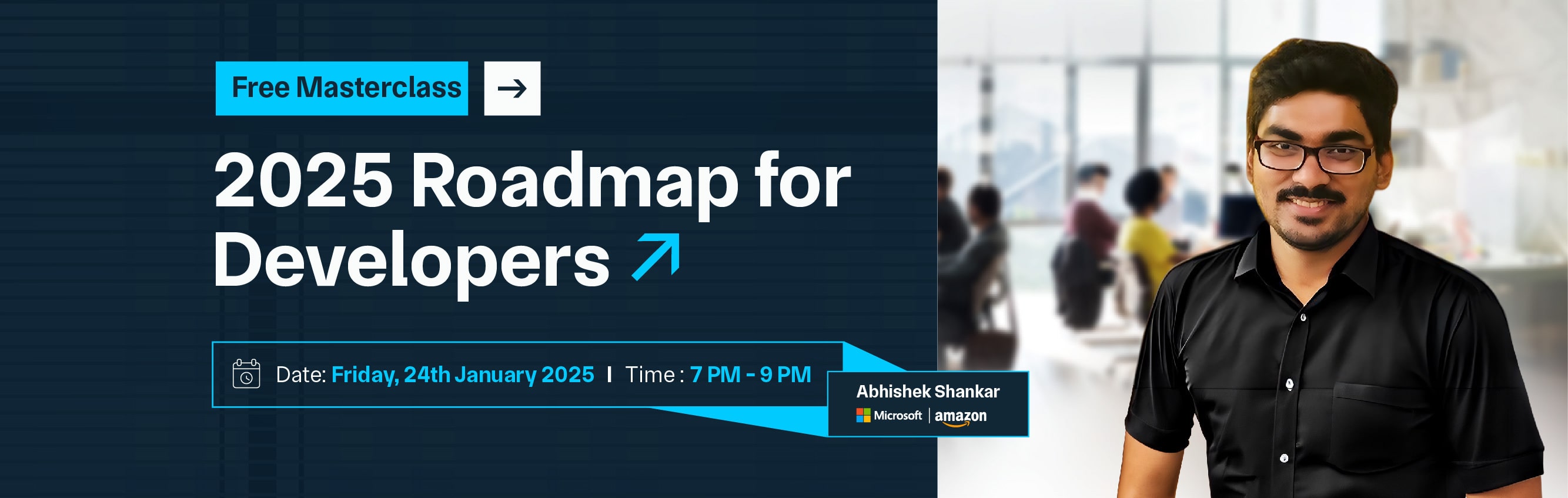

To run a Python program, ensure Python is installed on your computer by downloading it from python.org and following the installation instructions. Once installed, you can create a Python script using a text editor or an Integrated Development Environment (IDE) like PyCharm or VS Code. Save your script with a .py extension, such as my_script.py.
To execute the script, open a command prompt (Windows) or terminal (Mac/Linux) and navigate to the directory where your script is saved using the cd (change directory) commands. Then, type python my_script.py and press Enter. This command instructs the Python interpreter to execute your script. During execution, any output generated by your script, such as print statements or results of computations, will be displayed in the command prompt or terminal window.
If errors occur, Python will provide error messages that can help you debug your code. If you're using an IDE, running your script is often as simple as clicking a "Run" button or using a keyboard shortcut within the IDE itself. Python's versatility allows scripts to be executed on various operating systems, making it accessible for a wide range of applications, from simple scripts to complex software development and data analysis tasks.
A Python script is a file containing Python code, typically saved with a .py extension. It consists of instructions that the Python interpreter can execute sequentially. These scripts are written in plain text and can range from simple tasks like printing messages or performing basic calculations to complex operations such as web scraping, data analysis, or creating graphical user interfaces.
Python scripts are versatile and used for various purposes across different domains including web development, scientific computing, automation, and system administration. They leverage Python's readability, ease of use, and extensive libraries to accomplish tasks efficiently. To run a Python script, you invoke the Python interpreter from the command line followed by the filename of the script (python my_script.py).
The interpreter reads the script, interprets each line of code, and executes the instructions accordingly. Any output generated by the script, such as printed messages or computed results, is displayed in the command prompt or terminal window. Python scripts can also import modules and packages to extend functionality and reuse code, making them powerful tools for software development and rapid prototyping. Overall, Python scripts are fundamental components in leveraging Python's capabilities for creating applications and automating tasks across various platforms and environments.
Python is primarily considered a programming language rather than strictly a scripting language. The distinction between the two can sometimes be blurry, but here are the key points:
Python's design and versatility allow it to be used effectively for scripting purposes (writing small, quick scripts to automate tasks) as well as for developing large-scale applications. Therefore, while Python can be used as a scripting language, it is more accurately described as a powerful and versatile programming language that supports scripting among its many capabilities.
The Python interpreter is the core component of the Python programming language that executes Python code. It reads and interprets Python scripts (files containing Python code) and executes the instructions line by line. Here are key aspects of the Python interpreter:
Overall, the Python interpreter is fundamental to the execution and functionality of Python programs, providing a robust environment for developing applications, automating tasks, and performing data analysis across diverse computing environments.
To start the Python interpreter, follow these steps based on your operating system:
The Python interpreter in interactive mode allows you to test code snippets, explore libraries, and experiment with Python syntax directly. It's a valuable tool for learning Python and debugging small pieces of code before incorporating them into larger projects.
Running a Python script can be done in various ways, depending on your setup and requirements. You can execute scripts directly from the command line using python script_name.py, utilize integrated development environments (IDEs) like PyCharm or VS Code for debugging and advanced features, or even run scripts interactively in the Python shell.
For automation, scheduling tasks with cron jobs (on Unix-like systems) or Task Scheduler (Windows) is common, while web frameworks like Flask enable running scripts as part of web applications. Lastly, deploying scripts in containers (e.g., Docker) or serverless platforms (e.g., AWS Lambda) provides scalable execution options.
Each approach caters to different needs—from simple testing and development to complex automation and scalable deployments.There are several methods to run a Python script depending on your needs and environment:
1. Command Line/Terminal:
2. Integrated Development Environment (IDE):
3. Python Interactive Shell:
4. Scheduled Tasks (cron jobs, task scheduler):
5. Web Frameworks and Servers:
6. Containers and Serverless Environments:
Each method offers different capabilities and is suited to specific scenarios. Choosing the right method depends on factors such as project complexity, automation requirements, deployment environment, and personal preference for development and execution workflow.
Running Python code interactively allows you to execute commands or run scripts line by line directly within the Python interpreter or a Jupyter Notebook environment. Here’s how to do it:
1. Execute Code: Once you see the >>> prompt, you can start typing Python code directly. Press Enter after each line to execute it immediately.
>>> x = 10
>>> y = 5
>>> print(x + y)
15
2. Multiline Statements: For multiline statements like functions or loops, use an ellipsis ... to indicate continuation:
>>> def greet(name):
... print("Hello, " + name + "!")
...
>>> greet("Alice")
Hello, Alice!
3. Exit: To exit the Python interpreter, type exit() or press Ctrl + D (macOS/Linux) or Ctrl + Z followed by Enter (Windows).
Write and Execute Code: In a cell within the notebook, type your Python code and press Shift + Enter to execute it. Output (if any) will appear below the cell.
x = 10
y = 5
print(x + y)
Running Python interactively is useful for testing small snippets of code, exploring libraries, or experimenting with algorithms before integrating them into larger projects or scripts. It provides immediate feedback and allows for quick iteration and debugging.
Running a Python script using the command line is straightforward. Here’s a step-by-step guide:
1. Open Command Prompt/Terminal:
2. Navigate to the Directory:
Use the cd command to navigate to the directory where your Python script is located. For example:
cd path/to/your/script/directory
3. Run the Python Script:
Once you're in the correct directory, run your Python script by typing python script_name.py or python3 script_name.py (if you have multiple Python versions installed and need to specify Python 3):
python script_name.py
4. Execution:
5. Exiting:
This method is effective for running Python scripts from the command line and is commonly used for tasks such as automation, batch processing, or testing scripts without needing to open an IDE. It provides a straightforward way to interact with and execute Python code directly from the terminal or command prompt on various operating systems.
To run a Python script using Docker, follow these steps:
1. Create a Dockerfile:
Open the Dockerfile in a text editor and add the following lines:
# Use an official Python runtime as a parent image
FROM python:3
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy the current directory contents into the container at /usr/src/app
COPY . .
# Run script.py when the container launches
CMD ["python", "./script.py"]
2. Create Your Python Script:
3. Build the Docker Image:
Build the Docker image using the following command:
docker build -t my-python-app.
4. Run the Docker Container:
Once the Docker image is built, you can run a Docker container based on this image:
docker run my-python-app
5. View Output:
6. Stopping the Container:
This method allows you to package and run your Python script in a Docker container, providing isolation and ensuring that your script runs consistently across different environments without worrying about dependencies or system configurations. Adjust the Dockerfile and build process as needed based on specific requirements for your Python script and application.
Exiting a Python script can be achieved through various methods depending on your needs. The simplest way is to press Ctrl + C in the terminal or command prompt where the script is running, which sends a keyboard interrupt to terminate the script gracefully. Alternatively, using sys. exit() from the sys module allows you to programmatically exit the script at a specific point, while os._exit() from the os module terminates the script immediately without cleanup.
Python scripts also naturally exit when they reach the end of the file or complete the main function's execution. Handling exceptions and defining exit points within your script ensures controlled termination and proper cleanup of resources.
These methods provide flexibility based on different scenarios, ensuring scripts behave predictably and maintain system stability. Exiting or terminating a running Python script can be done in several ways depending on how the script is executed and its design:
1. Keyboard Interrupt (Ctrl + C):
2. Using sys.exit():
Example:
import sys
# Some code...
# Exit the script
sys.exit()
3. Using os._exit():
Example:
import os
# Some code...
# Exit the script immediately
os._exit(0)
4. End of Script Execution:
5. Handling Exceptions:
Example:
try:
# Code that may raise exceptions
...
except Exception as e:
# Handle the exception
...
# Optionally exit the script
sys.exit(1) # Exit with a non-zero status code indicating error
6. Closing the Interactive Interpreter:
Choose the method that best fits your script's logic and requirements for a clean and controlled exit. Handling exceptions and implementing appropriate cleanup routines (like closing files or connections) before exiting ensures that your script terminates gracefully and cleanly.
Running Python scripts using an Integrated Development Environment (IDE) or text editor involves opening your script in the respective tool and executing it with a click of a button or a keyboard shortcut.
IDEs like PyCharm or Visual Studio Code provide integrated debugging and project management features, while text editors such as Sublime Text or Atom offer simplicity and efficiency for running scripts via an integrated terminal or command line.
These tools streamline the development process by providing immediate feedback on script execution, facilitating code testing, debugging, and project organisation in a user-friendly environment. Running Python scripts using an Integrated Development Environment (IDE) or a text editor typically involves a few straightforward steps:
1. Open the IDE: Launch your preferred IDE (PyCharm, VS Code, etc.) and ensure your Python project or script is open.
2. Navigate to Script: Locate your Python script within the project directory structure.
3. Run the Script:
4. View Output: The IDE will execute the script and display any output (print statements, errors, etc.) in the output console or terminal window integrated into the IDE.
5. Debugging (Optional): IDEs offer debugging features like breakpoints, step-through execution, variable inspection, and more. Utilize these tools to troubleshoot and analyze script behavior interactively.
1. Open the Text Editor: Launch your preferred text editor and open your Python script.
2. Terminal Integration (Optional): Some text editors, like Sublime Text or Atom, have plugins or built-in features to run scripts directly from within the editor, utilizing an integrated terminal.
3. Run the Script:
4. View Output: The terminal or command prompt will display the script's output and any error messages generated during execution.
Running Python scripts via an IDE or text editor provides a convenient way to develop, test, and debug Python code with integrated tools and a user-friendly interface. IDEs offer more extensive features for project management, version control integration, and advanced debugging capabilities, making them ideal for larger projects and collaborative development. Text editors, while more lightweight, still offer essential functionality for running scripts efficiently in a straightforward manner.
How to Set Notepad to Run Python Program
Setting up Notepad to run Python programs involves a few manual steps because Notepad itself doesn't have built-in support for executing Python scripts like dedicated IDEs or text editors do. However, you can use Notepad to edit your Python code and then execute it via the command prompt (cmd) or PowerShell. Here’s a basic setup guide:
1. Install Python:
2. Create a Python Script:
3. Open Command Prompt (cmd):
4. Navigate to the Script Directory:
Use the cd command to navigate to the directory where your Python script is saved.
For example:
cd path\to\your\script\directory
5. Run the Python Script:
In the command prompt, type python my_script.py and press Enter.
python my_script.py
6. View Output:
Although Notepad lacks the integrated functionality of dedicated Python IDEs or text editors, you can effectively use it for writing Python code and executing scripts via the command line. For more advanced features like debugging or project management, consider using IDEs like PyCharm and Visual Studio Code or configuring more advanced text editors like Sublime Text or Atom with plugins for Python development.
To run a Python script directly in a Kivy file, you typically use the Kivy language (kv language) for defining the user interface and Python code for the application logic. Here’s a basic guide on how to achieve this:
1. Install Kivy:
Ensure Kivy is installed on your system. You can install it using pip:
pip install kivy
2. Create a Kivy File:
1. Create a Python Script:
from kivy.app import App
from kivy.uix.label import Label
from kivy.uix.boxlayout import BoxLayout
class MyApp(App):
def build(self):
# Load the kv file
return MyWidget()
class MyWidget(BoxLayout):
pass
if __name__ == '__main__':
MyApp().run()
2. In this example:
1. Link Python Script to Kivy File:
2. Run the Application:
Run the Python script:
python main.py
By following these steps, you can effectively run a Python script directly in a Kivy file, leveraging both the declarative design of the Kivy language for UI and the procedural power of Python for application logic.
Python script interactively typically involves executing commands or lines of code directly within a Python interpreter session or an interactive Python environment like Jupyter Notebook. Here’s how you can run Python scripts interactively:
1. Open Command Prompt/Terminal:
2. Launch Python Interpreter:
3. Execute Code:
>>> x = 10
>>> y = 5
>>> x + y
15
4. Multiline Statements:
>>> def add_numbers(a, b):
... return a + b
...
>>> add_numbers(10, 5)
15
5. Exit:
1. Launch Jupyter Notebook:
2. Create a New Notebook:
3. Write and Execute Code:
x = 10
y = 5
x + y
4. Add New Cells:
5. Save and Exit:
Running Python interactively allows you to test code snippets, explore libraries, and experiment with Python syntax in real-time. It’s particularly useful for rapid prototyping, learning, and data exploration tasks where immediate feedback and iteration are important.
Running Python scripts directly from a file manager (like Windows Explorer, macOS Finder, or Linux file managers) involves configuring the system to recognise and execute Python scripts with the Python interpreter. Here’s how you can set it up:
1. Associate .py Files with Python:
2. Run Python Scripts:
1. Set Execute Permissions:
Open Terminal and navigate to the directory containing your Python script:
cd path/to/your/script/directory
Make your script executable:
chmod +x script.py
2. Run Python Scripts:
1. Set Execute Permissions:
Open Terminal and navigate to the directory containing your Python script:
cd path/to/your/script/directory
Make your script executable:
chmod +x script.py
2. Run Python Scripts:
Running Python scripts from a file manager provides a convenient way to execute scripts without opening a terminal or command prompt manually. It leverages the system’s file association settings and permissions to seamlessly launch Python scripts with the appropriate interpreter directly from the graphical user interface.
Running Python scripts from a file manager offers several advantages:
running Python scripts directly from a file manager offers a user-friendly and convenient method to execute Python code without the need for command-line knowledge or interaction. This approach leverages the graphical interface of the operating system's file manager, allowing users to double-click on a .py file to initiate script execution simply. This method is advantageous for both technical and non-technical users alike. It enhances accessibility by integrating Python scripts into everyday workflows alongside other files and applications managed through the file manager.
This integration facilitates rapid testing, debugging, and iterative development cycles, as users can quickly modify scripts and observe their output in real time. Moreover, executing Python scripts from the file manager supports cross-platform compatibility, making it applicable across different operating systems such as Windows, macOS, and Linux. This versatility ensures a consistent experience for users regardless of their preferred platform. Overall, leveraging the file manager to run Python scripts enhances usability, promotes efficiency in script execution, and contributes to a smoother workflow for developers, analysts, and users engaging with Python applications in diverse computing environments.
Copy and paste below code to page Head section
You can run a Python script from the command line by navigating to the directory containing the script and typing python script_name.py. On some systems, you might need to use python3 instead of python depending on your Python installation.
Yes, you can run Python scripts using Integrated Development Environments (IDEs) like PyCharm or Visual Studio Code, or directly from file managers by associating .py files with Python.
Running Python interactively (using the Python interpreter or Jupyter Notebook) allows you to execute commands or lines of code one at a time and see immediate results. Running a script executes a complete set of instructions stored in a .py file from start to finish.
You can exit a running Python script by using Ctrl + C in the terminal or command prompt where the script is running, by calling sys.exit() or os._exit() within the script, or by letting the script reach its natural end.
Running Python scripts from a file manager provides ease of use, convenience for non-technical users, seamless integration with the operating system's graphical interface, and quick access to script execution without needing to open a terminal.
IDEs like PyCharm and Visual Studio Code offer built-in debugging tools such as breakpoints, variable inspection, and step-through execution. You can also use print statements or logging to debug scripts manually.