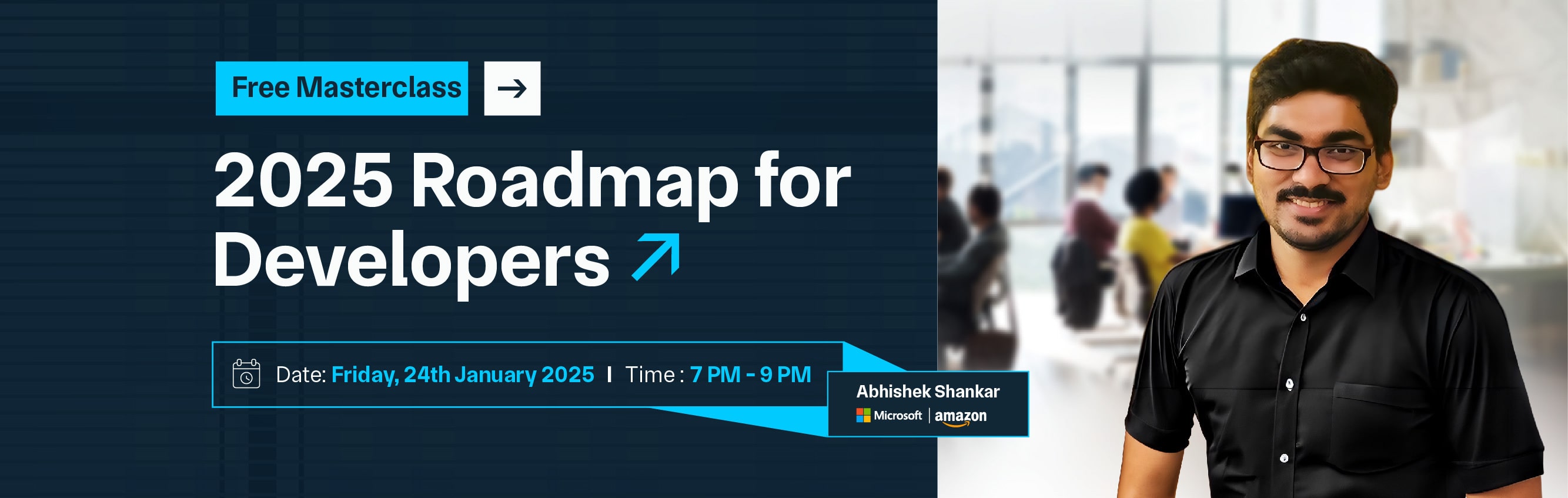

Installing Node.js and npm on Windows is straightforward and essential for JavaScript development. Begin by downloading the installer from the Node.js official website. Choose the LTS (Long-Term Support) version, which is recommended for most users due to its stability. Once the .msi file is downloaded, run the installer and follow the setup wizard. During the installation process, ensure you select the option to install npm (Node Package Manager) alongside Node.js.
After installation, it’s important to verify that both Node.js and npm are installed correctly. Open Command Prompt or PowerShell and type node -v to check the Node.js version installed. Similarly, type npm -v to see the npm version. Both commands should return version numbers, confirming a successful installation.
If you need to update npm to the latest version, you can do so by running npm install -g npm@latest in your Command Prompt or PowerShell. This setup will enable you to manage packages and dependencies effectively, paving the way for efficient JavaScript development. With Node.js and npm installed, you’re equipped to start building applications and using a wealth of libraries available in the npm ecosystem.
Node.js is an open-source, cross-platform JavaScript runtime environment that enables developers to execute JavaScript code server-side. Unlike traditional web servers that handle HTTP requests and responses, Node.js allows for the creation of scalable, high-performance applications by leveraging JavaScript’s asynchronous, event-driven architecture.
Here's a closer look at its key features:
In essence, Node.js extends JavaScript’s capabilities to server-side programming, allowing developers to build fast, scalable network applications efficiently.
npm, which stands for Node Package Manager, is a vital tool in the Node.js ecosystem. It is the default package manager for Node.js and serves several important functions:
Overall, npm simplifies the management of project dependencies, enhances productivity, and promotes code reuse within the Node.js ecosystem.
Before diving into the installation of Node.js on Windows, it's essential to ensure you have a few prerequisites in place. Having a compatible Windows operating system, administrative access, and a stable internet connection will streamline the setup process.
Additionally, while not strictly necessary, having a text editor or IDE can enhance your development experience. By preparing these elements in advance, you’ll be ready to quickly and efficiently install Node.js and start building your projects. Before installing and setting up Node.js on Windows, ensure you meet the following prerequisites.
1. Windows Operating System
2. Administrator Access
3. Internet Connection
4. Basic System Requirements
5. Optional: Text Editor or IDE
6. Previous Software Installations
Once these prerequisites are in place, you’re ready to download and install Node.js, setting up your environment for development with JavaScript.
Installing Node.js and npm on Windows is a quick and easy process that sets up your development environment for JavaScript applications. By following a few simple steps, you can download and install both tools, ensuring you're ready to start coding and managing packages efficiently.
This guide will walk you through each step to get Node.js and npm up and running on your Windows machine. Installing Node.js and npm on Windows is a straightforward process. Here’s a step-by-step guide to help you through it:
1. Visit the Node.js Website:
2. Choose the Installer:
1. Open the Installer:
2. Follow the Setup Wizard:
3. Complete the Installation:
1. Open Command Prompt:
2. Check Node.js Version:
3. Check npm Version:
1. Open Command Prompt:
2. Update npm:
1. Create a Test JavaScript File:
Enter the following code into app.js:
console.log('Hello, Node.js!');
In Command Prompt, navigate to the directory where app.js is saved. You can use the cd command to change directories.
For example:
cd path\to\your\directory
By following these steps, you’ve successfully installed Node.js and npm on Windows and verified that everything is working correctly. You’re now ready to start developing JavaScript applications and managing dependencies with npm.
To confirm if Node.js is installed on your Windows system, you can use Command Prompt or PowerShell to check the version of Node.js and npm. Simply open one of these terminals and run the commands node -v and npm -v.
If the installation is successful, you’ll see the version numbers for both Node.js and npm. If not, you may need to install or troubleshoot your Node.js setup. To verify if Node.js is installed on your system, follow these steps:
1. Open Command Prompt or PowerShell:
2. Check Node.js Version:
3. Check npm Version:
1. Open Command Prompt or PowerShell:
2. Run Node.js:
By following these steps, you can confirm whether Node.js and npm are properly installed on your Windows system.
Setting the NODE_HOME environment variable can be useful for specifying the installation directory of Node.js, especially if you have multiple versions installed or need to configure build tools. Here's how to set the NODE_HOME environment variable on a Windows system:
1. Find the Node.js Installation Path:
2. Open Environment Variables:
3. Add NODE_HOME Variable:
4. Update the PATH Variable (Optional but Recommended):
5. Verify the Setup:
By setting the NODE_HOME environment variable, you help ensure that Node.js tools and scripts can correctly locate the Node.js installation directory, aiding in various configurations and build processes.
To install packages using npm (Node Package Manager), you use the npm install command. Here’s how you use it in different scenarios:
Command:
npm install <package-name>
Example:
npm install express
This command installs the package express locally in the node_modules directory of your current project. It also updates the package.json file and creates or updates the package-lock.json file to include this dependency.
Command:
npm install <package-name>
Example:
npm install -g typescript
The -g flag stands for "global," meaning the package is installed globally on your system and is available for use across all projects. This is typically used for command-line tools or utilities that you want to access from anywhere on your system.
Command:
npm install <package-name>@<version>
Example:
npm install lodash@4.17.21
This command installs a specific version of a package. Replace <package-name> with the desired package name and <version> with the version number you want.
Command:
npm install
This command reads the package.json file in the current directory and installs all dependencies listed under dependencies and devDependencies. It’s commonly used after cloning a project from a repository.
Command:
npm uninstall <package-name>
Example:
npm uninstall express
This command removes the specified package from your project’s node_modules directory and updates the package.json and package-lock.json files accordingly. These commands are fundamental for managing packages and dependencies in a Node.js environment using npm.
Updating npm on Windows is a simple process that ensures you have the latest features and fixes. To update, open Command Prompt or PowerShell and run npm install -g npm@latest.
After the update completes, verify the new version with npm -v. This keeps your development environment current and secure. To update npm (Node Package Manager) on Windows, follow these steps:
Run the command:
npm -v
To update npm to the latest version, use the following command:
npm install -g npm@latest
After the update completes, check the new version of npm by running:
npm -v
By following these steps, you’ll ensure that npm is up-to-date, which provides access to the latest features, improvements, and security fixes.
Installing Node.js on Windows using Chocolatey, a popular package manager for Windows, is a convenient alternative to the traditional installer. Here’s how you can do it:
Install Chocolatey (if not already installed):
1. Open Command Prompt or PowerShell as Administrator:
For PowerShell, enter:
Set-ExecutionPolicy Bypass -Scope Process -Force; [System.Net.ServicePointManager]::SecurityProtocol = [System.Net.ServicePointManager]::SecurityProtocol -bor 3072; iex ((New-Object System.Net.WebClient).DownloadString('https://chocolatey.org/install.ps1'))
For Command Prompt, enter:
@powershell -NoProfile -InputFormat None -ExecutionPolicy Bypass -Command "iex ((New-Object System.Net.WebClient).DownloadString('https://chocolatey.org/install.ps1'))" -NoProfile -Command "choco upgrade chocolatey"
Install Node.js Using Chocolatey:
2. Open Command Prompt or PowerShell as Administrator:
Run the Installation Command:'
choco install nodejs-lts
3. Verify Installation:
Check Node.js Version:
node -v
Check npm Version:
npm -v
Using Chocolatey streamlines the installation process and integrates Node.js management into a broader package management system, making it a powerful alternative to manual installations.
Writing your first program in Node.js is a great way to get started with server-side JavaScript. Here’s a simple guide to help you create and run a basic Node.js program:
Ensure Node.js is installed on your system. You can check this by running:
node -v
If Node.js is not installed, follow the installation steps for your operating system.
1. Create a Directory:
Navigate to where you want to create your project directory and run:
mkdir my-node-app
cd my-node-app
2Initialize a Node.js Project (Optional):
1. Create a JavaScript File:
2. Write the Code:
Open app.js and add the following code:
// app.js
console.log('Hello, Node.js!');
1. Execute the File:
Run the program using Node.js:
node app.js
1. Add More Code:
2. Learn Node.js Modules:
By following these steps, you've created and executed your first Node.js program, providing a foundation to explore more complex projects and applications.
Uninstalling Node.js from your system can be done in a few straightforward steps, depending on your operating system. Here’s how you can remove Node.js from both Windows and macOS:
1. Open the Control Panel:
2. Navigate to Programs and Features:
3. Locate Node.js:
4. Uninstall Node.js:
5. Remove npm and Cache (Optional):
Open Command Prompt or PowerShell and run:
npm cache clean --force
6. Check for Residual Files:
Uninstalling Node.js might be necessary for various reasons, such as resolving conflicts, upgrading versions, or freeing up disk space. Here are some advantages and reasons for uninstalling Node.js:
1. Resolve Conflicts
2. Upgrade to a New Version
3. Free Up Disk Space
4. Fix Corrupted Installations
5. Remove Unnecessary Software
6. Compliance and Security
7. Troubleshooting
By understanding these advantages, you can make an informed decision about whether to uninstall Node.js and how to manage your development environment effectively.
Installing Node.js and npm is a crucial step for JavaScript developers looking to build and manage server-side applications efficiently. By following the installation process—whether using the official installer, Chocolatey on Windows, or a package manager on macOS—you ensure that both Node.js and npm are properly set up on your system.
This setup provides you with a powerful runtime environment for executing JavaScript and a robust package manager for handling dependencies. With Node.js and npm installed, you're equipped to start developing applications, manage project dependencies, and leverage a vast ecosystem of libraries and tools, paving the way for efficient and effective coding practices.
Copy and paste below code to page Head section
Node.js is a JavaScript runtime built on Chrome's V8 engine, allowing you to run JavaScript code on the server side. It is commonly used for building scalable network applications and servers. Installing Node.js enables you to develop server-side applications and utilise npm for managing packages and dependencies.
npm (Node Package Manager) is a package manager for Node.js that helps you manage and install libraries, frameworks, and tools needed for your projects. It simplifies the process of handling dependencies and integrating third-party packages into your applications.
Open Command Prompt or PowerShell and run node -v to check the Node.js version and npm -v to check the npm version. If the commands return version numbers, Node.js and npm are installed.
Ensure that you have a stable internet connection and administrative privileges. For installation issues, try running the installer or commands as an administrator. Check for any error messages for guidance on troubleshooting. You may also need to reinstall Node.js or update your PATH environment variable.
Yes, you can manage multiple versions of Node.js using tools like nvm (Node Version Manager) on macOS or Linux, and nvm-windows on Windows. These tools allow you to switch between different Node.js versions easily.
To update Node.js and npm, you can use the npm command npm install -g npm@latest to update npm to its latest version. For Node.js, you may need to download the latest installer from the Node.js website or use a version manager like nvm.