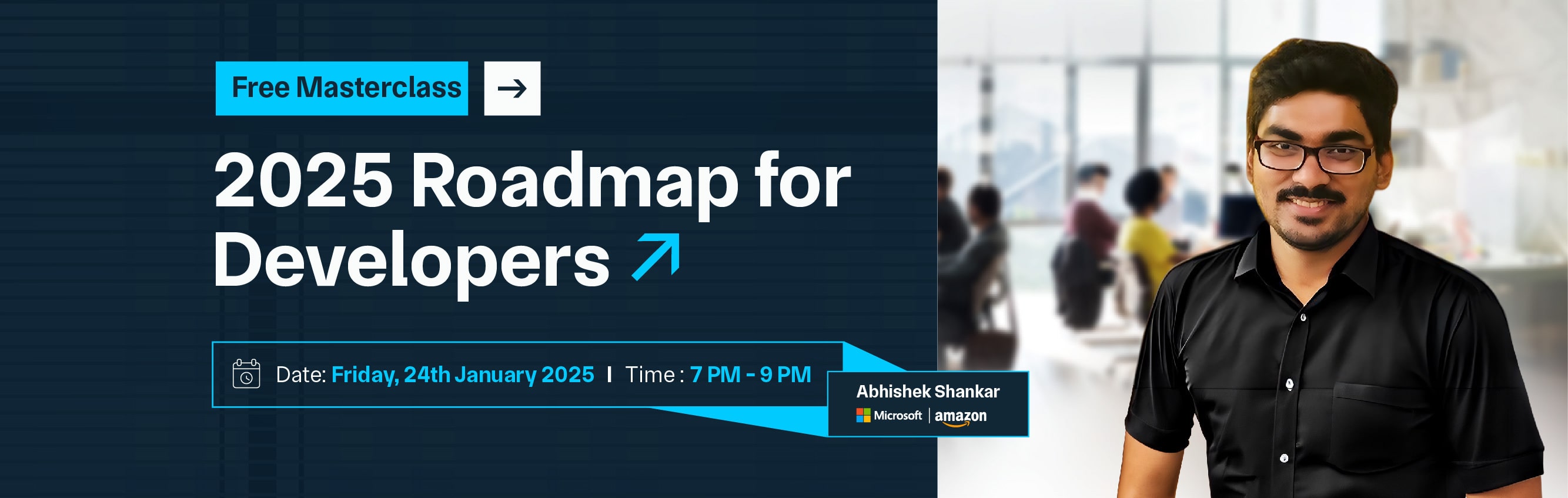

Higher-order functions (HOFs) in React are functions that take other functions as arguments or return a function as a result. They are a powerful concept derived from functional programming and are widely used in React to enhance the functionality and reusability of components. In React, HOFs can be used to modify or extend the behavior of components without altering their core logic.
A common example of a higher-order function in React is a Higher-Order Component (HOC). HOCs are functions that accept a component as an argument and return a new component with additional props or functionality. This allows developers to reuse logic across multiple components without repeating code. For example, a HOC can be used to add authentication checks or loading indicators to multiple components.
Another pattern similar to HOCs is the render prop, where a function is passed as a prop to a component to control how its UI is rendered. Both HOCs and render props help achieve code reuse, separation of concerns, and composability, which are core principles in React development.
A Higher-Order Component (HOC) is a pattern in React that allows you to enhance or modify the functionality of a component without changing its original code. It is a function that takes a component as an argument and returns a new component with additional props or behavior. HOCs enable you to reuse component logic across your application in a modular and efficient way, promoting code reusability and separation of concerns.
In simpler terms, a HOC is a function that wraps a component to add additional functionality, such as adding authentication, fetching data, or injecting props. HOCs don’t modify the original component; instead, they wrap it in a higher-level component with enhanced features.
Here’s a basic example of an HOC that adds a loading spinner to any component:
// HOC to add a loading spinner
function withLoading(Component) {
return function WithLoadingComponent({ isLoading, ...props }) {
if (isLoading) {
return <div>Loading...</div>;
}
return <Component {...props} />;
};
}
// Usage
function MyComponent({ data }) {
return <div>{data}</div>;
}
const MyComponentWithLoading = withLoading(MyComponent);
// Usage of the enhanced component
<MyComponentWithLoading isLoading={true} data="Data Loaded!" />
Higher-order functions (HOFs) are an essential concept in React and JavaScript, enabling you to modify or extend the behavior of components or functions without altering their core logic.
While React commonly uses Higher-Order Components (HOCs), several other HOFs are frequently used in JavaScript and React for various purposes like handling side effects, managing state, or enhancing component behavior. Below are some common higher-order functions in React development:
function withAuth(Component) {
return function WithAuth(props) {
if (!props. authenticated) {
Return <div>You must be logged in to view this content</div>;
}
return <Component {...props} />;
};
}
const items = ['Apple,' 'Banana,' 'Cherry'];
const listItems = items.map((item, index) => <li key={index}>{item}</li>);
function withState(Component) {
return function EnhancedComponent(props) {
const [state, setState] = useState(false);
return <Component {...props} state={state} setState={setState} />;
};
}
import { withRouter } from 'react-router-dom';
function MyComponent(props) {
const navigateToHome = () => {
props.history.push('/home');
};
return <button onClick={navigateToHome}>Go to Home</button>;
}
export default withRouter(MyComponent);
function withErrorBoundary(Component) {
return function ErrorBoundaryComponent(props) {
try {
return <Component {...props} />;
} catch (error) {
return <div>Error occurred: {error.message}</div>;
}
};
}
Higher-order components (HOCs) are a powerful pattern in React for reusing logic across multiple components. However, like any tool, they should be used in the right scenarios. HOCs can help make your React code more modular, reusable, and maintainable, but knowing when to use them is key to avoiding unnecessary complexity.
Here are some scenarios where HOCs are particularly useful in your React code:
When to use it: If you have logic that you need to apply to multiple components, HOCs are a great way to abstract and reuse that logic.
Example: Adding authentication checks, logging, or data fetching logic that needs to be applied to several components.
Why use HOCs: By wrapping components with an HOC, you can apply the same logic across many components without duplicating code.
function without(Component) {
return function WithAuth(props) {
if (!props. authenticated) {
Return <div>You need to log in to view this page</div>;
}
return <Component {...props} />;
};
}
When to use it: If you want to keep the UI logic separate from business logic, HOCs are ideal. For instance, if you need to add features like data fetching or error handling but want to make sure your components are clear with that logic.
Example: Fetching data from an API or managing loading states without mixing that logic directly into your component’s render method.
Why use HOCs: It promotes cleaner, more maintainable components by keeping your components focused on rendering UI rather than managing side effects or business logic.
function withDataFetching(Component) {
return function WithDataFetching(props) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetchData().then(data => {
setData(data);
setLoading(false);
});
}, []);
if (loading) return <div>Loading...</div>;
return <Component {...props} data={data} />;
};
}
When to use it: When you need to conditionally change the behavior or appearance of a component based on props or state.
Example: Conditionally rendering elements, handling permission checks, or adding a loading spinner.
Why use HOCs: HOCs allow you to abstract away these conditional behaviors, so your component logic remains focused on its core functionality.
function with loading(Component) {
return function WithLoading({ isLoading, ...props }) {
if (isLoading) {
return <div>Loading...</div>;
}
return <Component {...props} />;
};
A Higher-Order Function (HOF) is a function that either:
This concept is widely used in functional programming and can be applied in JavaScript to create more modular, reusable, and clean code. The key idea behind a higher-order function is that it treats other functions as first-class citizens, meaning they can be passed around and manipulated like any other data.
You can define a higher-order function that takes another function as an argument. This allows you to pass custom behavior or logic into your function.
function greet(name, callback) {
return `Hello, ${callback(name)}!`;
}
function capitalize(name) {
return name.toUpperCase();
}
console.log(greet("Alice", capitalize)); // Output: Hello, ALICE!
In this example, greet is a higher-order function because it accepts a function (capitalize) as an argument. The capitalize function is applied inside the greet function.
A higher-order function can also return a function that is customized with specific behavior.
function multiplier(factor) {
return function (number) {
return number * factor;
};
}
const double = multiplier(2);
console.log(double(5)); // Output: 10
const triple = multiplier(3);
console.log(triple(5)); // Output: 15
A higher-order function can both take a function as an argument and return a new function, combining both of the concepts.
function with logging(func) {
return function(...args) {
console.log('Arguments:', args);
const result = func(...args);
console.log('Result:', result);
return result;
};
}
function add(a, b) {
return a + b;
}
const addWithLogging = withLogging(add);
addWithLogging(3, 5);
// Output:
// Arguments: [3, 5]
// Result: 8
Here, the with logging function takes another function (add) as an argument and returns a new function that logs the arguments and results before calling the original add function.
A Higher-Order Function (HOF) is a function that either:
In simpler terms, a higher-order function is a function that works with other functions — it can either accept a function as an argument or return a function.
You can define a higher-order function that takes another function as a parameter. This is useful when you want to allow customization or extend the behavior of a function.
function greet(name, callback) {
return `Hello, ${callback(name)}!`;
}
function capitalize(name) {
return name.toUpperCase();
}
console.log(greet('Alice', capitalize)); // Output: "Hello, ALICE!"
In this example:
A higher-order function can also return a new function. This is useful for creating functions with customized behavior.
function multiplier(factor) {
return function(number) {
return number * factor;
};
}
const double = multiplier(2); // Create a function that multiplies by 2
console.log(double(5)); // Output: 10
const triple = multiplier(3); // Create a function that multiplies by 3
console.log(triple(5)); // Output: 15
In this example:
A higher-order function can take a function as an argument and also return a function.
function with logging(fn) {
return function(...args) {
console.log('Arguments:', args);
const result = fn(...args);
console.log('Result:', result);
return result;
};
}
function add(a, b) {
return a + b;
}
const addWithLogging = withLogging(add);
addWithLogging(3, 5);
// Output:
// Arguments: [3, 5]
// Result: 8
In React, a Higher-Order Component (HOC) is a pattern used to reuse component logic. A HOC is a function that takes a component and returns a new component, usually with additional props, functionality, or behavior.
HOCs are commonly used for tasks like authentication checks, data fetching, form handling, or styling components with additional features. The idea is to create a reusable logic container that can enhance the functionality of any component without directly modifying it.
An HOC is a function that takes a component as an argument and returns a new component. The new component typically renders the original component but adds extra functionality, such as additional props or side effects.
// A simple HOC that adds a "loading" prop to a component
function withLoading(Component) {
return function WithLoading({ isLoading, ...props }) {
if (isLoading) {
return <div>Loading...</div>;
}
return <Component {...props} />;
};
}
Here:
Now, you can use the HOC to enhance a component. This is done by passing the component to the HOC as an argument.
// A sample component that displays user info
function UserInfo({ name, age }) {
return (
<div>
<h2>{name}</h2>
<p>Age: {age}</p>
</div>
);
}
// Enhance UserInfo with the HOC
const UserInfoWithLoading = withLoading(UserInfo);
// Use the enhanced component
function App() {
const [isLoading, setIsLoading] = React.useState(true);
// Simulate data fetching
React.useEffect(() => {
setTimeout(() => setIsLoading(false), 2000); // Simulate a 2-second delay
}, []);
return (
<div>
<UserInfoWithLoading isLoading={isLoading} name="Alice" age={30} />
</div>
);
}
export default App;
In this example:
The HOC should forward all the props it receives to the wrapped component. This ensures that the enhanced component can still receive and utilize all the props it was originally designed to accept.
function withLogging(Component) {
return function WithLogging(props) {
console.log('Component props:', props);
return <Component {...props} />;
};
}
In this example:
You can apply multiple HOCs to a single component, and they will be executed in order (from bottom to top).
function withAuth(Component) {
return function WithAuth(props) {
if (!props.authenticated) {
return <div>You need to log in</div>;
}
return <Component {...props} />;
};
}
function withLogging(Component) {
return function WithLogging(props) {
console.log('Rendering component with props:', props);
return <Component {...props} />;
};
}
const EnhancedComponent = withLogging(withAuth(UserInfo));
In this example:
If you need to forward refs to the wrapped component (for example, when dealing with DOM elements), use React.forwardRef to pass the ref down.
function withFocus(Component) {
const ForwardedComponent = React.forwardRef((props, ref) => {
return <Component {...props} forwardedRef={ref} />;
});
return ForwardedComponent;
}
Here:
Let’s walk through a practical example of creating and using a Higher-Order Component (HOC) in React. We’ll build an HOC that handles loading states in a component. This HOC will add the ability to show a loading spinner while the data is being fetched and will display the actual component once the data is loaded.
We want to create an HOC that:
This is a typical use case for HOCs adding additional behavior to a component without modifying the original component itself.
First, we will create a withholding HOC that will wrap any component. The withLoading HOC will manage the loading state and data fetching logic and pass the necessary props to the wrapped component.
import React, { useState, useEffect } from 'react';
// Higher-Order Component that handles loading state
function withLoading(Component) {
return function WithLoading({ isLoading, data, ...props }) {
// If the data is not loaded, show a loading spinner
if (isLoading) {
return <div>Loading...</div>;
}
// Once data is loaded, render the passed component with the data
return <Component {...props} data={data} />;
};
}
export default withholding;
Now, let’s create a component that will display some user information. This component will receive the data prop (passed through the HOC) and display it.
import React from 'react';
// The component that displays user data
function UserProfile({ data }) {
return (
<div>
<h2>{data.name}</h2>
<p>Age: {data.age}</p>
<p>Email: {data.email}</p>
</div>
);
}
export default UserProfile;
This component receives data (which contains user information) as a prop and displays it.
Finally, we’ll integrate everything in the main App.js file. We’ll wrap the UserProfile component with the withLoading HOC to add the loading functionality.
import React, { useState, useEffect } from 'react';
import UserProfile from './UserProfile';
Import with loading from './withLoading';
// Create the enhanced component using the withholding HOC
const UserProfileWithLoading = withLoading(UserProfile);
function App() {
const [isLoading, setIsLoading] = useState(true);
const [userData, setUserData] = useState(null);
// Simulate data fetching
useEffect(() => {
setTimeout(() => {
// Once data is fetched, set the state to stop loading
setUserData({
name: 'John Doe',
age: 28,
email: 'john.doe@example.com',
});
setIsLoading(false);
}, 2000); // Simulate a 2-second delay
}, []);
return (
<div>
<h1>User Information</h1>
<UserProfileWithLoading isLoading={isLoading} data={userData} />
</div>
);
}
export default App;
Higher-order components (HOCs) in React are functions that take a component and return a new component with additional props or behavior. In this article, we’ll explore two practical examples of how to build and use HOCs to enhance components in React. The examples will cover conditional rendering (like user authentication) and logging behavior for tracking renders.
A common use case for HOCs is authorization or authentication checking if a user is authorized to access a certain page or feature. We can create an HOC to wrap a component and ensure the user is authenticated before rendering the component.
We need to protect access to a Dashboard component. If the user is not authenticated, we want to redirect them to a login page. If they are authenticated, we will display the Dashboard.
import React from 'react';
import { Redirect } from 'react-router-dom';
// Higher-order component for authentication
function withAuthorization(Component) {
return function WithAuthorization(props) {
const isAuthenticated = false; // For example, authentication state
if (!isAuthenticated) {
// If the user is not authenticated, redirect to the login
return <Redirect to="/login" />;
}
// If the user is authenticated, render the passed component
return <Component {...props} />;
};
}
export default with authorization;
import React from 'react';
// The component to be protected
function Dashboard() {
return (
<div>
<h1>Welcome to your Dashboard</h1>
</div>
);
}
export default Dashboard;
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import with authorization from './withAuthorization';
import Dashboard from './Dashboard';
function App() {
const DashboardWithAuth = withAuthorization(Dashboard); // Wrap the Dashboard component with the HOC
return (
<Router>
<Switch>
<Route path="/dashboard" component={DashboardWithAuth} />
<Route path="/login" render={() => <h2>Login Page</h2>} />
</Switch>
</Router>
);
}
export default App;
Another common use case for HOCs is adding logging behavior to components. For example, we can track when a component re-renders or logs the props passed to it.
We want to log the props and the number of times a component has been re-rendered. This can be useful for debugging and performance optimizations.
import React, { useEffect, useRef } from 'react';
// Higher-order component for logging props and render count
function withLogging(Component) {
return function WithLogging(props) {
const renderCount = useRef(0); // Track the number of times the component has rendered
useEffect(() => {
renderCount.current += 1; // Increment on each render
});
// Log the props and render count
console.log('Component props:', props);
console.log('Render count:', renderCount.current);
// Render the original component
return <Component {...props} />;
};
}
export default with logging;
import React from 'react';
function Profile({ name, age }) {
return (
<div>
<h1>{name}</h1>
<p>Age: {age}</p>
</div>
);
}
export default Profile;
import React, { useState } from 'react';
Import with logging from './withLogging';
import Profile from './Profile';
// Wrap the Profile component with the HOC
const ProfileWithLogging = withLogging(Profile);
function App() {
const [name, setName] = useState('John Doe');
const [age, setAge] = useState(30);
return (
<div>
<ProfileWithLogging name={name} age={age} />
<button onClick={() => setAge(age + 1)}>Increase Age</button>
</div>
);
}
export default App;
Higher-order components (HOCs) can sometimes make debugging more challenging because they introduce an additional layer of abstraction, making it harder to trace what’s happening inside a component. However, with the right approach, debugging HOCs becomes much easier. In this article, we'll cover practical strategies and techniques to debug HOCs in React effectively.
Higher-order components (HOCs) are a powerful feature in React, but there are some important guidelines you should follow to make your code clean and bug-free. Here are the key things to remember when using HOCs:
A pure function does not change or mutate its inputs. When building a HOC, make sure it doesn’t change the state or props of the wrapped component unexpectedly. It should only enhance or add behavior to the wrapped component, not alter it.
HOCs should forward all received props to the component they are wrapping. Remember to pass props through if you have a specific reason to modify them. Ensure that no props are dropped or incorrectly handled, as this can lead to bugs.
HOCs are useful for reusing logic across multiple components. However, use them sparingly. Using HOCs can make your code more complicated and easier to manage. For simpler scenarios, consider using other patterns like custom hooks or render props.
When you wrap a component in a HOC, React may show it as WithLogging(Component) in developer tools, which isn’t very clear. Use the displayName property to give the wrapped component a more meaningful name for easier debugging.
WithLogging.displayName = `WithLogging(${Component.displayName || Component.name})`;
Since HOCs wrap components and create new component instances, this can sometimes cause unnecessary re-renders. Use React. Memo or useMemo to optimize performance and avoid re-rendering the component unless its props change.
Higher-order components (HOCs) provide several advantages that make them an essential part of React development. By abstracting and reusing logic, HOCs can improve code modularity, maintainability, and performance. Below are the key benefits of using HOCs in Re
Higher-order components (HOCs) are a powerful and flexible pattern in React that allows developers to enhance the functionality of components without modifying their original code. By abstracting shared logic into reusable units, HOCs help promote code reusability, modularity, and separation of concerns.
The key advantages of HOCs include the ability to share functionality across components, keep components clean and focused on rendering UI, optimize performance through memoization, and encapsulate side effects like data fetching or error handling. Additionally, HOCs facilitate better testability, easier maintenance, and debugging by isolating logic into independent, reusable functions.
Copy and paste below code to page Head section
A HOC is a function that takes a component and returns a new component with added behavior or functionality.
Reusing logic (e.g., authentication, logging). Conditional rendering (e.g., loading states). Enhancing components with extra behavior (e.g., error boundaries).
A HOC wraps a component and returns a new component with enhanced functionality, usually by modifying props or adding additional logic.
Yes, HOCs can modify, add, or remove props before passing them to the wrapped component.
HOCs enhance components with extra behavior, while hooks manage state and side effects inside functional components.
This can lead to deeply nested components. Potential prop name conflicts. It may impact performance if overused.