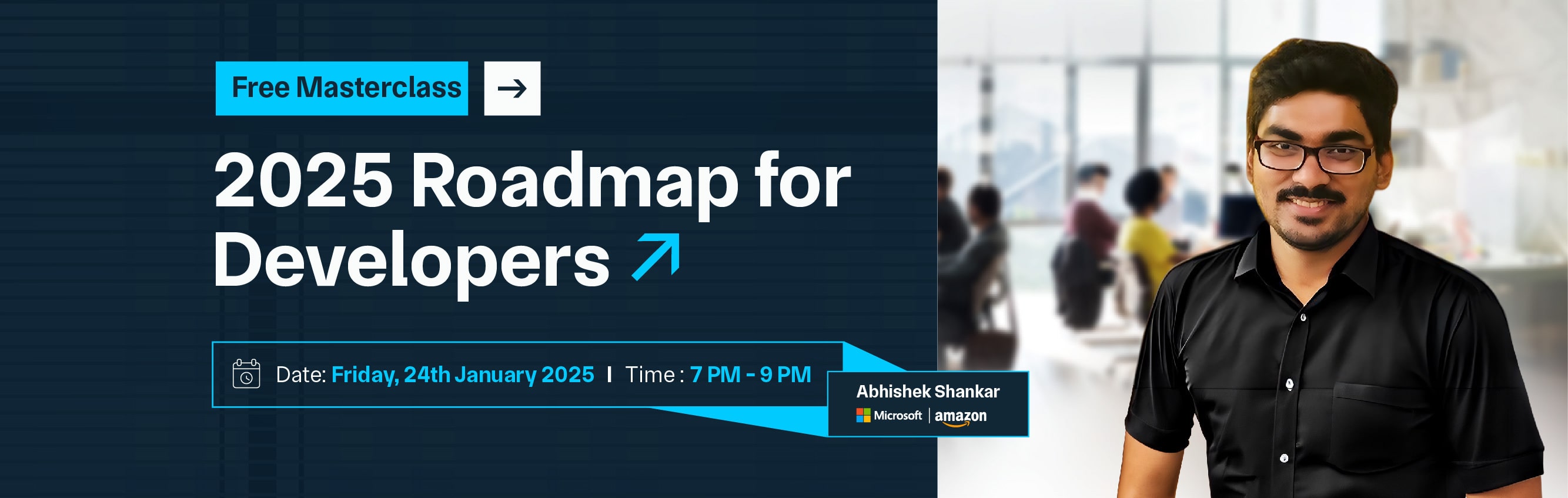

An array is a data structure in programming that stores a collection of elements, all of the same type, in a contiguous block of memory. It allows efficient data management by enabling quick access to its elements using an index. For example, in Python, an array can be represented as a list: grades = [90, 85, 88, 92], where each element corresponds to a student's score.
Arrays are indexed, starting from 0, meaning grades[0] would give you the first element (90), and grades[3] would access the fourth element (92). Arrays can be one-dimensional, as shown in the example above, or multidimensional, like a matrix. For instance, in Java, a two-dimensional array can represent a matrix: int[][] matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}.
Arrays are commonly used in scenarios where quick and efficient access to data is required, such as in sorting algorithms, processing numerical data, or representing complex structures like grids. However, arrays have some limitations: they have a fixed size in most programming languages, meaning the number of elements cannot be changed after creation. Despite this, arrays remain a fundamental and efficient tool for managing collections of data in programming.
An array is a data structure used in programming to store a fixed-size sequence of elements of the same data type. It is a collection of variables that are accessed using an index or a key, making it easy to store and manipulate multiple values under a single variable name. In most programming languages, arrays are indexed starting from 0, so the first element is accessed at index 0, the second element at index 1, and so on. For example, an array of integers in Python could be written as numbers = [10, 20, 30, 40]. In this case, numbers[0] would refer to the value 10, and numbers[2] would refer to 30.
Arrays allow efficient access to data, especially when you need to perform operations on large sets of values. They are often used in algorithms that require fast access to sequential data, such as sorting or searching. Arrays can be one-dimensional (like a list of values) or multidimensional (like a matrix with rows and columns). However, arrays typically have a fixed size, which means once they are defined, the number of elements cannot be changed. Despite this limitation, arrays remain one of the most essential and widely used data structures in programming.
Arrays have several key characteristics that make them an important data structure in programming. These characteristics include:
The syntax for creating and using arrays varies slightly across programming languages. Below are examples of array syntax in popular languages:
# Creating a 1D array (list in Python)
array = [10, 20, 30, 40]
# Accessing elements (0-based indexing)
print(array[0]) # Output: 10
print(array[2]) # Output: 30
// Creating and initializing an array
int[] array = {10, 20, 30, 40};
// Accessing elements (0-based indexing)
System.out.println(array[0]); // Output: 10
System.out.println(array[2]); // Output: 30
int[] array = new int[4]; // Creates an array of size 4
// Creating and initializing an array
int array[] = {10, 20, 30, 40};
// Accessing elements (0-based indexing)
cout << array[0] << endl; // Output: 10
cout << array[2] << endl; // Output: 30
int array[4]; // Creates an array of size 4
// Creating an array
let array = [10, 20, 30, 40];
// Accessing elements (0-based indexing)
console.log(array[0]); // Output: 10
console.log(array[2]); // Output: 30
// Creating and initializing an array
int[] array = {10, 20, 30, 40};
// Accessing elements (0-based indexing)
Console.WriteLine(array[0]); // Output: 10
Console.WriteLine(array[2]); // Output: 30
// Creating an indexed array
$array = [10, 20, 30, 40];
// Accessing elements (0-based indexing)
echo $array[0]; // Output: 10
echo $array[2]; // Output: 30
Arrays can be categorized into several types based on the number of dimensions they have and how they are structured. Here are the main types of arrays:
A one-dimensional array is the simplest form of an array, where data is stored in a linear sequence. It is like a list of values.
Example:
It is used to store a list of similar data items, such as numbers or strings.
A two-dimensional array is an array of arrays, essentially a matrix with rows and columns.
Example:
2D arrays are commonly used for representing tabular data, grids, or matrices.
A multidimensional array is an array with more than two dimensions. It is useful for representing higher-dimensional data, like 3D data.
Example:
These arrays are used in scientific computations, simulations, and scenarios where data is structured in more than two dimensions.
A jagged array is an array of arrays where each "inner array" can have a different size. This is particularly useful when dealing with data that does not follow a regular structure.
Example:
Unlike a 2D array, where each row must have the same number of elements, jagged arrays allow rows to have different lengths.
A dynamic array is an array that can grow or shrink in size during runtime. Unlike static arrays, dynamic arrays can adjust their size when elements are added or removed.
Example (in Python, lists function as dynamic arrays):
Dynamic arrays are commonly used in languages like Python, JavaScript, or C++ (via std::vector).
An associative array is a collection of key-value pairs where each element has a unique key associated with it. Although this is technically not a traditional array, it shares similarities in data organization.
Example:
This type of array is often used when you need to map unique keys to specific values, similar to a dictionary or hash map.
Here are a few examples of how arrays are used in different programming scenarios:
Example (Python):
grades = [85, 90, 78, 92, 88] # 1D Array (List)
print(grades[0]) # Output: 85 (First student's grade)
print(grades[3]) # Output: 92 (Fourth student's grade)
Example (Java):
char[][] board = {
{'X', 'O', 'X'},
{'O', 'X', 'O'},
{'X', 'O', 'X'}
};
// Accessing elements
System.out.println(board[0][0]); // Output: X (Top-left corner)
System.out.println(board[2][2]); // Output: X (Bottom-right corner)
Example (Python):
matrix_a = [[1, 2], [3, 4]]
matrix_b = [[5, 6], [7, 8]]
result = [[0, 0], [0, 0]]
for i in range(2):
for j in range(2):
for k in range(2):
result[i][j] += matrix_a[i][k] * matrix_b[k][j]
print(result) # Output: [[19, 22], [43, 50]]
Example (C++):
float prices[] = {12.99, 8.50, 15.75, 7.30}; // 1D Array
cout << "Price of first product: " << prices[0] << endl; // Output: 12.99
cout << "Price of second product: " << prices[1] << endl; // Output: 8.50
Example (Java):
String[][] employeePhones = {
{"123-456-7890", "987-654-3210"}, // Employee 1
{"555-555-5555"}, // Employee 2
{"666-666-6666", "777-777-7777"} // Employee 3
};
// Accessing elements
System.out.println(employeePhones[0][1]); // Output: 987-654-3210
System.out.println(employeePhones[1][0]); // Output: 555-555-5555
Example (Python):
image = [
[[255, 0, 0], [0, 255, 0], [0, 0, 255]], # First row of pixels (RGB)
[[255, 255, 0], [0, 255, 255], [255, 0, 255]] # Second row of pixels (RGB)
]
print(image[0][0]) # Output: [255, 0, 0] (Red color)
print(image[1][2]) # Output: [255, 0, 255] (Purple color)
Example (Python):
numbers = [1, 2, 3, 4]
numbers.append(5) # Dynamically adding a new element
print(numbers) # Output: [1, 2, 3, 4, 5]
Example (JavaScript):
let user = {
"name": "Alice",
"age": 25
};
console.log(user["name"]); // Output: Alice
console.log(user["age"]); // Output: 25
These examples show how arrays can be used to solve various types of real-world problems, from managing simple data like grades or prices to more complex tasks like matrix operations or image representation. The flexibility and efficiency of arrays make them essential in almost every programming application.
Arrays offer several advantages in programming, making them a fundamental data structure for managing and manipulating data. Some key advantages of using arrays include:
These advantages make arrays an essential and efficient data structure in programming, particularly when working with large datasets or when memory and speed are critical considerations.
While arrays are a fundamental and widely used data structure, they come with several limitations that can impact their usefulness in certain situations. Here are the key limitations of arrays:
Array operations are fundamental actions that can be performed on arrays to manipulate and access the data stored within them. Below are common array operations, along with a brief explanation of each:
Description: Accessing an element in an array involves using its index (position) in the array.
Description: Inserting an element into an array involves adding a new value either at the beginning, middle, or end.
Description: Deleting an element from an array involves removing a specific element by shifting elements to close the gap.
Description: Updating an element involves changing the value of an existing element at a specific index.
Description: Searching for an element in an array involves finding whether an element exists in the array and, if so, returning its index.
Description: Reversing an array involves changing the order of elements such that the first element becomes the last and vice versa.
Description: Finding the length of the array returns the number of elements present in the array.
Description: Concatenation involves joining two or more arrays into a single array.
Description: Copying an array involves creating a new array with the same elements as the original.
Description: Finding the maximum or minimum element in an array involves iterating over the array and comparing each element.
Description: Checking if a specific element exists in the array.
Description: Merging two arrays combines elements from both arrays into a new one.
Arrays are one of the most commonly used data structures in programming due to their simplicity and efficiency. Below are some common use cases where arrays play a critical role:
Arrays are ideal for storing collections of elements that are of the same data type. This is especially useful when you need to handle a large set of related data values.
For example, you might store the marks of students in an exam, where each element of the array represents a student's score. Using arrays, you can easily access and manipulate these values using indices.
In mathematical operations, arrays play a vital role in representing and manipulating matrices. Matrices, which are essentially two-dimensional arrays, are used in various mathematical fields, including linear algebra, computer graphics, and machine learning. Arrays allow you to efficiently perform operations such as matrix multiplication or inversion by treating each element as a matrix entry.
Arrays are perfect when you need to handle a fixed set of values whose size is known in advance. For example, if you have a predefined set of items, like the days of the week or the months of the year, you can use arrays to store these values. Arrays are efficient in such cases because their size is constant and doesn't require dynamic resizing during the program's execution.
Arrays are widely used in searching and sorting tasks. Whether you’re looking for a specific element or sorting an array in ascending or descending order, arrays are the structure of choice.
For example, you can implement algorithms like binary search for fast lookups in sorted arrays or quicksort to reorder elements. Arrays allow these algorithms to work efficiently and quickly, making them crucial in many software applications.
Arrays can be used to implement data structures like stacks and queues. A stack operates on a "last-in, first-out" (LIFO) principle, while a queue follows a "first-in, first-out" (FIFO) principle.
In both cases, arrays can provide a simple and efficient underlying structure. For example, in a stack, you push elements to the end of the array and pop them from the same end. In a queue, elements are added at the end and removed from the front.
Arrays are commonly used in caching mechanisms, where you store temporary data to speed up future access. For example, web applications often use arrays to store recent user queries or frequently accessed data, allowing for quick retrieval without repeated database access. The fixed-size nature of arrays can make them an efficient choice for this purpose, as long as the data set doesn’t change significantly.
Arrays are a fundamental data structure that plays a crucial role in computer programming and data management. They provide an efficient way to store and access multiple values of the same type in a structured and predictable manner. Arrays are commonly used in a wide range of applications, from storing data in scientific computing and image processing to handling collections of items in everyday programming tasks.
For example, if we want to store the ages of five students, an array allows us to store each student's age in a single container accessed by an index. This makes it easier to manipulate, search, or perform operations like calculating the average age, sorting the values, or even applying algorithms to the data.
Copy and paste below code to page Head section
An array is a data structure that stores a fixed-size sequence of elements of the same data type. It allows you to store multiple values in a single variable, making it easy to work with a collection of similar data.
There are several types of arrays: One-dimensional array: A simple list of elements. Two-dimensional array: A table-like structure often used to represent matrices. Multidimensional array: An array with more than two dimensions used for complex data structures. Jagged array: An array of arrays where each "sub-array" can have a different size.
In most programming languages, an array is declared by specifying the type of elements it will store and its size. For example, in C++: int arr[5]; // Declares an integer array of size 5
Efficiency: Arrays allow for fast access to elements via indexing. Memory management: Arrays provide a simple and fixed-size data storage solution. Ease of use: Arrays are simple to implement and use for storing and processing data.
Fixed-size: The size of an array is defined at the time of creation and cannot be changed dynamically. Homogeneous data: All elements in an array must be of the same data type. Memory wastage: If the array is larger than needed, unused spaces can lead to wasted memory.
Arrays: Have a fixed size, elements are stored in contiguous memory locations, and provide fast access using indices. Lists: In languages like Python, lists are dynamic, can store different data types, and grow or shrink as needed. Linked lists: Consists of nodes where each node points to the next, offering flexibility in size but with slower access times compared to arrays.