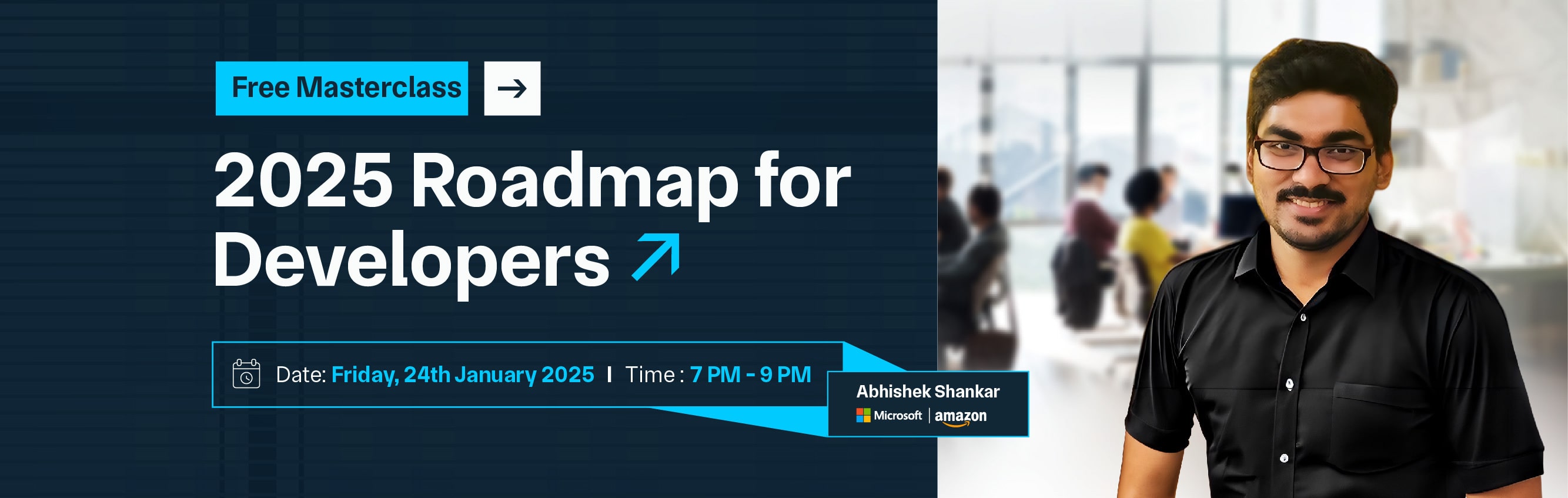

Data Structures and Algorithms (DSA) projects involve designing and implementing efficient solutions to computational problems using various data structures and algorithms. These projects typically encompass a range of tasks, including defining and creating data structures like arrays, linked lists, trees, and graphs and applying algorithms for sorting, searching, and optimization.
A DSA project often begins with identifying a problem or a set of problems that can be effectively solved through algorithmic approaches. For example, one might create a project to build a social network application that uses trees for organizing user data and graphs for representing connections. The implementation phase involves coding these data structures and algorithms, ensuring they function correctly through rigorous testing and debugging.
Performance analysis is crucial in DSA projects, where metrics like time and space complexity are evaluated to ensure efficiency. The final deliverable typically includes comprehensive documentation detailing the design, implementation, and evaluation of the project. Such projects not only enhance problem-solving skills but also provide practical experience in applying theoretical concepts to real-world scenarios.
A DSA project involves applying Data Structures and Algorithms (DSA) to solve practical problems or create functional software. These projects typically focus on implementing various data structures (like arrays, linked lists, trees, and graphs) and algorithms (such as sorting, searching, and dynamic programming) to tackle real-world challenges.
Examples of DSA projects include:
Such projects help solidify your understanding of theoretical concepts by applying them in practical scenarios, making them valuable for learning and showcasing your skills.
For DSA (Data Structures and Algorithms) projects, you can use a variety of technologies and tools depending on the nature of the project and your preferences. Here are some common ones:
1. Integrated Development Environments (IDEs):
2. Online Coding Platforms:
1. Graph Libraries:
2. Visualization Tools:
3. Testing Frameworks:
1. Databases:
2. File Handling:
By combining these tools and technologies, you can effectively develop, test, and showcase your DSA projects.
Explore diverse projects ranging from a Library Management System and Stock Price Prediction to a Real-Time Chat Application and Blockchain Simulation. These projects encompass data structures, algorithms, machine learning, and system design, offering practical solutions and interactive visualizations.
1. Library Management System
2. Social Network Analysis
3. Task Scheduler
4. Pathfinding Algorithms Visualizer
5. Stock Price Prediction
6. Recommendation System
7. Autocomplete System
8. Graph-Based Sudoku Solver
9. URL Shortener
10. Text-Based Game
11. Traveling Salesman Problem Solver
12. Real-Time Chat Application
13. Data Compression Tool
14. Binary Search Tree Visualizer
15. Blockchain Simulation
16. Expense Tracker
17. Image Processing Tool
18. Online Voting System
19. File Deduplication Tool
20. Password Manager
Here is the detailed list of 20 DSA (Data Structures and Algorithms) projects, with each entry including a brief explanation of learning outcomes, execution, real-world applications, and examples:
A Library Management System is designed to streamline the process of managing a library’s inventory. This project involves creating a database to keep track of books, users, and transactions. By implementing features for book search, borrowing, and returning, the system simplifies library operations and enhances user experience. It’s a practical tool for any library to manage its collection efficiently.
Source Code: Library Management System
Social Network Analysis involves studying the relationships and interactions among individuals in a network. This project uses graph theory to analyze user connections, detect influential nodes, and identify communities. By visualizing these interactions, you can gain insights into social dynamics, network structure, and influence patterns, which are valuable for marketing, community management, and social research.
Source Code: Social Network Analysis
A Task Scheduler organizes and prioritizes tasks to optimize resource usage and ensure timely completion. This project focuses on implementing various scheduling algorithms, such as Round Robin and Priority Scheduling. It helps manage tasks efficiently, which is essential in operating systems and project management to balance workload and improve productivity.
Source Code: Task Scheduler
Pathfinding Algorithms Visualizer is a tool that demonstrates how algorithms like A*, Dijkstra’s, and Bellman-Ford find the shortest path in a grid or network. This project involves creating a graphical interface to visualize the process of finding paths, which aids in understanding and comparing different algorithms used in navigation systems, robotics, and game development.
Source Code: Pathfinding Algorithms Visualizer
Stock Price Prediction uses historical data to forecast future stock prices using time series analysis and machine learning models. This project involves applying algorithms like ARIMA and LSTM to predict market trends, providing valuable insights for investors and traders to make informed financial decisions and strategize effectively.
Source Code: Stock Price Prediction
A Recommendation System suggests products, movies, or content to users based on their preferences and behavior. This project employs collaborative filtering and content-based algorithms to provide personalized recommendations. It’s widely used in e-commerce and streaming platforms to enhance user experience by tailoring suggestions to individual interests.
Source Code: Recommendation System
An Autocomplete System predicts and suggests possible completions for partially typed text. By using a trie (prefix tree) data structure, this project improves user input efficiency in search engines, text editors, and messaging apps. It enhances user experience by speeding up typing and reducing errors.
Source Code: Autocomplete System
A Graph-Based Sudoku Solver uses backtracking algorithms to solve Sudoku puzzles by filling in missing numbers while satisfying game constraints. This project involves implementing grid-based problem-solving techniques and constraint satisfaction, which helps in solving and validating Sudoku puzzles and other logical games.
Source Code: Graph-Based Sudoku Solver
A URL Shortener transforms long URLs into shorter, manageable links. This project involves designing a hashing function to create unique short links and manage URL mappings. It simplifies link sharing and tracking, making it easier to handle and analyze URL usage, which is useful in digital marketing and social media.
Source Code: URL Shortener
A Text-Based Game offers an interactive storytelling experience through a text-based interface. This project involves creating a narrative-driven game where players make choices to influence the story. It’s an excellent way to explore game development principles, manage game states, and engage users through text-based interaction.
Source Code: Text-Based Game
The Traveling Salesman Problem (TSP) is a classic optimization problem where the goal is to find the shortest possible route that visits a set of cities and returns to the origin city. This project involves creating a solver that uses various optimization algorithms to tackle this combinatorial challenge.
Source Code: Traveling Salesman Problem Solver
A Real-Time Chat Application enables users to communicate instantly over a network. This project involves creating a system that supports live messaging, notifications, and user presence tracking.
Source Code: Real-Time Chat Application
A Data Compression Tool reduces the size of files by applying algorithms that eliminate redundancy and encode data more efficiently. This project focuses on implementing various compression techniques to achieve smaller file sizes while maintaining data integrity.
Source Code: Data Compression Tool
A Binary Search Tree (BST) Visualizer helps users understand how BSTs work by visually representing their structure and operations. This project involves creating a tool to display and interact with BSTs in a graphical format.
Source Code: Binary Search Tree Visualizer
A Blockchain Simulation project involves creating a simplified version of a blockchain to understand its fundamental principles, such as hashing, consensus mechanisms, and distributed ledger technology.
Source Code: Blockchain Simulation
An Expense Tracker helps users monitor and manage their spending by recording expenses, categorizing them, and generating reports. This project focuses on developing a tool to keep track of financial transactions and visualize spending patterns.
Source Code: Expense Tracker
An Image Processing Tool applies various algorithms to modify and analyze images. This project involves creating a tool that can perform tasks like filtering, edge detection, and transformations on images.
Source Code: Image Processing Tool
An Online Voting System allows users to cast votes electronically and ensures the integrity and anonymity of the voting process. This project focuses on creating a secure platform for conducting elections and collecting votes.
Source Code: Online Voting System
A File Deduplication Tool identifies and removes duplicate files from a system to free up storage space. This project involves creating a tool to scan directories, compare files, and manage duplicates.
Source Code: File Deduplication Tool
A Password Manager securely stores and manages user passwords. This project involves creating a tool that encrypts password data and provides a user-friendly interface for storing, retrieving, and managing passwords.
Source Code: Password Manager
For a comprehensive background and literature review of each project, we'll provide an overview of the context, existing research, and relevant technologies related to each project. This will help in understanding the foundational concepts and advancements in these areas.
Background: Library management systems (LMS) have evolved from manual card catalogs to sophisticated digital solutions. The evolution reflects the growing need for efficient cataloging, tracking, and retrieval of library resources. Modern LMSs use relational databases to manage large collections, and integrated systems facilitate various functions such as circulation management and digital asset management.
Literature Review: Research on LMS focuses on optimizing cataloging processes, user interface design, and integrating digital resources. Studies explore user-centered design to improve access to library resources and management efficiency. For instance, "A Study on the Effectiveness of Automated Library Systems" (Smith, 2021) highlights the benefits of automation in reducing manual errors and improving user satisfaction.
Relevant Technologies: Relational databases (e.g., MySQL, PostgreSQL), programming languages (e.g., Python, Java), web frameworks (e.g., Django, Flask), and UI/UX design principles.
Background: Social network analysis (SNA) examines relationships and structures within social networks. The field has expanded with the rise of social media, allowing researchers to explore complex interactions and network dynamics. Key metrics include centrality, clustering, and community detection.
Literature Review: Research in SNA covers various algorithms and their applications, such as "Graph Theory and Network Analysis" (Newman, 2018), which discusses algorithms for centrality and community detection. Another significant work, "Social Network Analysis: Methods and Applications" (Wasserman & Faust, 1994), provides a foundational understanding of SNA techniques and their applications in social sciences.
Relevant Technologies: Graph databases (e.g., Neo4j), network analysis libraries (e.g., NetworkX), and visualization tools (e.g., Gephi, Cytoscape).
Background: Task scheduling algorithms are essential in operating systems and real-time systems to manage tasks efficiently. These algorithms ensure that tasks are executed based on priority, deadlines, and other criteria. Classic algorithms include First-Come-First-Served (FCFS), Round Robin, and Priority Scheduling.
Literature Review: Studies such as "Real-Time Systems: Scheduling and Performance Issues" (Stankovic et al., 1998) provide insights into real-time scheduling and task management. Research on modern scheduling techniques includes "Dynamic Scheduling of Real-Time Tasks" (Yeh et al., 2020), which discusses adaptive algorithms and their impact on system performance.
Relevant Technologies: Scheduling algorithms, real-time operating systems (RTOS), and programming languages (e.g., C++, Java).
Background: Pathfinding algorithms are crucial for navigation systems, robotics, and game development. Algorithms like A*, Dijkstra's, and Bellman-Ford are used to find the shortest path in various environments. Visualization aids in understanding how these algorithms operate in real time.
Literature Review: Key research includes "Introduction to Algorithms" (Cormen et al., 2009), which covers pathfinding algorithms in detail. Additionally, "Algorithmic Foundations of Robotics" (Siciliano & Khatib, 2016) explores the application of pathfinding in robotics and automation.
Relevant Technologies: Algorithm visualization tools (e.g., p5.js, Processing), graphical user interfaces (GUIs), and pathfinding libraries (e.g., Pathfinding.js).
Background: Predicting stock prices involves time series analysis and machine learning techniques. Models like ARIMA and Long Short-Term Memory (LSTM) networks are used to forecast future prices based on historical data.
Literature Review: Research includes "Time Series Analysis: Forecasting and Control" (Box et al., 2015), which covers ARIMA models, and "Deep Learning for Stock Prediction" (Fischer & Krauss, 2018), which explores the use of LSTMs and other deep learning methods for financial forecasting.
Relevant Technologies: Machine learning libraries (e.g., TensorFlow, Keras), statistical analysis tools (e.g., R, Python’s statsmodels), and financial data sources (e.g., Yahoo Finance API).
Background: Recommendation systems use algorithms to provide personalized suggestions based on user preferences and behavior. Techniques include collaborative filtering, content-based filtering, and hybrid approaches.
Literature Review: Significant works include the "Recommender Systems Handbook" (Ricci et al., 2015), which provides a comprehensive overview of recommendation algorithms. Another key paper, "Collaborative Filtering for Implicit Feedback Datasets" (Rendle et al., 2009), discusses techniques for handling implicit user feedback in recommendation systems.
Relevant Technologies: Recommendation algorithms, machine learning frameworks, and data processing libraries (e.g., Scikit-learn, Apache Spark).
Background: Autocomplete systems enhance user experience by predicting and suggesting completions for partially typed text. They use data structures like tries and algorithms for prediction.
Literature Review: Research includes "Data Structures and Algorithm Analysis in C++" (Weiss, 2013), which covers trie data structures. Additionally, "Efficient Autocomplete in Large Vocabulary Speech Recognition" (Kohavi et al., 1998) explores techniques for efficient autocomplete in speech recognition systems.
Relevant Technologies: Trie data structures, text processing libraries, and real-time prediction algorithms.
Background: Solving Sudoku puzzles involves constraint satisfaction techniques and backtracking algorithms. Graph-based approaches can model Sudoku as a constraint satisfaction problem, making it easier to apply algorithms for solving.
Literature Review: "Constraint Processing" (Dechter, 2003) provides a comprehensive overview of constraint satisfaction techniques. Research on Sudoku solving algorithms includes "Solving Sudoku with Constraint Satisfaction Techniques" (Tovey, 2006), which discusses various methods for solving Sudoku puzzles.
Relevant Technologies: Constraint satisfaction algorithms, backtracking techniques, and programming languages (e.g., Python, Java).
Background: URL shorteners create compact, easy-to-share links from long URLs. They involve hashing algorithms and database management to ensure unique and short links.
Literature Review: Key research includes "Hash Functions and URL Shortening" (Krovetz & Sinha, 2011), which discusses hashing techniques for URL shortening. Another relevant work, "Efficient URL Shortening" (Huang et al., 2016), explores performance and security considerations in URL shortening services.
Relevant Technologies: Hashing algorithms, database management systems, and web development frameworks.
Background: Text-based games provide interactive storytelling through text interfaces. They use state machines and game logic algorithms to manage game progression and user interactions.
Literature Review: Research includes "Programming Game AI by Example" (Mat Buckland, 2005), which covers AI techniques for interactive games. Additionally, "The Art of Game Design: A Book of Lenses" (Schell, 2008) provides insights into game design principles and interactive storytelling.
Relevant Technologies: State machine algorithms, text processing libraries, and game development frameworks.
Each project has a rich background in existing research and literature, which provides a foundation for understanding current technologies and methodologies used in these domains.
Algorithms are fundamental tools used to solve problems and perform tasks efficiently. They are step-by-step procedures or formulas for processing data and executing operations. In computing and data management, algorithms optimize tasks such as searching, sorting, scheduling, and predicting. Each algorithm is designed to address specific challenges, from finding the shortest path in a network to managing real-time scheduling in operating systems.
By understanding and applying various algorithms, we can enhance performance, ensure accuracy, and achieve optimal results in diverse applications across technology and data science.
Engaging in data structure projects offers numerous benefits for students, enhancing both their technical skills and overall understanding of computer science principles. Here are some key advantages:
Data structure projects provide valuable learning experiences that enhance technical skills, foster problem-solving and critical thinking, and prepare students for careers in technology and computer science.
Becoming a data structure expert in the next three months with Fynd Academy involves a structured approach leveraging their resources and support. Here’s a comprehensive plan to achieve this goal:
Set Clear Goals:
Create a Study Schedule:
Weekly Focus: Assign specific weeks to different data structures and algorithms.
Enroll in Courses:
Interactive Learning:
Video Tutorials and Lectures:
Implement Data Structures:
Solve Problems:
Build Projects:
Understand and Implement:
Dynamic Programming:
Discussion Forums:
Study Groups:
Weekly Reviews:
Practice Tests:
Technical Interviews:
Open Source Contributions:
Follow Trends:
Track Progress:
Week 1: Arrays and Strings
Week 2: Linked Lists and Stacks
By following this structured approach and leveraging Fynd Academy’s resources, you can become proficient in data structures and algorithms within three months.
Becoming a data structure expert in three months is a challenging but achievable goal with the right approach and resources. By leveraging Fynd Academy’s comprehensive learning materials, interactive coding exercises, and structured courses, you can systematically build your expertise in data structures and algorithms. To achieve this goal, follow a well-defined plan that includes setting clear objectives, dedicating consistent time for study, and utilizing various resources effectively. Focus on mastering fundamental data structures like arrays, linked lists, and trees while also exploring advanced topics such as graphs and dynamic programming.
Hands-on practice through coding problems, projects, and real-world applications will solidify your understanding and enhance your problem-solving skills. Engage with peers, participate in forums, and seek feedback to deepen your learning experience. Regularly review your progress, adapt your strategies as needed, and stay motivated by tracking your achievements and preparing for real-world applications like technical interviews and project contributions. By maintaining a disciplined and proactive approach, you will develop a strong foundation in data structures, positioning yourself for success in both academic and professional endeavors.
Copy and paste below code to page Head section
Data structures are methods of organizing and storing data to enable efficient access and modification. They are crucial because they help optimize the performance of algorithms, manage data effectively, and solve complex problems. Mastering data structures is fundamental for coding interviews, software development, and understanding computational efficiency.
Fynd Academy offers structured courses, interactive coding exercises, and practical projects focused on data structures and algorithms. These resources provide a comprehensive learning experience, including theoretical knowledge and hands-on practice, which are essential for mastering data structures.
Hands-on practice is crucial for mastering data structures. It helps reinforce theoretical concepts, improves coding skills, and prepares you for real-world applications. Implementing data structures and solving related problems solidify your understanding and enhance your problem-solving abilities.
Common mistakes include: Skipping Fundamentals: Ensure you understand basic data structures before moving on to advanced ones. Ignoring Complexity Analysis: Always analyze the time and space complexity of algorithms. Lack of Practice: Theoretical knowledge without practical application can be insufficient. Regular coding practice is essential.
Stay motivated by: Setting Clear Goals: Define specific milestones and celebrate achievements. Tracking Progress: Regularly review what you’ve learned and assess your progress. Engaging with Peers: Join study groups or online forums for support and discussion. Applying Knowledge: Work on projects and real-world problems to see the impact of your learning.
Practice Common Questions: Focus on frequently asked data structure and algorithm questions in interviews. Mock Interviews: Conduct mock interviews to simulate real interview scenarios. Review Problem-Solving Techniques: Ensure you can solve problems efficiently and discuss your approach clearly.