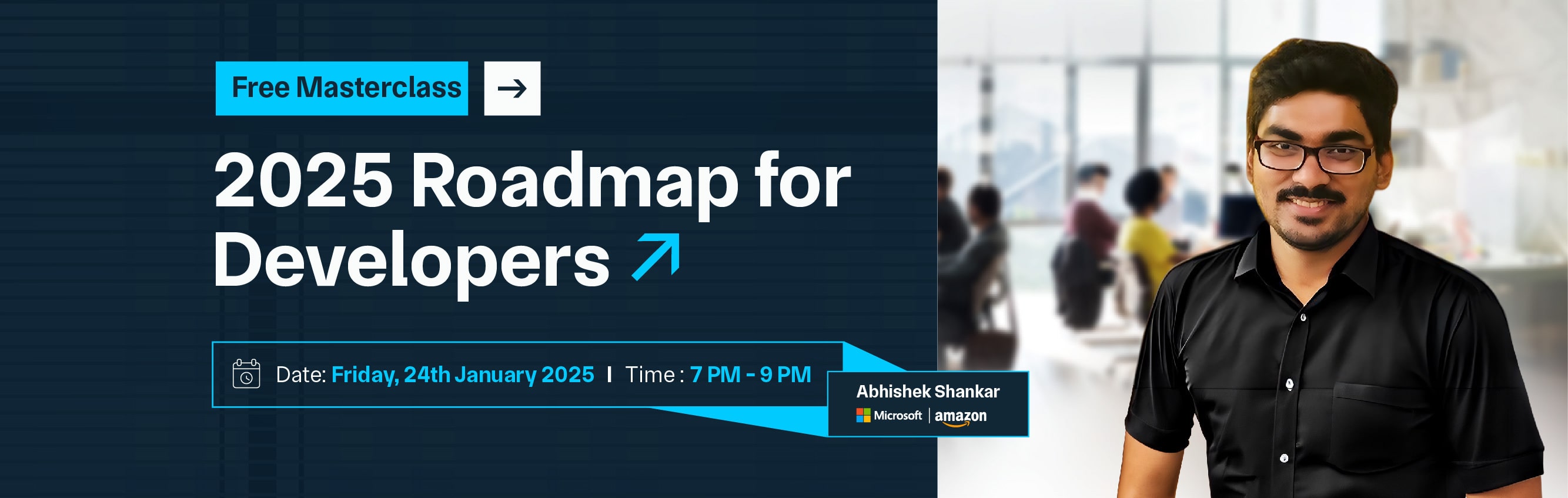

Docker image commands are essential for managing and optimizing containerized applications. These commands allow developers to interact with Docker images, which serve as blueprints for containers. Some of the most commonly used Docker image commands include docker pull, which downloads an image from a registry like Docker Hub, and docker build, which allows you to create custom images from a Dockerfile.
Once images are on your local machine, the docker images command lets you list them, while docker rmi is used to remove unused or unnecessary images to free up disk space. For those managing multiple versions of an image, docker tag helps in labeling images with unique tags, enabling efficient version control. Advanced commands like docker push allow you to upload your images to a Docker registry, making them accessible to others or for deployment in production.
docker save and docker load are used for exporting and importing images, which is helpful when transferring images between systems or archiving them. Additionally, docker inspect offers detailed metadata about an image, which can be useful for troubleshooting or optimization. By mastering these Docker image commands, developers can streamline their workflows, improve containerization practices, and ensure better application performance.
A Docker image is a lightweight, standalone, and executable package that contains everything needed to run a piece of software. It includes the application code, libraries, dependencies, environment variables, configuration files, and the operating system runtime. A Docker image is a template used to create containers which are running instances of these images.
Docker images are built in layers, where each layer represents an instruction in the Dockerfile, a script that defines how the image is constructed. These layers are cached, which helps in speeding up the build process and ensures efficiency. Docker images are immutable, meaning once they are created, they cannot be modified. If you need to make changes, you must build a new image. Docker images are typically stored in registries such as Docker Hub or private registries.
Once an image is pulled from a registry, it can be used to create containers, which are isolated environments where applications run. Because Docker images are portable, developers can be confident that an image will run the same way on any system, whether it's their local machine or a production server. In short, a Docker image is the blueprint for creating containers, making it a fundamental component of Docker's containerization platform.
Here is a list of essential Docker image commands used for managing and interacting with Docker images:
These commands form the core of Docker's image management, allowing you to build, pull, push, inspect, and manage Docker images efficiently.
Here are some common Docker image commands with practical examples:
Description: Downloads a Docker image from a registry (e.g., Docker Hub).
Example: Pull the latest version of the ubuntu image.
docker pull ubuntu:latest
Description: Builds a Docker image from a Docker file.
Example: Build an image from a Dockerfile in the current directory and tag it as myapp:v1.
docker build -t myapp:v1 .
Description: Lists all Docker images available on your local machine.
Example: List all images.
docker images
Description: Removes a Docker image from the local machine.
Example: Remove the myapp:v1 image.
docker rmi myapp:v1
Description: Tags an existing image with a new tag or version.
Example: Tag the ubuntu:latest image as myubuntu:v1.
docker tag ubuntu:latest myubuntu:v1
Description: Displays the history of an image, showing its layers.
Example: View the history of the ubuntu image.
docker history ubuntu:latest
Description: Saves a Docker image to a tarball file for transport or archiving.
Example: Save the myapp:v1 image to a file called myapp.tar.
docker save -o myapp.tar myapp:v1
Description: Loads a Docker image from a tarball file.
Example: Load the image from the myapp.tar file.
docker load -i myapp.tar
Description: Displays detailed information about an image, including its configuration, layers, and metadata.
Example: Inspect the ubuntu:latest image.
docker inspect ubuntu:latest
Description: Pushes a Docker image to a registry (e.g., Docker Hub).
Example: Push the myapp:v1 image to Docker Hub.
docker push myapp:v1
Description: Inspects and manipulates Docker image manifests, particularly for multi-platform images.
Example: Inspect the manifest of the ubuntu:latest image.
docker manifest inspect ubuntu:latest
These examples show how to perform key Docker image tasks such as pulling, building, tagging, and inspecting images. Each command allows you to manage your containerized applications efficiently.
Below are some advanced Docker image commands that provide more control over image building, management, and distribution.
Description: Allows the use of Docker BuildKit, enabling advanced features like multi-platform builds and improved caching.
Example: Build an image for multiple platforms (e.g., linux/amd64 and linux/arm64).
docker buildx build --platform linux/amd64,linux/arm64 -t myapp:multiarch.
Explanation: This command enables building images for different architectures from a single Dockerfile.
Description: Pushes a Docker image to a registry. When using multiple tags or pushing to private registries, this becomes essential.
Example: Push an image to a private registry.
docker push myregistry.com/myapp:v1
Example: Push multiple tags for the same image.
docker tag myapp:v1 myapp:latest
docker push myapp:latest
docker push myapp:v1
Description: Forces Docker not to use any cache when building an image. This ensures that every layer is rebuilt from scratch.
Example: Build the image without cache.
docker build --no-cache -t myapp:v2 .
Description: Passes build-time variables to the Dockerfile during the image build process.
Example: Use a build argument to specify the version of an application during the build.
docker build --build-arg VERSION=1.2.3 -t myapp:v1 .
Description: Re-tag images, enabling version control and facilitating image deployment to different environments or repositories.
Example: Tag an image with a version and also add the latest tag for production.
docker tag myapp:v1 myapp:v1.0
docker tag myapp:v1 myapp:latest
Description: Works with multi-platform images. Allows inspecting and managing image manifests to ensure compatibility across different platforms.
Example: Inspect a multi-platform image manifest.
docker manifest inspect myapp:multiarch
Description: Pushes an image to the Docker registry without verifying its content trust. This is useful for automated workflows.
Example: Disable content trust during the push.
DOCKER_CONTENT_TRUST=0 docker push myapp:v1
Description: Removes unused Docker images from your local system to free up disk space. This command is particularly useful for cleaning up images after running multiple builds.
Example: Remove dangling (unused) images.
docker image prune
Example: Remove all unused images, not just dangling ones.
docker image prune -a
Description: Export the contents of a container to a tarball file and import it as a new image. This is useful for creating images from running containers.
Example: Export a container’s filesystem to a tar file.
docker export <container-id> -o myapp-container.tar
Example: Import the exported tar file as a new Docker image.
docker import myapp-container.tar myapp:exported
Description: Squashes the layers of an image into a single layer, which helps in reducing the size of the final image.
Example: Build and squash layers into one.
docker build --squash -t myapp:optimized.
Docker images are powerful tools for creating, deploying, and managing applications in isolated environments. Here are some common and advanced use cases for Docker images across various stages of the software development lifecycle.
Docker images are an excellent way to create consistent and reproducible environments for software development. By encapsulating the entire runtime environment, including the operating system, libraries, and dependencies, Docker ensures that an application runs consistently across different machines.
Developers can define the environment through a Dockerfile, which can be version-controlled, making it easier to share with teams and deploy on various systems. This eliminates the common "it works on my machine" problem, where an app behaves differently on developers' machines due to environmental inconsistencies.
Docker images provide an isolated environment for running applications, ensuring that they do not interfere with other applications or system processes. This isolation helps prevent dependency conflicts, ensuring that each application has the specific versions of libraries it needs.
When a Docker image is deployed, it runs in a container that is isolated from the host machine. This makes it easier to deploy applications across different servers or cloud environments without worrying about compatibility or configuration issues.
Docker images support multi-platform deployments, which means developers can create images that run on different operating systems (Linux, Windows, macOS) and architectures (x86, ARM). This feature is particularly useful for companies with a diverse infrastructure, enabling them to use the same codebase across different platforms.
By leveraging tools like Docker Buildx, developers can build images for multiple platforms simultaneously, which is ideal for applications that need to be deployed on various environments, such as IoT devices, cloud servers, and desktop machines.
Docker images are a cornerstone of microservices architecture. Each microservice, which is typically a small, independent application with a specific function, can be packaged into its own Docker image. This allows microservices to be deployed, updated and scaled independently of one another. Docker makes managing multiple services easier by encapsulating them in isolated containers.
This approach also enhances fault tolerance, as issues in one microservice don’t affect the others. Tools like Docker Compose can orchestrate multiple microservices, making it easier to develop, test, and deploy complex applications.
Docker images play a crucial role in Continuous Integration and Continuous Deployment (CI/CD) pipelines. In CI/CD, Docker ensures that an application runs the same way in testing and production environments by packaging the app and all of its dependencies into a container.
As a result, automated pipelines can build, test, and deploy the application consistently without the risk of errors due to environmental differences. By using Docker images in CI/CD workflows, teams can streamline their processes, ensuring quicker and more reliable releases while maintaining the integrity of the application.
With Docker images, you can easily manage different versions of an application. Each Docker image can be tagged with a version number or a label (like v1, latest, or stable). This allows teams to quickly roll back to a previous version if a new one has issues.
By simply running an older image tag, developers can ensure the application functions as expected while the issue is being fixed. This versioning capability makes Docker a powerful tool for maintaining a stable production environment and for ensuring that the most recent features and updates are properly tested before being deployed.
Docker images make testing and development more efficient by providing isolated environments for each developer. This ensures that the application runs in a clean environment that mirrors the production setup, regardless of the developer's local setup.
Developers can create containers from images to test different versions of their applications, run integration tests, or experiment with new features. Docker also makes it easy to spin up temporary environments for testing, and once testing is done, containers can be destroyed, leaving no traces behind.
Docker images are highly effective for managing application dependencies and system configurations. Instead of relying on system-wide installations of libraries and tools, which may conflict with other applications, Docker images bundle all dependencies into the image itself.
This ensures that the application has everything it needs to run, regardless of the underlying system configuration. Moreover, Docker allows you to specify environment variables, mount volumes, and configure networking, making it easier to manage complex application configurations that can change depending on the environment.
In edge computing and IoT (Internet of Things), Docker images provide an efficient way to deploy and manage lightweight applications on resource-constrained devices. Docker’s portability allows developers to package applications with their dependencies and deploy them directly on IoT devices, ensuring they run the same way as in the cloud or data centers.
This approach simplifies managing and updating applications on large networks of devices while keeping them isolated and secure. Docker's small footprint also makes it ideal for running applications on devices with limited computational resources.
Docker images are also used to configure applications for different environments like production, development, or staging. Environment variables, set through Docker’s configuration options, allow you to easily adapt your application for specific environments without modifying the code.
This is particularly useful for setting things like database connections, API keys, or feature flags, which may change depending on whether you're deploying to a local machine or a production server. Docker simplifies environment configuration, ensuring that the app behaves consistently across all stages of deployment.
Understanding the anatomy of a Docker image command is essential for effectively working with Docker in various scenarios. Each Docker command follows a specific structure, with options, flags, and arguments that tell Docker what action to perform and how. Let's break down the components of a typical Docker image command to understand its structure and usage.
The core part of the Docker image command that defines the action you want to perform. The command specifies what Docker should do, such as build, pull, push, or remove an image.
Examples:
docker build
docker pull
docker push
docker rmi
Example Command:
docker pull ubuntu:latest
Command: docker pull specifies that we want to download an image from a registry.
The name of the Docker image on which the command is being executed. This name can be a predefined image from Docker Hub (or another registry) or a custom image you've built. It often includes a tag, like ubuntu:latest, where ubuntu is the name of the image, and latest is the tag.
Format: [repository/] image[:tag]
Example Command:
docker pull ubuntu:18.04
Image Name: ubuntu:18.04 refers to the specific version of the Ubuntu image, where 18.04 is the tag.
Flags and options are additional arguments that modify the behavior of the Docker image command. These can include settings for logging, memory limits, ports, or any specific configuration necessary for the command.
Format: Flags usually start with a double dash -- (for long options) or a single dash - (for short options).
Example Flags:
docker build -t myapp:v1 --no-cache.
Flag: -t myapp:v1 tags the image with the name myapp and version v1. The --no-cache flag ensures that the build doesn't use any cached layers.
Arguments are additional parameters that are passed to Docker commands. These may include paths (e.g., for build contexts), environment variables, or other configurations that influence the command's execution.
Example:
Example Command:
docker build -t myapp:v1 /path/to/Dockerfile
Subcommands are additional layers of commands used to perform more granular actions. Some Docker commands, such as docker build or docker volume, may include subcommands that further define the scope or behavior of the operation.
Example Subcommand: docker buildx for advanced build capabilities or docker image for working specifically with Docker images (e.g., docker image ls, docker image prune).
Example Command:
docker image prune -a
A Docker Image Repository is a place where Docker images are stored and made available for distribution. It acts as a central location for organizing and sharing Docker images, making it easier to pull and push images to and from various systems.
Docker repositories allow teams and organizations to maintain versioned images that can be used across different environments, from development to production. There are different types of Docker repositories, and they play a crucial role in ensuring the efficient distribution and management of Docker images.
Command to pull from a public repository:
docker pull nginx
Command to push to a private repository:
docker push myregistry.com/myapp:v1
Command to pull from Docker Hub:
docker pull ubuntu:20.04
Command to push to a DTR:
docker push mycompany.docker.com/myapp:latest
These include:
Command to push to an Amazon ECR repository:
docker push <aws_account_id>.dkr.ecr.<region>.amazonaws.com/myapp:v1
Command to tag an image:
docker tag myapp:latest myregistry.com/myapp:v2
Command to search Docker Hub:
docker search redis
Creating a Docker image involves packaging an application and its dependencies into a containerized environment that can run consistently across different systems. This process allows you to encapsulate everything needed for your application to run, including the operating system, libraries, configurations, and the application itself. Here's a step-by-step guide on how to create a Docker image.
Before you can create Docker images, you must have Docker installed on your machine. Docker provides installation guides for different operating systems (Linux, macOS, and Windows) on its official website. Ensure Docker is running by checking the Docker version:
docker --version
The heart of creating a Docker image is writing a Dockerfile, a simple text file that contains instructions for building the image. It defines everything from the base image to the application code, dependencies, and configuration files. The Dockerfile outlines the steps Docker should take to build the image.
Steps to create a Dockerfile:
1. Create a Directory: Start by creating a directory for your Docker image project.
mkdir myapp
cd myapp
2. Create the Dockerfile: Inside the project directory, create a file named Dockerfile (without any extension).
touch Dockerfile
3. Write Dockerfile Instructions: Edit the Dockerfile using any text editor. A basic example of a Dockerfile might look like this:
# Use an official base image
FROM ubuntu:20.04
# Install dependencies
RUN apt-get update && apt-get install -y python3 python3-pip
# Set the working directory inside the container
WORKDIR /app
# Copy application code to the container
COPY . /app
# Install Python dependencies
RUN pip3 install -r requirements.txt
# Expose port for communication
EXPOSE 5000
# Set the default command to run when the container starts
CMD ["python3", "app.py"]
Explanation of the Dockerfile:
Once the Dockerfile is ready, you can build the Docker image using the docker build command. You will need to specify the path to the directory containing the Dockerfile. If the Dockerfile is in the current directory, use. (dot) as the build context.
Command to build the Docker image:
docker build -t myapp:v1 .
Docker will execute the instructions in the Dockerfile step by step, downloading the base image, installing dependencies, copying files, and setting up the environment as specified.
After building the image, you can verify it using the docker images command, which will list all Docker images stored on your local machine.
docker images
This will display a list of images, showing the repository, tag, image ID, and other details, including the newly created image (myapp:v1).
After the image has been created, you can run it as a container using the docker run command.
Command to run the image:
docker run -d -p 5000:5000 myapp:v1
This will start the container, and the application inside will be accessible via port 5000.
If you want to share your Docker image with others or deploy it on another system, you can push it to a Docker image repository, such as Docker Hub or a private registry.
Steps to push the image to Docker Hub:
1. Log in to Docker Hub:
docker login
2. Tag the image: If your image is not already tagged with your Docker Hub username, you can tag it as follows:
3. Push the image:
docker push yourusername/myapp:v1
After pushing, your image will be available in your Docker Hub repository, where you can pull it from any machine using docker pull.
If you need to update or change the Docker image (for example, by modifying the application code or dependencies), you can make changes, update the Dockerfile, and rebuild the image.
docker build -t myapp:v2 .
This will create a new version (v2) of the image. You can then push the updated image to the repository, as shown in the previous step.
A Dockerfile is a text file that contains a set of instructions used by Docker to automate the process of building a Docker image. It is a blueprint for creating Docker images, specifying everything required for an application to run within a container.
Dockerfiles define the operating system, libraries, application code, environment variables, dependencies, and configuration files needed for a containerized application. By writing a Dockerfile, you can describe the steps necessary to set up your environment in a repeatable and predictable manner, which ensures that the application will run consistently across different environments (development, staging, production, etc.).
Docker image commands are essential tools for building, managing, and distributing containerized applications. From creating custom images using the docker build command to pulling pre-built images from repositories with docker pull, Docker provides a flexible and streamlined approach to containerization. Commands like docker images, docker tag, and docker push help you manage images, handle versioning, and share your applications across environments.
By mastering Docker image commands, developers can ensure that their applications are portable, consistent, and easily deployable across different systems. Whether you're working with public repositories like Docker Hub or using private registries, Docker images play a crucial role in modern application development and DevOps practices. Furthermore, with advanced commands such as docker history and docker save, you gain deeper control over image layers, caching, and storage, enhancing your ability to optimize and troubleshoot containerized applications.
Copy and paste below code to page Head section
A Docker image is a lightweight, standalone, and executable package that includes everything needed to run a piece of software—such as code, runtime, libraries, environment variables, and configuration files. Docker images are the building blocks of Docker containers and provide a consistent environment for your applications to run, ensuring portability across different systems and environments.
To create a Docker image, you need to write a Dockerfile that contains instructions for building the image. After creating the Dockerfile, you use the docker build command to generate the image. Here's the basic command: docker build -t <image_name>:<tag> .
docker build: This command is used to build a Docker image from a Dockerfile. It creates a new image based on the instructions in the Dockerfile. docker run: This command runs a container from an existing Docker image. It launches the image in a containerized environment.
You can list all the Docker images stored locally on your machine using the docker images or docker image ls command: docker images
A Docker repository is a collection of Docker images stored in a central location, making them accessible for sharing and distribution. Repositories can be public (like Docker Hub) or private (like AWS ECR, Google Container Registry, or custom solutions).
The docker history command shows the history of an image, including the layers that make up the image and the commands used to build it. This helps track changes to an image and understand how it's constructed: docker history <image_name>:<tag>