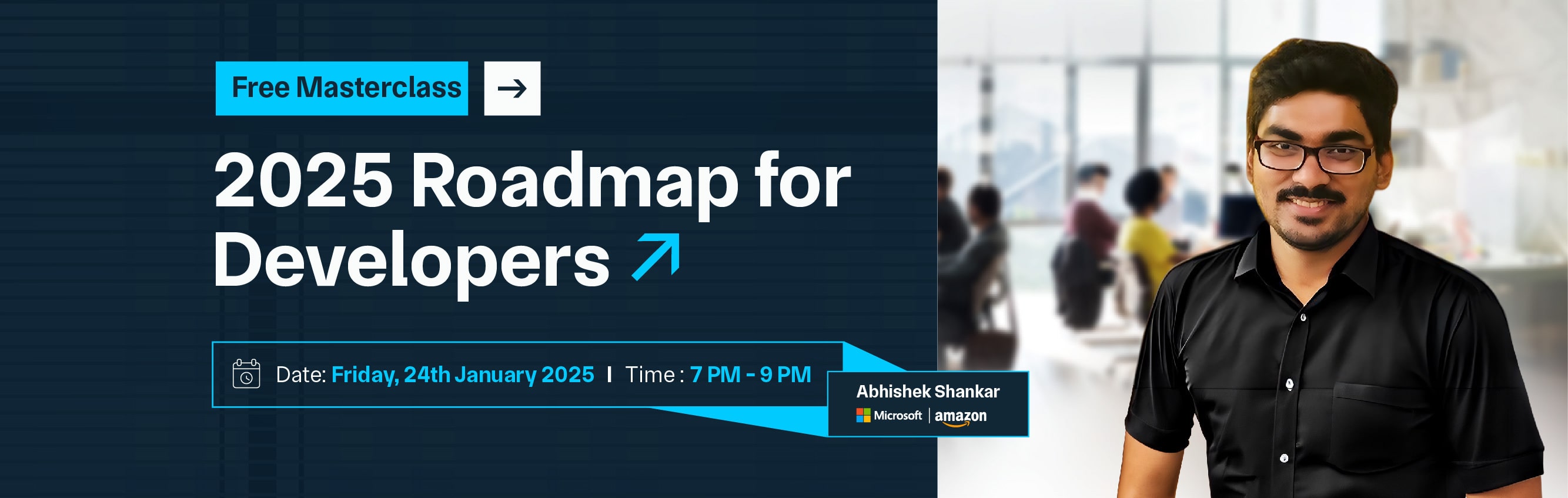

Pandas and NumPy are both powerful libraries in Python used for data analysis, but they serve different purposes and have distinct functionalities. NumPy is primarily focused on numerical operations and provides support for large, multi-dimensional arrays and matrices. It offers a wide range of mathematical functions to perform operations on these arrays, such as addition, subtraction, and element-wise manipulation. NumPy arrays are faster and more efficient than Python lists for large datasets due to their fixed size and homogeneity of data types.
On the other hand, Pandas is built on top of NumPy and provides data structures like Series (1D) and DataFrame (2D) for handling labeled data. It is designed for data manipulation and analysis, offering powerful tools for working with heterogeneous data (different types of columns). Pandas support functionalities like filtering, grouping, merging, and reshaping data, which are more intuitive and user-friendly compared to NumPy.
It is especially useful for time-series data, handling missing values, and performing complex data transformations. While NumPy is more suitable for numerical computations, Pandas excels in data wrangling and preprocessing tasks, making it a go-to library for real-world data analysis tasks that involve diverse data formats.
Pandas is a powerful and versatile open-source data analysis library in Python, widely used for manipulating and analyzing structured data. It provides two key data structures: Series and DataFrame. A Series is a one-dimensional array-like object, similar to a list or a column in a table. At the same time, a DataFrame is a two-dimensional table with rows and columns, similar to a spreadsheet or SQL table.
These structures allow for the efficient handling of heterogeneous data types, such as numbers, strings, or dates. Pandas is designed to simplify the process of data wrangling, which involves cleaning, transforming, and organizing data. It provides an array of functions to handle missing data, merge datasets, filter rows, group data, and perform aggregations.
With its powerful indexing capabilities, you can easily access, slice, and modify data, making it ideal for tasks like exploratory data analysis (EDA), data preprocessing, and time-series analysis. In addition, Pandas integrates well with other libraries such as NumPy, Matplotlib, and Scikit-learn, making it an essential tool for data scientists and analysts. It also supports various file formats like CSV, Excel, SQL, and JSON, enabling easy import and export of data for different applications.
NumPy (Numerical Python) is a fundamental library for scientific computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays efficiently. Unlike Python's built-in lists, NumPy arrays are homogeneous, meaning they contain elements of the same data type, which allows for more efficient memory usage and faster computation.
At the core of NumPy is the ndarray object, which is an n-dimensional array that can hold data of any type but most commonly stores numerical data. NumPy arrays allow for fast element-wise operations, such as addition, multiplication, and mathematical functions (e.g., square root, logarithm, etc.) to be performed on entire arrays without the need for explicit loops.
NumPy also provides tools for performing linear algebra operations, random number generation, Fourier transforms, and statistical analysis. Its vectorized operations, which allow for the use of operations over entire arrays without the need for Python loops, make it an essential tool for handling large datasets and performing high-performance computations in fields like data science, machine learning, and scientific research. Additionally, NumPy integrates seamlessly with other libraries like Pandas and Matplotlib, making it a foundational component of the Python data science ecosystem.
Pandas and NumPy are both essential libraries in Python for data analysis, but they serve different purposes and have distinct features. While NumPy is optimized for numerical operations and handling large, multi-dimensional arrays, Pandas is more suitable for data manipulation, analysis, and handling labeled or heterogeneous data.
Pandas and NumPy are two of the most widely used libraries in Python for data analysis. While both serve essential roles in data science and machine learning workflows, they have different focuses and features.
NumPy is primarily used for numerical computing and handling arrays, whereas Pandas extends NumPy and provides more advanced tools for manipulating and analyzing labeled heterogeneous data. Below is a table comparing their key differences.
NumPy is ideal for numerical operations on homogeneous, multi-dimensional arrays.
import numpy as np
# Create a NumPy array
arr = np.array([1, 2, 3, 4, 5])
# Element-wise operations
arr_squared = arr ** 2
arr_sum = np.sum(arr)
arr_mean = np.mean(arr)
print("Original Array:", arr)
print("Squared Array:", arr_squared)
print("Sum of Array:", arr_sum)
print("Mean of Array:", arr_mean)
Output:
Original Array: [1 2 3 4 5]
Squared Array: [ 1 4 9 16 25]
Sum of Array: 15
Mean of Array: 3.0
Pandas are useful for data manipulation and analysis, especially with labeled or heterogeneous data.
import pandas as pd
# Create a Pandas DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [24, 27, 22, 32],
'City': ['New York', 'Los Angeles', 'Chicago', 'Houston']}
df = pd.DataFrame(data)
# Filter rows where Age > 25
filtered_df = df[df['Age'] > 25]
# Calculate mean age
mean_age = df['Age'].mean()
# Add a new column
df['Age Group'] = ['Young' if age < 30 else 'Adult' for age in df['Age']]
print("Original DataFrame:\n", df)
print("\nFiltered DataFrame (Age > 25):\n", filtered_df)
print("\nMean Age:", mean_age)
Output:
Original DataFrame:
Name Age City Age Group
0 Alice 24 New York Young
1 Bob 27 Los Angeles Young
2 Charlie 22 Chicago Young
3 David 32 Houston Adult
Filtered DataFrame (Age > 25):
Name Age City Age Group
1 Bob 27 Los Angeles Young
3 David 32 Houston Adult
Mean Age: 26.25
Both Pandas and NumPy are powerful tools in Python for data analysis and numerical computations. However, they are designed for different purposes, and knowing when to use each can significantly improve the efficiency and clarity of your code.
NumPy is best suited for numerical computations with homogeneous data, while Pandas is ideal for handling structured data (like tables) with a mix of different data types.
Pandas and NumPy are both powerful libraries in Python used for data analysis, but they have distinct purposes and strengths. NumPy is primarily designed for efficient numerical computations, especially for working with large multi-dimensional arrays and performing element-wise operations.
It operates on homogeneous data, meaning the elements within a NumPy array must be of the same type, usually numbers. This allows for faster and more memory-efficient computations, making it ideal for numerical tasks like matrix operations, linear algebra, and mathematical functions.
Copy and paste below code to page Head section
NumPy is mainly used for numerical computations and working with large, multi-dimensional arrays of homogeneous data (usually numbers). Pandas is built on top of NumPy and provides more advanced tools for handling structured, labeled data (Series and DataFrames), allowing for easier data manipulation, cleaning, and analysis. While NumPy is more efficient for numerical tasks, Pandas is better suited for data preprocessing and analysis tasks.
You should use Pandas when working with labeled data, heterogeneous data types (numbers, strings, dates), or when performing complex data manipulation tasks such as grouping, merging, reshaping, or handling missing values. Use NumPy when you need to perform fast numerical computations or operations on large, homogeneous datasets.
While Pandas is built on top of NumPy and relies on NumPy arrays internally, you can still use Pandas independently. However, for numerical computations, NumPy is more efficient and should be used in conjunction with Pandas for optimized performance.
No, NumPy is generally faster than Pandas for numerical operations due to its optimized design for handling homogeneous numerical data. Pandas offers more flexible data structures but at the cost of slightly slower performance compared to NumPy when it comes to raw numerical computations.
Pandas do not offer the same level of performance and flexibility for matrix operations as NumPy. While you can perform some matrix-like operations using Pandas DataFrames, for advanced numerical tasks like matrix multiplication, eigenvalues, or linear algebra, NumPy is the preferred choice.
Yes, Pandas has robust support for handling missing data, including tools to identify, fill, or drop missing values in datasets. It provides functions like fillna(), dropna(), and others for handling missing data, which makes it particularly suitable for real-world data analysis tasks.