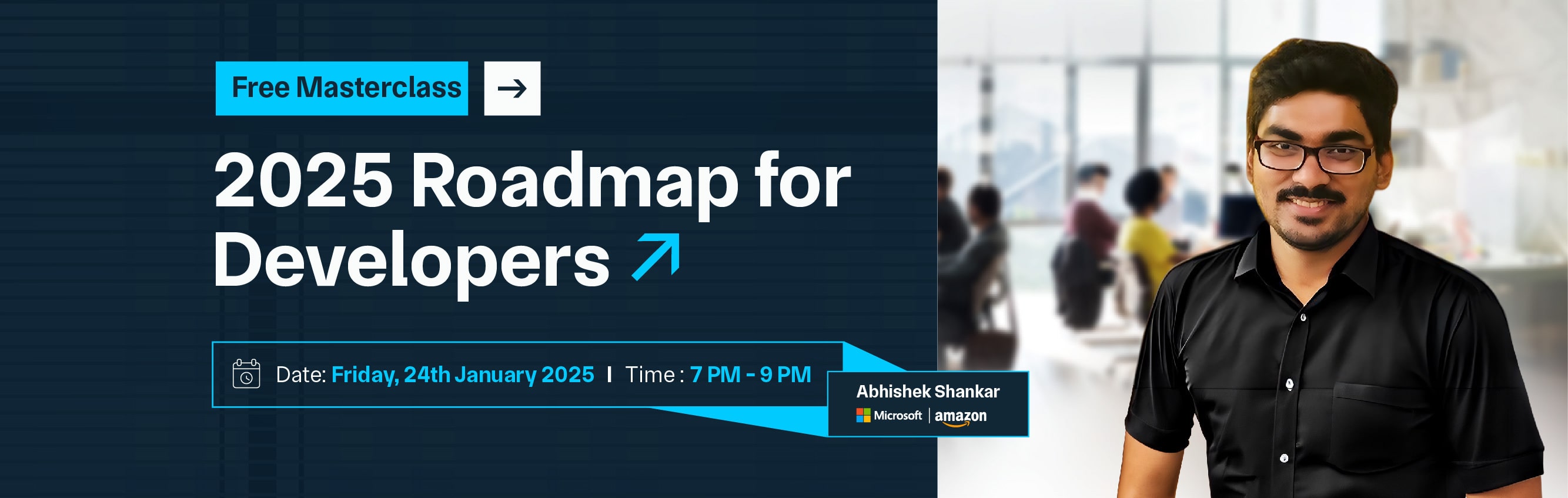

In Python, a delimiter is a character or sequence of characters used to separate elements within strings or data structures, making it essential for parsing and managing text and data. Delimiters play a crucial role in operations such as splitting strings and reading structured data files. For instance, the split() method uses a specified delimiter to divide a string into a list of substrings, for example, text. split(',') splits a string at each comma.
Similarly, when working with CSV files, the csv. Reader function utilizes delimiters to parse each line into a list of values, where a comma is commonly used to separate fields. Additionally, the join() method combines a list of strings into a single string, with a chosen delimiter inserted between each element; for instance, ' '.join(words) creates a sentence from a list of words using a space as the delimiter. Delimiters, therefore, are fundamental tools in Python for handling and structuring textual and tabular data, ensuring effective data manipulation and retrieval.
In Python, delimiters are integral for managing and processing data by defining boundaries within strings or files. For example, in parsing logs or configuration files, custom delimiters can be used to handle specific formats. Delimiters also assist in data transformation tasks, such as converting between different file formats, ensuring compatibility and ease of data handling across various applications.
In Python, delimiters are used to split or join strings and manage data formats. Here are several common examples of how delimiters are employed:
The split() method is used to divide a string into a list of substrings based on a delimiter.
Example:
text = "apple;banana;cherry"
fruits = text.split(';')
print(fruits)
Output:
['apple', 'banana', 'cherry']
Here, the semicolon (;) is the delimiter used to separate each fruit name.
The join() method concatenates elements of a list into a single string using a delimiter between elements.
Example:
words = ["Python", "is", "fun"]
sentence = ' '.join(words)
print(sentence)
Output:
Python is fun
In this example, a space (' ') is the delimiter used to join the words into a sentence.
When working with CSV files, delimiters like commas are used to separate values. The csv module can handle such files efficiently.
Example:
import csv
csv_data = """ name,age,city
Alice,30, New York
Bob,25,Los Angeles"""
# Simulating reading from a file
from io import StringIO
file = StringIO(csv_data)
reader = csv.reader(file, delimiter=',')
for row in reader:
print(row)
Output:
['name,' 'age,' 'city']
['Alice', '30', 'New York']
['Bob', '25', 'Los Angeles']
Here, the comma (,) acts as the delimiter to separate fields in each row.
You can use custom delimiters to format strings in various ways.
Example:
data = "2024-07-30|John Doe|Software Engineer"
parts = data.split('|')
print(parts)
Output:
['2024-07-30', 'John Doe,' 'Software Engineer']
In this case, the pipe (|) is the delimiter separating the date, name, and occupation.
Delimiters are versatile tools in Python for managing and manipulating text and data, helping to parse, format, and organize information effectively.
In Python, delimiters are characters or sequences used to separate or organize elements within strings and data. They play a crucial role in parsing, formatting, and processing text and structured data. Common delimiters include commas for CSV files, spaces for word separation, and tabs for columns in tab-delimited files.
They help in splitting strings into lists, joining lists into strings, and managing different data formats efficiently. By understanding and using these delimiters effectively, Python developers can handle and manipulate data with greater precision and ease.
Delimiter
Description
Example
Comma (,)
Commonly used to separate items in lists, CSV files, and parameters.
text = "apple,banana,cherry"<br>items = text.split(',')<br>Output: ['apple', 'banana', 'cherry']
Space (' ')
Used to separate words or elements in text.
sentence = "Python is awesome"<br>words = sentence.split(' ')<br>Output: ['Python', 'is', 'awesome']
Semicolon (;)
Often used in CSV files or data formats to separate values.
data = "2024-07-30;John Doe;Software Engineer"<br>parts = data.split(';')<br>Output: ['2024-07-30', 'John Doe', 'Software Engineer']
Tab (\t)
Used to separate columns in tab-delimited files or data.
data = "Name\tAge\tCity"<br>columns = data.split('\t')<br>Output: ['Name', 'Age', 'City']
**Pipe (`
`)**
Used in some text files and custom data formats to separate fields.
Colon (:)
Used to separate keys and values or in time formats.
time = "14:30:00"<br>components = time.split(':')<br>Output: ['14', '30', '00']
Hyphen (-)
Used in date formats, IDs, or to separate parts of strings.
date = "2024-07-30"<br>parts = date.split('-')<br>Output: ['2024', '07', '30']
Slash (/)
Common in date formats, paths, and URLs.
url = "http://example.com/path/to/resource"<br>segments = url.split('/')<br>Output: ['http:,' '', 'example.com,' 'path,' 'to,' 'resource']
Period (.)
Used in file extensions, decimal numbers, and domain names.
filename = "document.txt"<br>name, ext = filename.split('.')<br>Output: ['document,' 'txt']
Double Quotes (")
Used to denote string literals or separate fields in data.
Data = '"John," "Doe," "30"'<br>fields = data.split('","')<br>Output: ['"John",' '"Doe",' '"30"']
In Python, delimiters are characters or sequences used to separate or terminate sections of data, code, or text. They are crucial in various contexts, such as string manipulation, file parsing, and code formatting. Here’s an overview of how delimiters work in different scenarios in Python:
a. Splitting Strings
When you have a string and you want to break it into parts based on a delimiter, you use the split() method.
For example:
text = "apple,banana,cherry"
parts = text.split(",")
print(parts) # Output: ['apple', 'banana', 'cherry']
Here, the comma , is the delimiter that separates each fruit in the string.
b. Joining Strings
To combine a list of strings into a single string with a delimiter, you use the join() method:
Fruits = ['apple,' 'banana,' 'cherry']
text = ", ".join(fruits)
print(text) # Output: "apple, banana, cherry"
In this case, "" is the delimiter used to join the list elements.
When reading from or writing to files, delimiters help in parsing structured data formats. For example, CSV (Comma-Separated Values) files use commas as delimiters to separate values.
import csv
with open('data.csv', mode='r') as file:
csv_reader = csv.reader(file, delimiter=',')
for row in csv_reader:
print(row)
Here, ',' is the delimiter used by the csv.reader to parse the CSV file.
Delimiters in regular expressions can be used to specify patterns for matching or splitting strings. For instance, to split a string by any of several delimiters:
import re
text = "apple;banana,orange|grape"
parts = re.split(r'[;,|]', text)
print(parts) # Output: ['apple', 'banana', 'orange', 'grape']
In this case, the regular expression [;,|] matches any of the characters;, ,, or |, effectively using them as delimiters.
When formatting strings with placeholders, delimiters can help in organizing the format. For example, in f-strings or using the format() method, delimiters are used to specify where and how values should be inserted:
name = "Alice"
age = 30
formatted_string = f"Name: {name}, Age: {age}"
print(formatted_string) # Output: "Name: Alice, Age: 30"
Here, ", " serves as a delimiter between the different pieces of information in the formatted string.
When handling command-line arguments, delimiters can be used to parse options and values. For example, when using argparse:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('-n', '--name', type=str, help='Name of the user')
args = parser.parse_args()
print(args.name)
Here, -n or --name acts as a delimiter to specify different options for the command-line arguments.
The split() method in Python is a string method used to divide a string into a list of substrings based on a specified delimiter. By default, it separates the string at each whitespace character (such as spaces, tabs, or newlines). You can also provide a custom delimiter as an argument to the split() method, which tells Python where to make the splits.
For example, calling "apple,banana,cherry".split(',') would produce the list ['apple', 'banana', 'cherry']. Additionally, you can limit the number of splits by specifying the maxsplit parameter, which controls how many times the string is divided. If no delimiter is found or the string is empty, the method handles these cases gracefully, returning either a list with the original string or an empty list, respectively.
The Python string split() function is a method used to divide a string into a list of substrings based on a specified delimiter. It is a commonly used function for parsing and manipulating strings.
str.split([separator[, maxsplit]])
1. Basic Usage
text = "Hello world"
result = text.split()
print(result)
Output:
['Hello', 'world']
Here, the string is split at each space.
2. Using a Custom Separator
data = "apple,banana,cherry"
result = data.split(',')
print(result)
Output:
['apple', 'banana', 'cherry']
In this example, the string is split at each comma.
3. Limiting the Number of Splits
text = "one two three four"
result = text.split(' ', 2)
print(result)
Output:
['one', 'two', 'three four']
Here, the string is split into a maximum of three parts.
4. No Delimiter Provided
text = " a b c "
result = text.split()
print(result)
Output:
['a', 'b', 'c']
When no delimiter is specified, the method splits on any whitespace and ignores extra whitespace.
The syntax of the split() method for strings in Python is as follows:
str.split([separator[, maxsplit]])
1. separator (optional):
2. maxsplit (optional):
1. Basic Usage (Default separator: whitespace)
text = "Hello world"
result = text.split()
print(result)
Output:
['Hello', 'world']
2. Using a Custom Separator
data = "apple,banana,cherry"
result = data.split(',')
print(result)
Output:
['apple', 'banana', 'cherry']
3. Limiting the Number of Splits
text = "one two three four"
result = text.split(' ', 2)
print(result)
Output:
['one', 'two', 'three four']
4. Empty String with No Separator
text = " a b c "
result = text.split()
print(result)
Output:
['a', 'b', 'c']
The split() function in Python is crucial for various text-processing tasks. It enables developers to parse and manage strings by dividing them into a list of substrings based on a specified delimiter. This capability is particularly useful for parsing structured data formats, such as CSV files, where commas or other delimiters separate fields.
It also facilitates tokenization in text analysis, allowing the breakdown of sentences into individual words or tokens. Additionally, split() helps in data cleaning by normalizing and removing unwanted whitespace from strings. It is essential for extracting specific pieces of information from structured text, such as date components or URL parts. Overall, the split() function simplifies string manipulation and analysis, making it an indispensable tool in programming.
The split() function in Python is a powerful tool for dividing strings into lists of substrings based on a specified delimiter. By default, it splits a string at any whitespace, but you can also provide a custom separator to target specific characters or patterns. For example, calling split() on a string like "apple, banana, cherry" with a comma as the delimiter will yield a list of individual fruit names.
Additionally, the max split parameter allows you to control the number of splits, enabling you to limit how many parts the string is divided into. This function is particularly useful for parsing data, such as CSV files, processing user inputs, or tokenizing text for analysis. It simplifies string manipulation tasks by allowing you to break down and work with text data easily.
Consider you have a string containing several words separated by spaces, and you want to divide this string into a list of individual words.
text = "Python is a versatile language"
words = text.split()
print(words)
Explanation:
Output:
['Python', 'is', 'a', 'versatile', 'language']
In this example, the string "Python is a versatile language" is split into individual words, resulting in a list where each word is an element. This method is particularly useful for tasks such as text analysis, where breaking down sentences into words or tokens is necessary.
The parameters for the split() method in Python are:
To parse a string in Python using the split() method, follow these steps:
Suppose you have a string containing a comma-separated list of names and ages, and you want to parse this information into separate components:
# Example string
data = "John Doe,30,New York"
# Step 1: Identify the delimiter (comma in this case)
# Step 2: Use the split() method to parse the string
parsed_data = data.split(',')
# Step 3: Process the resulting list
name = parsed_data[0]
age = parsed_data[1]
city = parsed_data[2]
print(f"Name: {name}")
print(f"Age: {age}")
print(f"City: {city}")
Output:
Name: John Doe
Age: 30
City: New York
To use the split() method in Python with parameters, follow these steps:
str.split([separator[, maxsplit]])
1. Using a Custom Separator
text = "apple;banana;cherry"
# Split using ';' as the separator
fruits = text.split(';')
print(fruits)
Output:
['apple', 'banana', 'cherry']
Here, the separator is ';', so the string is split at each semicolon.
2. Limiting the Number of Splits
text = "one two three four"
# Split using ' ' (space) with a maximum of 2 splits
parts = text.split(' ', 2)
print(parts)
Output:
['one', 'two', 'three four']
In this example, the maxsplit parameter is 2, so the string is split into three parts: the first two splits occur at spaces, and the remainder of the string is left as the last part.
3. Using Both Parameters
text = "apple,banana,grape,orange"
# Split using ',' as the separator with a maximum of 2 splits
result = text.split(',', 2)
print(result)
Output:
['apple', 'banana', 'grape,orange']
Here, the string is split at the first two commas, and the rest of the string is left intact.
The split() function in Python is a versatile tool for breaking down strings into lists of substrings based on specified delimiters. By default, it splits by whitespace, but you can customize the separator to handle different delimiters, limit the number of splits, or use regular expressions for more complex scenarios. This functionality is essential for tasks like parsing text, processing user input, and cleaning data.
The split() function in Python offers flexible ways to divide strings into substrings based on specified delimiters. It's useful for tasks like text processing and data parsing. Understanding tips and best practices, such as handling multiple delimiters and managing performance, can enhance its effectiveness and efficiency in various applications.
Delimiters are used in a variety of scenarios in Python for managing and organizing data, text, and code. Here’s a detailed look at some common use cases for delimiters:
a. Splitting Strings
Delimiters are often used to split a string into parts based on a specific character or sequence. This is useful for parsing text data or extracting information.
text = "one;two;three;four"
parts = text.split(";")
print(parts) # Output: ['one', 'two', 'three', 'four']
b. Joining Strings
Conversely, delimiters can be used to combine a list of strings into a single string, with the delimiter separating the individual elements.
words = ['apple', 'banana', 'cherry']
sentence = " | ".join(words)
print(sentence) # Output: "apple | banana | cherry"
a. CSV Files
CSV files use commas (or other characters) as delimiters to separate values. The csv module in Python handles these delimiters to read and write data in CSV format.
import csv
# Reading a CSV file
with open('data.csv', mode='r') as file:
csv_reader = csv.reader(file, delimiter=',')
for row in csv_reader:
print(row)
# Writing to a CSV file
with open('data.csv', mode='w', newline='') as file:
csv_writer = csv.writer(file, delimiter=',')
csv_writer.writerow(['Name', 'Age', 'City'])
csv_writer.writerow(['Alice', '30', 'New York'])
csv_writer.writerow(['Bob', '25', 'Los Angeles'])
b. Tab-Delimited Files
For tab-delimited data, you can specify the tab character as a delimiter.
with open('data.tsv', mode='r') as file:
tsv_reader = csv.reader(file, delimiter='\t')
for row in tsv_reader:
print(row)
In regular expressions, delimiters are used to define patterns for searching or splitting strings based on complex criteria.
import re
text = "apple;banana|cherry,grape"
parts = re.split(r'[;|,]', text)
print(parts) # Output: ['apple', 'banana', 'cherry', 'grape']
Command-line arguments often use delimiters to separate options and their values. The argparse module helps parse these arguments.
import argparse
parser = argparse.ArgumentParser(description='Process some integers.')
parser.add_argument('-n', '--name', type=str, help='Name of the user')
parser.add_argument('-a', '--age', type=int, help='Age of the user')
args = parser.parse_args()
print(f"Name: {args.name}")
print(f"Age: {args.age}")
In various text file formats, delimiters define the structure of the data. For example, in INI files, sections are delimited by headers:
[Section1]
key1=value1
key2=value2
[Section2]
keyA=valueA
keyB=valueB
Python’s configparser module can read such files, treating = as the delimiter between keys and values.
from configparser import ConfigParser
config = ConfigParser()
config.read('config.ini')
print(config['Section1']['key1']) # Output: value1
In data serialization formats like JSON, delimiters help structure the data into key-value pairs.
import json
data = {
"name": "Alice",
"age": 30,
"city": "New York"
}
json_string = json.dumps(data)
print(json_string) # Output: {"name": "Alice", "age": 30, "city": "New York"}
parsed_data = json.loads(json_string)
print(parsed_data) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York'}
Log files often use delimiters to separate fields for better readability and parsing. For instance, log entries might be separated by spaces, tabs, or custom characters.
log_entry = "2024-07-30 12:34:56 INFO ModuleName - Message"
timestamp, level, message = log_entry.split(" ", 2)
print(f"Timestamp: {timestamp}")
print(f"Level: {level}")
print(f"Message: {message}")
Delimiters are fundamental in Python for managing and structuring various types of data. They are pivotal in string manipulation, where they help in splitting and joining text to facilitate data extraction and processing. In file handling, delimiters such as commas in CSV or tabs in TSV files enable structured reading and writing, which is essential for managing large datasets. Regular expressions utilize delimiters to define complex search patterns, allowing precise text manipulations. In command-line interfaces, delimiters parse user inputs and options, making scripts and applications more flexible.
Configuration files and data serialization formats like JSON use delimiters to organize settings and data structures, streamlining configuration and data exchange. Additionally, delimiters in log files aid in parsing and analyzing log entries, which is crucial for debugging and monitoring. Overall, a deep understanding of delimiters enhances your ability to process and manage data efficiently, leading to more organized and readable code.
Copy and paste below code to page Head section
A delimiter is a character or sequence of characters used to separate or define boundaries between different pieces of data within a string or file. It helps in organizing and parsing data.
You can use the split() method of a string to split it by a specific delimiter. For example: text = "apple;banana;cherry" parts = text.split(";") print(parts) # Output: ['apple', 'banana', 'cherry']
Common delimiters in data files include: Commas (,): Used in CSV (Comma-Separated Values) files. Tabs (\t): Used in TSV (Tab-Separated Values) files. Semicolons (;): Sometimes used in CSV files, especially in European locales.
Yes, delimiters can be used in regular expressions to define patterns for searching or splitting text. For example: import re text = "apple;banana|cherry,grape" parts = re.split(r'[;|,]', text) print(parts) # Output: ['apple', 'banana', 'cherry', 'grape']
Delimiters in configuration files, such as =, :, or -, help separate keys and values, sections, or different configuration parameters, making it easier to read and manage settings.
Delimiters in command-line arguments separate options and values. For example, in argparse, you can define options and their delimiters: import argparse parser = argparse.ArgumentParser() parser.add_argument('-n', '--name', type=str, help='Name of the user') parser.add_argument('-a', '--age', type=int, help='Age of the user') args = parser.parse_args() print(f"Name: {args.name}") print(f"Age: {args.age}")