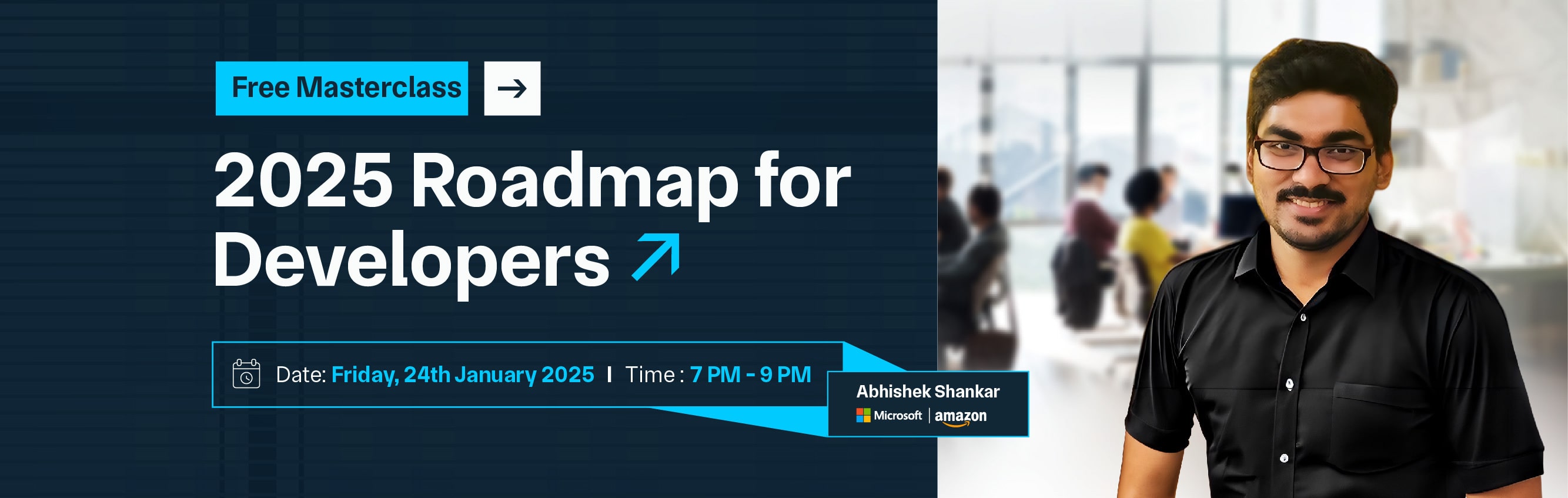

Data cleaning is a crucial step in the data science process, as it directly impacts the quality and reliability of analysis results. This process involves identifying and correcting errors, inconsistencies, and inaccuracies in datasets. Common issues that data cleaning addresses include missing values, duplicate records, outliers, and incorrect data types. By applying techniques such as imputation for missing values, normalization for inconsistent formats, and deduplication for repetitive entries, data scientists can significantly enhance data quality.
Effective data cleaning improves model performance and ensures that insights derived from the data are valid and actionable. It also involves standardizing data formats and eliminating irrelevant information that may skew results. In addition, tools like Python libraries (Pandas, NumPy) and R packages (dplyr, tidyr) facilitate data cleaning tasks, enabling more efficient workflows.
Ultimately, investing time and effort in data cleaning is essential for building robust models and making informed decisions based on accurate, high-quality data. A well-cleaned dataset lays the foundation for successful data analysis, machine learning applications, and overall data-driven strategies in organizations.
Data cleaning in data science is the process of identifying and rectifying errors, inconsistencies, and inaccuracies in datasets to improve their quality and reliability. It is a critical step in the data preparation phase, ensuring that the data used for analysis, modeling, and decision-making is accurate and trustworthy.
Overall, effective data cleaning is essential for accurate analysis and robust decision-making, as high-quality data leads to reliable insights and models in data science.
Data cleaning is vital for several reasons:
Data cleaning is essential for ensuring that data-driven initiatives are successful, reliable, and capable of delivering meaningful insights.
Data collection is the foundational step where raw data is gathered from various sources, such as databases, spreadsheets, APIs, or surveys. It's essential to ensure that the data collected is relevant to the analysis goals.
This stage involves defining the scope and objectives of the data collection process, determining the sources of data, and gathering it systematically. High-quality initial data leads to better cleaning outcomes, making this step crucial for setting the stage for the subsequent cleaning process.
Data profiling involves analyzing the dataset to understand its characteristics, structure, and quality. This step includes assessing data types, distributions, and overall completeness. During profiling, you identify issues such as missing values, duplicates, inconsistencies, and outliers.
By generating summary statistics and visualizations, you gain insights into the data's integrity and identify areas that require attention. This initial assessment informs the specific cleaning actions needed and helps prioritize efforts based on the data's condition.
Handling missing values is a critical step in the data-cleaning process. Missing data can arise from various sources, including data entry errors or incomplete records. In this step, you must decide how to address these gaps.
Options include imputation, where you fill in missing values based on other data points, or removal, where incomplete records are deleted from the dataset. The choice of method depends on the extent of the missing data and its potential impact on analysis. Proper handling ensures that the dataset remains robust for future analysis.
Removing duplicates is essential for ensuring the uniqueness and accuracy of each record in the dataset. Duplicate entries can skew analysis, lead to incorrect conclusions, and waste resources. This step involves identifying duplicate records based on specific criteria, such as identical values across key fields.
Once duplicates are identified, you can choose to keep one entry and discard the rest or aggregate data as needed. This process helps maintain the integrity of the dataset, facilitating more accurate insights and decision-making.
Correcting errors involves identifying and fixing inaccuracies in the dataset. This includes typographical errors, inconsistencies in data entry, and incorrect data types (e.g., numbers stored as text). Standardizing formats, such as ensuring consistent date representations or naming conventions, is also part of this step.
By systematically reviewing the data for mistakes and inconsistencies, you enhance the overall quality and reliability of the dataset. This step is crucial for ensuring that subsequent analyses are based on accurate and clean data.
Identifying outliers is an important step in understanding the distribution of your data. Outliers can indicate data entry errors or represent significant variations that warrant further investigation. This step involves analyzing the dataset for anomalies using statistical methods (like z-scores) or visualization techniques (like box plots).
Once outliers are identified, you must decide how to handle them whether to keep, adjust, or remove them based on their potential impact on the analysis. This ensures that the dataset accurately reflects the underlying patterns without being skewed by extreme values.
Filtering irrelevant data involves removing unnecessary or non-contributory information from the dataset. This step ensures that only the most relevant data points are retained for analysis, which improves the efficiency and clarity of subsequent processes.
Irrelevant data can include columns that do not serve the analysis objectives or records that do not meet specific criteria. By focusing on essential data, you streamline the dataset, making it easier to analyze and interpret, ultimately leading to more meaningful insights.
Documentation and validation are crucial for maintaining transparency and reproducibility in the data-cleaning process. In this final step, you should document each cleaning action taken, including the rationale behind the decisions made, methods used, and any transformations applied.
Validation involves checking the cleaned dataset against quality standards to ensure it meets the required criteria for accuracy and consistency. This step not only aids in future reviews and audits but also helps others understand and trust the cleaned data, fostering better collaboration and decision-making.
Here are some popular data cleaning tools that can help streamline the data cleaning process:
Pandas is a powerful data manipulation library in Python that provides data structures like DataFrames. It offers numerous functions for data cleaning, such as handling missing values, removing duplicates, and transforming data types. Its intuitive syntax makes it a favorite among data scientists for preprocessing tasks.
OpenRefine is a standalone tool specifically designed for data cleaning and transformation. It allows users to explore large datasets, identify inconsistencies, and apply various cleaning techniques. Its powerful clustering algorithms help identify duplicates, while its user-friendly interface supports multiple data formats.
Trifacta is a data preparation tool that provides a visual interface for data cleaning and transformation. It offers smart suggestions for cleaning tasks and allows users to manipulate data using drag-and-drop functionality easily. It's particularly useful for users who prefer a graphical interface over coding.
Dplyr is an R package designed for data manipulation and cleaning. It provides a set of functions for filtering, grouping, summarizing, and transforming data. Its straightforward syntax makes data-cleaning tasks efficient and intuitive, especially for R users.
DataWrangler is a web-based tool that allows users to clean and transform data interactively. It provides a step-by-step interface to manipulate data, with automatic suggestions for cleaning actions based on user input. It's ideal for users looking for a quick and easy solution.
Microsoft Excel is a widely used spreadsheet application that offers various built-in functions for data cleaning, such as removing duplicates, filtering data, and handling missing values. While not specialized, its accessibility and familiarity make it a common choice for basic data-cleaning tasks.
Talend is an open-source data integration tool that offers data cleaning capabilities as part of its data management suite. It provides an intuitive interface for designing data workflows, allowing users to perform complex transformations and data quality checks easily.
Apache Nifi is a powerful data integration tool that automates data flow between systems. It includes features for data cleaning, transformation, and validation, making it suitable for large-scale data processing tasks.
Google Sheets is another spreadsheet tool that provides data-cleaning functions similar to Excel. Its collaborative features make it useful for teams working together on data-cleaning tasks.
DataRobot is an automated machine learning platform that includes data cleaning and preprocessing features. It helps identify data quality issues and suggests transformations, streamlining the preparation process for machine learning models. These tools can significantly enhance the efficiency and effectiveness of data cleaning efforts, helping data professionals produce high-quality, reliable datasets for analysis.
Here’s a basic guide for implementing database cleaning using Python. We'll utilize libraries such as Pandas for data manipulation and SQLite for handling a sample database. This example focuses on cleaning a dataset by addressing missing values, duplicates, and data type inconsistencies.
First, ensure you have the necessary libraries installed. You can install them using pip:
Pip install pandas sqlite3
We'll create a simple SQLite database and populate it with some sample data.
import sqlite3
Import pandas as pd
# Create a sample SQLite database
conn = sqlite3.connect('sample_database.db')
# Create a sample table
conn.execute('''
CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY,
name TEXT,
email TEXT,
age INTEGER
)
''')
# Insert sample data with some inconsistencies
data = [
(1, 'Alice', 'alice@example.com', 30),
(2, 'Bob', None, 25), # Missing email
(3, 'Charlie,' 'charlie@example.com,' None), # Missing age
(4, 'David,' 'david@example.com', 40),
(5, 'Eve', 'alice@example.com', 30), # Duplicate email
]
conn.executemany('INSERT INTO users (id, name, email, age) VALUES (?, ?, ?, ?)', data)
conn.commit()
Next, we’ll load the data from the database into a Pandas DataFrame for cleaning.
# Load data into a Pandas DataFrame
df = pd.read_sql_query('SELECT * FROM users', conn)
print("Original Data:")
print(df)
Now, let's perform some common cleaning operations:
# 1. Handling Missing Values
df['email'].fillna('unknown@example.com', inplace=True) # Fill missing emails
df['age'].fillna(df['age'].median(), inplace=True) # Fill missing ages with median
# 2. Removing Duplicates
df.drop_duplicates(subset=['email'], inplace=True) # Remove duplicates based on email
# 3. Fixing Data Types
df['age'] = df['age'].astype(int) # Ensure age is an integer type
print("\nCleaned Data:")
print(df)
After cleaning, you can save the cleaned DataFrame back to the database.
# Save cleaned data back to the database
df.to_sql('cleaned_users', conn, if_exists='replace', index=False)
# Close the database connection
conn.close()
Data cleaning is a vital step in the data science process, offering numerous benefits that significantly impact the quality of analysis and decision-making. Here are some key advantages:
Clean data ensures that the insights derived from analysis are accurate. By addressing errors and inconsistencies, data cleaning reduces the risk of faulty conclusions, leading to more reliable outcomes.
In machine learning, the quality of input data directly affects model accuracy. Clean datasets lead to better model performance, resulting in more reliable predictions and effective decision-making.
By removing duplicates and irrelevant information, data cleaning streamlines datasets, making them easier to work with. This efficiency saves time in data processing and analysis, allowing data scientists to focus on generating insights.
High-quality data supports informed decision-making. Clean data provides a solid foundation for understanding trends and patterns, leading to more effective strategies and actions.
Clean data fosters trust among stakeholders. When data is accurate and reliable, it enhances confidence in data-driven processes, leading to greater collaboration and alignment within organizations.
In many industries, maintaining data quality is essential for regulatory compliance. Data cleaning helps ensure that datasets meet required standards, reducing the risk of legal issues and penalties.
Clean and well-organized data makes it easier for teams to collaborate on projects. When everyone works from a reliable dataset, it minimizes misunderstandings and miscommunication.
Investing in data cleaning upfront can lead to significant long-term cost savings. By reducing errors and improving data quality, organizations can avoid costly rework and lost opportunities. Overall, data cleaning is essential for ensuring that data-driven initiatives are successful, reliable, and capable of delivering meaningful insights in data science.
Data cleaning and data transformation are two critical processes in data preparation for analysis. While they are often used together, they serve distinct purposes. Data cleaning focuses on improving data quality by addressing inaccuracies and inconsistencies, while data transformation involves modifying data to fit specific formats or structures suitable for analysis.
Data cleaning is a fundamental process in data science that significantly impacts the quality of analysis and insights. While it offers numerous advantages, such as improved accuracy and enhanced model performance, it also presents certain challenges, including time consumption and potential data loss.
Understanding these advantages and disadvantages helps data professionals weigh the benefits of thorough data cleaning against the resources required, ultimately leading to more informed and effective data management practices. Below is a comparative overview of the advantages and disadvantages of data cleaning.
Data cleaning is an essential step in the data science workflow that directly influences the quality and reliability of analysis. By systematically identifying and rectifying inaccuracies, inconsistencies, and missing values, data cleaning ensures that datasets are robust and trustworthy. The benefits of this process such as improved accuracy, enhanced model performance, and increased trust in data-driven decisions underscore its importance in any analytical endeavor.
While it can be time-consuming and require ongoing effort, the investment in data cleaning ultimately leads to more meaningful insights and better outcomes in data science projects. Prioritizing data cleaning not only enhances the integrity of analysis but also fosters a culture of data quality within organizations.
Copy and paste below code to page Head section
Data cleaning in data science refers to the process of identifying and rectifying inaccuracies, inconsistencies, and errors in datasets to ensure the data is suitable for analysis.
Clean data is essential for producing reliable insights and accurate predictions. It helps prevent biases in analysis and ensures that models perform optimally.
Common issues include missing values, duplicate records, outliers, inconsistent data formats, and incorrect data types.
You can use data profiling techniques or functions within data manipulation libraries (like Pandas) to check for null or NaN values in your dataset.
Techniques include imputation (filling in values), removing records, or using predictive models that can accommodate missing values.
Popular tools include Pandas and NumPy (Python), OpenRefine, Dplyr (R), and ETL tools like Talend and Apache NiFi.