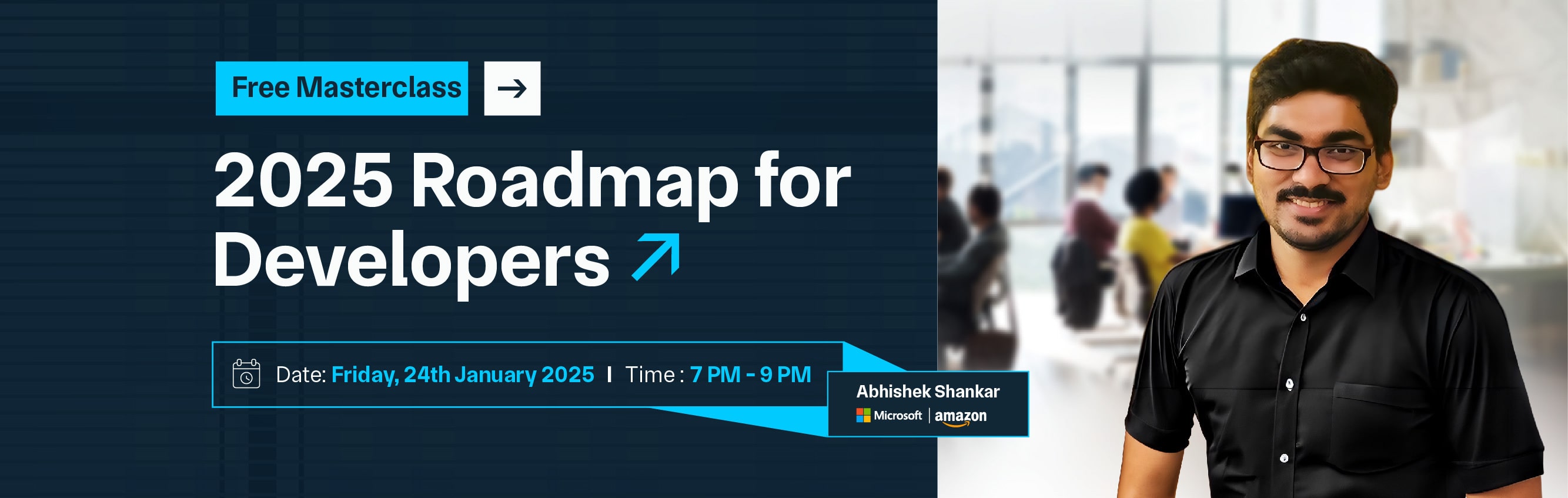

Embarking on practical projects is an excellent way to deepen your understanding of C++ programming. Whether you're starting or aiming to advance your skills, these projects offer hands-on experience and insight into various aspects of the language. Create a text-based RPG game to explore classes and inheritance, or develop an inventory management system utilising data structures like arrays and linked lists.
For a more challenging endeavour, design a banking system to handle transactions securely or dive into image processing by building a tool for manipulating images with filters and resizing functionalities. Alternatively, implementing advanced algorithms such as sorting or graph algorithms will sharpen your problem-solving abilities and algorithmic thinking. Each project not only reinforces C++ fundamentals but also equips you with valuable experience applicable to real-world software development scenarios.
Start coding today and watch your skills flourish through practical application and exploration in C++. Embarking on practical projects is an excellent way to deepen your understanding of C++ programming. Whether you're starting or aiming to advance your skills, these projects offer hands-on experience and insight into various aspects of the language. Create a text-based RPG game to explore classes and inheritance, or develop an inventory management system utilising data structures like arrays and linked lists.
"C++ projects" typically refer to programming tasks or applications written in the C++ programming language. These projects can range from simple exercises to complex applications or systems designed to practice and demonstrate proficiency in C++. Examples include developing games, creating software tools, building algorithms, implementing data structures, or even working on system-level programming tasks.
C++ projects are instrumental in learning and mastering the language, as they provide hands-on experience in applying C++ syntax, concepts, and best practices to solve real-world problems or simulate practical scenarios.
1. Syntax and Language Features: Mastery of basic and advanced C++ syntax, such as variables, loops, functions, classes, inheritance, polymorphism, templates, and exception handling.
2. Data Structures: Understanding and implementation of fundamental data structures like arrays, linked lists, stacks, queues, trees, and graphs to manage and manipulate data efficiently.
3. Algorithms: Application and implementation of algorithms, including sorting (e.g., bubble sort, quicksort), searching (e.g., binary search), graph algorithms (e.g., Dijkstra's algorithm), and dynamic programming techniques.
4. Memory Management: Proficiency in managing memory dynamically using pointers, arrays, and smart pointers (e.g., std::unique_ptr, std::shared_ptr) to prevent memory leaks and optimise performance.
5. File Handling: Ability to read from and write to files using file streams (ifstream, ofstream) for data persistence and manipulation.
6. Object-Oriented Design: Utilization of object-oriented principles such as encapsulation, inheritance, and polymorphism to create modular, reusable, and maintainable code.
7. User Interface (UI) Development: Implementation of graphical user interfaces (GUI) using libraries like Qt or SDL or command-line interfaces (CLI) for interactive applications.
8. Testing and Debugging: Proficiency in debugging techniques, using tools like debuggers (e.g., GDB) and writing unit tests (e.g., using frameworks like Google Test) to ensure code correctness and reliability.
9. Performance Optimization: Awareness of performance considerations, such as algorithmic complexity (big-O notation), optimisation techniques (e.g., loop unrolling, caching), and profiling tools (e.g., Valgrind, perf).
10. Documentation and Collaboration: Effective documentation practices (e.g., comments, README files) and collaboration tools (e.g., version control systems like Git) to facilitate teamwork and project maintenance.
These elements collectively contribute to the development of robust and efficient C++ projects, enhancing both technical skills and understanding of software development principles.
Explore these diverse C++ project ideas designed to enhance your programming skills and practical application knowledge. From basic console applications to sophisticated systems with graphical interfaces, each project offers opportunities to delve into fundamental concepts and tackle real-world challenges.
Whether you're a beginner looking to build foundational skills or an experienced programmer seeking to expand your portfolio, these projects cover a wide range of domains including software development, data management, gaming, finance, and more. Choose projects that align with your interests and goals, and embark on a journey of learning, problem-solving, and creativity with C++.
1. Simple Calculator: Implement a basic arithmetic calculator.
2. To-Do List: Create a console-based to-do list manager.
3. Temperature Converter: Convert between Celsius, Fahrenheit, and Kelvin.
4. Number Guessing Game: Generate a random number and have the user guess it.
5. BMI Calculator: Calculate Body Mass Index based on user input.
6. Bank Account Manager: Implement basic banking operations like deposit, withdrawal, and balance inquiry.
7. Hangman Game: Classic word-guessing game implemented in the console.
8. Simple File Management System: Create, read, update, and delete files.
9. Quiz Game: Design a quiz with multiple-choice questions and keep score.
10. Text-based RPG: Create a simple text adventure or role-playing game.
11. Student Database: Manage student records with CRUD operations.
12. Library Management System: Handle books, members, and borrowing.
13. Inventory Management System: Track items in stock, add/remove items, etc.
14. Chess Game: Implement a two-player chess game with basic rules.
15. Flight Reservation System: Manage flights, bookings, and passenger details.
16. Simple Paint Application: Basic drawing tools like pencil, shapes, colors.
17. Employee Payroll System: Calculate salaries, deductions, and bonuses.
18. Tic-Tac-Toe: Two-player console version with basic AI for single-player mode.
19. ATM Simulator: Simulate ATM functionalities like balance inquiry, withdrawal, etc.
20. Music Player: Basic music player with play, pause, stop functions.
21. Chat Application: Implement a simple peer-to-peer chat system.
22. Online Bookstore: Create a web-based bookstore with shopping cart functionality.
23. Compiler for a Simple Language: Design and implement a compiler or interpreter.
24. Database Management System: Create a basic DBMS with SQL-like commands.
25. Graphical Calculator: Build a calculator with a graphical user interface (GUI).
26. Stock Market Simulator: Simulate buying/selling stocks and track portfolio.
27. Racing Game: 2D racing game with tracks, vehicles, and scoring.
28. Image Editor: Basic image editing functions like crop, rotate, filters.
29. Remote Desktop Application: Share desktop screens over a network.
30. Virtual Memory Manager: Simulate virtual memory operations like paging.
31. Operating System: Build a basic OS kernel with process management.
32. Blockchain Implementation: Create a simple blockchain with basic consensus.
33. Real-time Strategy Game: Multiplayer game with real-time strategy elements.
34. Neural Network Library: Implement a library for training and testing neural networks.
35. Distributed File System: Design and implement a distributed file storage system.
36. 3D Rendering Engine: Build a basic 3D engine with rendering capabilities.
37. Compiler Optimizations: Implement optimization passes for a compiler.
38. Voice Recognition System: Create a system that recognizes spoken commands.
39. Network Packet Sniffer: Capture and analyze network packets.
40. Genetic Algorithm Simulator: Implement a framework for genetic algorithms.
41. Encryption/Decryption Tool: Implement various encryption algorithms.
42. Data Compression Tool: Build a utility for compressing and decompressing files.
43. Code Editor with Syntax Highlighting: Create a text editor for code files.
44. Online Quiz Platform: Web-based platform for conducting quizzes.
45. Video Streaming Server: Stream video over a network using sockets.
46. Digital Signal Processing Tool: Implement basic DSP algorithms.
47. IoT-based Home Automation System: Control home devices via IoT.
48. Recipe Management System: Manage recipes, ingredients, and meal planning.
49. E-commerce Platform: Create a basic online shopping platform.
50. Peer-to-Peer File Sharing System: Share files directly between users.
51. Chess Game with GUI: Implement chess with a graphical interface.
52. Music Library Organizer: GUI application for managing a music collection.
53. Weather App: Display current weather and forecast using API data.
54. Calendar Application: GUI calendar with reminders and events.
55. Drawing Application: Create a simple drawing tool with brushes and colours.
56. Personal Finance Tracker: Track income, expenses, and budgeting.
57. Video Player with Playlist: GUI-based video player with playlist management.
58. Task Scheduler: GUI application to schedule tasks and reminders.
These project ideas cover a wide range of complexity levels, from beginner to expert, and span various domains such as games, utilities, simulations, and system software. Choose a project that aligns with your interests and skill level to get started!
Embark on your journey into C++ programming with these beginner-friendly project ideas. These projects are designed to help you grasp essential concepts while building practical applications. Each project introduces fundamental programming techniques, from basic data manipulation to simple algorithm implementation.
Whether you're new to programming or transitioning from another language, these projects provide a solid foundation for mastering C++ and laying the groundwork for more complex programming challenges ahead. Choose a project that interests you and start honing your coding skills with hands-on experience in C++.
A simple calculator project involves creating a console-based application that performs basic arithmetic operations such as addition, subtraction, multiplication, and division. Users input two operands and choose an operator (+, -, *, /). The program validates inputs to ensure they are numeric and handles edge cases like division by zero.
It then computes the result and displays it to the user. Error handling ensures the program gracefully handles invalid inputs and provides feedback to guide the user. This project is ideal for learning fundamental programming concepts like variables, conditional statements, loops, and functions/methods. It also introduces basic input/output operations and error-handling techniques in C++.
Source Code: Click Here
A to-do list manager allows users to organize tasks by adding, viewing, updating, and deleting them via a console interface. Users can input tasks with descriptions and optionally set priorities or due dates. The program stores tasks in memory or uses file handling to persist data across sessions. Features include sorting tasks by different criteria (priority, due date), marking tasks as completed, and displaying task lists in a readable format.
Input validation ensures that tasks are correctly formatted, and error handling prevents crashes due to invalid user inputs. This project enhances skills in data structures such as arrays or linked lists for storing tasks, file handling for data persistence, and implementing CRUD (Create, Read, Update, Delete) operations. It also involves user interaction design by providing clear prompts and feedback during task management operations.
Source Code: Click Here
A temperature converter project allows users to convert temperatures between Celsius, Fahrenheit, and Kelvin scales. The program prompts the user to input a temperature along with its current scale (e.g., 25 Celsius). The user then selects the desired scale for conversion (e.g., Fahrenheit) and the program calculates and displays the converted temperature.
Input validation ensures that numeric values are entered and that valid scale selections are made. Error handling prevents inappropriate conversions (like negative temperatures in Kelvin). This project enhances understanding of basic arithmetic operations, conditional statements for decision-making, and input/output operations in C++. It also reinforces the concept of modular programming by encapsulating conversion logic into functions or methods for reusability and clarity.
Source Code: Click Here
The number-guessing game is a console-based game where the program randomly selects a number within a specified range (e.g., 1 to 100). The user tries to guess the number within a limited number of attempts. After each guess, the program provides feedback (too high, too low) to guide the user toward the correct answer. The game tracks the number of attempts made and ends when the user guesses correctly or exhausts all attempts. It includes features like replayability, where users can play again after completing a game session.
This project enhances skills in random number generation, conditional statements for decision-making, loops for iteration, and handling user input. It also introduces game logic design, where feedback and game state management (attempts remaining, correct guesses) are crucial for a satisfying user experience. Error handling ensures that inputs are valid and within the expected range, preventing crashes or unexpected behaviour during gameplay.
Source Code: Click Here
A BMI (Body Mass Index) calculator computes the BMI based on user input of height and weight. The program then interprets the BMI value to classify the user's weight status (underweight, normal weight, overweight, obese) and provides health advice accordingly. Input validation ensures that numeric values are entered for height and weight, and error handling prevents division by zero or other invalid calculations.
This project enhances skills in arithmetic calculations, conditional statements for BMI classification, and output formatting for a clear presentation of results. It also introduces concepts of interpreting and applying formulas in real-world contexts, reinforcing mathematical operations in programming. Additionally, incorporating user-friendly prompts and health advice based on BMI categories enhances the interactive experience, requiring consideration of user input and logical branching in the program flow.
Source Code: Click Here
The Bank Account Manager project simulates fundamental banking operations through a console-based application. Users can create accounts, deposit funds, withdraw funds, and check balances. The program manages multiple accounts by storing data securely and maintains transaction histories for each account. It ensures transaction security by validating operations such as sufficient funds for withdrawals and valid account numbers for transfers. Error handling is crucial to handle scenarios like invalid inputs or insufficient funds gracefully, preventing crashes and maintaining data integrity.
This project enhances skills in object-oriented programming (OOP) by implementing classes for accounts and transactions, file handling for data storage, and user input validation. It also introduces concepts of data encapsulation, inheritance (for different types of accounts), and polymorphism (for handling different transaction types). Overall, it provides practical experience in simulating real-world banking systems while emphasizing secure and accurate financial interactions.
Source Code: Click Here
The Hangman Game is a classic word-guessing game implemented in the console. The program selects a word at random from a predefined list and displays it as underscores representing each letter. Players guess letters one by one within a limited number of attempts. The game tracks guesses, updates the displayed word with correctly guessed letters, and manages the game state by keeping count of remaining attempts.
Feedback on each guess informs the player whether the guessed letter is correct or not. This project enhances skills in string manipulation, randomisation (for word selection), conditional statements, loops, and user input handling. It also introduces game logic design by managing game states (such as game over conditions) and providing a satisfying user experience through interactive feedback. Error handling ensures that inputs are valid and within expected ranges, enhancing the robustness of the game.
Source Code: Click Here
The Simple File Management System allows users to perform basic file operations through console commands. Users can create new files, read content from existing files, update file contents, and delete files. The system manages these operations by handling file existence checks, permission validations, and error handling for operations like file not found or insufficient permissions.
This project enhances skills in file input/output (I/O) operations, string manipulation (for file content handling), error handling, and command-line interface (CLI) interaction design. It also reinforces an understanding of data structures and algorithms for efficient file management operations. By implementing CRUD (Create, Read, Update, Delete) functionalities for files, the project provides practical experience in developing file-handling utilities commonly used in software development.
Source Code: Click Here
The Quiz Game is an interactive console-based application where users participate in quizzes with multiple-choice questions. The program tracks scores based on correct answers and displays results at the end of the quiz. Questions can be stored in files or arrays, and the game randomly selects questions to present to the user. The program validates user input to prevent errors such as choosing an invalid option or skipping questions. Features like random question selection and diverse quiz topics enhance user engagement and replayability.
This project enhances skills in data structures (for storing questions and options), file handling (for quiz data storage), conditional statements (for user input validation), and loops (for iterative quiz flow). It also introduces concepts of modular programming by encapsulating quiz logic into functions or classes, promoting code reusability and maintainability. Overall, it provides practical experience in designing interactive applications with structured data and user interaction.
Source Code: Click Here
The Text-based RPG project creates an interactive role-playing game where players navigate through a story by making choices that impact the outcome. Players can create characters, manage inventory items, engage in combat encounters, and experience branching storylines based on their decisions. The game manages player statistics (stats), items, and progression through text-based interactions and scenario descriptions. Skills like combat resolution, inventory management, and character progression are handled through a series of choices and randomised outcomes.
This project enhances skills in object-oriented design (OOP) by implementing classes for characters, items, and game mechanics. It also involves data structures (for managing game states and inventories), decision-making algorithms (for branching storylines), and input handling (for user choices). Error handling ensures that the game responds appropriately to unexpected inputs or invalid actions, maintaining a smooth gameplay experience. Overall, it provides practical experience in designing and implementing complex game systems within a text-based environment, emphasising narrative design and player choice impact.
Source Code: Click Here
Transition to the next level of C++ proficiency with these intermediate project ideas. Designed to challenge your understanding of object-oriented programming, data structures, and algorithms, these projects go beyond basic concepts. You'll delve into creating more sophisticated applications that require deeper problem-solving and implementation skills.
Each project offers an opportunity to apply advanced C++ features like templates, inheritance, and polymorphism, enhancing your ability to design robust and efficient software solutions. Whether you're looking to strengthen your portfolio or prepare for more complex programming tasks, these projects will elevate your programming prowess in C++.
The Student Database project involves managing student records with CRUD (Create, Read, Update, Delete) operations. It allows users to add new students, view existing records, update student information, and delete records when necessary. The database can be implemented using arrays, linked lists, or databases like SQLite for more advanced implementations.
Error handling ensures that operations like adding duplicate records or accessing nonexistent records are managed gracefully. This project enhances skills in data structures (for storing student information), file handling (for persistent storage), and user interface design for displaying and managing student data effectively.
Source Code: Click Here
A Library Management System handles books, members, and borrowing processes. It allows librarians to add new books to the collection, manage member registrations, track borrowed and returned books, and handle fines for overdue books. The system maintains inventory levels, updates book availability, and provides search functionalities for books and members. Implementation may involve databases for storing book and member information, as well as transactions for borrowing and returning books.
Error handling includes managing book availability (preventing over-borrowing), handling membership renewals, and ensuring data integrity in the library database. This project enhances skills in database management, transaction processing, user authentication, and designing systems for efficient book management and member services.
Source Code: Click Here
An Inventory Management System tracks items in stock, allows the addition or removal of items, and manages inventory levels. It includes functionalities for adding new items to the inventory, updating item quantities, generating reports on stock levels, and handling orders or shipments. Error handling ensures accurate inventory updates and prevents stock discrepancies.
This project enhances skills in data structures (for managing item information), file handling or database management (for storing inventory data), and algorithm design for efficient inventory operations. It also emphasises user interface design for intuitive management of stock levels and inventory transactions.
Source Code: Click Here
A Chess Game project implements a two-player chess game with basic rules. Players take turns moving pieces on a chessboard, following standard chess rules for movement and capturing. The game manages piece positions, checks for legal moves, validates player inputs and detects checkmate or stalemate conditions. Features may include move validation, piece promotion, casting, and en passant. Error handling ensures that only valid moves are accepted and prevents illegal board states.
This project enhances skills in object-oriented programming (OOP) by modelling chess pieces and the board, algorithm design (for move validation and game logic), and graphical user interface (GUI) design for displaying the chessboard and capturing user inputs.
Source Code: Click Here
A Flight Reservation System manages flights, bookings, and passenger details. It allows users to search for flights based on criteria like destination and date, book available seats, manage passenger information, and generate booking confirmations. The system handles seat availability, updates passenger manifests, and manages flight schedules. Implementation may involve databases for storing flight and booking data, as well as algorithms for seat allocation and booking validations.
Error handling includes managing overbooking situations, handling cancellations or reschedules, and ensuring data consistency in flight and booking records. This project enhances skills in database design and management, transaction processing, user interface design for flight searches and bookings, and system scalability for handling multiple concurrent users and flight operations.
Source Code: Click Here
A Simple Paint Application offers basic drawing tools such as pencil, shapes (like lines, rectangles, circles), and colour selection. Users can draw on a canvas, select different drawing tools, adjust line thickness or fill colours, and save or export their drawings. The application manages drawing operations through mouse or keyboard inputs and updates the canvas in real time.
Error handling ensures smooth drawing operations and prevents crashes due to invalid drawing inputs or canvas manipulations. This project enhances skills in graphical user interface (GUI) programming, event-driven programming (for handling user inputs), algorithms for drawing shapes and managing colours, and file handling for saving or exporting drawings in various formats.
Source Code: Click Here
An Employee Payroll System calculates salaries, deductions, and bonuses for employees. It allows users to input employee information, such as hours worked, hourly rates, and deductions (like taxes or insurance). The system computes gross pay, deducts taxes and other withholdings, and calculates net pay for each employee. Features may include generating pay stubs, managing employee records, and generating payroll reports.
Error handling ensures accurate payroll calculations and prevents discrepancies in pay amounts or tax withholdings. This project enhances skills in mathematical computations (for payroll calculations), data validation (for employee inputs), file handling (for storing payroll records), and report generation for summarising payroll data.
Source Code: Click Here
A Tic-Tac-Toe project is a two-player console game where players take turns marking spaces in a 3x3 grid to achieve a line of their symbol (X or O). The game includes features like checking for win conditions, handling ties (draws), and optionally implementing a basic AI for single-player mode. Players input their moves through the console, and the game updates the grid and checks for win conditions after each move.
Error handling ensures valid player inputs (like selecting an occupied space) and prevents illegal moves. This project enhances skills in array manipulation (for managing the game board), algorithm design (for checking win conditions and implementing AI), and user interaction design for console-based gameplay.
Source Code: Click Here
An ATM Simulator project simulates ATM functionalities such as balance inquiry, withdrawal, deposit, and account transfers. Users interact with the ATM through a console interface, inputting PINs and selecting operations like checking their balance or withdrawing cash. The simulator manages account data, validates PIN inputs, and updates account balances based on transaction results.
Error handling ensures secure transactions (like preventing overdrafts or invalid withdrawals) and manages unexpected scenarios (like network errors or card retention). This project enhances skills in state machine design (for managing ATM states), transaction processing (for updating account balances), user authentication, and error handling for handling various transaction outcomes securely.
Source Code: Click Here
A Basic Music Player project allows users to play, pause, and stop music tracks through a console or graphical interface. Users can select music files from a library, control playback (play, pause, stop), adjust volume, and implement features like playlists or shuffle modes. The player manages audio playback through system libraries or APIs, handles user inputs for playback controls, and provides feedback on current track status. Error handling ensures smooth playback operations and prevents crashes due to unsupported audio formats or file corruption.
This project enhances skills in multimedia programming (for audio playback), user interface design (for music controls), file handling (for managing music files), and event-driven programming (for handling user interactions and playback events).These projects cover a range of applications and complexities, providing practical experience in various aspects of software development using C++. They are excellent for learning core programming concepts and applying them to real-world scenarios.
Source Code: Click Here
Step into advanced C++ programming with these challenging project ideas. These projects are tailored for experienced programmers looking to tackle complex algorithms, optimize performance, and build scalable applications. You'll explore advanced topics such as concurrency, networking, memory management, and optimization techniques.
Each project provides an opportunity to push the boundaries of your C++ skills, from designing high-performance data structures to implementing sophisticated algorithms and integrating with external libraries and APIs. Whether you're aiming to deepen your expertise or tackle real-world engineering problems, these projects will challenge and elevate your proficiency in C++.
A Chat Application project involves implementing a simple peer-to-peer (P2P) chat system where users can connect over a network and exchange messages in real time. The application manages user connections, message routing, and user interface for sending/receiving messages. Features may include user authentication, private messaging, and group chat functionalities.
Error handling ensures reliable message delivery and manages network failures or unexpected user actions. This project enhances skills in network programming (for socket communication), multi-threading (for handling multiple connections simultaneously), user interface design (for chat windows and message display), and event-driven programming (for handling incoming messages and user interactions).
Source Code: Click Here
An Online Bookstore project creates a web-based platform where users can browse, search, and purchase books. It includes functionalities for managing book categories, displaying book details (like title, author, price), adding books to a shopping cart, and processing orders. The system manages user accounts, handles payments securely, and updates inventory levels upon purchase.
Implementation involves front-end development (HTML, CSS, JavaScript) for the user interface, back-end development (using frameworks like Django, Flask, or Node.js) for server-side logic, and database management (SQL or NoSQL) for storing book and user data. Error handling ensures transaction security, prevents duplicate purchases, and manages stock availability. This project enhances skills in web development, database integration, secure payment processing, and user experience design for e-commerce applications.
Source Code: Click Here
A Compiler for a Simple Language project involves designing and implementing a compiler or interpreter for a basic programming language. The compiler translates source code written in the language into executable machine code or interprets it directly. It includes lexical analysis (tokenisation), syntax parsing (using techniques like recursive descent parsing), semantic analysis (type checking), code generation, and optionally, optimisation phases.
Error handling ensures that the compiler detects and reports syntax errors, semantic errors, and other issues in the source code. This project enhances skills in language design, compiler construction (implementing compiler phases), data structures (for symbol tables and abstract syntax trees), and algorithm design (for parsing and code generation). It also provides insight into how programming languages are processed and executed by computers.
Source Code: Click Here
A Database Management System (DBMS) project involves creating a basic system that manages structured data with SQL-like commands. The DBMS allows users to create and manage databases, define tables and relationships, and insert, update, delete, and query data using SQL queries. It includes functionalities for data indexing, transaction management (commit and rollback), and concurrency control (for handling multiple users accessing data simultaneously).
An implementation may use file storage or a simple database engine for data persistence. Error handling ensures data integrity and manages scenarios like concurrent transactions or query optimisation. This project enhances skills in database design, SQL query processing, transaction management, file handling, and concurrency control mechanisms.
Source Code: Click Here
A Graphical Calculator project builds a calculator application with a graphical user interface (GUI) where users can perform arithmetic operations using buttons and display results in a graphical window. The calculator supports basic operations like addition, subtraction, multiplication, and division, and may include advanced functions like square root, exponentiation, and trigonometric functions. Error handling ensures that mathematical operations are performed correctly and manages input validation to prevent invalid calculations.
This project enhances skills in GUI programming (using libraries like Qt, GTK, or Java Swing), event-driven programming (handling button clicks and input events), mathematical operations in programming, and user interface design for interactive applications.
Source Code: Click Here
A Stock Market Simulator project simulates buying and selling stocks in a virtual market environment. Users can create portfolios, browse stock listings, place buy/sell orders, and track portfolio performance with real-time or historical data. The simulator may include features like stock price updates, market trends, and portfolio analytics (like profit/loss calculations and performance metrics).
Implementation involves integrating with financial APIs for real-time market data, database management for storing user portfolios and transactions, and front-end development for user interaction. Error handling ensures accurate transaction processing, prevents invalid orders, and manages data synchronization with market updates. This project enhances skills in financial markets, API integration, database management, real-time data processing, and user interface design for financial applications.
Source Code: Click Here
A Racing Game project creates a 2D racing game where players compete on tracks with vehicles. The game includes features like vehicle selection, track selection, racing against AI or other players (multiplayer), scoring based on race performance, and possibly power-ups or obstacles on tracks. It manages game physics (like vehicle movement and collision detection), game state (like lap counts and race positions), and user interface for displaying race information and controls.
Error handling ensures smooth gameplay and manages unexpected events like collisions or track boundary violations. This project enhances skills in game development (using frameworks like Unity or SDL), physics simulation, AI programming (for opponent vehicles), multiplayer networking (if multiplayer is implemented), and user experience design for gaming applications.
Source Code: Click Here
An Image Editor project offers basic image editing functions like cropping, rotating, applying filters (like grayscale or sepia), and adjusting brightness/contrast. Users can open image files, perform edits, and save modified images. The editor manages image data manipulation, user inputs for editing operations, and updates to the image display.
Error handling ensures that image files are loaded correctly, edits are applied without corrupting image data and saves preserve image quality. This project enhances skills in image processing algorithms, file handling (for image I/O), graphical user interface (GUI) programming, and algorithm optimisation for efficient image manipulation operations.
Source Code: Click Here
A Remote Desktop Application project allows users to share their desktop screens over a network connection. It includes functionalities for remote desktop viewing, mouse and keyboard input forwarding, and file transfer between connected systems. The application manages network communication, screen capturing, and user authentication for secure connections.
Error handling ensures reliable screen sharing and prevents unauthorised access or data leaks. This project enhances skills in network programming (for socket communication), graphical desktop interaction (for screen capturing and input forwarding), security protocols (for data encryption and authentication), and user interface design for remote access applications.
Source Code: Click Here
A Virtual Memory Manager project simulates virtual memory operations like paging in an operating system environment. It manages memory allocation and paging mechanisms to optimise memory usage for running processes. The manager includes functionalities for page allocation, page replacement algorithms (like LRU or FIFO), and handling page faults (when required pages are not in memory). Implementation involves simulating memory structures, managing page tables, and monitoring process memory usage.
Error handling ensures efficient memory management, prevents memory leaks or thrashing, and manages process interruptions due to memory access violations. This project enhances skills in operating system concepts (like virtual memory management), data structures (for managing memory mappings), algorithm design (for page replacement policies), and system performance optimisation for running applications.
Source Code: Click Here
Embark on expert-level C++ projects designed to push the boundaries of your programming skills and knowledge. These projects are tailored for seasoned developers seeking to master advanced concepts and solve complex engineering challenges. You'll tackle topics such as parallel computing, advanced data structures, low-level optimizations, and large-scale system design.
Each project offers an opportunity to demonstrate mastery in C++ by implementing cutting-edge solutions, optimizing performance, and handling intricate technical requirements. Whether you're aiming to innovate in software development or contribute to high-impact projects, these challenges will stretch your abilities and showcase your expertise in C++.
Building a basic OS kernel with process management involves creating fundamental components of an operating system. This includes implementing process scheduling, memory management, interrupt handling and basic file system operations. The kernel interacts directly with hardware and manages system resources to provide a platform for running applications.
Error handling and robust design are crucial to ensure the stability and reliability of the OS. This project enhances skills in systems programming, understanding computer architecture, concurrency management, and low-level programming (possibly in C or assembly). It provides deep insight into how operating systems manage hardware resources and execute processes.
Source Code: Click Here
Creating a simple blockchain involves designing and implementing the core components of a decentralised ledger. This includes building blocks, a consensus mechanism (like Proof of Work or Proof of Stake), transaction validation, and possibly a peer-to-peer network for communication between nodes. The blockchain should support adding new blocks to the chain, validating the integrity of the blockchain, and handling conflicts or forks.
Error handling and security are critical to prevent tampering and ensure data consistency across the network. This project enhances skills in cryptography, distributed systems, network programming, and algorithm design. It also provides practical experience in understanding blockchain technology and its application in decentralised applications (dApps).
Source Code: Click Here
Developing a multiplayer real-time strategy (RTS) game involves creating game mechanics like resource management, unit control, strategy planning, and possibly combat simulation. The game runs in real time, requiring efficient networking for multiplayer interactions and synchronisation. Features may include AI for computer-controlled opponents, game balance, and user interface design for displaying game elements and controls.
Error handling ensures smooth gameplay and manages synchronization issues between players. This project enhances skills in game development (using game engines like Unity or custom engines), multiplayer networking, AI programming, graphical user interface (GUI) design, and real-time simulation.
Source Code: Click Here
Implementing a neural network library involves creating a framework for designing, training, and testing neural networks. The library should support different types of neural networks (like feedforward, convolutional, recurrent), activation functions, loss functions, and optimization algorithms (like gradient descent). It includes functionalities for forward and backward propagation, weight updates, and model evaluation.
Error handling ensures numerical stability and prevents divergence during training. This project enhances skills in machine learning algorithms, deep learning concepts, numerical computing (using libraries like NumPy), and software library design. It provides practical experience in implementing state-of-the-art neural networks and understanding their applications in various domains.
Source Code: Click Here
Designing and implementing a distributed file system (DFS) involves creating a system for storing and accessing files across multiple nodes in a network. The DFS should handle file distribution, replication for fault tolerance, file access permissions, and possibly caching for performance optimization. It includes components for metadata management, data placement strategies, and synchronization across nodes.
Error handling ensures data consistency and fault tolerance in distributed environments. This project enhances skills in distributed systems, network programming, data replication strategies, concurrency control, and fault-tolerant design. It provides insight into challenges faced in large-scale distributed storage systems.
Source Code: Click Here
Building a basic 3D rendering engine involves creating software capable of rendering three-dimensional scenes from geometric models and textures. The engine should support rendering techniques like rasterisation or ray tracing, handling lighting and shading effects, camera controls, and possibly physics simulation for interactions in the 3D environment.
It includes optimisations for real-time rendering and user interface components for interacting with the scene. Error handling ensures visual correctness and performance optimisation during rendering. This project enhances skills in computer graphics algorithms, GPU programming (using APIs like OpenGL or DirectX), mathematics (for transformations and projections), and software architecture for managing complex rendering pipelines.
Source Code: Click Here
Implementing optimisation passes for a compiler involves enhancing the efficiency and performance of generated code. This includes techniques like constant folding, loop optimisation, function inlining, and register allocation. Optimisation passes analyze intermediate representations of code to transform and improve its execution speed or memory usage.
Error handling ensures that optimisations preserve program semantics and correctness. This project enhances skills in compiler construction, intermediate representation (IR) design, algorithm design for optimisation techniques, and understanding performance trade-offs in software execution. It provides practical experience in enhancing compiler output for better runtime efficiency of programs.
Source Code: Click Here
Creating a voice recognition system involves developing software that can accurately recognize and interpret spoken commands or phrases. The system should include components for audio input processing, feature extraction, pattern recognition using machine learning models (like Hidden Markov Models or deep learning models), and natural language understanding for interpreting commands.
Error handling ensures accurate speech recognition and robust performance across different speakers and environments. This project enhances skills in signal processing, machine learning algorithms for speech recognition, software integration of audio processing libraries, and user interface design for voice-controlled applications.
Source Code: Click Here
Building a network packet sniffer involves creating software that captures and analyzes network packets passing through a network interface. The sniffer decodes packet headers, extracts payload data, and analyzes protocols like TCP/IP or UDP. It may include features for filtering packets based on criteria, displaying packet information in real-time, and possibly recording packet traces for later analysis.
Error handling ensures packet integrity and manages unexpected packet formats or network errors. This project enhances skills in network programming, protocol analysis, low-level packet processing (using libraries like libpcap), data visualization, and cybersecurity concepts related to network traffic monitoring.
Source Code: Click Here
Implementing a framework for genetic algorithms involves creating software that can simulate evolutionary processes for solving optimization problems. The simulator includes components for defining genetic representations (like chromosomes), fitness evaluation functions, selection mechanisms (like roulette wheel or tournament selection), crossover and mutation operators, and termination criteria.
It may support parallel processing for evaluating multiple solutions simultaneously. Error handling ensures genetic diversity and prevents premature convergence in the optimization process. This project enhances skills in evolutionary computation, algorithm design, parallel computing (for performance optimization), and problem-solving using heuristic search methods.
Source Code: Click Here
Dive into a variety of miscellaneous C++ projects that offer unique challenges and opportunities for exploration. These projects span different domains and levels of complexity, providing diverse avenues to apply and expand your C++ skills. From niche applications to creative implementations, each project presents an opportunity to delve into specialized areas of interest or experiment with unconventional uses of C++.
Whether you're looking to broaden your portfolio, explore new technologies, or pursue a passion project, these miscellaneous ideas offer a range of exciting opportunities to deepen your understanding and proficiency in C++.
An Encryption/Decryption Tool project involves implementing various encryption algorithms (like AES, RSA, or DES) for securing data and decrypting it back to its original form. The tool should handle key management, encryption modes (like ECB or CBC), and padding schemes.
Error handling ensures secure encryption and decryption processes, preventing data loss or corruption due to invalid inputs or algorithm failures. This project enhances skills in cryptography, algorithm implementation, data security practices, and software testing for validating encryption algorithms.
Source Code: Click Here
Building a Data Compression Tool involves creating software that can compress files to reduce storage space and decompress them back to their original form. The tool implements compression algorithms like Huffman coding, LZ77, or LZ78, along with methods for handling file metadata and managing compressed data efficiently.
Error handling ensures data integrity during compression and prevents data loss during decompression. This project enhances skills in algorithm design, data structures for efficient data compression, file handling (for reading/writing compressed files), and understanding trade-offs between compression ratio and compression speed.
Source Code: Click Here
Creating a Code Editor with Syntax Highlighting involves developing a text editor tailored for editing code files with features like syntax coloring, code folding, and auto-indentation. The editor supports multiple programming languages and enhances the coding experience with features like error highlighting and intelligent code completion.
It may include integration with compilers or interpreters for running code directly from the editor. Error handling ensures proper syntax highlighting and manages unexpected inputs or editor interactions. This project enhances skills in graphical user interface (GUI) programming, text processing algorithms, language parsing (for syntax highlighting), and integration with external tools for software development.
Source Code: Click Here
An Online Quiz Platform project creates a web-based platform for conducting quizzes with features like user registration, quiz creation, timer management, scoring, and result tracking. The platform supports different question types (like multiple-choice or short answer), allows quiz customization (like difficulty levels or categories), and provides real-time feedback to users.
Error handling ensures reliable quiz sessions and manages unexpected user inputs or system failures. This project enhances skills in web development (using frameworks like Django, Flask, or Node.js), database design (for storing quiz data and user results), user authentication, and scalable web application architecture for handling concurrent quiz sessions.
Source Code: Click Here
Building a Video Streaming Server involves creating software that can stream video content over a network using protocols like RTSP or HTTP. The server manages video encoding, network transmission, and client interactions for seamless playback. Features may include adaptive bitrate streaming, real-time transcoding, and support for different media formats.
Error handling ensures smooth video playback and manages network congestion or client-side buffering issues. This project enhances skills in multimedia streaming technologies, network programming (using sockets or streaming protocols), video encoding algorithms, and server-side optimizations for delivering high-quality video content.
Source Code: Click Here
Implementing a Digital Signal Processing (DSP) Tool involves creating software that can analyze and process digital signals using algorithms like Fourier transforms, filters (like low-pass or high-pass filters), and noise reduction techniques.
The tool may include features for visualizing signal data, applying transformations, and performing spectral analysis. Error handling ensures accurate signal processing results and manages data overflow or underflow during computations. This project enhances skills in signal processing algorithms, numerical computing (using libraries like NumPy or MATLAB), algorithm optimization for real-time processing, and graphical representation of signal data.
Source Code: Click Here
Developing an IoT-based Home Automation System involves creating a network of IoT devices that can control home appliances like lights, HVAC systems, or security cameras. The system includes components for device communication (using protocols like MQTT or CoAP), sensor data processing, and user interface for remote control via mobile apps or web interfaces.
Features may include scheduling tasks, monitoring energy consumption, and integrating with voice assistants. Error handling ensures reliable device communication and prevents unauthorized access to home automation controls. This project enhances skills in IoT device integration, wireless communication protocols, cloud computing (for data storage and processing), and user experience design for smart home applications.
Source Code: Click Here
A Recipe Management System project manages recipes, ingredients, and meal planning for users. The system allows users to create, search, and categorize recipes, manage ingredient lists, and plan meals based on dietary preferences or nutritional goals. It includes features for ingredient substitution, recipe scaling, and generating shopping lists.
Error handling ensures accurate recipe calculations and manages user inputs for ingredient quantities or preparation steps. This project enhances skills in database design (for storing recipe and ingredient data), user interface design for recipe browsing and editing, algorithm design (for meal planning and ingredient substitution), and understanding nutrition and culinary concepts.
Source Code: Click Here
Creating an E-commerce Platform involves developing a web-based platform for online shopping with features like product listings, shopping cart management, secure payments, order processing, and user reviews. The platform supports user registration, product search, and personalized recommendations based on user behavior. It includes administrative tools for managing inventory, orders, and customer support.
Error handling ensures transaction security and manages order fulfillment errors or payment failures. This project enhances skills in full-stack web development (using frameworks like Django, Ruby on Rails, or ASP.NET), secure payment integration (using APIs like PayPal or Stripe), database management for storing product and order data, and scalable architecture for handling concurrent user sessions and transactions.
Source Code: Click Here
Building a Peer-to-Peer (P2P) File Sharing System involves creating software that allows users to share files directly between devices without a central server. The system manages file discovery, peer-to-peer communication (using protocols like BitTorrent or IPFS), and file integrity verification.
It includes features for bandwidth management, download/upload tracking, and peer authentication for secure file transfers. Error handling ensures data integrity during file sharing and manages network errors or peer disconnections. This project enhances skills in network programming (for P2P communication), distributed systems design, algorithm design (for file chunking and reassembly), and understanding decentralized file sharing protocols.
Source Code: Click Here
Delve into the world of graphical user interfaces (GUIs) with these engaging C++ project ideas. These projects focus on creating interactive applications with visual components, enhancing user experience and usability.
Whether you're interested in game development, multimedia applications, or productivity tools, these GUI-based projects offer a hands-on approach to mastering C++ while building practical software solutions.
Implementing a Chess Game with a graphical user interface (GUI) involves creating a software application where players can interact with a virtual chessboard and play against each other or against a computer AI. The GUI displays the chessboard, pieces, and allows players to make moves by clicking and dragging pieces. Features may include legal move validation, check/checkmate detection, piece promotion, and support for different game modes (like standard chess or chess variants).
Error handling ensures valid game states and manages unexpected player actions. This project enhances skills in GUI programming (using libraries like JavaFX, PyQt, or Tkinter), algorithm design (for chess rules and AI strategies), user interface design for game interactions, and multiplayer networking (if implementing online play).
Source Code: Click Here
Creating a Music Library Organizer involves developing a GUI application for managing a collection of music tracks. The organizer allows users to add, edit, and delete music entries, categorize tracks by genres or playlists, and perform searches or filters based on metadata like artist, album, or release year. It includes features for playing music tracks, creating custom playlists, and possibly integrating with online music databases for automatic metadata retrieval.
Error handling ensures data consistency and manages file I/O operations for music file management. This project enhances skills in GUI design for media applications, database management (for storing music metadata), multimedia playback integration, and user experience design for music enthusiasts.
Source Code: Click Here
Developing a Weather App involves creating a GUI application that displays current weather conditions and forecasts for a specified location using weather APIs. The app fetches data from a weather service provider, parses JSON or XML responses, and displays weather information such as temperature, humidity, wind speed, and precipitation.
It may include features for displaying weather maps, hourly forecasts, or weather alerts. Error handling ensures reliable data retrieval and manages API request errors or network connectivity issues. This project enhances skills in GUI development for data-driven applications, API integration (for fetching weather data), data parsing and formatting, and real-time data visualization for weather updates.
Source Code: Click Here
A Calendar Application project involves developing a GUI-based calendar tool that allows users to manage events, appointments, and reminders. The application displays a calendar interface with navigation controls for switching between months or years, highlights event dates, and allows users to add, edit, or delete events. It includes features for setting reminders, recurring events, and integrating with other calendar services (like Google Calendar or Outlook).
Error handling ensures data consistency and manages calendar conflicts or reminder notifications. This project enhances skills in GUI programming (using frameworks like JavaFX, Qt, or Cocoa), date and time handling, event management systems, and synchronization with online calendar services.
Source Code: Click Here
Building a Drawing Application involves creating a GUI-based tool for digital drawing and painting. The application provides a canvas area where users can draw with different brushes, colors, and drawing tools (like pencils, pens, shapes, and erasers). It includes features for undo/redo operations, layer management, and exporting drawings in various formats (like PNG or JPEG). Error handling ensures smooth drawing interactions and manages user input for accurate rendering of strokes and shapes.
This project enhances skills in GUI programming (using frameworks like Qt, HTML5 Canvas, or JavaFX), graphics rendering algorithms, user interface design for creative tools, and interactive application development for artists and designers.
Source Code: Click Here
Developing a Personal Finance Tracker involves creating a GUI application for managing income, expenses, and budgeting. The tracker allows users to categorise transactions, set budget goals, and generate reports or visualisations (like pie charts or bar graphs) to track financial health over time. It includes features for importing bank statements or receipts, setting up recurring transactions, and integrating with financial APIs for real-time balance updates.
Error handling ensures accurate financial calculations and manages data synchronisation with external financial services. This project enhances skills in GUI development for financial applications, database management (for storing transaction data securely), financial analysis algorithms, and personal finance management principles.
Source Code: Click Here
Implementing a Video Player with a Playlist involves creating a GUI application that allows users to play and manage a collection of video files. The player supports common video formats and includes features for playback controls (like play, pause, stop, and seek), fullscreen mode, and volume adjustment. It also allows users to create and manage playlists, add videos to the playlist, and save playlists for later use.
Error handling ensures smooth video playback and manages codec compatibility issues or corrupted video files. This project enhances skills in multimedia application development, GUI design for media players, video processing algorithms, and user interface design for media consumption.
Source Code: Click Here
Creating a Task Scheduler involves developing a GUI application for scheduling tasks, reminders, and appointments. The scheduler allows users to create new tasks, set due dates and times, prioritize tasks, and receive notifications or alerts when tasks are due. It includes features for recurring tasks, task categories, and possibly integrating with calendar applications or productivity tools. Error handling ensures reliable task scheduling and manages notifications for overdue tasks or missed reminders.
This project enhances skills in GUI programming (using frameworks like JavaFX, Tkinter, or Electron), event-driven programming for handling user inputs and system notifications, database integration (for storing task data), and usability design for productivity applications.
Source Code: Click Here
C++ projects offer several advantages, making it a preferred choice for various applications:
1. Efficiency: C++ allows for close-to-the-hardware programming, making it highly efficient in terms of speed and memory usage. This efficiency is crucial for applications requiring high performance, such as games, real-time systems, and embedded systems.
2. Portability: C++ programs can be compiled to run on different platforms with minimal changes, thanks to its standardized language features and compilers available on most operating systems.
3. Scalability: C++ supports both procedural and object-oriented programming paradigms, enabling developers to build scalable applications from small utilities to large-scale systems.
4. Control: With C++, developers have fine-grained control over system resources and memory management, allowing them to optimize code for specific hardware or performance requirements.
5. Compatibility: C++ has a long-standing compatibility with C, allowing seamless integration with existing C libraries and systems, which is advantageous for leveraging legacy codebases.
6. Flexibility: C++ offers a wide range of features such as templates, operator overloading, and multiple inheritance, providing developers with the flexibility to implement complex algorithms and data structures efficiently.
7. Community and Support: C++ has a large and active community of developers, libraries, and frameworks, providing ample resources, documentation, and support for building robust applications.
8. Industry Adoption: Many critical systems and applications in industries such as finance, gaming, telecommunications, and aerospace are built using C++, showcasing its reliability and suitability for mission-critical applications.
C++ projects offer a robust platform for developing high-performance applications across diverse domains. With its emphasis on efficiency, portability, and control over system resources, C++ enables developers to create scalable solutions ranging from small utilities to complex systems. Its compatibility with C, flexibility in programming paradigms, and extensive community support further enhance its appeal in industries requiring reliable and efficient software solutions.
Mastery of C++ not only provides developers with the tools to optimize performance and manage resources effectively but also opens doors to career opportunities in fields where performance and reliability are critical. Whether you're building games, embedded systems, financial applications, or multimedia tools, C++ remains a powerful choice for developers aiming to push the boundaries of software engineering.
Copy and paste below code to page Head section
Simple Calculator, To-Do List Application, Contact Management System, Basic Game (like Tic-Tac-Toe), and Text-based RPG are great starting points.
Music Player with GUI, Weather App, Inventory Management System, Quiz Game with database integration, and Online Shopping System are suitable for intermediate programmers.
Develop a Concurrent Web Server, Implement a Compiler or Interpreter for a custom language, Build a Real-time Strategy Game Engine, Design an Automated Trading System, or Create a Neural Network Library.
C++ offers high performance, control over hardware resources, compatibility with C, support for both procedural and object-oriented programming, and a large community with extensive libraries and frameworks.
Start with small projects to grasp basic concepts, gradually move to more complex projects that challenge your understanding of data structures, algorithms, and design patterns. Utilize resources like online tutorials, forums, and documentation to deepen your knowledge.
GUI projects enhance your understanding of event-driven programming, user interface design, and integrating external libraries. They also make applications more user-friendly and visually appealing.