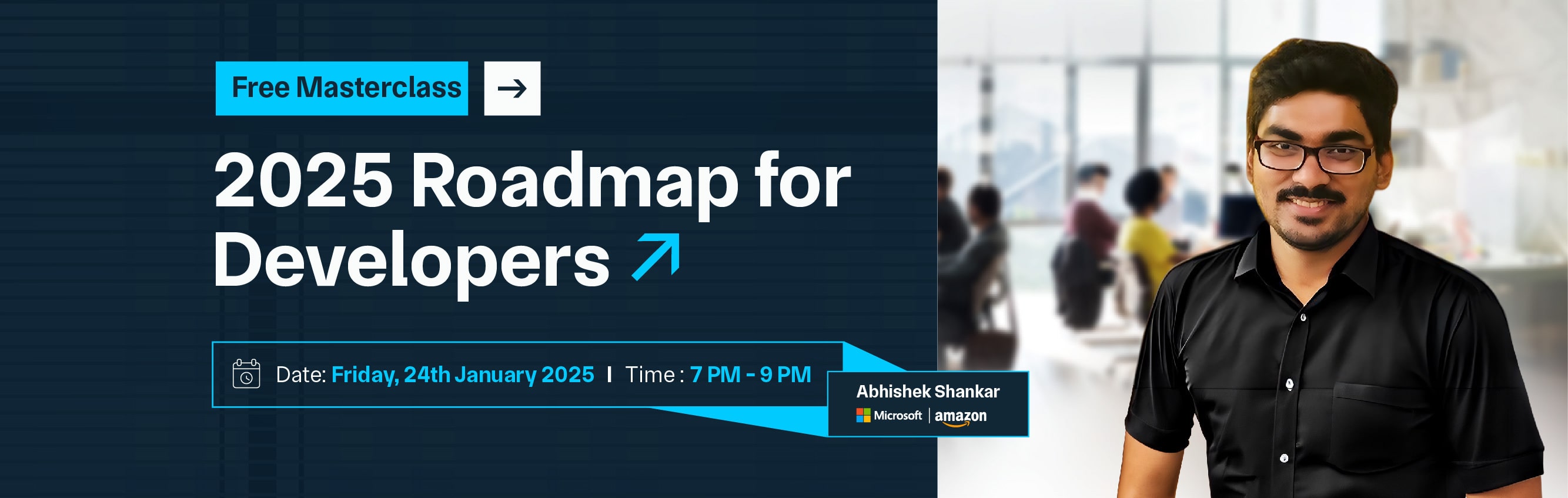

Backend tools are essential for developers building and maintaining web applications, as they power the server-side operations, handle data management, and ensure security and performance. These tools can be categorized into various types, including frameworks, databases, API management, security solutions, and deployment tools. Web frameworks like Node.js, Django, and Ruby on Rails provide the necessary structure to build scalable, efficient applications, simplifying tasks like routing, authentication, and database management.
For data storage, relational databases (such as MySQL and PostgreSQL) offer robust, structured data storage, while NoSQL databases like MongoDB cater to applications requiring flexibility in data modeling. API tools like RESTful APIs, and GraphQL enable communication between the frontend and backend, while API management tools such as Kong and Amazon API Gateway handle scalability and security concerns.
Security is a major consideration, with tools like OAuth, JWT, and SSL/TLS encryption ensuring safe communication and protecting user data. For hosting and deployment, cloud platforms like AWS, Google Cloud, and Heroku offer scalable infrastructure, while Docker and Kubernetes facilitate containerization and orchestration for easier management of microservices. Ultimately, selecting the right combination of backend tools depends on the application’s specific needs, including scalability, security, and performance.
Backend development refers to the server-side part of web development, focusing on how the application works behind the scenes. Unlike frontend development, which deals with the visual aspects that users interact with directly, backend development is responsible for handling the logic, database interactions, authentication, and other essential components that ensure an application functions properly.
The backend is made up of a combination of technologies, including server, database, and application logic. It typically involves three main components:
Backend development ensures that all the components of an application work together smoothly, handling data processing, user requests, and server management behind the scenes to provide a seamless experience for users.
When building a backend for a web application, developers rely on a variety of tools that span several essential categories. Each category addresses different aspects of backend development, from data management to application performance and security. Here are the essential categories of backend tools:
Web frameworks are essential for backend development as they provide a structured environment for building web applications. They offer libraries, tools, and conventions to streamline common backend tasks, such as routing requests, managing databases, and handling authentication. Popular frameworks like Node.js (with Express, Koa, and NestJS) allow JavaScript developers to build fast and scalable applications.
Django, a Python-based framework, is known for its "batteries-included" approach, providing built-in functionalities like admin panels and authentication. Ruby on Rails simplifies backend development with its focus on convention over configuration, while Laravel offers an elegant, feature-rich framework for PHP developers. These frameworks help developers save time and reduce boilerplate code while providing a robust foundation for their backend systems.
Databases are the backbone of backend systems, storing, retrieving, and managing the data used by applications. Relational databases, such as MySQL and PostgreSQL, are widely used for their structured data storage and support for complex queries. These databases organize data into tables with predefined schemas, ensuring consistency and integrity.
On the other hand, NoSQL databases like MongoDB are more flexible, allowing unstructured data storage, which is beneficial for applications that handle large amounts of varied data. Redis, a key-value store, is often used for caching and managing temporary data, improving performance by reducing database load. Choosing the right type of database depends on the application’s needs, such as scalability, data structure, and query complexity.
APIs (Application Programming Interfaces) are the communication layer between the front and backend of a web application, allowing them to exchange data and interact with external services. RESTful APIs are a standard for building APIs, enabling operations like retrieving, creating, updating, and deleting data using HTTP methods.
GraphQL, on the other hand, provides more flexibility by allowing clients to request only the data they need, reducing unnecessary data transfers. API management tools like Kong and AWS API Gateway help developers secure, monitor, and scale APIs, ensuring high availability and consistent performance. API management platforms also offer features like rate limiting, authentication, and logging, which are crucial for maintaining API security and preventing misuse.
Hosting and server management tools provide the infrastructure needed to deploy and run backend applications. Cloud hosting services like AWS, Google Cloud, and Microsoft Azure offer scalable infrastructure, allowing developers to deploy applications on virtual servers and manage databases easily. These cloud platforms also provide additional services such as storage (S3, Google Cloud Storage) and managed databases (Amazon RDS, Azure SQL).
VPS hosting, through providers like DigitalOcean and Linode, gives developers more control over the server environment at a lower cost, though it requires more technical expertise. For applications using containers, Docker simplifies the process of packaging and deploying apps consistently across various environments. At the same time, Kubernetes automates container orchestration, making it easier to scale and manage microservices-based architectures.
Security is a critical aspect of backend development, as it ensures that data is protected and applications remain resilient to attacks. Authentication and authorization protocols like OAuth and JWT (JSON Web Tokens) are used to verify users and control their access to resources. SSL/TLS encryption ensures that data transmitted between the client and server is secure, preventing man-in-the-middle attacks.
Web Application Firewalls (WAFs) like Cloudflare and AWS WAF protect applications from common attacks such as SQL injection, cross-site scripting (XSS), and DDoS (Distributed Denial of Service). Developers can also use tools like OWASP ZAP to perform security testing and identify vulnerabilities in their applications. By integrating these security tools, developers can ensure that their applications meet industry standards for data privacy and integrity.
Development tools help streamline the process of building, testing, and deploying backend applications. Integrated Development Environments (IDEs) like Visual Studio Code and PyCharm provide a rich environment with code editing, debugging, and testing features tailored to backend languages and frameworks. Version control systems like Git track changes in the source code, allowing multiple developers to collaborate on the same project without overwriting each other’s work.
Platforms like GitHub, GitLab, and Bitbucket host Git repositories and offer collaborative features such as pull requests, issue tracking, and CI/CD pipelines. Continuous Integration (CI) and Continuous Deployment (CD) tools like Jenkins, CircleCI, and GitLab CI automate testing and deployment processes, helping teams deliver code updates quickly and reliably.
Monitoring tools are vital for maintaining the health and performance of backend systems in production environments. Application Performance Monitoring (APM) tools like Datadog and New Relic provide real-time insights into the performance of applications, helping developers detect bottlenecks, errors, or slow transactions. Log management tools, such as the ELK Stack (Elasticsearch, Logstash, Kibana), aggregate and visualize logs, making it easier to analyze system events, troubleshoot issues, and track application behavior over time.
Error tracking platforms like Sentry and Rollbar capture runtime errors and exceptions, enabling quick response and resolution of issues. These monitoring tools help ensure that applications are running smoothly and that potential problems are identified and addressed before they impact users.
Caching is an optimization technique used to store frequently accessed data in memory to reduce the load on databases and improve application performance. Redis is one of the most popular caching tools, offering fast, in-memory data storage that can be used to cache query results, session data, or other frequently accessed information.
Memcached is another in-memory caching system often used for storing key-value pairs and reducing the number of database queries. By using caching tools, backend developers can dramatically improve the response time of applications, reduce latency, and decrease database load, resulting in a smoother user experience, especially for high-traffic applications.
Here’s a list of 25 essential backend tools that are widely used in modern web development. These tools span across various categories like frameworks, databases, API management, security, hosting, development, and monitoring.
Node.js is a JavaScript runtime built on Chrome's V8 engine that enables backend development using JavaScript. It’s highly efficient for handling asynchronous operations and is especially suited for I/O-heavy applications like real-time chat applications or APIs. Node.js supports event-driven, non-blocking architecture, making it fast and scalable, which is why it’s popular for microservices and large-scale applications.
Express.js is a lightweight web framework for Node.js, providing an easy way to build and manage web servers and APIs. It simplifies tasks like routing, middleware integration, and handling HTTP requests. Express is highly flexible, allowing developers to structure their apps as needed. With a large ecosystem of plugins, it is an excellent choice for building RESTful APIs or web applications with minimal configuration.
Django is a high-level Python framework that promotes rapid development and clean, pragmatic design. It includes many built-in features, such as an admin interface, ORM for database management, and built-in security practices, which reduce development time. Django follows the "don't repeat yourself" (DRY) principle, which encourages efficient, reusable code, making it an excellent choice for building large, secure web applications quickly.
Ruby on Rails is a full-stack framework for Ruby, known for its "convention over configuration" philosophy, which speeds up the development process. Rails provides an extensive library of pre-built modules for common tasks like database management, authentication, and routing. With its active community and large number of gems (libraries), it’s great for building web apps with clean, maintainable code, especially for startups and rapid prototyping.
Laravel is a PHP framework designed to make backend development elegant and enjoyable. It offers a range of built-in tools for database management, routing, authentication, and security. Laravel's powerful ORM, Eloquent, makes working with databases straightforward, while its blade templating engine allows for efficient views. With features like queues, task scheduling, and a robust testing suite, Laravel is ideal for building modern, scalable web applications.
Spring Boot is a Java-based framework that simplifies the development of production-ready applications. It eliminates boilerplate code and provides built-in tools for configuring web servers, databases, and security. By leveraging Spring Boot, developers can quickly set up microservices architectures with minimal configuration. It’s commonly used for building enterprise-level, large-scale applications with complex business logic and high scalability requirements.
PostgreSQL is a powerful, open-source relational database known for its robustness and feature set. It supports advanced data types, such as JSON, and complex queries, making it suitable for applications with complex data relationships. PostgreSQL is highly extensible, allowing developers to create custom functions and manage large datasets efficiently. It’s ideal for applications requiring ACID compliance, data integrity, and scalability.
MySQL is one of the most popular open-source relational databases, known for its speed, reliability, and ease of use. It supports SQL queries for managing structured data and is widely used in web applications. MySQL provides strong transactional support and allows for high-performance operations in large databases, making it a popular choice for both small and large-scale applications, especially in e-commerce, social networks, and content management systems.
MongoDB is a NoSQL database designed for flexibility, scalability, and high performance. It stores data in JSON-like documents, making it a good fit for applications that deal with unstructured or semi-structured data. MongoDB excels in handling large volumes of data, real-time analytics, and distributed applications. It’s commonly used in scenarios where relational databases might be too rigid or inefficient, such as social media platforms or big data applications.
Redis is an open-source, in-memory key-value store often used for caching and session management. Its high performance makes it suitable for applications requiring fast data retrieval. Redis supports a wide range of data types, such as strings, lists, and sets, and can be used for real-time analytics, message queues, and task management. It's widely integrated into applications to improve response times and reduce the load on primary databases.
Apache Cassandra is a highly scalable, distributed NoSQL database designed for handling large amounts of data across many commodity servers. It offers high availability with no single point of failure, making it perfect for applications that require 24/7 uptime and massive scalability, such as IoT systems, social media platforms, and data-driven apps. Cassandra is optimized for write-heavy applications and excels in handling big data in distributed environments.
Neo4j is a graph database that stores data as nodes and relationships, making it ideal for applications that require complex querying of relationships, such as social networks, fraud detection systems, and recommendation engines. Neo4j provides a high level of flexibility and performance for querying connected data using a graph query language called Cypher. It's used in scenarios where data relationships are central to the application's functionality.
Docker is a platform for containerizing applications, enabling developers to package and deploy them in a consistent environment across different stages of development and production. Containers are lightweight, portable, and fast, ensuring that an application behaves the same regardless of where it's deployed. Docker simplifies the management of microservices architectures, reduces setup time, and helps with scaling applications across cloud platforms and on-premise environments.
Kubernetes is an open-source container orchestration platform that automates the deployment, scaling, and management of containerized applications. It is designed to manage complex, microservices-based applications by providing features like load balancing, self-healing, and automatic scaling. Kubernetes allows developers to abstract infrastructure complexities and efficiently manage containers in large-scale environments, making it essential for modern cloud-native applications and microservices architectures.
Nginx is a high-performance web server and reverse proxy server widely used for serving static content and handling HTTP requests. It's particularly known for its ability to handle large volumes of concurrent connections with low resource consumption. Nginx also functions as a load balancer, distributing traffic across multiple backend servers and as a reverse proxy, improving the security and performance of web applications by masking their underlying infrastructure.
The Apache HTTP Server (Apache) is an open-source web server that serves static content and can be extended with modules to handle dynamic content. Apache supports a wide range of features, including SSL/TLS encryption, URL rewriting, and authentication. It's highly configurable and widely used for serving websites, web applications, and APIs, particularly when paired with a content management system or a PHP-based framework like WordPress.
Kong is an open-source API gateway and microservices management platform that provides features like load balancing, API traffic routing, security (rate limiting, authentication), and monitoring. It allows backend applications to scale and perform reliably by managing API calls and centralizing service management. Kong integrates well with microservices architectures, simplifying API management, reducing overhead, and improving security.
AWS API Gateway is a fully managed service provided by Amazon Web Services that enables developers to create, publish, and manage APIs at any scale. It supports RESTful APIs and WebSocket APIs, offering powerful features like security (via AWS IAM), monitoring (via CloudWatch), and traffic management. AWS API Gateway simplifies the process of integrating APIs with AWS services, making it an essential tool for developers building serverless or microservices-based applications.
JWT is a compact, URL-safe token format used to transmit information between parties securely. It’s commonly used for stateless authentication, where the token contains all the necessary user information, eliminating the need for sessions on the server. JWT is widely used in modern web and mobile applications for securely managing user sessions and handling authorization across multiple platforms without requiring a centralized session store.
OAuth is an open standard for authorization, allowing users to share their private resources between different applications without giving out their credentials. OAuth 2.0, the most widely used version, enables third-party applications to access user data with permission. OAuth is commonly used in social logins (e.g., logging into an app with Google or Facebook) and is integral for APIs that need secure access without exposing sensitive data like passwords.
Cloudflare is a global web infrastructure and security company that provides services like content delivery, DDoS protection, and web application firewall (WAF). It acts as a reverse proxy to optimize the delivery of content and protect web applications from malicious traffic. By caching static content and mitigating attacks, Cloudflare improves website performance and security, ensuring high availability and reliability for websites and applications across the globe.
Git is a distributed version control system that tracks changes in source code, enabling developers to collaborate efficiently on software projects. Git allows multiple developers to work on the same codebase without overwriting each other’s changes. It also supports branching and merging, making it easy to experiment with new features and then integrate them into the main project. Git is the foundation for most code-hosting platforms, such as GitHub, GitLab, and Bitbucket.
Jenkins is an open-source automation server used to implement Continuous Integration (CI) and Continuous Delivery (CD) pipelines. It automates repetitive tasks such as testing, building, and deploying applications, improving development speed and consistency. Jenkins integrates with a wide variety of plugins and version control systems, making it highly customizable for different development workflows. It’s especially useful for maintaining high code quality and quickly deploying new features.
Datadog is a cloud-based monitoring and analytics platform for tracking the health and performance of applications, databases, and servers. It integrates seamlessly with cloud services and offers features like real-time dashboards, alerting, and log management. Datadog provides full-stack observability, allowing developers and operations teams to monitor metrics, traces, and logs in a single view. It’s commonly used for ensuring optimal performance, identifying bottlenecks, and troubleshooting issues in production environments.
Sentry is an open-source error-tracking platform that helps developers monitor and fix crashes in real time. By automatically capturing errors, exceptions, and performance issues, Sentry allows teams to identify and resolve bugs before they affect users quickly. It integrates with various programming languages and frameworks, providing detailed stack traces, contextual data, and tools for prioritizing and managing issues. Sentry is invaluable for maintaining high-quality, bug-free applications.
Backend frameworks are software libraries or platforms that provide a foundation for building backend services in web applications. These frameworks offer predefined structures, tools, and conventions to streamline common tasks such as routing, database management, authentication, and API creation. By abstracting away repetitive tasks, backend frameworks allow developers to focus on writing application-specific code and delivering features quickly.
Node.js is not technically a framework but a runtime environment that allows you to run JavaScript on the server side. It uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, ideal for building scalable network applications and real-time services like chat apps and APIs. Though often paired with frameworks like Express.js, Koa, or NestJS, Node.js itself is a foundation for developing fast, event-driven backend systems.
Express.js is the most popular and minimalistic backend framework for Node.js. It simplifies the creation of web servers and APIs by providing routing capabilities, middleware support, and HTTP utilities.
Express helps in handling HTTP requests and responses efficiently and is often the starting point for creating RESTful APIs. Its flexibility makes it easy to integrate with various database systems, authentication solutions, and templating engines.
Django is a high-level Python framework that encourages rapid development and clean, pragmatic design. It comes with many built-in features, such as an ORM (Object-Relational Mapping), an admin interface, and robust security features like protection against SQL injection and cross-site scripting. Django is an excellent choice for building scalable, maintainable web applications with a strong focus on security and developer productivity.
Flask is a micro-framework for Python that’s lightweight and flexible, designed to be minimal yet extensible. Unlike Django, Flask doesn't come with built-in ORM or form-handling libraries, allowing developers to choose their tools and create a custom stack.
It is perfect for small to medium-sized applications or when you need more control over how your project is structured. Flask is highly favored for building RESTful APIs and lightweight web apps.
Ruby on Rails (Rails) is a full-stack framework built on the Ruby programming language, emphasizing the principle of "convention over configuration." It allows developers to quickly build robust web applications by using built-in conventions for everything from routing to database migrations.
Rails has a powerful ORM (ActiveRecord) and comes with built-in tools for authentication, testing, and deploying applications. It’s often favored for rapid prototyping and startups due to its productivity-boosting features.
Laravel is a PHP framework known for its elegant syntax and powerful features. It follows the MVC (Model-View-Controller) design pattern and offers a wide range of tools, such as built-in authentication, routing, caching, and database migrations.
Laravel includes an ORM called Eloquent, which makes working with databases easy. It also has integrated tools for testing, deployment, and scheduling tasks, making it a comprehensive choice for modern PHP-based applications.
Spring Boot is part of the larger Spring Framework, designed for simplifying the development of Java-based applications. It provides built-in tools to handle web requests, database management, security, and more.
Spring Boot is known for its "convention over configuration" approach, reducing the complexity of application setup. It supports building microservices-based applications and is highly favored for enterprise applications due to its scalability, security, and modularity.
NestJS is a progressive Node.js framework built with TypeScript, designed to help developers create scalable and maintainable backend applications. It’s heavily inspired by Angular, with a modular architecture that makes it easy to build large applications.
NestJS supports a variety of databases and includes powerful tools for building REST APIs, WebSockets, microservices, and more. It’s ideal for creating enterprise-level applications using modern JavaScript features.
ASP.NET Core is an open-source, cross-platform framework for building modern web applications and APIs using .NET. It’s known for its high performance, scalability, and tight integration with other Microsoft technologies. ASP.NET Core is lightweight and optimized for building cloud-based applications and microservices. It is often used in enterprise environments due to its reliability and integration with the wider Microsoft stack.
Phoenix is a backend framework written in Elixir, a functional programming language built on the Erlang virtual machine (VM). Phoenix is designed for high-performance, scalable web applications, especially real-time systems like chat apps and live updates.
Phoenix uses the Channel abstraction for WebSockets, allowing developers to build real-time, distributed applications easily. It’s an excellent choice for handling high-concurrency scenarios and offers a developer-friendly experience.
Databases are a critical component of backend systems, providing a structured way to store, retrieve, and manage data. There are two primary categories of databases: relational (SQL) and non-relational (NoSQL).
Each type has its strengths and weaknesses, and the choice of database depends on the requirements of the application, such as data structure, scalability, and performance.
PostgreSQL is a powerful, open-source relational database system known for its robustness, extensibility, and SQL compliance. It supports advanced data types like JSON, arrays, and history and allows developers to define custom data types, functions, and operators.
PostgreSQL is ACID-compliant, ensuring data integrity in transactions. It’s suitable for complex queries, large datasets, and applications that require high levels of data consistency and integrity, such as financial applications, content management systems, and geospatial applications.
MySQL is one of the most popular relational databases used for web applications. Known for its speed and reliability, MySQL supports structured query language (SQL) to manage structured data.
It is commonly used in combination with web frameworks like PHP or JavaScript (Node.js). MySQL is often chosen for its ease of use, scalability, and support for ACID transactions, making it ideal for web applications, e-commerce platforms, and content management systems (CMS).
SQLite is a serverless, self-contained relational database engine often used in embedded systems, mobile applications, and local development environments. Unlike traditional databases, SQLite doesn’t require a server to operate, making it lightweight and easy to set up.
While it lacks some advanced features of larger systems like PostgreSQL, its simplicity and low resource requirements make it ideal for lightweight applications, testing, and prototyping.
MongoDB is a popular NoSQL document-based database that stores data in flexible, JSON-like documents. It provides high scalability and flexibility, allowing developers to handle unstructured or semi-structured data.
MongoDB is ideal for applications that require rapid development and high availability, such as content management systems, real-time analytics platforms, and Internet of Things (IoT) applications. It supports horizontal scaling, enabling it to handle large amounts of unstructured data across multiple servers.
Apache Cassandra is a highly scalable, distributed NoSQL database designed for handling massive amounts of data across many commodity servers. Known for its high availability and fault tolerance, Cassandra is often used in systems where uptime and data replication across distributed systems are critical, such as in large-scale web applications, social networks, and IoT systems.
Cassandra provides eventual consistency, meaning it may not guarantee immediate consistency but ensures that data will eventually become consistent across all nodes.
Redis is an in-memory key-value store that is primarily used for caching, session management, and real-time applications. It supports data structures like strings, hashes, lists, sets, and sorted sets, making it versatile for use cases such as message queuing, leaderboards, and real-time analytics.
Redis is highly performant due to its in-memory nature, offering sub-millisecond latency and high throughput, making it an essential tool for improving the speed of web applications by reducing the load on relational databases.
Neo4j is a graph database designed to handle complex, interconnected data efficiently. It stores data as nodes and relationships, making it ideal for applications that require advanced queries on data relationships, such as social networks, fraud detection, and recommendation systems.
Neo4j uses the Cypher query language to express complex graph-based queries, providing an intuitive and efficient way to interact with highly relational data.
Amazon DynamoDB is a fully managed NoSQL database service provided by AWS, designed for applications that require consistent, single-digit millisecond response times at any scale.
DynamoDB is highly scalable and automatically adjusts to the load of your application, making it suitable for use cases like gaming, mobile apps, IoT, and e-commerce platforms. DynamoDB supports both key-value and document data models, offering flexible, scalable solutions for managing unstructured data.
Firebase Firestore is a serverless, scalable NoSQL cloud database from Google Firebase, designed for building real-time applications. It supports automatic synchronization of data across clients, which makes it ideal for real-time features like live updates, chat apps, and collaborative applications.
Firestore is fully managed, easy to set up, and integrates well with other Firebase services. It's especially popular for mobile applications, but it can be used in web apps as well.
CouchDB is a document-oriented NoSQL database that stores data in JSON format. It features Multi-Version Concurrency Control (MVCC), which allows it to manage multiple versions of the same data, making it ideal for applications where concurrent access and offline capabilities are important.
CouchDB's simple HTTP/REST API allows easy integration with web applications. It's well-suited for mobile and distributed applications where data replication is key to ensuring data availability and reliability across multiple devices.
APIs (Application Programming Interfaces) allow different software systems to communicate and share data or functionality. Backend systems often use APIs to expose services, share data with other applications, and enable third-party integrations.
API management refers to the process of designing, deploying, and managing APIs efficiently and securely. Effective API management ensures that APIs are used properly, performing well, and secure.
RESTful APIs (Representational State Transfer) are the most common type of APIs used for web services. They are based on stateless HTTP protocols and allow clients to interact with backend services using standard HTTP methods (GET, POST, PUT, DELETE).
RESTful APIs are lightweight, scalable, and flexible, making them ideal for mobile and web applications. They often return data in JSON format, which is easy to process on the client side. RESTful APIs are typically used to build public APIs, third-party integrations, and microservices architectures.
GraphQL is a query language for APIs and a runtime for executing queries by using a type system that defines the data you can query. Unlike REST, which returns predefined data, GraphQL allows clients to request exactly the data they need, avoiding over-fetching and under-fetching issues.
GraphQL is highly flexible and works well for applications that require real-time data or those with complex relationships between data objects. It’s widely used in modern web and mobile applications, especially where multiple data sources need to be aggregated.
SOAP (Simple Object Access Protocol) is a protocol for exchanging structured information in the implementation of web services. SOAP APIs are known for being highly standardized, and they support XML messaging to ensure strict security, transaction compliance, and messaging integrity.
While SOAP is less commonly used for new applications compared to REST and GraphQL, it is still popular in legacy systems, financial services, and enterprise environments where security and data consistency are critical.
OpenAPI (formerly Swagger) is a specification for defining and documenting RESTful APIs. It provides a standardized way to describe the structure of APIs, including endpoints, request/response formats, and authentication.
With OpenAPI, developers can auto-generate documentation, client SDKs, and even server stubs, saving significant time. It also makes it easier for teams to collaborate on API development, as the specification acts as a clear contract between front-end and back-end developers.
An API Gateway is a server that acts as an entry point into a system and handles incoming API requests. It performs tasks like request routing, load balancing, caching, authentication, and rate limiting.
API gateways are crucial in microservices architectures, where they can consolidate multiple backend services under a single endpoint. They simplify API management by providing a central place to manage access control and monitoring. Popular API gateways include Kong, Amazon API Gateway, and Nginx.
Proper authentication and authorization are critical for ensuring that only authorized users and systems can access API resources. OAuth and JWT (JSON Web Tokens) are the most common authentication methods used with APIs.
OAuth allows clients to securely access resources on behalf of users without sharing credentials. JWTs are often used for stateless authentication, where tokens are issued to authenticate and authorize users. Secure API access is essential for preventing unauthorized data access and ensuring compliance with privacy standards like GDPR.
Proper API documentation is vital for ensuring that developers can easily understand and integrate with your API. Tools like Swagger UI, Redoc, and Postman automatically generate interactive, user-friendly documentation based on your OpenAPI specification or API definitions.
Well-documented APIs reduce development time, improve the developer experience, and make it easier for external developers to use and integrate your API into their applications.
API testing is essential to ensure that your API functions as expected and handles edge cases properly. Tools like Postman, SoapUI, and Insomnia are widely used for testing APIs by sending requests, validating responses, and ensuring that APIs behave correctly under various conditions.
Automated testing frameworks like JUnit (for Java) and Mocha (for Node.js) also support API testing, helping to identify issues early in the development cycle and improve the reliability of your API.
Rate limiting is the practice of limiting the number of requests a user or service can make to an API within a given time window (e.g., 1000 requests per hour). Rate limiting protects your API from abuse and helps maintain the performance and availability of your services.
It prevents excessive traffic from overloading your servers and ensures that API resources are allocated fairly. Tools like Nginx, Kong, and API Gateway services often offer built-in support for rate limiting.
API monitoring and analytics tools allow developers to track the performance, usage, and error rates of their APIs. Tools like Datadog, New Relic, Prometheus, and Grafana can be integrated with APIs to provide real-time insights into traffic patterns, latency, error rates, and other critical metrics.
This helps developers and operations teams quickly identify issues, optimize performance, and ensure that APIs are meeting service-level objectives (SLOs) and service-level agreements (SLAs).
Hosting and server management are essential components of backend infrastructure. They enable applications and services to be deployed, run, and accessed by users.
Hosting refers to the provisioning of servers to host websites, databases, and applications, while server management involves configuring, monitoring, and maintaining these servers to ensure optimal performance, security, and uptime. Proper hosting and server management are crucial for ensuring that applications run efficiently, scale effectively, and remain secure.
Shared hosting is a cost-effective option where multiple websites or applications share the same server resources, such as CPU, RAM, and storage. This makes shared hosting affordable for small businesses, blogs, or personal websites.
However, shared hosting has its limitations in terms of performance, scalability, and customization since resources are shared with other tenants on the same server. It's ideal for smaller projects but may not be suitable for high-traffic or resource-intensive applications.
A Virtual Private Server (VPS) is a more powerful option compared to shared hosting. With VPS hosting, a physical server is partitioned into multiple virtual servers, each with its resources like CPU, RAM, and storage.
This provides greater control and customization compared to shared hosting while still being more affordable than dedicated hosting. VPS hosting is ideal for medium-sized businesses, growing applications, and websites that require more resources and flexibility but don’t yet need a full dedicated server.
Dedicated hosting gives users an entire physical server dedicated solely to their website or application. This offers the highest level of performance, security, and control, as all server resources are allocated exclusively to one client.
Dedicated hosting is ideal for large enterprises or high-traffic websites that need significant resources, full control over their environment, and the ability to customize the server configuration. However, dedicated hosting is more expensive and requires advanced server management skills.
Cloud hosting refers to the hosting of applications and data on virtual servers that draw resources from a network of physical servers, often provided by a cloud service provider (e.g., Amazon Web Services, Google Cloud, Microsoft Azure).
Cloud hosting is highly scalable, cost-efficient, and flexible, as resources can be adjusted dynamically to meet the needs of the application. Cloud hosting is ideal for applications that experience fluctuating traffic, need to scale rapidly, or require high availability and redundancy.
Managed hosting is a service where the hosting provider takes care of all aspects of server management, including configuration, monitoring, security, and maintenance. Managed hosting is ideal for businesses or developers who want to focus on their applications and don’t have the resources to handle the complexities of server management.
This type of hosting is commonly used in environments where uptime, security, and performance are critical, such as e-commerce sites and large-scale applications.
Serverless hosting is a model where developers deploy code without worrying about server management. In serverless architectures, cloud providers like AWS Lambda, Google Cloud Functions, or Azure Functions automatically manage the server infrastructure, scaling resources up or down based on demand.
Serverless hosting is ideal for event-driven applications, microservices, or workloads that don’t require a constantly running server. It’s cost-effective because you only pay for the actual computing resources consumed during code execution.
Web hosting platforms like cPanel and Plesk provide an easy-to-use interface for managing websites, databases, and server configurations. These platforms are designed to simplify server management tasks, such as setting up domains, email, file management, and security configurations.
They are often used in shared, VPS, and dedicated hosting environments to streamline administrative tasks and provide a user-friendly experience for server administrators. They are particularly useful for users who need to become more familiar with command-line server management.
Containerization is a technology that allows applications to be packaged with all the dependencies they need to run, making them portable across different environments. Docker is a popular containerization platform that allows developers to create lightweight, consistent environments for their applications.
Kubernetes is an open-source container orchestration platform that automates the deployment, scaling, and management of containerized applications. Both Docker and Kubernetes are widely used in cloud environments to manage microservices architectures, ensuring scalability, portability, and efficient resource utilization.
Load balancers distribute incoming network traffic across multiple servers to ensure that no single server becomes overwhelmed, improving application performance and reliability. Load balancing can be done at different layers, including HTTP(S), TCP, and DNS, and is essential for applications that need to scale horizontally.
Load balancers can also improve fault tolerance, as traffic is automatically redirected to healthy servers in the event of a failure. Popular load balancers include HAProxy, NGINX, and cloud-native load balancers provided by platforms like AWS and Google Cloud.
A Content Delivery Network (CDN) is a system of geographically distributed servers that cache and deliver content (such as images, videos, JavaScript, and CSS) to users based on their location. CDNs reduce latency by delivering content from the nearest server, improving page load times, and enhancing the user experience.
They also help reduce the load on the origin server, improve website availability, and provide protection against DDoS attacks. Popular CDN providers include Cloudflare, Akamai, and AWS CloudFront.
In the context of backend development, security is a critical concern. Protecting data, preventing unauthorized access, and ensuring the overall integrity of backend systems are essential for maintaining trust and compliance. Backend systems often store sensitive information such as user data, payment details, and business logic, making them prime targets for cyberattacks.
Security tools help backend developers safeguard their systems, detect vulnerabilities, and mitigate risks. These tools cover various aspects of security, including encryption, authentication, intrusion detection, vulnerability scanning, and compliance monitoring.
Encryption ensures that sensitive data is protected both in transit and at rest. Encryption tools help backend developers secure communication between users and servers, as well as protect data stored in databases and file systems.
For example:
Encryption tools are indispensable for ensuring data privacy and meeting regulatory requirements such as GDPR and HIPAA.
Authentication verifies the identity of a user or system, while authorization determines what resources or actions an authenticated user is allowed to access. Secure authentication and authorization mechanisms are foundational to backend security.
Common tools include:
These tools help ensure that only legitimate users can access sensitive backend resources and that they can only perform authorized actions.
Firewalls and network security tools are essential for controlling incoming and outgoing traffic to and from backend systems. These tools prevent unauthorized access and malicious traffic from reaching your servers.
Some of the most widely used tools include:
Firewalls are essential for protecting backend servers from unauthorized access and defending against network attacks such as DDoS (Distributed Denial of Service).
Intrusion Detection Systems (IDS) monitor network traffic and log activities to detect potential security breaches, while Intrusion Prevention Systems (IPS) actively block suspicious activities.
Key tools include:
IDS and IPS tools are vital for detecting and preventing unauthorized access and malicious activities in real time.
Vulnerability scanning tools help identify weaknesses in backend systems, applications, and networks before attackers can exploit them. These tools perform regular scans and generate reports on potential security risks, helping developers and administrators fix vulnerabilities.
Popular tools include:
Regular vulnerability scanning is an essential part of maintaining a secure backend environment and ensuring compliance with security standards.
Penetration testing (or ethical hacking) simulates cyberattacks on backend systems to identify potential weaknesses and vulnerabilities. Penetration testing tools help developers and security teams proactively find and fix security gaps.
Popular tools include:
Penetration testing tools are crucial for identifying and mitigating risks in your backend systems before attackers can exploit them.
SIEM tools collect and analyze security event data from multiple sources to detect, investigate, and respond to security incidents. They help backend administrators maintain visibility into the security posture of their systems and ensure compliance with security policies.
Key SIEM tools include:
SIEM tools are essential for monitoring backend systems, detecting unusual activities, and responding to security breaches quickly.
Backup and disaster recovery tools ensure that backend data can be restored in case of an attack, system failure, or other disasters. These tools help prevent data loss and ensure that systems can be quickly brought back online after an incident.
Popular tools include:
Regular backups and disaster recovery plans are essential for ensuring business continuity and minimizing downtime in case of an attack.
Compliance monitoring tools help ensure that backend systems adhere to industry regulations and standards, such as GDPR, HIPAA, and PCI-DSS. These tools track data handling, access controls, and audit logs to ensure compliance and avoid costly fines.
Popular compliance tools include:
Compliance tools are important for maintaining the security and privacy of sensitive data and ensuring that backend systems meet legal requirements.
Endpoint security tools protect devices (endpoints) that connect to backend systems, such as servers, laptops, and mobile devices. These tools ensure that malicious activities are detected and prevented before they can compromise backend infrastructure.
Key endpoint security tools include:
Endpoint security tools are essential for preventing malware and other security threats from compromising backend systems through connected devices.
In backend development, the right tools and environments are essential for building, testing, deploying, and maintaining applications. These tools help streamline development processes, improve productivity, and ensure that the application is scalable, maintainable, and secure.
From integrated development environments (IDEs) to version control systems and containerization tools, the choice of development tools and environments plays a significant role in the overall success of backend projects. This section explores key tools and environments that backend developers rely on to build efficient and robust backend systems.
IDEs are software applications that provide comprehensive facilities for software development, such as code editing, debugging, and testing. They significantly enhance a developer’s productivity by offering integrated tools in a single interface.
Popular backend development IDEs include:
IDEs help developers write code efficiently, catch errors early, and manage the overall development lifecycle, making them indispensable tools for backend development.
While IDEs provide a comprehensive set of features, text editors are lighter-weight options preferred by developers for quick coding and configurations. Text editors are simpler but can be customized with plugins and extensions to support various languages and workflows.
Popular text editors include:
Text editors are ideal for small projects, quick edits, or developers who prefer a minimalist environment without the overhead of full IDEs.
Version control systems are vital for managing and tracking changes in code, especially in collaborative projects. These tools allow developers to track revisions, branch features, and merge changes smoothly.
Key VCS tools include:
Version control systems ensure code consistency, enable collaborative workflows, and maintain a history of code changes, preventing conflicts and making codebase management more efficient.
Containerization allows developers to package applications and their dependencies into lightweight, isolated containers. This ensures consistency across different environments, from development to production.
The most common containerization tools are:
Containerization helps backend developers create consistent development environments, improve scalability, and isolate services to make backend systems more modular and manageable.
Virtualization involves creating virtual instances of physical machines to run multiple isolated environments on a single host. These tools are commonly used to replicate production environments locally, ensuring consistency and reducing conflicts between development and production setups.
Common tools include:
Virtualization tools enable developers to test applications in isolated environments that closely resemble production, improving reliability and minimizing the risk of issues when deploying code.
CI/CD tools are crucial for automating the process of integrating code changes, testing, and deploying applications. They help ensure that backend applications are continuously updated, tested, and released with minimal manual intervention.
Popular CI/CD tools include:
CI/CD tools improve collaboration, reduce the time to market, and ensure that code is thoroughly tested and deployed with minimal errors, enhancing overall development efficiency.
Databases are a core component of backend systems, providing persistent storage for application data. Along with choosing a suitable database, developers rely on tools to manage, monitor, and optimize database performance.
Popular database-related tools include:
These tools help developers interact with databases more effectively, whether managing data schemas, optimizing queries, or troubleshooting performance issues.
Frameworks and libraries are pre-written code that backend developers can use to avoid reinventing the wheel. These tools simplify development by providing common functions and structures.
Key backend frameworks include:
Frameworks accelerate development, provide standardized structures, and make backend systems more scalable, maintainable, and secure.
Testing is a critical part of backend development to ensure that applications function as expected and are free from bugs. Backend developers use a variety of testing tools for unit testing, integration testing, and performance testing.
Popular testing tools include:
Testing tools are essential for ensuring that backend systems are reliable, bug-free, and perform as expected under various conditions.
Monitoring and logging tools help backend developers track application performance, detect issues in real time, and ensure that systems are operating smoothly.
Some popular tools include:
Monitoring and logging tools help backend teams ensure that their applications are running efficiently, can quickly diagnose issues, and maintain system health over time.
In backend development, monitoring, and analytics tools are vital for ensuring system reliability, performance, and user satisfaction. These tools help developers and operations teams track the health of their applications, identify issues before they escalate, and make data-driven decisions to optimize performance.
With the complexity of modern applications, particularly in distributed environments like microservices and cloud-native architectures, robust monitoring and analytics tools are essential for ensuring smooth operation. This section explores key monitoring and analytics tools that backend developers and DevOps teams rely on to manage and optimize their systems.
Application Performance Monitoring (APM) tools provide insights into the performance of backend systems by tracking metrics like response times, error rates, throughput, and resource consumption. These tools help identify bottlenecks, troubleshoot issues, and ensure that applications are performing optimally.
Popular APM tools include:
APM tools are crucial for tracking backend application health, identifying issues in real time, and improving overall performance.
Infrastructure monitoring tools focus on the health and performance of the servers, networks, and other infrastructure components that support backend applications. These tools provide visibility into system resources like CPU usage, memory, disk space, and network traffic, ensuring that the underlying infrastructure is performing optimally.
Key tools include:
These tools are essential for ensuring that the infrastructure supporting backend systems is running smoothly and that resources are allocated efficiently.
Logs are a fundamental source of information for diagnosing issues and understanding application behavior. Log management tools aggregate, store, and analyze log data from different parts of a system, providing a unified view for debugging and monitoring.
Key tools include:
Centralized logging tools are critical for troubleshooting and ensuring backend systems are functioning as expected. They help developers quickly identify issues, such as errors, crashes, or unexpected behaviors.
Real-time monitoring tools provide live tracking of system performance and automatically alert teams when predefined thresholds or anomalies are detected. This proactive approach helps to address issues before they impact users or cause downtime.
Notable tools include:
Real-time monitoring tools allow for quick identification of issues, helping backend teams respond efficiently and minimize downtime or user impact.
User Experience Monitoring (UEM) and Real User Monitoring (RUM) tools focus on capturing and analyzing the end-user experience, providing insights into how backend systems impact users. These tools track metrics like page load times, API response times, and user behavior to optimize the application’s overall user experience.
Popular tools include:
RUM tools are vital for understanding how backend performance affects end-users and for identifying areas that need optimization to improve user experience.
Database monitoring tools help track the performance and health of backend databases, ensuring that they are running efficiently and without issues. These tools monitor database query performance, index optimization, and resource utilization.
Key database monitoring tools include:
Database monitoring tools are crucial for identifying slow queries, optimizing database performance, and ensuring that the database layer supports backend applications efficiently.
Log and event analysis tools aggregate logs from multiple sources, analyze them for patterns, and provide actionable insights to improve backend performance. These tools help developers identify recurring issues, system anomalies, and potential security threats.
Notable tools include:
These tools are essential for analyzing logs and identifying patterns that indicate issues, security threats, or opportunities for improvement.
In today's fast-paced development world, backend tools are indispensable for building, managing, and scaling applications effectively. From databases and frameworks to security and monitoring tools, every aspect of backend development is supported by specialized tools that streamline workflows, improve performance, and enhance security. The right set of backend tools empowers developers to create robust, scalable, and maintainable systems that deliver optimal user experiences.
The evolution of backend tools, especially in areas like API management, containerization, and CI/CD pipelines, has transformed the way developers approach challenges related to deployment, performance monitoring, and system reliability. With the increasing complexity of applications, particularly in distributed and microservices architectures, a strong backend infrastructure is more important than ever.
Copy and paste below code to page Head section
Backend tools are software or platforms used by developers to build, manage, and maintain the server side of web applications. They assist in tasks such as database management, server handling, API development, testing, deployment, monitoring, and security. Common backend tools include programming frameworks, databases, version control systems, and CI/CD pipelines.
Backend tools are crucial because they streamline development workflows, improve the quality of code, ensure system reliability, and simplify complex tasks like managing databases, handling APIs, and deploying applications. They help developers save time, reduce errors, and ensure scalability, security, and performance in backend systems.
Databases store, manage, and retrieve data for web applications. They are a core component of backend development. Tools like MySQL, PostgreSQL, MongoDB, and SQLite allow developers to interact with databases efficiently. Choosing the right database system is essential for performance, scalability, and reliability in the backend.
Monitoring tools help track the performance, uptime, and health of backend systems. They allow developers to spot issues like slow performance, errors, and bottlenecks in real time, enabling quick resolution. Tools like Prometheus, Datadog, New Relic, and ELK Stack provide valuable insights that help ensure systems run smoothly and scale as needed.
CI/CD stands for Continuous Integration and Continuous Deployment. CI refers to the practice of automatically testing and integrating code changes into a shared repository, while CD automates the deployment of these changes to production. Tools like Jenkins, GitLab CI, and CircleCI facilitate these processes, improving code quality and accelerating the release cycle.
Security tools help protect backend systems from threats like unauthorized access, data breaches, and cyberattacks. Tools like OWASP ZAP for vulnerability scanning, JWT for secure token-based authentication, and SSL/TLS for encrypted communication ensure the confidentiality, integrity, and security of both data and applications.