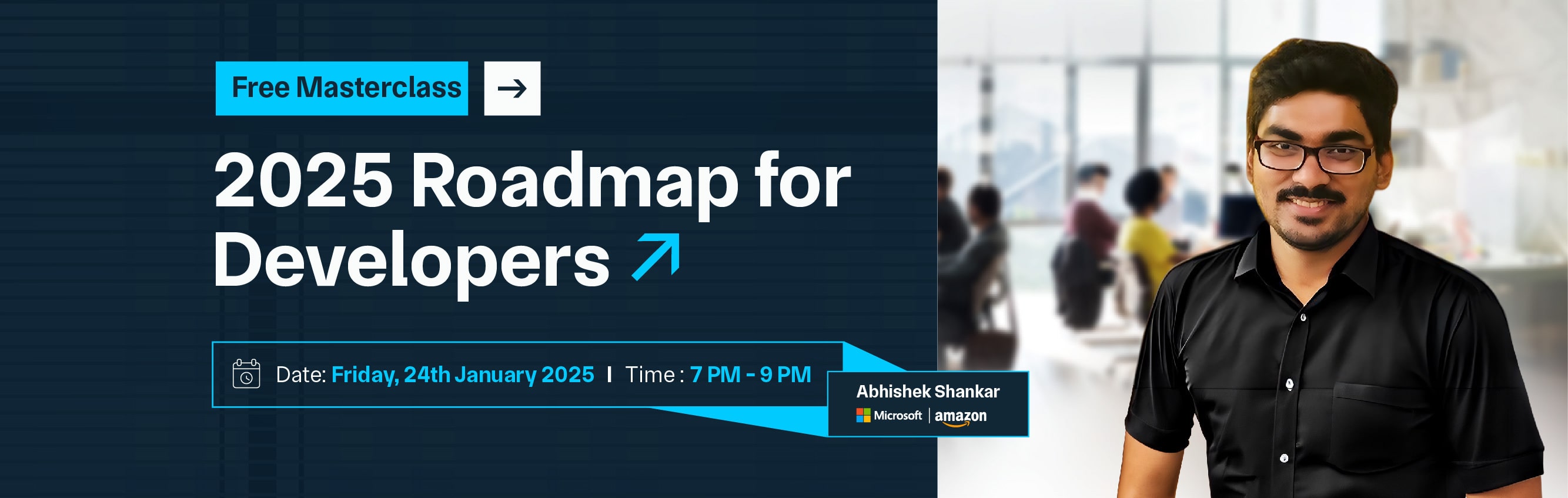

Discover a collection of backend projects, complete with source code, designed to elevate your development skills and showcase best practices. These projects cover a wide range of functionalities, including user authentication systems, RESTful APIs, real-time data processing, and database management. Each project comes with detailed documentation, making it easy to understand the code and integrate various features.
Whether you're building a blog platform, an e-commerce backend, or a chat application, these projects offer practical examples and reusable components to help streamline your development process. By exploring and modifying these source codes, you can gain hands-on experience with different backend technologies such as Node.js, Django, Flask, Ruby on Rails, and more.
Perfect for developers looking to enhance their portfolios or dive into backend development, these projects not only offer code but also provide insights into architectural design, security practices, and performance optimization. Start building robust and scalable applications with these comprehensive backend solutions, and see your development skills reach new heights. These backend projects include diverse scenarios like microservices architecture, payment integration, and user data analytics. Explore these examples to implement advanced features and refine your backend development expertise.
A backend project involves developing the server-side components of an application that manages data processing, business logic, and database interactions. Unlike the front end, which focuses on user interfaces and user experience, the back end handles the behind-the-scenes functionality that powers an application.
Key aspects of a backend project typically include:
Backend projects can range from simple CRUD (Create, Read, Update, Delete) applications to complex systems involving microservices, real-time data processing, and advanced security protocols.
A categorized list of backend projects designed to help you develop your skills at different levels:
Each project offers hands-on experience with various technologies and design patterns, making it easier to grow as a backend developer.
1. Basic CRUD API: Build a simple API that performs Create, Read, Update, and Delete operations on a data model.
2. User Authentication System: Implement user registration and login functionalities with basic session or token-based authentication.
3. To-Do List Application: Develop a backend for a to-do list app where users can add, edit, and delete tasks.
4. Simple Blogging Platform: Create a backend that allows users to write, edit, and delete blog posts with basic user management.
5. Weather Information API: Build an API that fetches and returns weather data from a public weather API.
6. Contact Management System: Develop a backend to manage and store contact information, including adding, updating, and deleting contacts.
7. Bookstore Inventory Management: Create a backend for managing books in a bookstore, including stock management and basic search functionality.
8. Basic Chat Application: Implement a simple backend for a chat application, supporting basic messaging between users.
9. URL Shortener: Build a backend that shortens long URLs and redirects users to the original URL.
10. Expense Tracker: Develop a backend for tracking and categorizing user expenses.
11. E-Commerce Backend: Implement a backend for an e-commerce site with product listings, user accounts, shopping carts, and order management.
12. Task Management System: Create a backend that supports task assignment, project tracking, and team collaboration features.
13. Social Media API: Build a backend for a social media platform that includes user profiles, posts, likes, and comments.
14. Recipe Sharing Platform: Develop a backend for a platform where users can share and discover recipes, with features for reviews and ratings.
15. Real-Time Notification System: Implement a backend that sends real-time notifications to users using technologies like WebSockets or server-sent events.
16. Forum/Discussion Board: Create a backend for a forum that supports threads, posts, and user management.
17. Job Board API: Build an API for a job board where employers can post job listings and job seekers can apply.
18. Content Management System (CMS): Develop a backend for a CMS that allows content creation, editing, and publishing with role-based permissions.
19. Online Learning Platform: Implement a backend for an educational platform with course management, user progress tracking, and quizzes.
20. Inventory Management System: Create a backend to manage inventory for a business, including stock levels, ordering, and reporting.
21. Microservices Architecture: Develop a set of interconnected microservices handling different aspects of a complex application, such as user management, billing, and notifications.
22. Real-Time Analytics Dashboard: Create a backend that processes and visualizes real-time data analytics, supporting large data volumes and fast updates.
23. Machine Learning AP: Implement a backend that serves machine learning models, providing predictions or classifications via an API.
24. Multi-Tenant SaaS Application: Build a scalable backend for a Software as a Service (SaaS) application that supports multiple clients with isolated data.
25. Blockchain-Based Application: Develop a backend that integrates with a blockchain for secure transactions or smart contract execution.
26. Event-Driven System with Kafka/RabbitMQ: Create a backend that uses event streaming platforms like Apache Kafka or RabbitMQ to handle asynchronous processing and messaging.
27. GraphQL API: Implement a backend with a GraphQL API, providing flexible querying and efficient data retrieval.
28. High-Performance Search Engine: Develop a backend for a search engine that indexes and retrieves large datasets quickly and accurately.
29. Distributed File Storage System: Create a backend for managing and accessing files across a distributed storage system, with redundancy and fault tolerance.
30. Custom Authentication Service: Implement a sophisticated authentication service with features like OAuth2, JWT, multi-factor authentication, and fine-grained access controls.
These projects vary in complexity and require skills, allowing you to build your backend development expertise progressively.
Beginner-level backend development projects focus on building foundational skills by creating simple applications and services.
Develop a simple API that handles Create, Read, Update, and Delete (CRUD) operations on a data model. This project helps you grasp RESTful principles and basic database interactions. For instance, you could create an API for managing user profiles where you can add new users, retrieve user information, update details, and delete users. This foundational project is great for learning how to build and structure RESTful services.
Create a backend system for user registration and login with session or token-based authentication. This project focuses on securely managing user credentials and sessions. Users should be able to register, log in, and access protected resources based on their authentication status. Implementing this system involves handling password encryption, user sessions or tokens, and secure login procedures.
Develop a backend for a to-do list application that allows users to create, update, and delete tasks. This project involves managing user-specific data and implementing basic CRUD operations within a task management context. Users can add new tasks, update existing ones, and remove completed or unwanted tasks. This project helps you understand user data management and task organization.
Build a backend for a blogging platform where users can write, edit, and delete blog posts. This project involves user management and content creation functionalities. Users should be able to create new posts, modify existing content, and delete posts. It also includes basic user roles and permissions, allowing for a simple content management system.
Create an API that retrieves and returns weather data from a public weather service. This project involves integrating with third-party APIs to fetch weather information and process it. For example, users can request current weather conditions for specific cities and receive details like temperature, humidity, and forecasts. It’s a practical exercise in API integration and data handling.
Develop a backend to manage and store contact information, enabling users to add, update, and delete contacts. This project focuses on organizing personal data efficiently. Users can create contact entries, edit details such as phone numbers and emails, and delete contacts as needed. It’s useful for practicing data CRUD operations and user data management.
Create a backend system for managing a bookstore’s inventory, including stock levels and search functionality. This project involves handling book data, managing stock quantities, and providing search capabilities. Users can add new books, update inventory counts, and search for books by title, author, or genre, helping you practice data management and querying.
Implement a backend for a basic chat application that supports real-time messaging between users. This project introduces real-time communication and message handling using technologies like WebSockets. Users can initiate chats, send and receive messages, and view message history. It’s a great way to learn about real-time data transfer and message management.
Build a backend that shortens long URLs and redirects users to the original links. This project involves handling URL shortening and redirection processes. Users input long URLs to receive shorter, more manageable versions. The system then redirects users from the shortened URL to the original link, which is useful for learning about URL handling and redirection.
Develop a backend for an expense-tracking application where users can log, categorize, and manage their expenses. This project involves organizing financial data and providing summaries of spending. Users can add new expenses, categorize them (e.g., food, transport), and view detailed reports of their expenditures. It’s a practical way to work with financial data and user management.
Intermediate-level backend development projects build on foundational skills by introducing more complex features and architectural considerations. These projects often involve integrating multiple components and technologies, enhancing your ability to handle real-world scenarios. Examples include developing an e-commerce backend with product listings, user accounts, and order management or creating a task management system with project tracking and team collaboration tools.
You might also work on a social media API with user profiles, posts, and comments or a recipe-sharing platform with review and rating functionalities. These projects challenge you to manage more sophisticated data interactions, implement robust security measures, and handle higher traffic loads, preparing you for more advanced backend development roles.
Implement a backend for an e-commerce site with functionalities for managing product listings, user accounts, shopping carts, and order processing. This project covers various aspects like product catalog management, user authentication, shopping cart operations, and order tracking. For example, users can browse products, add items to their cart, and place orders, while administrators can manage inventory and process transactions. This comprehensive backend supports essential e-commerce operations and ensures a seamless shopping experience.
Create a backend for a task management system that supports task assignment, project tracking, and team collaboration. This project includes features for creating and assigning tasks, tracking progress, and managing projects. For example, users can assign tasks to team members, monitor project milestones, and collaborate on task updates. The system helps in organising workflows and enhancing team productivity through effective task management.
Build a backend for a social media platform with user profiles, posts, likes, and comments. This project involves creating endpoints for user registration, profile management, posting content, liking posts, and commenting. For example, users can create and update profiles, share posts, interact with others’ content, and engage in discussions through comments. This API supports core social media functionalities and user interaction.
Develop a backend for a recipe-sharing platform where users can share, discover, and rate recipes. This project includes features for recipe submission, search, and review management. For example, users can upload their recipes, search for recipes based on ingredients or cuisine, and rate or comment on other users’ recipes. The system facilitates recipe sharing and community engagement through ratings and reviews.
Implement a backend that delivers real-time notifications to users using technologies such as WebSockets or server-sent events. This project enables instant updates and communication. For example, users can receive notifications about new messages, system alerts, or updates in real time. The backend ensures timely delivery of notifications and enhances user engagement with immediate feedback.
Create a backend for a forum or discussion board that supports threads, posts, and user management. This project involves features for creating discussion threads, posting comments, and moderating content. For example, users can start new threads, participate in discussions, and manage user profiles. The system supports community interactions and content organization in a forum setting.
Build an API for a job board where employers can post job listings, and job seekers can apply for positions. This project includes endpoints for creating job listings, searching for jobs, and submitting applications. For example, employers can list job openings with details, while job seekers can browse listings and apply for positions. The API facilitates job search and application processes.
Develop a backend for a Content Management System (CMS) that allows users to create, edit, and publish content with role-based permissions. This project includes features for content creation, workflow management, and user roles. For example, users can draft articles, review and approve content, and publish updates while administrators manage permissions and content access.
Implement a backend for an educational platform with features for course management, user progress tracking, and quizzes. This project involves managing courses, tracking student progress, and administering quizzes. For example, instructors can create and update courses, track students’ learning progress, and administer quizzes, while students can enroll in courses and complete assessments.
Create a backend for managing inventory, including stock levels, ordering, and reporting. This project focuses on tracking inventory levels, managing orders, and generating reports. For example, users can view stock quantities, place orders for restocking, and access inventory reports. The system supports efficient inventory management and helps businesses maintain optimal stock levels.
Advanced-level backend development projects push the boundaries of foundational skills, introducing sophisticated architectural patterns, scalability concerns, and integration of cutting-edge technologies. These projects often involve handling complex data flows, optimizing performance, and ensuring high availability.
Develop a set of interconnected microservices that manage different aspects of a complex application, such as user management, billing, and notifications. Each microservice handles a specific function and communicates with others through APIs. For example, one microservice might handle user authentication, another manages billing, and another sends notifications. This approach allows for scalable and maintainable systems, as each microservice can be developed, deployed, and scaled independently.
Create a backend that processes and visualizes real-time data analytics, supporting large data volumes and fast updates. This project involves collecting data in real-time, processing it, and presenting it through a dashboard. For example, you might track user behavior on a website and display live metrics such as traffic, user engagement, and conversion rates. This backend supports dynamic data analysis and visualization.
Implement a backend that serves machine learning models, providing predictions or classifications through an API. This project involves integrating trained machine learning models and exposing their functionality via API endpoints. For example, you could build an API that classifies images or predicts customer churn based on input data. It’s an effective way to deploy machine learning models for real-world applications.
Build a scalable backend for a Software as a Service (SaaS) application that supports multiple clients with isolated data. This project involves creating a system where each client (tenant) has its separate data while sharing the same application instance. For example, a CRM system for various companies, where each company’s data is isolated but managed under a single application framework.
Develop a backend that integrates with a blockchain for secure transactions or smart contract execution. This project involves creating a system where blockchain technology is used for managing transactions or executing contracts. For example, you could implement a backend for a decentralized application (dApp) that handles secure transactions or manages digital assets via smart contracts.
Create a backend that uses event streaming platforms like Apache Kafka or RabbitMQ to handle asynchronous processing and messaging. This project involves setting up a system where events are published, processed, and consumed in real time. For example, a backend that processes user activity logs or transaction events asynchronously to ensure smooth and scalable operations.
Implement a backend with a GraphQL API, offering flexible querying and efficient data retrieval. Unlike REST, GraphQL allows clients to request exactly the data they need, reducing over-fetching. For example, a backend for a product catalog where clients can query product details, reviews, and inventory levels in a single request tailored to their specific needs.
Develop a backend for a search engine capable of indexing and retrieving large datasets quickly and accurately. This project focuses on optimizing search queries and indexing strategies. For example, a backend for a search engine that provides fast and relevant search results across a vast collection of documents or products.
Create a backend for managing and accessing files across a distributed storage system with redundancy and fault tolerance. This project involves designing a system where files are stored across multiple nodes to ensure reliability and scalability. For example, a backend that manages file uploads ensures data redundancy and provides efficient access across distributed storage locations.
Implement a sophisticated authentication service with features like OAuth2, JWT, multi-factor authentication, and fine-grained access controls. This project involves creating a robust system for securing user access and managing permissions. For example, users can log in using OAuth2, receive JWT tokens for session management, and use multi-factor authentication for enhanced security.
Including backend projects in your portfolio is crucial for several reasons:
Backend projects showcase your ability to handle server-side logic, database management, and API development. They complement frontend skills, demonstrating that you can build comprehensive applications from end to end.
Backend development often involves solving complex problems related to data management, performance optimization, and system architecture. Featuring these projects shows your capability to tackle and resolve such challenges effectively.
Working on backend projects allows you to demonstrate proficiency in key technologies and frameworks such as Node.js, Django, or Ruby on Rails. It also highlights your knowledge of database systems (SQL/NoSQL), authentication mechanisms, and API design.
Backend projects can highlight your understanding of how to build scalable systems that perform efficiently under different loads. This is essential for applications with growing user bases or large datasets.
Backend projects often involve integrating with third-party services, handling asynchronous tasks, and implementing robust security measures. These aspects demonstrate your ability to work with complex systems and manage various technical requirements.
Backend development requires strong analytical skills to design efficient database schemas, optimize queries, and ensure system reliability. Showcasing these projects reflects your problem-solving abilities and attention to detail.
Employers and clients look for developers who can handle the full spectrum of development tasks. Including backend projects in your portfolio makes you more attractive to potential employers or clients, especially for roles requiring end-to-end development capabilities.
A diverse portfolio that includes backend projects demonstrates your versatility and depth as a developer. It provides a well-rounded view of your skills and experience, helping you stand out in competitive job markets.
In summary, backend projects are vital for demonstrating your technical expertise, problem-solving abilities, and full-stack development skills. They enhance your portfolio by showcasing your capability to build, manage, and optimize server-side systems.
Including backend projects in your portfolio is essential for showcasing a well-rounded skill set and enhancing your marketability. These projects highlight your ability to handle server-side development, manage databases, and integrate APIs, demonstrating full-stack competency.
They also showcase your problem-solving skills by illustrating your ability to tackle complex issues such as data consistency, security, and scalability. By working on backend projects, you can prove your technical expertise with server-side technologies and frameworks and your capability to build scalable and efficient systems.
Additionally, these projects reflect your experience in integrating complex systems and handling asynchronous processes, making your portfolio more comprehensive and attractive to potential employers. Overall, backend projects not only enhance your portfolio by providing a complete view of your development skills but also make you a more versatile and competitive candidate in the job market.
When working on backend projects, selecting the right platforms and tools can significantly enhance your development experience and efficiency. Here are some of the best platforms to consider:
GitHub is an essential platform for version control and collaborative development. It allows you to host your code repositories, track changes, and collaborate with other developers. GitHub also provides a wide range of integrations with other tools and services.
GitLab offers similar functionality to GitHub but also includes built-in Continuous Integration/Continuous Deployment (CI/CD) pipelines, issue tracking, and project management features. It's a comprehensive platform for managing your backend projects throughout the development lifecycle.
Heroku is a cloud platform that simplifies the deployment and scaling of backend applications. It supports multiple programming languages and offers a range of add-ons for databases, monitoring, and more. It's ideal for quickly getting your backend projects up and running.
AWS provides a robust set of cloud services for backend development, including compute power (EC2), databases (RDS, DynamoDB), and serverless options (Lambda). AWS is highly scalable and offers extensive documentation and support.
Google Cloud Platform offers a suite of cloud services for backend development, including virtual machines (Compute Engine), managed databases (Cloud SQL), and serverless computing (Cloud Functions). GCP is known for its powerful data analytics and machine learning tools.
Microsoft Azure provides a wide range of cloud services for building, deploying, and managing backend applications. It offers virtual machines, managed databases, and serverless computing, as well as integrations with various development tools.
DigitalOcean is known for its simplicity and ease of use. It offers scalable virtual private servers (Droplets) and managed databases. It's a great option for developers looking for a straightforward and cost-effective cloud platform.
Docker is a platform for containerizing applications, making it easier to develop, deploy, and run backend projects in isolated environments. Containers ensure consistency across different development and production environments.
Kubernetes is an open-source platform for automating containerized application deployment, scaling, and management. It is ideal for managing complex backend systems and microservices architectures.
Postman is a powerful tool for API development and testing. It allows you to create, test, and document your backend APIs efficiently, making it easier to ensure your APIs work as expected.
These platforms provide a wide range of tools and services to support various aspects of backend development, from version control and deployment to cloud computing and containerization.
Backend development projects focus on building and managing the server-side components of software applications. These projects are crucial for creating the infrastructure that powers the functionality and logic of applications, handles data storage, and manages server-side processes.
1. APIs (Application Programming Interfaces) are a core aspect, allowing different software components to communicate. APIs enable interactions between frontend applications, mobile apps, and backend systems, facilitating operations and data retrieval.
2. Database Management is another critical area, involving the design, implementation, and maintenance of databases. This includes creating schemas, managing data relationships, and optimizing queries. Databases can be relational, like MySQL or PostgreSQL, or non-relational, such as MongoDB or Redis.
3. Authentication and Authorization are essential for secure user management. This involves implementing registration and login systems, handling password management, and setting up role-based access controls, often using technologies like OAuth2, JWT (JSON Web Tokens), and multi-factor authentication.
4. Server-side logic encompasses writing the code that processes client requests, performs computations, and interacts with databases. This includes implementing business rules, validating data, and providing core application functionalities.
5. Data Processing and Analysis involves handling large volumes of data, performing analytics, and generating reports. This can include batch processing, real-time data analytics, and integration with data processing frameworks.
6. Integration with Third-Party Services is common in backend projects, involving the connection with external services and APIs such as payment gateways, email services, or social media platforms. This includes managing API requests and ensuring smooth data exchanges.
7. Microservices Architecture is often used for complex applications where different services manage specific functionalities. This approach supports scalability and maintainability by decoupling services, allowing them to operate independently.
8. Deployment and Scaling involve deploying applications to servers or cloud platforms and managing their scaling to handle varying loads. This includes configuring servers, load balancing, and using containerization technologies like Docker and Kubernetes.
9. Security Measures are crucial for protecting data and ensuring application integrity. This includes encrypting sensitive information, securing API endpoints, and defending against vulnerabilities such as SQL injection and cross-site scripting (XSS).
10. Performance Optimization ensures applications run efficiently by optimizing database queries, improving server response times, and implementing caching strategies to reduce latency.
In essence, backend development projects create the server-side infrastructure necessary for applications to function effectively, securely, and efficiently.
To successfully tackle backend developer projects, certain prerequisites are essential to ensure that you can design, implement, and manage server-side components effectively. Here’s a comprehensive list of prerequisites:
A solid understanding of programming languages commonly used in backend development is crucial. Languages such as JavaScript (Node.js), Python, Java, Ruby, PHP, and C# are widely used. Proficiency in at least one backend language is fundamental for writing server-side code.
Knowledge of how web servers operate is important. Familiarity with server software like Apache, Nginx, or IIS helps in configuring and managing server environments, handling HTTP requests, and optimizing server performance.
A good grasp of database management is necessary. This includes understanding SQL for relational databases (e.g., MySQL, PostgreSQL) and familiarity with NoSQL databases (e.g., MongoDB, Redis) for handling unstructured data. Knowing how to design schemas, write queries, and perform data manipulation is essential.
Experience in designing and implementing APIs is crucial. This involves creating RESTful APIs, understanding HTTP methods, and managing endpoints. Familiarity with tools like Postman for testing APIs and understanding API documentation practices is beneficial.
Understanding authentication mechanisms and security best practices is important. This includes knowledge of OAuth2, JWT (JSON Web Tokens), and concepts like encryption, secure password storage, and protection against common vulnerabilities (e.g., SQL injection, XSS).
Proficiency with version control systems like Git is essential for managing code changes, collaborating with other developers, and maintaining a history of project modifications. Knowing how to use platforms like GitHub or GitLab is also valuable.
Familiarity with the Model-View-Controller (MVC) architecture helps in organizing code and separating concerns in a web application. Understanding how to implement this pattern can streamline the development process and improve code maintainability.
Experience with server-side frameworks and libraries enhances productivity. For instance, Express.js for Node.js, Django or Flask for Python, and Spring Boot for Java provide robust tools and abstractions for building and managing backend applications.
An understanding of basic networking concepts, such as IP addresses, DNS, HTTP/HTTPS protocols, and TCP/IP, is important for backend development. This knowledge helps in troubleshooting connectivity issues and ensuring reliable communication between clients and servers.
Experience with deploying applications to cloud platforms or servers is valuable. Familiarity with services like AWS, Google Cloud Platform, or Azure, as well as containerization tools like Docker and orchestration platforms like Kubernetes, helps in managing and scaling applications effectively.
Knowledge of testing frameworks and debugging techniques is crucial for ensuring that your backend code is reliable and free of bugs. Understanding unit testing, integration testing, and debugging practices can help in maintaining code quality.
Strong analytical and problem-solving skills are essential for tackling complex backend challenges. Being able to design efficient algorithms, optimize performance, and troubleshoot issues effectively is a key part of backend development.
Having these prerequisites in place will equip you with the necessary skills and knowledge to successfully work on backend development projects, ensuring that you can create robust, scalable, and secure server-side applications.
Working on backend projects is essential for several reasons that significantly contribute to your growth as a developer and enhance your career prospects. Backend projects deepen your technical expertise by providing hands-on experience with server-side technologies, databases, and APIs. They offer a deeper understanding of how applications operate behind the scenes, including server management, data handling, and security practices. Engaging in these projects also enhances your problem-solving skills, as backend development often involves tackling complex issues related to data management, system performance, and scalability. Having experience with backend projects broadens your career opportunities. Employers highly value developers who can handle both frontend and backend tasks, making you a more versatile candidate for roles such as full-stack developer, backend engineer, or systems architect.
Additionally, including backend projects in your portfolio builds a comprehensive view of your skills. It showcases your ability to work on server-side components, handle data interactions, and implement business logic, providing a complete picture of your development capabilities. Working on backend projects also improves your understanding of system architecture. You learn about different components like databases, server infrastructure, and microservices and how they interact to form a cohesive application. This knowledge is crucial for designing scalable and maintainable systems. Furthermore, these projects facilitate learning modern tools and technologies such as cloud platforms, containerization, and orchestration, enhancing your ability to manage and deploy applications effectively.
Real-world experience is another benefit of backend projects. They offer practical insights into how large-scale applications are built, maintained, and scaled, which is invaluable for understanding industry standards and best practices. Backend development encourages continuous learning, keeping you updated with the latest trends and advancements in the field. Lastly, working on backend projects often involves collaboration with other developers, designers, and stakeholders. This experience fosters communication and teamwork skills, which are essential for successful project outcomes. Overall, backend projects are crucial for developing a well-rounded skill set, enhancing career opportunities, and gaining practical experience in the field of software development.
To learn backend development effectively, follow these steps:
This approach balances learning with practical experience, ensuring a solid foundation in backend development.
Fynd.Academy is a premier educational platform designed to fast-track your journey to becoming a proficient backend developer. Here’s why Fynd.Academy should be your go-to choice for backend development training:
By choosing Fynd.Academy, you’re investing in a structured, supportive, and practical approach to backend development. Their comprehensive resources and expert guidance ensure you gain the skills needed to excel in the field and advance your career.
Being a backend developer offers several key advantages
These advantages make backend development a rewarding and dynamic career choice.
Backend development is a vital and rewarding field within the tech industry, offering numerous benefits and opportunities for growth. With its high demand, competitive salaries, and critical role in shaping application performance and functionality, backend development stands out as a key career path. The versatility of skills, opportunities for specialization, and involvement with innovative technologies contribute to a dynamic and engaging career.
By mastering backend technologies and engaging in practical projects, you can unlock significant career advancements and make a substantial impact on how software applications operate and deliver value.
Copy and paste below code to page Head section
Backend development involves building and maintaining the server side of web applications. It focuses on managing databases, server logic, authentication, and APIs to ensure that the application functions correctly and efficiently.
Key skills include proficiency in programming languages (such as JavaScript, Python, Java, or PHP), understanding of databases (both SQL and NoSQL), experience with web servers, knowledge of API security practices, and familiarity with backend frameworks and tools.
Popular programming languages for backend development include JavaScript (Node.js), Python, Java, Ruby, PHP, and C#. Each language has its strengths and is used in different contexts.
Frontend development focuses on the user interface and experience, involving technologies like HTML, CSS, and JavaScript. Backend development, on the other hand, deals with the server-side logic, databases, and application functionality that support the front end.
Start by learning a backend programming language, understanding basic web server operations, and working with databases. Build simple projects, create APIs, and gradually explore more complex topics. Online courses, tutorials, and practical experience are key to getting started.
Common backend frameworks include Express.js for Node.js, Django and Flask for Python, Spring Boot for Java, and Ruby on Rails for Ruby. These frameworks provide tools and libraries to streamline development.