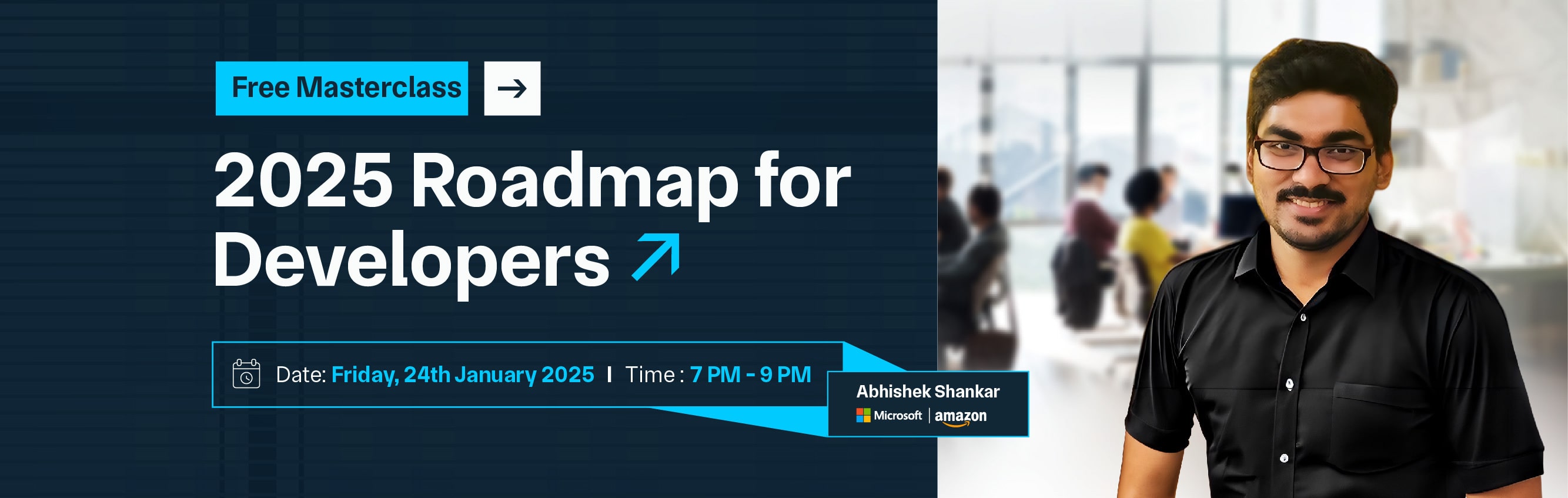

Trees are a fundamental data structure in computer science, offering efficient ways to organize and manage data. One of their primary applications is in hierarchical data representation, such as file systems, where directories and subdirectories are structured as trees, allowing for efficient navigation and retrieval. Trees are also essential in database indexing; B-trees and B+ trees optimize search, insert, and delete operations, significantly improving database performance.
In compiler design, Abstract Syntax Trees (ASTs) represent the structure of source code, aiding in parsing and code generation. Networking benefits from tree structures as well, particularly in routing protocols like Spanning Trees, which ensure efficient data transmission across networks by minimizing loops. In artificial intelligence, decision trees provide a framework for making decisions based on data, while game trees are utilized in strategy games to evaluate possible moves.
Additionally, trees play a critical role in data compression algorithms, such as Huffman coding, which reduces file sizes for efficient storage. Overall, trees enhance the efficiency and effectiveness of various applications, making them a vital component of modern computing.
In data structures, a tree is a hierarchical structure that consists of nodes connected by edges. Each tree has a root node, which is the topmost node, and every other node is a descendant of that root. Here are some key concepts and types of trees in data structures:
Trees are essential for organizing data efficiently and are widely used in various applications, including databases, compilers, and artificial intelligence.
Trees are versatile data structures that find applications across various fields in computer science and real-world scenarios. Here are some key applications:
Tree Structure:
Program Code:
Class Node:
def __init__(self, name):
self.name = name
self.children = []
Class DirectoryTree:
def __init__(self):
self.root = Node("Root")
def add(self, parent_name, child_name):
parent_node = self.find(self.root, parent_name)
if parent_node:
parent_node.children.append(Node(child_name))
def find(self, node, name):
if node.name == name:
return node
For a child in node.children:
result = self.find(child, name)
If result:
return result
return None
Tree Structure:
Program Code:
Class BTreeNode:
def __init__(self, t, leaf=False):
self.t = t # Minimum degree
self.leaf = leaf
self.keys = []
self.children = []
Class BTree:
def __init__(self, t):
self.root = BTreeNode(t, True)
self.t = t
def insert(self, k):
Root = self.root
if len(root.keys) == (2 * self.t) - 1:
new_root = BTreeNode(self.t, False)
new_root.children.append(root)
self.split_child(new_root, 0, root)
self.root = new_root
self.insert_non_full(new_root, k)
Else:
self.insert_non_full(root, k)
def insert_non_full(self, node, k):
# Insert logic here...
def split_child(self, parent, i, full_child):
# Split logic here...
Tree Structure:
Program Code:
Class ExprNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def build_ast(expression):
# Simplified AST construction logic based on expression...
pass
Tree Structure:
Program Code:
Class Graph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
If you not in yourself.graph:
self.graph[u] = []
self.graph[u].append(v)
def prim(self):
# Prim's algorithm implementation for MST...
pass
Tree Structure:
Program Code:
Class DecisionTreeNode:
def __init__(self, feature=None, value=None):
self.feature = feature
self.value = value
self.children = {}
def build_decision_tree(data):
# Decision tree construction logic...
pass
Tree Structure:
Program Code:
class HuffmanNode:
def __init__(self, char, freq):
self.char = char
self.freq = freq
self.left = None
self.right = None
def build_huffman_tree(frequencies):
# Huffman tree construction logic...
pass
Tree Structure:
Program Code:
class GCNode:
def __init__(self, obj):
self.obj = obj
self.children = []
def track_objects(root):
# Logic to traverse and track object references...
pass
Tree Structure:
Program Code:
class QuadTreeNode:
def __init__(self, boundary):
self.boundary = boundary
self.children = []
self.points = []
def insert(qt, point):
# Insertion logic for points in the quadtree...
pass
This structure provides a clear view of each application, its corresponding tree structure, and code examples to illustrate their implementation. Let me know if you need further details or specific implementations!
Trees are versatile data structures that offer numerous advantages in various applications. Here are some key benefits:
Overall, trees offer a powerful combination of efficiency, flexibility, and organization, making them a fundamental choice for many data management and algorithmic applications.
While trees are a powerful data structure with numerous benefits, they also come with certain challenges and considerations that developers and data scientists should keep in mind:
Trees are a fundamental and versatile data structure that plays a crucial role in various applications across computer science and technology. Their hierarchical nature allows for efficient organization and management of data, making them particularly well-suited for representing hierarchical relationships, such as file systems and organizational structures.
In databases, tree structures like B-trees and B+ trees significantly enhance data retrieval and indexing, enabling efficient search operations. In the realm of compilers, Abstract Syntax Trees (ASTs) facilitate the parsing and understanding of source code. At the same time, in artificial intelligence, decision trees, and game trees help in making informed decisions based on data analysis and strategy evaluation.
Copy and paste below code to page Head section
A tree is a hierarchical data structure consisting of nodes connected by edges. It has a root node at the top and can have multiple levels of child nodes, forming a parent-child relationship.
Trees provide efficient searching, insertion, and deletion operations, typically with O(logn)O(\log n)O(logn) complexity in balanced trees. They also offer a natural way to represent hierarchical data and are flexible for various data types.
Challenges include complexity in implementation, memory overhead, potential performance degradation with unbalanced trees, and difficulties in insertion, deletion, and traversal operations.
Balancing techniques vary by tree type. For example, AVL trees use rotations to maintain balance during insertions and deletions, while Red-Black trees enforce specific properties to ensure balanced height.
Yes, but special care must be taken to handle concurrency. Using locking mechanisms or concurrent data structures can help prevent inconsistencies during simultaneous modifications.
A decision tree is a flowchart-like structure used for classification tasks in machine learning. It represents decisions based on feature values, with branches leading to outcomes or further decisions.