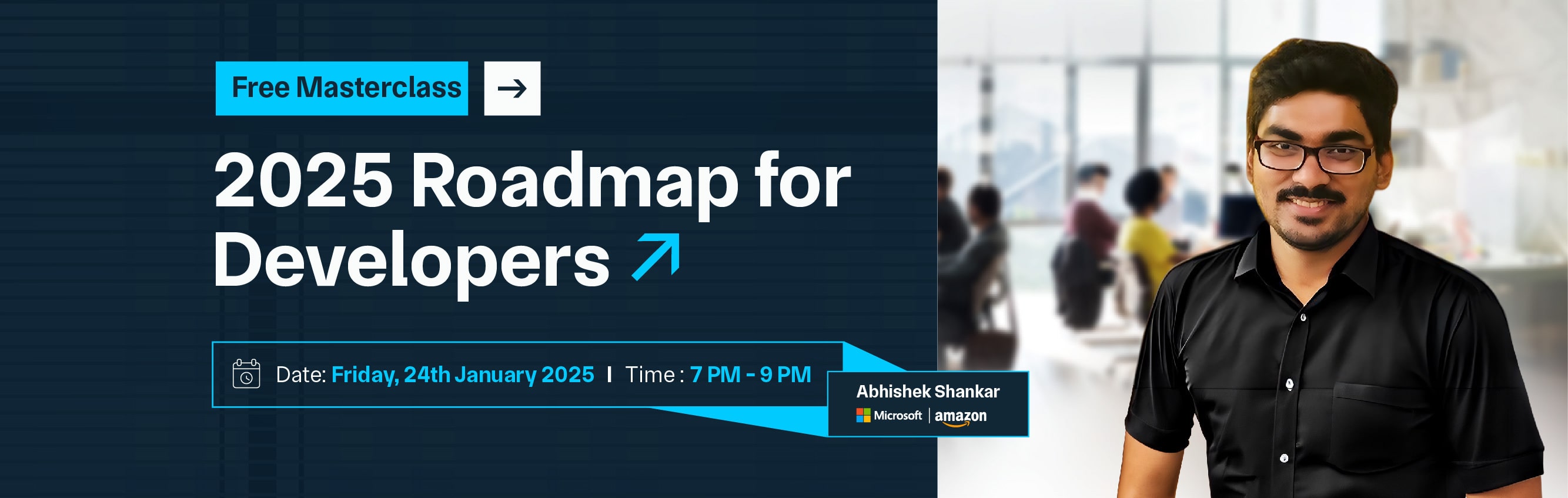

Queues are fundamental data structures that operate on a First-In-First-Out (FIFO) principle, making them essential for various applications in computer science. One primary application is in task scheduling, where processes or tasks are managed in the order they arrive. Operating systems use queues to handle CPU scheduling, ensuring fair allocation of resources to processes.
Another significant application is in data buffering, such as when streaming audio or video; queues temporarily store data to ensure smooth playback. In networking, queues manage packets of data waiting to be processed or transmitted, enhancing communication efficiency. Queues are also crucial in algorithms like breadth-first search (BFS), where they facilitate the exploration of nodes in graph and tree structures level by level.
This makes queues valuable in artificial intelligence for pathfinding and traversing structures. Additionally, queues can be employed in simulation systems to model real-world scenarios, such as customer service lines or resource allocation. Overall, the versatility and efficiency of queues make them indispensable in both theoretical and practical applications, providing a structured approach to managing sequences of data and tasks in various computing contexts.
A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle, meaning that the first element added to the queue will be the first one to be removed. Think of it like a line of people waiting to buy tickets: the person who gets in line first is served.
Queues support two main operations:
In addition to these basic operations, queues often provide methods to check if they are empty and to view the front element without removing it. Queues can be implemented using arrays or linked lists and are widely used in various applications, including task scheduling, resource management in operating systems, and breadth-first search in graph algorithms. Their structured approach to handling data makes queues essential for efficiently managing sequences of tasks or events.
A queue is a fundamental data structure that operates on a First-In-First-Out (FIFO) principle, meaning that the first element added is the first to be removed. It is widely used in various applications, such as task scheduling and resource management. Below are the basic operations of a queue:
The enqueue operation adds an element to the back (or rear) of the queue. When a new item is enqueued, it is placed in the position after the current last item. If the queue is implemented using an array, this may require checking if there's enough space, and if it’s implemented using a linked list, it involves creating a new node and linking it to the current last node. Enqueue operations are essential for inserting data in the order it arrives.
The dequeue operation removes and returns the element at the front of the queue. This operation is crucial for retrieving the next item that was added. In an array implementation, the front element's index is updated, and if necessary, the elements may be shifted forward.
In a linked list implementation, the front node is removed, and the pointer to the front is updated to the next node. Dequeueing maintains the FIFO nature of the queue.
The peek operation allows you to view the front element of the queue without removing it. This is useful when you want to check which element will be dequeued next without altering the state of the queue.
It provides a way to access the data at the front efficiently, ensuring that subsequent dequeue operations remain unaffected. This operation is particularly helpful in scenarios where the next item needs to be inspected before deciding to process it.
The isEmpty operation checks whether the queue is empty by determining if there are any elements present. It returns true if there are no elements in the queue and false otherwise.
This operation is important for preventing errors during dequeue operations, as attempting to remove an item from an empty queue would typically result in an underflow condition. Knowing whether the queue is empty helps manage flow control in applications using queues.
The size operation returns the number of elements currently in the queue. It is a useful metric for understanding how many items are waiting to be processed or managed. In a linked list implementation, this can be achieved by maintaining a count that increments with each enqueue and decrements with each dequeue.
In an array implementation, it can be determined by the difference between the front and rear indices. Knowing the size helps in managing resources effectively and optimizing performance.
Queues come in various types, each designed for specific use cases and operational characteristics. Here are the main types of queues in data structures:
A simple queue operates on the First-In-First-Out (FIFO) principle. Elements are added at the rear and removed from the front. It’s the most basic type of queue, often used for straightforward task scheduling.
In a circular queue, the last position is connected back to the first position, forming a circle. This allows for efficient use of space since it can reuse empty slots without shifting elements, overcoming the limitation of a linear queue where space may be wasted.
A priority queue allows elements to be removed based on their priority rather than their order of insertion. Each element is associated with a priority level, and elements with higher priority are dequeued before those with lower priority, making it ideal for scheduling tasks that need urgent attention.
A deque allows the insertion and removal of elements from both the front and the back. This flexibility makes it suitable for applications that require access to both ends, such as undo mechanisms in software or managing tasks in complex scheduling scenarios.
In an input-restricted deque, insertion is only allowed at one end (either front or rear), while removal can occur from both ends. This structure is useful for certain applications where you want to control input while retaining flexibility in removal.
In an output-restricted deque, removal is only allowed from one end, while insertion can occur at both ends. This setup is useful in scenarios where the output needs to be managed while still allowing for flexibility in data entry.
A blocking queue supports operations that can block the calling thread until the queue has space for more elements or until an element becomes available for removal. This type is often used in concurrent programming to manage communication between threads.
A concurrent queue is designed for safe access by multiple threads. It allows for thread-safe operations, ensuring that elements can be added or removed without causing inconsistencies due to concurrent modifications.
Each type of queue serves specific needs and can be implemented using different underlying data structures, such as arrays or linked lists, depending on the performance requirements and use cases.
Queues are widely used in various applications due to their efficient management of data in a First-In-First-Out (FIFO) manner. Here are some common applications of the queue data structure:
Queues are vital for task scheduling in operating systems, where processes are managed in the order they arrive. The scheduler uses a queue to allocate CPU time fairly, ensuring that all processes get a chance to execute. This FIFO approach helps prevent starvation and ensures efficient use of system resources, contributing to overall system performance and responsiveness.
Queues function as buffers in scenarios like data streaming and I/O operations. They temporarily store data packets, allowing the system to manage differences in processing speeds between producers and consumers.
For instance, in video streaming, queues ensure smooth playback by queuing data even when retrieval is delayed, preventing interruptions and enhancing user experience.
Queues are crucial in graph and tree traversal algorithms, particularly in breadth-first search (BFS). This algorithm explores nodes level by level, storing unvisited nodes in a queue.
By processing nodes in the order they are discovered, BFS efficiently finds the shortest path in unweighted graphs, making it valuable in network routing and other applications that require systematic exploration.
Print spooling utilizes queues to manage print jobs sent to printers. As documents are submitted for printing, they are queued in the order received, ensuring that they are printed sequentially.
This organization prevents chaos and delays, allowing multiple users to send print jobs without conflicts, leading to a smoother printing process and improved user satisfaction.
In call centers, queues manage incoming calls, placing them in line until available agents can answer them. This system ensures that calls are handled in the order they arrive, reducing wait times and providing a structured approach to customer service. By effectively managing call flow, queues improve efficiency and enhance the overall customer experience.
Queues play a significant role in customer service environments like banks or restaurants. They organize customers in the order they arrive, ensuring fair and efficient service. This structured approach reduces wait times and helps staff manage their workload effectively, leading to improved customer satisfaction and streamlined operations.
Queues are essential for handling tasks in real-time systems, such as event handling in user interfaces or processing messages in chat applications. They allow systems to manage incoming data quickly and efficiently, ensuring that urgent tasks are prioritized and processed in a timely manner, which is crucial for maintaining responsiveness and user engagement.
In multithreaded programming, queues facilitate safe access to shared resources. By using a queue to manage requests, threads can communicate and coordinate effectively without causing race conditions.
This control mechanism enhances program stability and ensures that multiple threads can operate smoothly without interfering with each other.
Queues are used in simulation systems to model real-world processes, such as customer arrivals at service points or vehicles at toll booths. By queuing entities, these systems analyze performance metrics, optimize resource allocation, and identify potential bottlenecks. This modeling helps organizations improve efficiency and plan better for varying demand scenarios.
In networking, queues manage data packets waiting to be transmitted or processed. By organizing packets, queues help optimize bandwidth usage and reduce congestion, ensuring that data flows smoothly through the network. This management is critical for maintaining quality of service and minimizing delays in data transmission, particularly in high-traffic environments.
Queues are versatile data structures that operate on a First-In-First-Out (FIFO) basis, making them essential for managing sequences of data and tasks. Their ability to efficiently handle ordered elements allows for a wide range of applications across various fields.
From job scheduling in operating systems to asynchronous data processing in web services, queues play a crucial role in ensuring smooth operations and improved performance in both software and hardware environments. Here are some notable applications of queue data structures.
Queues are used in job scheduling to manage the execution order of tasks. The operating system places jobs in a queue based on their arrival time, ensuring efficient CPU utilization and managing system workload.
In applications where data is produced and consumed at different rates, queues enable asynchronous processing. For example, a producer can enqueue data for a consumer to process later, decoupling the two processes and enhancing performance.
Web servers use queues to manage incoming requests. When multiple users access a server simultaneously, requests are queued, ensuring that they are processed in the order they are received, thus preventing server overload and ensuring fair access.
In event-driven programming, queues are used to manage events that need to be processed. Events are enqueued as they occur, allowing the system to handle them in the order they are generated, which is crucial for responsive applications.
In load-balancing scenarios, queues help distribute incoming requests across multiple servers. By queuing requests, the load balancer can efficiently route them to servers that are less busy, optimizing resource usage and response times.
Queues model various real-world processes, such as customers waiting in line at a store or tasks waiting to be executed in a computing environment. This modeling aids in analyzing and improving system performance.
In distributed systems, message queues facilitate communication between different components. They enable asynchronous message delivery, allowing services to send and receive messages without needing to be directly connected, thus improving system resilience and scalability.
Queues are useful in managing data streams, such as sensor data in IoT applications. They allow for temporary storage and processing of data as it flows in, ensuring timely analysis and action based on real-time inputs.
Many applications implement queues to manage undo operations. Actions taken by users are enqueued, and when an undo request is made, the most recent action is dequeued and reversed, allowing for efficient state management.
In multimedia applications, such as video editing or audio processing, queues are used to manage data streams. They can buffer video frames or audio samples, ensuring smooth playback and editing by organizing data for timely processing.These applications highlight the versatility of queues across different domains, showcasing their ability to manage and process data in a variety of contexts efficiently.
Queues play a vital role in operating systems, facilitating various essential functions that enhance efficiency and resource management. Here are some key applications of queue data structures in operating systems:
Queues are used to manage the scheduling of processes. When processes are created, they are placed in a ready queue. The scheduler uses this queue to determine which process to execute next, ensuring fair allocation of CPU time and optimizing system performance.
In batch processing systems, job queues manage the order of job execution. Jobs submitted to the system are queued based on arrival time or priority, allowing the operating system to process them in an orderly fashion and maintain efficient resource utilization.
Operating systems use queues to manage input/output requests. When processes request access to I/O devices, such as disks or printers, these requests are queued. The system processes them in the order they arrive, ensuring that all processes get fair access to resources.
Queues assist in memory management tasks, such as paging. When a page fault occurs, the system can use a queue to track which pages need to be loaded into memory, ensuring efficient use of memory resources and minimizing delays.
Queues facilitate communication between processes in IPC mechanisms. Message queues allow processes to send and receive messages in an ordered manner, enabling coordination and synchronization between concurrent processes.
In multi-threaded environments, queues are used to manage thread execution. Threads waiting for CPU time can be placed in a queue, allowing the scheduler to prioritize and allocate resources effectively based on various criteria.
Queues are employed in event-driven systems to manage events that require processing. Events are queued as they occur, enabling the operating system to handle them in the order they are generated, which is crucial for responsiveness.
Operating systems use queues to manage requests for resources such as CPU, memory, and I/O devices. By queuing resource requests, the system can allocate resources efficiently and prevent deadlocks.
In network systems, queues manage incoming and outgoing packets. Queues ensure that data packets are processed in the order they arrive, maintaining the integrity of communications and preventing packet loss.
Queues are used to collect and manage system logs and monitoring data. By queuing log entries, the operating system can efficiently process and analyze logs without overwhelming the system with immediate write operations.
These applications illustrate how queues are integral to the efficient functioning of operating systems, helping to manage processes, resources, and communication in a structured manner.
Queues are fundamental data structures that play a crucial role in various algorithms across computer science. Their First-In-First-Out (FIFO) nature makes them ideal for specific tasks where order and timing are essential. Here are some key areas where queues are utilized in algorithms:
BFS is a graph traversal algorithm that explores all nodes at the present depth before moving on to nodes at the next depth level. A queue is used to keep track of nodes to be explored, ensuring that nodes are processed in the order they are discovered. This approach is particularly effective in finding the shortest path in unweighted graphs.
In Dijkstra's algorithm for finding the shortest paths from a source node to all other nodes in a weighted graph, a priority queue is often used. The queue helps efficiently retrieve the node with the smallest tentative distance, optimizing the algorithm's performance.
Queues are used in various job scheduling algorithms to manage processes in operating systems. For example, round-robin scheduling utilizes a simple queue to ensure that each process gets an equal share of CPU time, processing tasks in a fair and orderly manner.
In tree data structures, level order traversal (or breadth-first traversal) uses a queue to explore nodes level by level. Nodes are enqueued as they are discovered, allowing for systematic processing of each level before moving to the next.
Queues are essential in simulation algorithms, such as those modeling customer service scenarios (like queues at a bank). They help track the state of the system, manage incoming entities, and ensure accurate timing of events.
In network flow problems, queues are utilized to manage nodes and paths efficiently. Algorithms like the Ford-Fulkerson method use queues to keep track of vertices during path searching, facilitating the calculation of maximum flow in a network.
Some backtracking algorithms can employ queues to manage states and decisions. By queuing potential solutions, the algorithm can explore paths systematically and revert to previous states as necessary.
In event-driven algorithms, queues manage events that need to be processed. Events are enqueued as they occur, allowing the program to handle them in the order of arrival, which is crucial for maintaining responsiveness in applications.
This hybrid algorithm combines the depth-first search's space efficiency with the breadth-first search's completeness. It uses a queue to manage iterations and levels, enabling systematic exploration of nodes.
Some caching algorithms use queues to manage data efficiently. For example, the Least Recently Used (LRU) cache can implement a queue to track the order of access to items, helping to decide which items to evict when the cache reaches its limit.
These applications demonstrate how queues are integral to the design and implementation of algorithms, providing structured ways to manage data flow and processing order in various computational tasks.
Implementing a queue using arrays in Java involves creating a class that manages the queue's elements, keeps track of the front and rear indices, and provides the basic operations: enqueue, dequeue, peek, and isEmpty. Here’s a simple implementation:
class ArrayQueue {
private int[] queue;
private int front;
private int rear;
private int capacity;
private int size;
// Constructor to initialize the queue
public ArrayQueue(int capacity) {
this.capacity = capacity;
queue = new int[capacity];
front = 0;
rear = -1;
size = 0;
}
// Method to add an element to the queue
public void enqueue(int item) {
if (size == capacity) {
System. out.println("Queue is full! Cannot enqueue " + item);
return;
}
rear = (rear + 1) % capacity; // Circular increment
queue[rear] = item;
size++;
System.out.println("Enqueued: " + item);
}
// Method to remove and return the front element
public int dequeue() {
if (isEmpty()) {
System. out.println("Queue is empty! Cannot dequeue.");
return -1; // Indicating an empty queue
}
int item = queue[front];
front = (front + 1) % capacity; // Circular increment
size--;
System.out.println("Dequeued: " + item);
return item;
}
// Method to return the front element without removing it
public int peek() {
if (isEmpty()) {
System. out.println("Queue is empty! No front element.");
return -1; // Indicating an empty queue
}
return queue[front];
}
// Method to check if the queue is empty
public boolean isEmpty() {
return size == 0;
}
// Method to get the current size of the queue
public int size() {
return size;
}
}
// Main class to demonstrate the queue implementation
public class QueueDemo {
public static void main(String[] args) {
ArrayQueue queue = new ArrayQueue(5); // Creating a queue with capacity 5
queue.enqueue(10);
queue.enqueue(20);
queue.enqueue(30);
System.out.println("Front element: " + queue.peek());
queue.dequeue();
queue.enqueue(40);
queue.enqueue(50);
queue.enqueue(60); // This will indicate that the queue is full
System.out.println("Current size: " + queue.size());
queue.dequeue();
queue.dequeue();
System.out.println("Front element: " + queue.peek());
}
}
While queues are incredibly useful in various applications, they come with some challenges and issues that can affect their performance and effectiveness. Here are some common issues associated with the applications of queue data structures:
In array-based implementations, queues have a fixed size, which can lead to overflow if the queue reaches its capacity. This limitation can hinder performance, especially in applications with unpredictable workloads, requiring dynamic resizing or linked list implementations to overcome.
If a dequeue operation is attempted on an empty queue, it leads to an underflow condition, which can cause errors or exceptions in the application. Proper error handling is necessary to prevent crashes or unintended behavior.
In certain implementations, especially with arrays, memory can be wasted if elements are dequeued from the front, leaving empty spaces that cannot be reused until the queue is reset. This can be mitigated with circular queues but may complicate the implementation.
Implementing queues with advanced features, like priority queues or concurrent queues, can introduce complexity. Managing the priority of elements or ensuring thread safety requires additional logic and careful design.
In systems with high throughput, queues can introduce latency. For instance, in producer-consumer scenarios, if the queue is not processed quickly enough, it may lead to delays and bottlenecks, affecting overall system performance.
Standard queues operate on a strict FIFO principle, which may not be suitable for all applications. For instance, situations that require prioritizing certain tasks may necessitate alternative data structures, such as priority queues or stacks.
In scenarios where queues are used for resource allocation (like I/O devices), managing multiple queues for different resources can become complex. This can lead to inefficiencies if not handled properly.
In multithreaded environments, managing access to a queue can lead to race conditions if not implemented correctly. Ensuring thread safety adds overhead and complexity to the queue implementation.
In applications like call centers or customer service systems, if incoming requests exceed the processing capability, the queue can become saturated. This can result in increased wait times and poor user experience.
In real-time applications, queues may introduce unacceptable latency. If tasks must be processed within strict time limits, using queues can complicate meeting those requirements.
These issues highlight the need for careful consideration when implementing and using queues in applications. Solutions such as dynamic sizing, circular implementations, or alternative data structures can help mitigate some of these challenges.
Navigating the queue landscape involves understanding the various types of queues, their applications, and the challenges they present. Queues are essential for managing tasks and data efficiently in numerous domains, from operating systems to real-time applications. However, it’s crucial to be aware of issues such as fixed size limitations, underflow conditions, and concurrency challenges.
By leveraging the right type of queue for specific applications whether it’s a simple queue, priority queue, or circular queue developers can optimize performance and resource management. Additionally, implementing robust error handling and considering scalability can enhance the reliability of queue systems.
Ultimately, a well-structured approach to queues can significantly improve the efficiency and effectiveness of software solutions, enabling smoother workflows and better user experiences.
Queues are a fundamental data structure utilized across various programming languages, each offering unique implementations and features tailored to their syntax and paradigms. Here’s an overview of how queues are represented and utilized in different programming languages:
In Java, queues can be implemented using the Queue interface, which is part of the Java Collections Framework. The LinkedList class is commonly used to implement a queue due to its dynamic size and efficient operations. Java also provides PriorityQueue for priority-based ordering.
import java.util.LinkedList;
Import java. Util.Queue;
public class QueueExample {
public static void main(String[] args) {
Queue<Integer> queue = new LinkedList<>();
queue.add(1);
queue.add(2);
System.out.println(queue.poll()); // Outputs: 1
}
}
Python provides a built-in queue module, which includes various types of queues like Queue, LifoQueue, and PriorityQueue. The collections. Deque is also widely used for queue implementation due to its fast appends and pops from both ends.
from collections import deque
queue = deque()
queue.append(1)
queue.append(2)
print(queue.popleft()) # Outputs: 1
In C++, the Standard Template Library (STL) provides the queue container adapter. It uses underlying containers like deque or list to manage elements. C++ queues provide efficient operations for enqueueing and dequeueing.
#include <iostream>
#include <queue>
int main() {
std::queue<int> q;
q.push(1);
q.push(2);
std::cout << q.front(); // Outputs: 1
q.pop();
}
JavaScript does not have a built-in queue structure, but queues can be easily implemented using arrays. The push method can add elements to the end, and the shift can remove elements from the front.
let queue = [];
queue.push(1);
queue.push(2);
console.log(queue.shift()); // Outputs: 1
In C#, the Queue<T> class from the System.Collections.Generic namespace provides a straightforward way to implement queues. It supports generic types and offers methods for adding and removing elements.
using System;
Using System.Collections.Generic;
class Program {
static void Main() {
Queue<int> queue = new Queue<int>();
queue.Enqueue(1);
queue.Enqueue(2);
Console.WriteLine(queue.Dequeue()); // Outputs: 1
}
}
Ruby provides an easy way to implement queues using arrays or the Queue class from the standard library, which is thread-safe. The << operator is commonly used to enqueue elements, while shift is used to dequeue.
Queue = Queue. new
queue.push(1)
queue.push(2)
Puts queue.pop # Outputs: 1
In Go, queues can be implemented using slices or by defining a custom struct. The language doesn’t provide a built-in queue but allows developers to create their own using channels for concurrency.
package main
import "fmt"
func main() {
queue := []int{}
queue = append(queue, 1)
queue = append(queue, 2)
fmt.Println(queue[0]) // Outputs: 1
queue = queue[1:] // Dequeue
}
The application of queues in data structures is vast and varied, serving essential roles across multiple domains, including operating systems, data processing, and real-time systems. Their First-In-First-Out (FIFO) nature ensures that tasks are handled in the order they arrive, promoting fairness and efficiency in resource management.
Despite some challenges, such as fixed-size limitations and potential performance bottlenecks, the versatility of queues allows them to be adapted for numerous use cases. By effectively implementing queues, developers can improve system performance, enhance user experience, and streamline workflows, making queues a vital component in the toolkit of data structures.
Copy and paste below code to page Head section
Queues are used in operating systems for process scheduling, managing I/O requests, and handling job scheduling. They ensure that processes are executed in an orderly fashion, improving system efficiency.
Web servers use queues to manage incoming requests. When multiple requests arrive simultaneously, they are queued to ensure that each request is processed in the order it was received, preventing server overload.
Yes, queues are essential in network applications for managing data packets. They ensure that packets are processed in the order they arrive, maintaining the integrity and flow of communication.
In event-driven programming, queues manage events that need processing. Events are enqueued as they occur, allowing the system to handle them in the order they arrive, ensuring responsiveness.
Queues are used in simulation algorithms to model real-world processes, such as customers waiting in line. They help track the state of the system and manage the timing of events effectively.
Yes, queues can be used to manage undo operations in applications. Actions are enqueued, and when an undo is requested, the most recent action is dequeued and reversed.