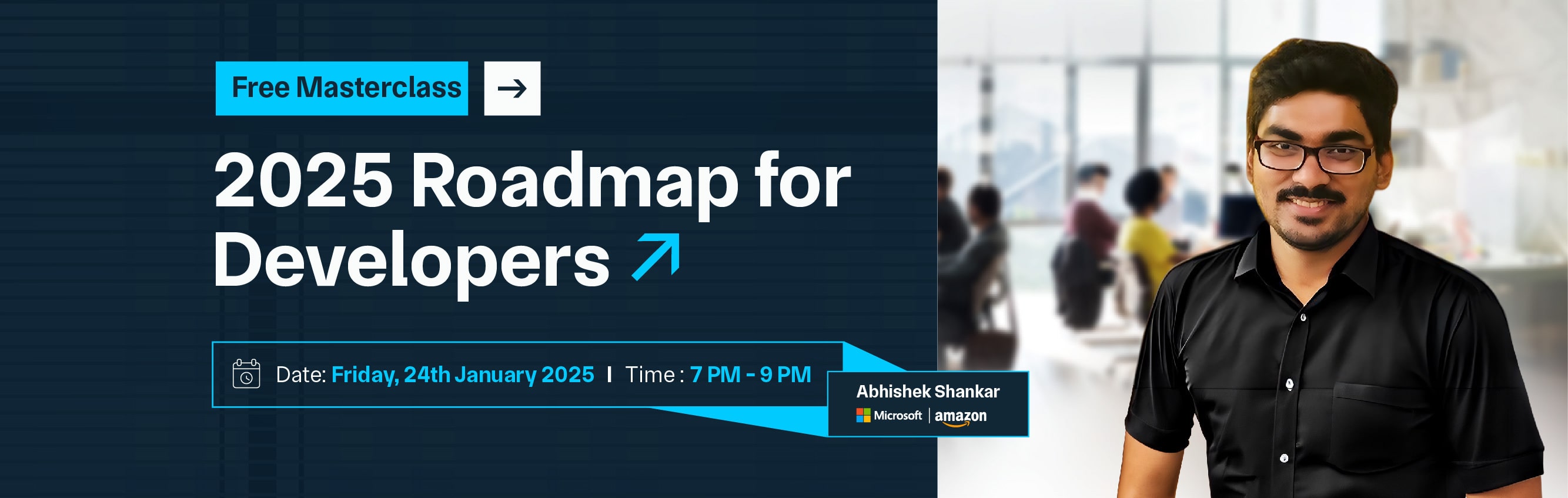

APIs (Application Programming Interfaces) in JavaScript enable communication between different software components, allowing web applications to interact with external servers, databases, or services. JavaScript APIs can be broadly categorized into Web APIs (for frontend interaction with remote services), Browser APIs (for interacting with the browser's environment), and Node.js APIs (for server-side functionality). In web development, the Fetch API is the most commonly used method for making HTTP requests to interact with web APIs.
This API allows developers to retrieve, send, update, or delete data from a server using HTTP methods like GET, POST, PUT, and DELETE. Fetch returns a promise, making it easier to handle asynchronous operations. JavaScript can easily parse and manipulate API responses by utilizing JSON as the data format. For better readability and cleaner code, modern JavaScript also supports async/await, which allows developers to write asynchronous code in a synchronous-like manner.
Additionally, handling errors is essential in API requests; developers need to manage both network issues and unexpected responses. Authentication through API keys or Bearer tokens is often necessary to interact with secure APIs. Overall, JavaScript APIs are foundational for creating dynamic, data-driven applications, enabling developers to build interactive and feature-rich web experiences.
An API (Application Programming Interface) is a set of rules that allows different software applications to communicate with each other. In the context of JavaScript, APIs enable web pages and applications to interact with external services, databases, or other resources, allowing developers to fetch, send, or manipulate data. APIs are essential for creating dynamic, interactive web applications by allowing them to access external data without reloading the page.
In JavaScript, APIs can be divided into Web APIs, Browser APIs, and Node.js APIs:
By utilizing these APIs, JavaScript enables developers to build feature-rich, responsive, and data-driven web applications that can easily communicate with both internal and external systems.
JavaScript APIs are interfaces that allow developers to interact with various services, systems, or features within a web environment or server-side runtime like Node.js. These APIs enable dynamic behavior and data exchange between applications, allowing them to create rich, interactive, and data-driven user experiences. JavaScript APIs can be broadly categorized into three main types: Web APIs, Browser APIs, and Node.js APIs.
Web APIs allow JavaScript applications to interact with external services or resources over the internet. These APIs typically communicate using HTTP (Hypertext Transfer Protocol) requests and often return data in formats like JSON or XML. Web APIs can be used for tasks like fetching data, submitting forms, or interacting with third-party services.
Examples of Web APIs:
Use Case: Fetching data from a server or interacting with a third-party service like a payment gateway or social media platform.
Browser APIs, or Client-Side APIs, are built into web browsers and provide functionalities for interacting with the user's environment. These APIs allow JavaScript to access and modify elements in the browser, manage local storage, and even interact with the device hardware (e.g., geolocation, camera).
Examples of Browser APIs:
Use Case: Creating interactive web pages, storing user data locally, or enabling geolocation-based features.
Node.js provides a range of server-side APIs that allow developers to interact with the operating system, file system, and network. These APIs are used to create scalable backend services and manage server-side tasks like handling HTTP requests, reading and writing files, and managing processes.
Examples of Node.js APIs:
Use Case: Building web servers, handling file uploads, or performing backend operations like database queries.
Web APIs are an essential part of modern web development, enabling JavaScript applications to communicate with external servers or services over the internet. By using Web APIs, developers can fetch data, send data, and interact with third-party services like social media platforms, payment gateways, or weather services, all without refreshing the page. In this section, we will explore how to work with Web APIs in JavaScript, focusing on the Fetch API and the key steps involved in making HTTP requests.
When working with Web APIs, the most common HTTP methods are:
Each method serves a specific purpose and is used depending on the type of operation you need to perform with the API.
The Fetch API is a native JavaScript API that allows you to make HTTP requests to a server. It returns a promise, which makes it easier to handle asynchronous operations like API calls. The Fetch API is more modern and flexible than the older XMLHttpRequest.
fetch(url, options)
.then(response => response.json()) // Parse response body as JSON
.then(data => console.log(data)) // Handle the data
.catch(error => console.log('Error:', error)); // Handle any errors
A GET request is the most common operation for fetching data from an API.
fetch('https://api.example.com/data') // URL of the API
.then(response => response.json()) // Parse JSON response
.then(data => console.log(data)) // Log the data
.catch(error => console.log('Error:', error)); // Handle any errors
In this example, we’re making a simple GET request to an API endpoint (https://api.example.com/data). The response.json() method is used to parse the returned JSON data.
A POST request is typically used to send data to the server, such as submitting a form or creating a new resource.
fetch('https://api.example.com/data', {
method: 'POST', // Specify the HTTP method
headers: { // Define the headers
'Content-Type': 'application/json' // Indicate the content type
},
body: JSON.stringify({ // Send data as JSON
name: 'John Doe',
age: 30
})
})
.then(response => response.json()) // Parse response as JSON
.then(data => console.log(data)) // Handle the data
.catch(error => console.log('Error:', error)); // Handle errors
age: 30
})
})
.then(response => response.json()) // Parse response as JSON
.then(data => console.log(data)) // Handle the data
.catch(error => console.log('Error:', error)); // Handle errors
In this example, we send a POST request to create a new resource. We include a headers object to tell the server that we're sending JSON data, and the body contains the JSON object we want to send.
When you interact with a Web API, you’ll typically receive a response from the server. The response may contain data (in JSON, XML, etc.), or it may just be an acknowledgment (e.g., status code 200 for success).
You can check the response status using the .ok property to ensure the request was successful. If the request fails, you can handle the error appropriately.
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json(); // Parse the JSON response
})
.then(data => console.log(data)) // Handle the data
.catch(error => console.log('Error:', error)); // Handle errors
In this example, if the response.ok property is false (indicating a failed request), and an error is thrown and handled in the .catch() block.
The async/await syntax is a modern approach to working with asynchronous code, making it easier to read and write. It allows you to write asynchronous code more synchronously, improving clarity and avoiding callback hell.
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data'); // Wait for the response
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json(); // Wait for the data to be parsed
console.log(data);
} catch (error) {
console.log('Error:', error); // Handle any errors
}
}
fetchData(); // Call the async function
Here, await is used to pause the function until the response is fetched and parsed, simplifying error handling and making the code more readable.
Proper error handling is crucial when working with Web APIs, as network issues, invalid responses, or server errors can occur. JavaScript’s Promise API provides a .catch() method to handle errors that may arise from a failed API call.
You should always check the response status and handle different HTTP status codes appropriately, such as 404 (Not Found) or 500 (Internal Server Error).
The Fetch API is a modern, promise-based API for making asynchronous HTTP requests in JavaScript. It is designed to replace older methods like XMLHttpRequest with a simpler, more flexible approach.
The Fetch API allows developers to request resources from a server, handle responses, and manage errors, all in an intuitive way. It’s used for fetching data, submitting data, and interacting with various web services over the network.
The Fetch API works by calling the fetch() function, which returns a promise that resolves to the Response object representing the response to the request. You can then parse the response as JSON or text and handle it accordingly.
Basic Syntax:
fetch(url, options)
.then(response => response.json()) // Parse the response as JSON
.then(data => console.log(data)) // Process the data
.catch(error => console.log('Error:', error)); // Handle errors
A GET request is the most common way to retrieve data from a server. With the Fetch API, making a GET request is straightforward. By default, fetch() uses the GET method if no other method is specified.
Example - GET Request:
fetch('https://api.example.com/data') // URL of the API
.then(response => response.json()) // Parse the response as JSON
.then(data => console.log(data)) // Handle the received data
.catch(error => console.log('Error:', error)); // Handle any errors
In this example, fetch() sends a GET request to the provided URL (https://api.example.com/data), and when the response is returned, it is parsed as JSON and logged to the console.
A POST request is used to send data to the server, for example, when submitting a form or creating a new resource.
Example - POST Request:
fetch('https://api.example.com/data', {
method: 'POST', // Specify the HTTP method
headers: { // Define headers for the request
'Content-Type': 'application/json' // Specify the content type
},
body: JSON.stringify({ // Send data in the request body
name: 'John Doe',
age: 30
})
})
.then(response => response.json()) // Parse the response as JSON
.then(data => console.log(data)) // Handle the response data
.catch(error => console.log('Error:', error)); // Handle any errors
In this example, the method: 'POST' specifies that we’re sending a POST request. The body of the request contains the data being sent to the server, which is stringified as JSON.
The response status is crucial in understanding whether the request was successful. The Fetch API does not automatically throw an error for HTTP error statuses (e.g., 404, 500). Instead, you should check the response.ok property to verify that the response was successful.
Example - Handling Response Status:
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) { // Check if the response was successful
throw new Error('Network response was not ok');
}
return response.json(); // Parse the response as JSON
})
.then(data => console.log(data)) // Handle the response data
.catch(error => console.log('Error:', error)); // Handle errors
Here, we check response.ok to see if the HTTP status code indicates a successful request. If the request fails, we throw an error, which is caught and handled in the .catch() block.
While .then() is commonly used with Fetch, async/await provides a cleaner and more readable approach to handling asynchronous code. It allows you to write asynchronous code as though it were synchronous, making it easier to manage.
Example - Using Async/Await:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data'); // Wait for the response
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json(); // Wait for the response to be parsed as JSON
console.log(data);
} catch (error) {
console.log('Error:', error); // Handle any errors
}
}
fetchData(); // Call the async function
In this example, await is used to pause the function’s execution until the promise returned by fetch() resolves, and we can then handle the response.
Proper error handling is vital when working with the Fetch API. Errors can occur due to network issues, invalid responses, or if the API server fails. You should use .catch() or a try/catch block (with async/await) to handle errors appropriately.
Example - Handling Fetch Errors:
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('API call failed');
}
The .catch() block captures network errors (e.g., no internet connection) or failed HTTP responses (e.g., 404 or 500 status codes).
JSON (JavaScript Object Notation) is a lightweight data format used for storing and exchanging data, and it is commonly used in web APIs for sending and receiving data. It is easy to read and write for humans and easy to parse and generate for machines. Since JSON is a text-based format, it can be easily transferred between a server and a client.
JavaScript natively supports JSON through the JSON object, which provides methods to parse JSON data into JavaScript objects and convert JavaScript objects into JSON strings. Here's how you can work with JSON in JavaScript.
When you receive data from a web API or from a file that is in JSON format (often as a string), you need to convert it into a JavaScript object before you can use it. This is done using the JSON.parse() method.
// A JSON string
const jsonString = '{"name": "John", "age": 30, "city": "New York"}';
// Convert JSON string to JavaScript object
const parsedObject = JSON.parse(jsonString);
// Accessing data from the parsed object
console.log(parsedObject.name); // Output: John
console.log(parsedObject.age); // Output: 30
console.log(parsedObject.city); // Output: New York
JSON.parse() takes a JSON string and returns a JavaScript object. Once it's parsed, you can work with it like any other object.
If you want to send data to a server or store it in a text-based format, you need to convert a JavaScript object into a JSON string. This is done using the JSON.stringify() method.
// A JavaScript object
const person = {
name: "John",
age: 30,
city: "New York"
};
// Convert JavaScript object to JSON string
const jsonString = JSON.stringify(person);
// Display the JSON string
console.log(jsonString);
// Output: {"name":"John","age":30,"city":"New York"}
JSON.stringify() converts a JavaScript object into a JSON-formatted string. You can send this string as part of an HTTP request, store it, or log it.
When interacting with web APIs, handling the responses properly is crucial to ensure that your application can handle both successful and unsuccessful requests. API responses often come in various formats (commonly JSON), and they may contain useful data or indicate errors that require special attention. In this section, we’ll explore the key steps for handling API responses in JavaScript, including status checks, parsing response data, and error handling.
When you make an API request, the response from the server will contain a status code that indicates whether the request was successful or if there was an error. In the Fetch API, the response.ok property can help you easily check if the request was successful (status code 200–299) or if an error occurred (status code 400–500).
Example - Checking Response Status:
fetch('https://api.example.com/data')
.then(response => {
// Check if the response status is OK (status code 200-299)
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json(); // Parse the JSON data
})
.then(data => console.log(data)) // Handle the data
.catch(error => console.log('Error:', error)); // Handle errors
API responses often contain structured data in the form of JSON, XML, or text. In most cases, when working with web APIs, the data returned is JSON. Using the Fetch API, you can easily parse the response data with the .json() method.
Example - Parsing JSON Data from API Response:
fetch('https://api.example.com/data')
.then(response => response.json()) // Parse the JSON response
.then(data => {
console.log(data); // Handle the data, typically accessing the properties of the object
})
.catch(error => console.log('Error:', error)); // Handle errors
When working with APIs that require authentication in JavaScript, you typically pass credentials such as API keys, bearer tokens, or OAuth tokens in the request to gain access to protected resources. API keys are often included either as query parameters or in the request headers. At the same time, more secure bearer tokens are generally sent in the Authorization header in the format Bearer <token>. Bearer tokens are commonly used with OAuth 2.0, a more complex authentication protocol where users authenticate through a third-party service (like Google or Facebook) and receive an access token for making API requests. OAuth 2.0 typically involves redirecting the user to an external authorization page and then exchanging an authorization code for the access token.
In addition, session authentication via cookies is frequently used, where the browser automatically includes session cookies in requests to authenticate the user. However, managing token expiration is important, as tokens may become invalid over time, requiring the user to refresh their token or login again. Proper handling of errors such as 401 (Unauthorized) status codes, which indicate token expiration or invalid credentials, ensures secure and reliable API communication. By correctly managing authentication and token lifecycle, you can safeguard sensitive data and maintain smooth interaction with web services.
Copy and paste below code to page Head section
API authentication is the process of verifying the identity of a user or application requesting an API. It ensures that only authorized users or services can access protected resources. Common methods of API authentication include API keys, bearer tokens, and OAuth 2.0.
To authenticate an API request with an API key, you typically include the key either in the query string or in the request headers. For example, send the key as ?api_key=YOUR_API_KEY in the URL or the Authorization header like Authorization: Bearer YOUR_API_KEY.
A Bearer token is a security token used in authentication that is passed in the Authorization header of an HTTP request. It is often issued after the user successfully logs in via OAuth 2.0. The format is usually Authorization: Bearer <token>, and the server verifies the token to grant access to protected resources.
OAuth 2.0 is a more complex authentication framework that allows third-party applications to access user data without requiring the user to share their credentials. In JavaScript, OAuth 2.0 is often implemented by redirecting users to an authentication provider (e.g., Google, Facebook), where they grant permission for the app to access their data. Once authorized, the app receives an access token to use in subsequent API requests.
Tokens, such as Bearer tokens or OAuth access tokens, may expire after a certain period. When this happens, the API will return a 401 (Unauthorized) status code. To handle this, you can refresh the token (if a refresh token is provided) or prompt the user to log in again to obtain a new access token.
Cookies are commonly used for session-based authentication. When a user logs in, the server sends a session cookie containing authentication details (like a session ID or token). On subsequent requests, the browser automatically includes this cookie in the request, allowing the server to verify the user's identity without needing to pass credentials manually.