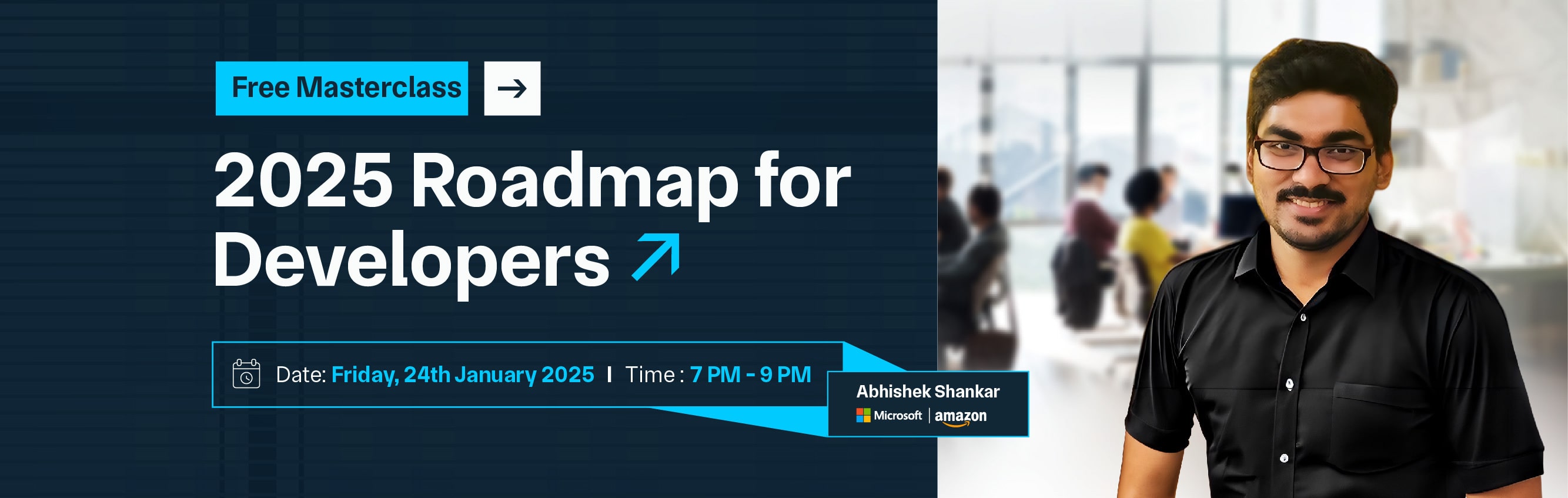

Calling an API in Angular involves several key steps that enable you to retrieve or send data to a server efficiently. First, you'll need to import the HttpClientModule in your Angular module. This module provides a robust way to handle HTTP requests. Once imported, you can inject the HttpClient service into your component or service.To make an API call, you can use methods like get(), post(), put(), or delete() provided by the HttpClient.
For instance, to retrieve data, you would typically use the get() method, which returns an Observable. You can subscribe to this Observable to handle the data once it’s received. It's essential to manage errors by using the catchError operator from RxJS, ensuring your application can gracefully handle issues like network errors.Additionally, define interfaces to model the data you expect from the API, providing better type safety.
Finally, remember to unsubscribe from Observables when the component is destroyed to prevent memory leaks. By following these steps, you can effectively integrate APIs into your Angular applications, enhancing their functionality and user experience.
Setting up the Angular environment for API calls involves several steps to ensure you can effectively communicate with your backend services. Here’s how to get started:
npm install -g @angular/cli
ng new my-app
cd my-app
import { HttpClientModule } from '@angular/common/http';
@NgModule({
imports: [HttpClientModule],
})
ng generate service API
Use HttpClient: In your service, import HttpClient and create methods for your API calls:
constructor(private http: HttpClient) {}
getData(): Observable<any> {
return this.http.get('https://api.example.com/data');
}
With these steps, you'll be ready to make API calls in your Angular application!
Making API calls in an Angular app involves several steps. Here’s a concise step-by-step guide:
Create a New Project:
ng new my-app
cd my-app
Open app.module.ts and import HttpClientModule:
import { HttpClientModule } from '@angular/common/http';
@NgModule({
imports: [
BrowserModule,
HttpClientModule // Add this line
],
})
export class AppModule { }
Generate a Service:
ng generate service API
Implement API Methods in api.service.ts:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
provided: 'root,'
})
export class ApiService {
private apiUrl = 'https://api.example.com/data';
constructor(private http: HttpClient) {}
getData(): Observable<any> {
return this.http.get(this.apiUrl);
}
postData(data: any): Observable<any> {
return this.http.post(this.apiUrl, data);
}
}
Inject the Service in your component (e.g., app.component.ts):
import { Component, OnInit } from '@angular/core';
import { ApiService } from './api.service';
@Component({
selector: 'app-root,'
templateUrl: './app.component.html',
})
Export class AppComponent implements OnInit {
data: any;
constructor(private apiService: ApiService) {}
ngOnInit() {
this.apiService.getData().subscribe(response => {
this.data = response;
});
}
}
Update the Component Template (e.g., app.component.html):
<div *ngIf="data">
<pre>{{ data | json }}</pre>
</div>
Implement Error Handling in your service using catchError:
import { catch error } from 'rxjs/operators';
import { throwError } from 'rxjs';
getData(): Observable<any> {
return this.http.get(this.apiUrl).pipe(
catchError(error => {
console.error('Error occurred:', error);
return throwError(error);
})
);
}
Using the HttpClient library in Angular is straightforward and powerful for making HTTP requests. Here's a step-by-step guide on how to use it:
Create a New Project (if you haven't already):
ng new my-app
cd my-app
Open app.module.ts and import HttpClientModule:
import { HttpClientModule } from '@angular/common/http';
@NgModule({
imports: [
BrowserModule,
HttpClientModule // Add this line
],
})
export class AppModule { }
Generate a Service:
ng generate service API
Implement API Methods in api.service.ts:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
provided: 'root,'
})
export class ApiService {
private apiUrl = 'https://api.example.com/data';
constructor(private http: HttpClient) {}
// GET request
getData(): Observable<any> {
return this.http.get<any>(this.apiUrl);
}
// POST request
postData(data: any): Observable<any> {
return this.http.post<any>(this.apiUrl, data);
}
}
Inject the Service in your component (e.g., app.component.ts):
import { Component, OnInit } from '@angular/core';
import { ApiService } from './api.service';
@Component({
selector: 'app-root,'
templateUrl: './app.component.html',
})
Export class AppComponent implements OnInit {
data: any;
constructor(private apiService: ApiService) {}
ngOnInit() {
// Fetch data on initialization
this.apiService.getData().subscribe(response => {
this.data = response;
});
}
// Method to post data
submitData(newData: any) {
this.apiService.postData(newData).subscribe(response => {
console.log('Data submitted:', response);
});
}
}
Update the Component Template (e.g., app.component.html):
<div *ngIf="data">
<h3>Fetched Data:</h3>
<pre>{{ data | json }}</pre>
</div>
Implement Error Handling in your service using catchError:
import { catch error } from 'rxjs/operators';
import { throwError } from 'rxjs';
getData(): Observable<any> {
return this.http.get<any>(this.apiUrl).pipe(
catchError(error => {
console.error('Error occurred:', error);
return throwError(error);
})
);
}
Interceptors in Angular provide a powerful way to manage and manipulate HTTP requests and responses globally. By using interceptors, you can apply consistent behavior across your application without modifying each API call. This section will guide you through the process of creating and implementing an interceptor.
Interceptors are services that implement the HttpInterceptor interface, allowing you to intercept and transform HTTP requests and responses. They can be used for various purposes, including:
Generate the Interceptor: Use Angular CLI to create a new interceptor:
Ng generates interceptor auth
Implement the Interceptor: Open the generated auth.interceptor.ts file and implement the HttpInterceptor interface.
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable()
Export class AuthInterceptor implements HttpInterceptor {
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
// Clone the request to add the new header
const clonedRequest = request.clone({
set header: {
Authorization: `Bearer your-token-here` // Add your token or other headers
}
});
// Log the request for debugging
console.log('HTTP Request:', clonedRequest);
// Pass the cloned request instead of the original request to the next handle
return next.handle(clonedRequest);
}
}
Register the Interceptor: To make the interceptor active, you need to register it in your app module. Open app.module.ts and add it to the provider's array:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http';
import { AppComponent } from './app.component';
import { AuthInterceptor } from './auth.interceptor'; // Import the interceptor
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [
{
provide HTTP_INTERCEPTORS,
Use class: AuthInterceptor,
Multi: true // Allow multiple interceptors
}
],
bootstrap: [AppComponent]
})
export class AppModule { }
Using the HttpClient library in Angular offers several advantages that enhance both development efficiency and application performance. Here are some key benefits:
By leveraging these benefits, developers can create robust, maintainable, and efficient Angular applications that interact seamlessly with backend services.
To use the HttpClient library in your Angular application, you need to import and configure it properly. Here’s how to do that step by step:
Import HttpClientModule from @angular/common/http:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { HttpClientModule } from '@angular/common/http'; // Import HttpClientModule
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule // Add HttpClientModule here
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
1. Creating an API Service:
Ng generates service API
2. Implementing the Service (api.service.ts):
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
provided: 'root,'
})
export class ApiService {
private apiUrl = 'https://api.example.com/data'; // Replace with your API URL
constructor(private http: HttpClient) {}
// Example GET request
getData(): Observable<any> {
return this.http.get<any>(this.apiUrl);
}
// Example POST request
postData(data: any): Observable<any> {
const headers = new HttpHeaders({ 'Content-Type': 'application/json' }); // Optional: Set headers
return this.http.post<any>(this.apiUrl, data, { headers });
}
}
3. Using the Service in a Component:
import { Component, OnInit } from '@angular/core';
import { ApiService } from './api.service';
@Component({
selector: 'app-root,'
templateUrl: './app.component.html',
})
Export class AppComponent implements OnInit {
data: any;
constructor(private apiService: ApiService) {}
ngOnInit() {
// Fetch data on initialization
this.apiService.getData().subscribe(response => {
this.data = response;
});
}
}
In Angular, both HttpClient and HttpBackend are part of the @angular/common/http package, but they serve different purposes. HttpClient is the primary interface for making HTTP requests, while HttpBackend provides a lower-level API that can be used for custom HTTP implementations. The following table outlines the key differences between the two:
One common mistake when making API calls in Angular is not unsubscribing from Observables. When you subscribe to an Observable returned by HttpClient, it creates a subscription that can remain active even after the component is destroyed. This can lead to memory leaks and unexpected behavior in your application.
import { Component, OnDestroy } from '@angular/core';
import { Subject } from 'rxjs';
Import { take until } from 'rxjs/operators';
@Component({
selector: 'app-my-component,'
templateUrl: './my-component.component.html',
})
Export class MyComponent implements OnDestroy {
private unsubscribe$ = new Subject<void>();
constructor(private apiService: ApiService) {}
ngOnInit() {
this.apiService.getData()
.pipe(takeUntil(this.unsubscribe$))
.subscribe(response => {
// Handle response
});
}
ngOnDestroy() {
this.unsubscribe$.next();
this.unsubscribe$.complete();
}
}
<div *ngIf="data$ | async as data">
<pre>{{ data | json }}</pre>
</div>
By being mindful of subscriptions and their lifecycle, you can prevent memory leaks and ensure your Angular applications run efficiently.
Enhancing API calls in Angular applications can lead to improved performance, maintainability, and user experience. Here are some practical tips to consider:
HTTP interceptors are a powerful feature in Angular that allows you to intercept and modify HTTP requests and responses globally. By implementing interceptors, you can add common functionality such as logging, adding authorization headers, or handling global error responses in one central location.
This reduces code duplication across your application and ensures consistent behavior for all API calls. For example, you can easily implement a refresh token mechanism or show loading indicators during requests without modifying each service.
Caching API responses can significantly improve the performance of your Angular application by reducing the number of network requests made to the server. By storing previously fetched data in memory or local storage, you can serve repeated requests from the cache rather than making new calls.
This not only speeds up data retrieval but also decreases the load on your server. Implementing caching can be done using libraries like ngrx/store or even simple in-memory objects, allowing you to decide how long to keep data before refreshing it.
Using the async pipe in Angular templates is a best practice for managing subscriptions to Observables. The async pipe automatically subscribes to an Observable when the component is initialized and unsubscribes when the component is destroyed, eliminating the need for manual subscription handling.
This not only reduces boilerplate code but also helps prevent memory leaks, making your application more efficient and easier to maintain. It is especially useful when displaying data from API calls directly in the template.
Implementing proper error handling in your API calls is crucial for providing a good user experience. By using RxJS operators like catchError, you can manage error responses from your API calls effectively.
This allows you to display user-friendly error messages or take alternative actions when an API call fails rather than failing silently. By handling errors gracefully, you can guide users through issues and enhance the overall robustness of your application.
Defining interfaces for the data structures returned by your API enhances type safety in your Angular application. By using TypeScript interfaces, you can ensure that your components and services interact with the correct data shapes, which reduces runtime errors and improves code readability.
This practice makes it easier to refactor and maintain your code, as TypeScript can provide helpful hints and catch potential issues during development.
Minimizing the amount of data sent over the network is crucial for improving application performance. Techniques like pagination, filtering, and selecting only necessary fields can help reduce the payload size of API responses.
By requesting only the data you need, you not only enhance the speed of data retrieval but also improve the overall efficiency of your application, especially when working with large datasets.
Batching multiple API requests into a single request can significantly improve performance by reducing the number of HTTP requests made. When you can combine requests, such as fetching related resources in one go, you decrease the latency involved in multiple round trips to the server.
This is particularly useful in scenarios where you need data that can be fetched together, and it helps streamline your application's data retrieval process.
Reactive programming with RxJS allows you to handle asynchronous data streams more effectively. By utilizing various RxJS operators, you can transform, combine, and manipulate data flows seamlessly.
This makes your code cleaner and easier to manage, especially when dealing with complex asynchronous workflows. Reactive programming enables better handling of events, such as user interactions or data updates, leading to a more responsive application.
Monitoring the performance of your API calls is essential for identifying bottlenecks in your application. By using Angular’s built-in profiling tools or external monitoring services, you can gather data on response times and the frequency of API calls.
This insight allows you to make informed decisions on where to optimize your application, ensuring a smoother user experience and helping you address issues before they affect users.
Centralizing API calls within dedicated services promotes better organization and reusability in your Angular application. By following the service-oriented architecture, you isolate the logic for making HTTP requests, making it easier to maintain and test.
This practice reduces code duplication, as multiple components can reuse the same service for API interactions, and it helps keep your components focused on presentation and behavior rather than data-fetching logic. By implementing these strategies, you can significantly enhance the performance, maintainability, and user experience of your Angular applications, ensuring efficient and effective API interactions.
API calls are crucial in Angular applications for several reasons, playing a key role in how these applications interact with backend services and manage data. Here are some of the primary reasons why API calls are important:
API calls enable Angular applications to retrieve, send, update, and delete data from a server. This interaction is essential for dynamic applications that require up-to-date information, such as user profiles, product listings, or real-time data feeds. Without API calls, applications would be static and unable to interact with external data sources.
By using APIs, Angular applications can maintain a clear separation between the front end and back end. This separation allows developers to focus on building user interfaces and user experiences while relying on backend services for data management. This architecture promotes cleaner code and easier maintenance.
APIs facilitate scalability by allowing the front end and back end to evolve independently. As applications grow, you can update the backend logic or database structure without needing to overhaul the front end. This flexibility supports long-term development and scaling efforts.
API calls enable features like real-time updates, data validation, and user interactions that enhance user experience. For example, users can see live notifications or changes without refreshing the page, leading to a more interactive and engaging application.
APIs allow Angular applications to integrate with various third-party services, such as payment gateways, social media platforms, or data analytics tools. This integration can enhance functionality and provide users with additional features without requiring extensive custom development.
API calls often include security measures such as token-based authentication, which helps protect sensitive data and ensure that only authorized users can access certain resources. This security layer is essential for building trustworthy applications.
API calls are essential to the success of Angular applications, serving as the backbone for dynamic data interactions and seamless user experiences. By enabling efficient data retrieval, manipulation, and integration with backend services, API calls facilitate a clear separation of concerns that promotes maintainable and scalable architectures. They enhance user engagement through real-time updates and interactions while providing robust security measures.
Furthermore, the modular nature of APIs simplifies testing and debugging, allowing for continuous improvement and adaptation to changing requirements. As modern web applications increasingly rely on real-time data and third-party integrations, understanding and effectively implementing API calls is crucial for developers aiming to build responsive, reliable, and feature-rich Angular applications.
Copy and paste below code to page Head section
An API call is a request made from a client (like an Angular application) to a server to retrieve or send data. It typically uses HTTP methods such as GET, POST, PUT, and DELETE.
HttpClient is the recommended way to make HTTP requests in Angular because it provides a simpler API, supports RxJS Observables for handling asynchronous data, and includes features like automatic JSON parsing and error handling.
You can handle errors using the catchError operator from RxJS within your service methods. This allows you to manage error responses gracefully and provide user-friendly feedback.
Interceptors are a powerful feature that allows you to intercept HTTP requests and responses globally. They can be used for tasks such as adding authentication tokens, logging requests, or handling error responses in a centralized manner.
You can cancel an ongoing API request by unsubscribing from the Observable returned by HttpClient. Alternatively, you can use the take-until operator with a Subject to manage subscriptions based on the component’s lifecycle.
Yes, you can use async/await with API calls in Angular by converting the Observable returned by HttpClient into a Promise using the toPromise() method. However, it’s generally recommended to stick with Observables for better integration with Angular’s reactive programming model.