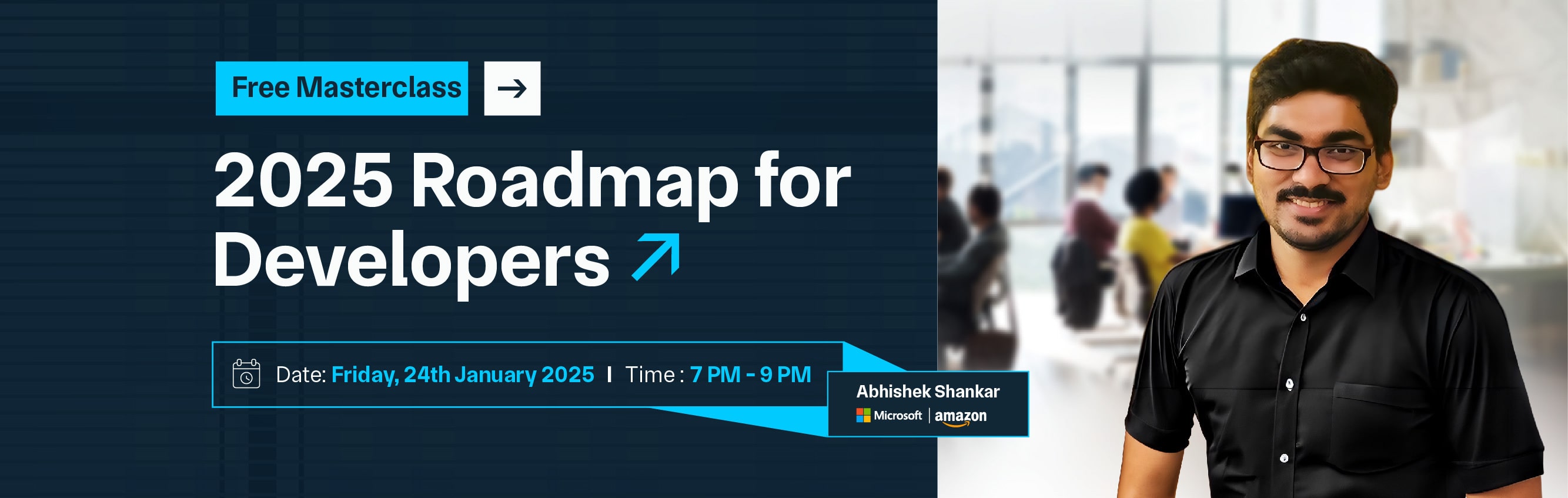

React Router is an essential library for managing navigation and routing in React applications. It allows developers to build single-page applications that offer multiple views and URLs, ensuring a seamless user experience by synchronising the application's UI state with the URL. To integrate React Router into your project, start by installing it via npm or yarn. Once installed, you can define routes using <Route> components within a <BrowserRouter> or <HashRouter>, depending on your routing needs.
Each <Route> specifies a path and the component to render when that path matches the current URL. For instance, you might define routes for a home page, an about page, and a 404 error page using <Route> components nested inside a <Switch> component to ensure only one route matches at a time. React Router also supports features like nested routes, route parameters, redirects, and programmatic navigation through hooks like useHistory and useParams, providing flexibility and power in managing navigation flows within your React application.
React Router simplifies navigation and state management in React apps, offering nested routes, route parameters, redirects, and hooks like useHistory for seamless user experiences.Overall, React Router simplifies the process of implementing complex routing logic, making it indispensable for creating dynamic and user-friendly web applications.
React Router is a library for React applications that enables declarative routing and navigation. It allows developers to define how different components should render based on the browser's URL. React Router helps create single-page applications with multiple views and URLs, managing the application's UI state in sync with the URL.
This library provides components like <BrowserRouter>, <Route>, <Switch>, and hooks like useHistory and useParams to facilitate dynamic routing, parameter handling, and navigation control within React applications. Overall, React Router simplifies the implementation of complex routing logic, making it easier to build interactive and responsive web applications using React.
A simple example of how React Router can be used in a React application:
First, you need to install React Router DOM:
npm install react-router-dom
Then, you can create a basic application with routing:
// App.js
import React from 'react';
import { BrowserRouter as Router, Route, Switch, Link } from 'react-router-dom';
import Home from './components/Home';
import About from './components/About';
import Contact from './components/Contact';
import NotFound from './components/NotFound';
const App = () => {
return (
<Router>
<div>
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
<li><Link to="/contact">Contact</Link></li>
</ul>
</nav>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route component={NotFound} />
</Switch>
</div>
</Router>
);
}
export default App;
In this example:
For instance, if the user navigates to /about, the About component will be rendered. React Router manages the rendering of components based on the current URL, providing a seamless navigation experience in single-page applications built with React.
Using React Router in your React application enables seamless navigation and routing, which is essential for creating dynamic single-page applications. Start by installing React Router DOM via npm (npm install react-router-dom).
Then, wrap your application with a <BrowserRouter> or <HashRouter> component to manage routing context. Define routes using <Route> components to map specific paths to corresponding components. Use <Link> components for navigation, ensuring smooth transitions between views without full page reloads.
React Router supports advanced features like nested routes, route parameters, redirects, and hooks (useHistory, useParams), empowering developers to create intuitive and responsive web experiences. React Router involves several steps to set up routing and navigation within your React application:
React Router DOM is the version of React Router designed for web applications. To install it, use npm (Node Package Manager) in your terminal:
bash
npm install react-router-dom
This command installs React Router DOM and its dependencies, making it available for use in your project.
After installing React Router DOM, you need to wrap your application with a router component (<BrowserRouter> or <HashRouter>). This component provides the routing context for your application:
import { BrowserRouter as Router } from 'react-router-dom';
function App() {
return (
<Router>
{/* Your application content here */}
</Router>
);
}
export default App;
1. <BrowserRouter>: Uses HTML5 history API for routing, suitable for modern browsers.
2. <HashRouter>: Uses the hash portion (#) of the URL for routing, suitable for environments where server-side rendering is not available or difficult to configure.
Choose <BrowserRouter> for most web applications unless you have specific requirements for <HashRouter>.
Inside the <Router> component, define your routes using <Route> components. Each <Route> specifies a path and the component to render when that path matches the current URL:
import { Route, Switch } from 'react-router-dom';
import Home from './components/Home';
import About from './components/About';
import Contact from './components/Contact';
import NotFound from './components/NotFound';
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route component={NotFound} /> {/* This serves as a 404 page */}
</Switch>
</Router>
);
}
export default App;
Use exact with <Route> to ensure that the path matches exactly.
<Switch> ensures that only the first matching <Route> is rendered. It helps in rendering a "404 Not Found" page when no other routes match.
Use <Link> components from React Router DOM instead of traditional anchor (<a>) tags for navigation between different routes. This prevents full page reloads and provides a smoother user experience:
import { Link } from 'react-router-dom';
function Navigation() {
return (
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
<li><Link to="/contact">Contact</Link></li>
</ul>
</nav>
);
}
The to prop in <Link> specifies the target URL path.
React Router offers additional features for more complex routing scenarios:
By following these steps and utilizing these features, you can effectively integrate React Router into your React application, enabling seamless navigation and state management based on the URL structure. This setup is crucial for building modern, single-page applications that provide a fluid and intuitive user experience.
React Router DOM provides essential components for implementing navigation and routing in React applications. These components include <BrowserRouter> for modern browser routing using HTML5 history, <HashRouter> for environments not supporting HTML5 history, <Route> for rendering UI based on URL paths, <Switch> for rendering the first matching route, <Link> for declarative navigation without full page reloads, and <Redirect> for programmatically directing users to different routes.
Together, these components enable developers to create dynamic single-page applications with seamless navigation, clean URLs, and optimised user experiences by synchronising the application state with the URL.
The <BrowserRouter> component uses the HTML5 history API to synchronise the UI with the URL in modern web browsers. It allows for declarative routing in React applications, enabling navigation between different views without full page reloads.
This component should wrap your entire application to provide the routing context, ensuring that changes in the URL update the displayed components accordingly. It's ideal for applications deployed to servers that support HTML5 history API, offering a more intuitive and seamless user experience by maintaining the application state across navigation actions.
The <HashRouter> component uses the hash portion (#) of the URL to keep the UI in sync with the URL. It's suitable for environments where server-side rendering is not available or when dealing with older browsers that don't fully support the HTML5 history API.
This component wraps your application and provides routing capabilities by using the hash part of the URL to simulate different pages or states within a single HTML file. While it's simpler to set up, it may provide a different clean URLs and user experience than <BrowserRouter> in modern applications.
The <Route> component is used to render some UI when the location matches the route's path. It's a fundamental building block of React Router DOM, defining which component should be rendered based on the current URL.
The path prop specifies the URL path that the route matches, and the component prop specifies the React component to render when the path matches. Optionally, you can use exact to ensure the path matches exactly. <Route> components can be nested and used within <Switch> to control which components are rendered based on the current location.
The <Switch> component is used to render only the first <Route> or <Redirect> that matches the current location. It renders the first child <Route> or <Redirect> that matches the location without rendering any subsequent routes. This behavior ensures that only one route is matched and rendered at a time, making it useful for handling 404 pages or providing fallback routes.
By wrapping multiple <Route> components inside <Switch>, you can define different routes and control which component is displayed based on the URL path, optimizing performance and ensuring predictable routing behavior.
The <Link> component is used to create navigation links in React applications. It's similar to the <a> tag in HTML but prevents the browser from performing a full page reload when clicked. Instead, it uses the React Router DOM's internal history API to update the URL, ensuring a smooth and fast navigation experience.
The to prop specifies the target URL path or location descriptor object that the link should navigate to. By using <Link> components, you can create navigation menus, buttons, or any other clickable elements that seamlessly transition users between different routes within your single-page application.
The <Redirect> component is used to redirect users to another location programmatically. It renders a new location in the browser's history stack, replacing the current location with the new one. This component is typically used inside <Switch> components or within conditional rendering logic to direct users to a different route based on certain conditions or authentication states.
The to prop specifies the target URL path or location descriptor object that the user should be redirected to. <Redirect> helps manage navigation flows and ensures users are directed to the correct route or page as per the application's logic.
Implementing React Router in your React application allows you to manage navigation and routing seamlessly. Start by installing React Router DOM via npm (npm install react-router-dom). Wrap your application in a <BrowserRouter> or <HashRouter> component to establish routing context.
Define routes using <Route> components to map URL paths to specific components. Use <Link> components for navigation, ensuring smooth transitions between views without full page reloads.
React Router also offers advanced features like nested routes, route parameters, redirects, and hooks (useHistory, useParams), providing flexibility for complex navigation scenarios. This integration enhances user experience by synchronizing UI state with the URL structure effectively.
Begin by installing React Router DOM, which is tailored for web applications:
bash
npm install react-router-dom
This command installs React Router DOM and its dependencies.
Wrap your application with a <BrowserRouter> or <HashRouter> component to provide the routing context:
import { BrowserRouter as Router } from 'react-router-dom';
function App() {
return (
<Router>
{/* Your application content here */}
</Router>
);
}
export default App;
1. <BrowserRouter> uses the HTML5 history API for cleaner URLs.
2. <HashRouter> uses the hash portion (#) of the URL for older browser support.
Inside the <Router> component, define routes using <Route> components. Each <Route> specifies a path and the component to render when that path matches the current URL:
import { Route, Switch } from 'react-router-dom';
import Home from './components/Home';
import About from './components/About';
import Contact from './components/Contact';
import NotFound from './components/NotFound';
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route component={NotFound} /> {/* 404 page */}
</Switch>
</Router>
);
}
export default App;
<Switch> ensures only one route is rendered at a time.
Use exact with <Route> to match paths exactly.
Link Navigation:
Use <Link> components for navigation between routes, preventing full page reloads:
import { Link } from 'react-router-dom';
function Navigation() {
return (
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
<li><Link to="/contact">Contact</Link></li>
</ul>
</nav>
);
}
React Router supports nested routes, route parameters (: param), redirects (<Redirect>), and programmatic navigation via history manipulation or hooks (useHistory, useParams). These features enhance routing flexibility and user interaction in your application.
By following these steps, you can effectively integrate React Router into your React application, enabling seamless navigation and state management based on URL changes. This setup supports building modern single-page applications with clear, manageable routing and enhanced user experience.
React Router is essential in React applications primarily because React itself is designed for building single-page applications (SPAs). Unlike traditional multi-page applications where each page corresponds to a separate HTML file loaded from the server, SPAs load a single HTML page and dynamically update the content as the user interacts with the application. Here’s why React Router is necessary:
React Router simplifies the process of managing navigation and state in React applications, making it a fundamental tool for building modern, responsive, and user-friendly SPAs. It enhances the development experience by providing a structured way to handle routing complexities while maintaining optimal performance and user experience.
React Router's flexibility and features cater to diverse application needs, from simple SPAs to complex, data-driven interfaces, making it an essential tool for modern web development.
React Router offers several advantages that make it a preferred choice for managing navigation and routing in React applications:
1. Declarative Routing: React Router allows developers to define routes and their corresponding components using JSX syntax, providing a declarative way to manage application navigation.
2. Single-Page Application (SPA) Support: Facilitates the creation of SPAs where content updates dynamically based on URL changes, enhancing user experience by eliminating full-page reloads.
3. Nested Routing: Supports nested routes and layouts, enabling developers to create complex UI structures and manage application complexity more efficiently.
4. Dynamic Routing: Enables dynamic routing based on data fetched from APIs or user interactions, allowing for flexible and personalised content rendering.
5. Code Splitting and Lazy Loading: Integrates seamlessly with code splitting techniques, loading only necessary components when navigating to different routes, which improves initial load times and overall performance.
6. History Management: Handles browser history and location state, enabling features like back and forward navigation through browser buttons and programmatic navigation using hooks like useHistory.
7. SEO-Friendly: Supports server-side rendering (SSR) for improved search engine optimisation (SEO), ensuring that search engines can crawl and index content effectively.
While React Router is a powerful tool for managing navigation in React applications, it does have some limitations and considerations:
React Router stands as a foundational library for managing navigation and routing within React applications, offering developers powerful tools to create dynamic and engaging user experiences. Despite its strengths, such as declarative routing, support for single-page applications (SPAs), and extensive community support, React Router does come with considerations. Navigating through potential limitations such as initial learning curves, complexities in server-side rendering (SSR) setup, and route management challenges underscores the importance of thoughtful implementation and ongoing optimisation.
Addressing these aspects ensures smooth application performance, efficient SEO integration, and seamless user interactions across different devices and browsers. Ultimately, React Router remains a versatile and indispensable tool in the React ecosystem, enabling developers to architect sophisticated web applications with structured navigation, enhanced user experience, and scalability. By leveraging its capabilities and understanding its limitations, developers can harness React Router to its fullest potential, delivering robust and responsive applications tailored to modern web standards and user expectations.
Copy and paste below code to page Head section
React Router is a library that provides navigation and routing functionalities for React applications. It allows you to manage the application's URL and map them to different components, enabling seamless transitions between views without reloading the entire page.
You can install React Router DOM, the version of React Router tailored for web applications, using npm: npm install react-router-dom This command installs React Router DOM and its dependencies.
React Router DOM includes components such as <BrowserRouter>, <Route>, <Switch>, <Link>, <Redirect>, and <NavLink>. These components facilitate declarative routing, nested routes, dynamic rendering, and navigation within your React application.
<BrowserRouter> uses the HTML5 history API for cleaner URLs. It's suitable for modern browsers and server environments that support history management. <HashRouter> uses the hash portion (#) of the URL for routing. It's useful for environments without proper server-side support for single-page applications (SPAs) and older browsers.
Routes are defined using the <Route> component within a <Switch> component. Each <Route> specifies a path and the component to render when that path matches the current URL. Use the exact prop to match paths exactly and prevent unintended rendering.
Navigation between routes is achieved using the <Link> component, which generates anchor tags (<a>) to navigate between different routes within the application. This prevents full page reloads, providing a smoother user experience.