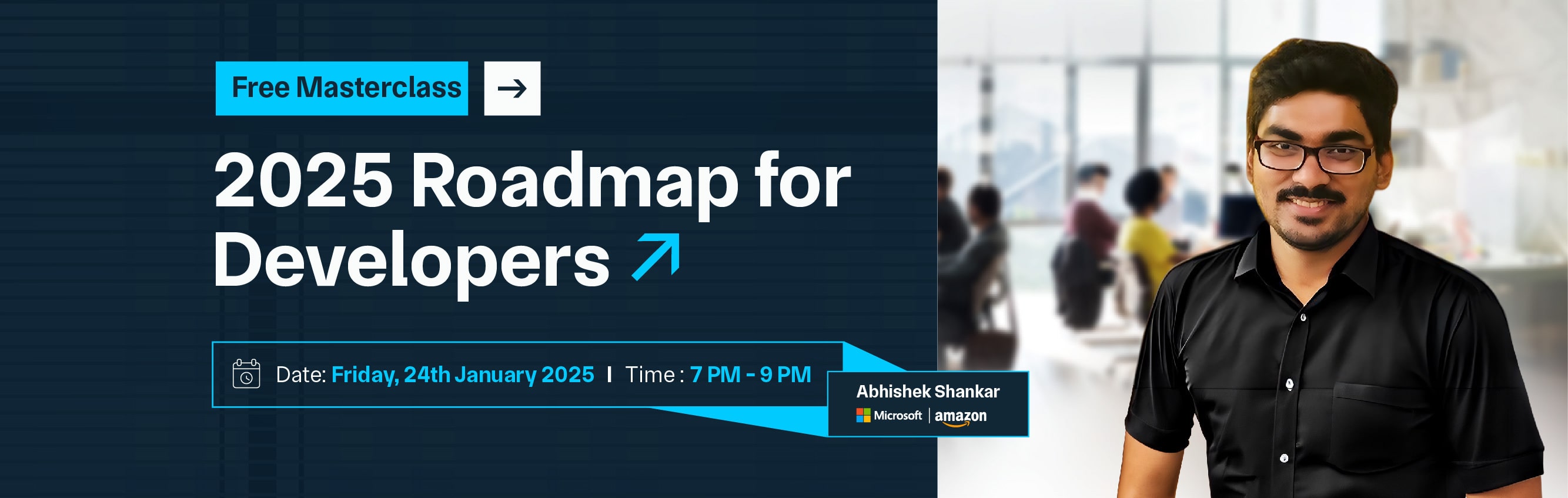

Interceptors in Angular are a powerful feature of the HttpClientModule that allows you to intercept HTTP requests and responses globally or on a per-request basis. They provide a centralized way to handle tasks like logging, adding headers, modifying requests, or handling errors consistently across your application. To implement an interceptor, you first create a service that implements the HttpInterceptor interface.
This involves defining the intercept method where you can inspect and optionally modify outgoing requests before passing them along the chain using next. handle(req). Similarly, you can intercept incoming responses and handle errors within the tap operator. Once implemented, you need to provide the interceptor in your Angular application by including it in the provider array of your module. Angular allows you to specify multiple interceptors and control their execution order using the multi-property.
Interceptors are invaluable for tasks such as authentication token management, request/response logging, caching, or even transforming data. They promote cleaner code by separating concerns and ensuring consistent behaviour across HTTP requests, making them a fundamental tool for building robust and maintainable Angular applications. Integrating interceptors enhances both the security and reliability of your application's API interactions.
Angular is a popular open-source web application framework maintained by Google and a community of developers. It is used to build single-page client-side applications. Angular is written in TypeScript and follows a component-based architecture.
Angular has a strong ecosystem with tools like Angular CLI for scaffolding projects, Angular Universal for server-side rendering, and Angular Material for pre-designed UI components. It's widely adopted for building enterprise-scale applications due to its robust features, strong community support, and continuous updates from Google.
An Angular HTTP interceptor is a middleware mechanism provided by Angular's HttpClientModule that allows you to intercept HTTP requests and responses globally or on a per-request basis. Interceptors provide a way to modify or transform HTTP requests before they are sent to the server and to manipulate responses before they reach the application.
Key Features and Use Cases of HTTP Interceptors
Implementing an HTTP interceptor in Angular involves a few practical steps. Let's go through a detailed guide on how to implement and use an interceptor effectively:
First, generate a new Angular service for your interceptor. You can use Angular CLI to create the service:
ng generate service interceptors/http-interceptor
This will create a service file named http-interceptor.service.ts (or similar) in your src/app/interceptors/ directory.
Open the generated interceptor service (HTTP-interceptor.service.ts) and implement the HttpInterceptor interface from @angular/common/http. This interface requires you to implement the intercept method, where you can intercept and modify outgoing requests and incoming responses.
Here’s an example of a simple interceptor that logs request and response information:
import { Injectable } from '@angular/core';
import {
HttpInterceptor,
HttpRequest,
HttpHandler,
HttpEvent,
HttpResponse,
HttpErrorResponse
} from '@angular/common/http';
import { Observable, throwError } from 'rxjs';
import { catchError, tap } from 'rxjs/operators';
@Injectable()
export class HttpInterceptorService implements HttpInterceptor {
intercept(
req: HttpRequest<any>,
next: HttpHandler
): Observable<HttpEvent<any>> {
console.log('Outgoing request');
console.log(req); // Log the request
// Optionally modify the request
// const modifiedReq = req.clone({ headers: req.headers.set(...) });
// Pass the modified request to the next interceptor or HttpClient
return next.handle(req).pipe(
tap(
event => {
if (event instanceof HttpResponse) {
console.log('Incoming response');
console.log(event); // Log the response
}
},
error => {
if (error instanceof HttpErrorResponse) {
console.error('Error occurred:', error.error.message);
} else {
console.error('Unknown error occurred');
}
}
)
);
}
}
Next, you need to provide your interceptor in the Angular application by adding it to the provider array of your module. This typically happens in your AppModule (located in src/app/app.module.ts).
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http';
import { HttpInterceptorService } from './interceptors/http-interceptor.service'; // Adjust the path as needed
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: HttpInterceptorService, multi: true }
],
bootstrap: [AppComponent]
})
export class AppModule { }
If you have multiple interceptors, you can specify them in the provider's array with the multi-property to control their execution order:
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: Interceptor1, multi: true },
{ provide: HTTP_INTERCEPTORS, useClass: Interceptor2, multi: true }
]
Once your interceptor is set up, test it by making HTTP requests in your application. Open the browser's developer console to view the logged request and response information.
Interceptors are valuable for various tasks, such as:
HTTP interceptors in Angular offer several powerful features that enhance the flexibility, maintainability, and security of your applications. Here are the key features of HTTP interceptors:
1. Global Handling: Interceptors allow you to apply common behaviors or modifications to all HTTP requests or responses across your application. This ensures consistent handling of tasks such as logging, error handling, or adding headers.
2. Request Modification: Interceptors can modify outgoing requests before they are sent to the server. This includes adding authentication tokens, custom headers, or modifying the request body based on application requirements.
3. Response Modification: They can intercept and modify incoming responses from the server before passing them to the application code. This allows transformations of response data, handling of error responses, or enforcing common data formats.
4. Error Handling: Interceptors provide a central place to handle HTTP errors. You can intercept error responses, retry requests on failure, or transform error payloads for consistent error handling across your application.
5. Logging and Debugging: They enable logging of HTTP requests and responses for debugging purposes. This helps developers trace the flow of HTTP interactions, inspect request details like headers and payloads, and log response data or errors.
6. Authentication: Interceptors are commonly used for managing authentication flows. They can automatically add authentication tokens or headers to outgoing requests, ensuring secure communication with backend services without manually adding tokens in every request.
7. Caching: Implementing caching mechanisms within interceptors allows you to cache HTTP responses based on certain conditions. This optimizes performance by reducing the number of network requests and improving application responsiveness.
8. Testing and Mocking: Interceptors facilitate easier testing by abstracting HTTP-related logic into separate services. This allows for easier mocking of HTTP interactions during unit tests, ensuring isolated and predictable test scenarios.
9. Request Transformation: They support transforming requests based on application needs, such as converting request payloads to a different format or adding metadata before sending requests to the server.
10. Performance Optimization: By intercepting and optimizing HTTP requests and responses, interceptors can contribute to improving application performance, reducing latency, and optimizing bandwidth usage.
In summary, Angular HTTP interceptors are a versatile toolset that empowers developers to implement cross-cutting concerns, enforce consistent behaviors, and enhance the overall reliability and performance of HTTP communication within Angular applications. Their modular and reusable nature promotes cleaner code architecture and facilitates easier maintenance and scaling of Angular projects.
HTTP interceptors in Angular work by intercepting HTTP requests and responses made by the Angular HttpClient. They operate as middleware, allowing you to apply common logic or modifications to these requests and responses across your application.
1. Interception Point: When an HTTP request is made from your Angular application using the HttpClient, it passes through the registered interceptors before reaching the server.
2. Intercept Method: Each interceptor implements the HttpInterceptor interface, which requires the implementation of the intercept method. This method takes two parameters:
3. Request Modification: Inside the intercept method, you can modify the req object:
4. Chain of Responsibility: After processing the request within the interceptor, you call next.handle(req) to pass the modified request to the next interceptor in the chain or to the HttpClient if there are no more interceptors.
5. Response Handling: Once the request is sent to the server and a response is received:
1. The response travels back through the interceptor chain in reverse order.
2. Each interceptor can intercept and modify the response:
6. Completion: The final response (or error) is eventually returned to the application code that initiated the HTTP request.
Consider an interceptor that adds an authorization token to every outgoing request:
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
// Add authorization token to the request
const authToken = 'Bearer ' + localStorage.getItem('token');
const authReq = req.clone({
setHeaders: {
Authorization: authToken
}
});
// Log the modified request
console.log('Modified Request:');
console.log(authReq);
// Pass the request to the next interceptor or HttpClient
return next.handle(authReq).pipe(
tap(
event => {
if (event instanceof HttpResponse) {
// Log the response if needed
console.log('Response Received:');
console.log(event);
}
},
error => {
// Handle errors if any
console.error('Error occurred:', error);
}
)
);
}
}
In conclusion, Angular HTTP interceptors provide a powerful mechanism to extend and customize HTTP requests and responses, offering flexibility and consistency in managing network communications within your Angular applications.
Creating a simple HTTP interceptor in Angular involves several steps, from setting up the interceptor service to registering it in your application. Below is a step-by-step guide to create a basic HTTP interceptor:
First, generate a new Angular service for your interceptor using Angular CLI:
ng generate service interceptors/HTTP-interceptor
This command will create a service file named http-interceptor.service.ts (or similar) in the src/app/interceptors/ directory.
Open the generated interceptor service (HTTP-interceptor.service.ts) and implement the HttpInterceptor interface from @angular/common/HTTP. This interface requires you to implement the intercept method, where you can intercept and modify outgoing requests and incoming responses.
Here’s a basic example of an interceptor that logs request and response information:
import { Injectable } from '@angular/core';
import {
HttpInterceptor,
HttpRequest,
HttpHandler,
HttpEvent,
HttpResponse,
HttpErrorResponse
} from '@angular/common/http';
import { Observable } from 'rxjs';
import { tap } from 'rxjs/operators';
@Injectable()
export class HttpInterceptorService implements HttpInterceptor {
intercept(
req: HttpRequest<any>,
next: HttpHandler
): Observable<HttpEvent<any>> {
console.log('Outgoing request');
console.log(req); // Log the request
// Pass the request to the next handler
return next.handle(req).pipe(
tap(
event => {
if (event instanceof HttpResponse) {
console.log('Incoming response');
console.log(event); // Log the response
}
},
error => {
if (error instanceof HttpErrorResponse) {
console.error('Error occurred:', error.error.message);
} else {
console.error('Unknown error occurred');
}
}
)
);
}
}
Next, you need to provide your interceptor in the Angular application by adding it to the provider array of your module. Typically, you'll register interceptors in your AppModule (located in src/app/app.module.ts).
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http';
import { HttpInterceptorService } from './interceptors/http-interceptor.service'; // Adjust the path as needed
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: HttpInterceptorService, multi: true }
],
bootstrap: [AppComponent]
})
export class AppModule { }
Once your interceptor is set up, you can test it by making HTTP requests in your Angular application. Open the browser's developer console (usually accessed via F12 or right-clicking and selecting "Inspect") to view the logged request and response information.
Interceptors can be used for a variety of tasks, such as
HTTP interceptors in Angular operate by intercepting HTTP requests and responses at specific points during their lifecycle. Here’s a detailed look at the operations of HTTP interceptors:
1. Interception Point: When an HTTP request is initiated from an Angular application using HttpClient, it first passes through any registered HTTP interceptors.
2. Intercept Method: Each interceptor implements the HttpInterceptor interface, which mandates the implementation of the intercept method. This method takes two parameters:
3. Request Modification: Inside the intercept method, you can:
4. Passing to Next Interceptor: After modifying the request as needed, you call next.handle(req) to pass the modified request to the next interceptor in the chain or to the HttpClient if it’s the last interceptor.
1. Response Flow: Once the modified request is sent to the server, it processes the request and generates a response.
2. Intercepting Responses: After the server sends a response:
3. Response Handling: Within the intercept method, you can:
1. Error Interception: Interceptors also handle errors that occur during the HTTP request lifecycle:
Here’s a simplified example of an HTTP interceptor that logs request and response details:
import { Injectable } from '@angular/core';
import {
HttpInterceptor,
HttpRequest,
HttpHandler,
HttpEvent,
HttpResponse,
HttpErrorResponse
} from '@angular/common/http';
import { Observable } from 'rxjs';
import { tap } from 'rxjs/operators';
@Injectable()
export class HttpInterceptorService implements HttpInterceptor {
intercept(
req: HttpRequest<any>,
next: HttpHandler
): Observable<HttpEvent<any>> {
console.log('Outgoing request');
console.log(req); // Log the request
// Pass the request to the next handler
return next.handle(req).pipe(
tap(
event => {
if (event instanceof HttpResponse) {
console.log('Incoming response');
console.log(event); // Log the response
}
},
error => {
if (error instanceof HttpErrorResponse) {
console.error('Error occurred:', error.error.message);
} else {
console.error('Unknown error occurred');
}
}
)
);
}
}
In conclusion, Angular HTTP interceptors are essential tools for managing and enhancing HTTP communication within Angular applications. They enable developers to implement cross-cutting concerns effectively, ensuring robust and scalable web applications.
HTTP interceptors offer several benefits in web development, primarily in enhancing security, managing requests/responses, and improving code maintainability. Here are some key benefits:
HTTP interceptors in Angular are versatile tools that can be used for various purposes to enhance the functionality and maintainability of your application:
While HTTP interceptors provide powerful capabilities, they also come with certain limitations and considerations:
Integrating HTTP interceptors into an Angular application involves setting up the interceptor service, registering it in the application module, and using it to perform tasks like authentication, logging, or error handling across HTTP requests. Here's a step-by-step guide to implementing an Angular application with an HTTP interceptor:
First, generate a new Angular service for your interceptor using Angular CLI:
ng generate service interceptors/http-interceptor
This command will create a service file named http-interceptor.service.ts (or similar) in the src/app/interceptors/ directory.
Open the generated interceptor service (HTTP-interceptor.service.ts) and implement the HttpInterceptor interface from @angular/common/http. This interface requires the implementation of the intercept method, where you can intercept and modify outgoing requests and incoming responses based on your application needs.
Here’s an example of an interceptor that adds an authentication token to every outgoing request:
import { Injectable } from '@angular/core';
import {
HttpInterceptor,
HttpRequest,
HttpHandler,
HttpEvent,
HttpResponse,
HttpErrorResponse
} from '@angular/common/http';
import { Observable, throwError } from 'rxjs';
import { catchError } from 'rxjs/operators';
@Injectable()
export class HttpInterceptorService implements HttpInterceptor {
intercept(
req: HttpRequest<any>,
next: HttpHandler
): Observable<HttpEvent<any>> {
// Get authentication token from local storage or wherever it is stored
const authToken = localStorage.getItem('token');
// Clone the request and add the authorization header
const authReq = req.clone({
setHeaders: {
Authorization: `Bearer ${authToken}`
}
});
// Pass the cloned request to the next interceptor or HttpClient
return next.handle(authReq).pipe(
catchError((error: HttpErrorResponse) => {
// Handle HTTP errors
console.error('Error occurred:', error);
return throwError(error);
})
);
}
}
Next, you need to provide your interceptor in the Angular application by adding it to the provider array of your module. Typically, you'll register interceptors in your AppModule (located in src/app/app.module.ts).
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http';
import { HttpInterceptorService } from './interceptors/http-interceptor.service'; // Adjust the path as needed
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: HttpInterceptorService, multi: true }
],
bootstrap: [AppComponent]
})
export class AppModule { }
Now that the interceptor is registered, you can use Angular's HttpClient service to make HTTP requests as usual in your application components or services. The interceptor will automatically intercept and modify requests before they are sent to the server.
Here’s an example of using HttpClient in a component:
import { Component } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-root',
template: `
<button (click)="getData()">Get Data</button>
<p>{{ responseData }}</p>
`
})
export class AppComponent {
responseData: any;
constructor(private http: HttpClient) {}
getData() {
this.http.get('https://api.example.com/data').subscribe(
(response) => {
this.responseData = response;
},
(error) => {
console.error('Error fetching data:', error);
}
);
}
}
Test your interceptor by making HTTP requests in your application. Open the browser's developer tools to inspect the request headers and any logged information from the interceptor for debugging purposes.
You can implement multiple interceptors for different functionalities (e.g., logging, error handling) by providing them in the provider array with the multi-property set to true. Angular will execute these interceptors in the order they are listed.
HTTP interceptors in Angular provide several advantages that contribute to the robustness, maintainability, and security of applications:
Angular HTTP interceptors are indispensable tools for enhancing the robustness, security, and maintainability of web applications built with Angular. They provide a centralized mechanism to intercept and modify HTTP requests and responses, allowing developers to implement cross-cutting concerns such as authentication, logging, error handling, and request/response transformation in a unified manner. By leveraging HTTP interceptors, developers can enforce consistent behaviours across their applications, ensuring that all HTTP interactions adhere to predefined policies and logic. This promotes code reusability, reduces duplication of effort, and adheres to best practices such as the DRY (Don't Repeat Yourself) principle.
Interceptors also facilitate improved security by enabling the addition of authentication tokens or headers to outgoing requests, thereby securing communication between frontend and backend services. Moreover, interceptors contribute to enhanced debugging capabilities by providing visibility into HTTP traffic. They enable logging of request and response details, aiding in diagnosing issues, monitoring application performance, and gathering analytics data. This transparency is crucial for maintaining and optimizing applications over time. Furthermore, Angular interceptors support flexibility and extensibility, allowing developers to adapt HTTP interactions to specific application requirements. Whether implementing caching mechanisms, retry strategies for failed requests, or custom transformations of data, interceptors offer the flexibility needed to tailor HTTP behaviour according to business needs.
Copy and paste below code to page Head section
Angular HTTP interceptors are middleware that intercepts HTTP requests and responses globally or per a request basis. They allow developers to implement cross-cutting concerns such as authentication, logging, error handling, and request/response transformation in a modular and reusable way.
HTTP interceptors in Angular implement the HttpInterceptor interface, which requires the intercept method. This method intercepts outgoing HTTP requests before they are sent and incoming HTTP responses before they reach the application. Interceptors can modify requests, handle errors, log interactions, add headers, and more.
Yes, Angular allows chaining multiple interceptors. They are executed in the order they are provided in the provider's array of the AppModule. Each interceptor can modify the request or response before passing it to the next interceptor or to the HttpClient.
Angular HTTP interceptors aid in testing by centralizing HTTP-related logic into separate services. This allows easier mocking and testing of HTTP interactions without directly invoking HttpClient calls in tests. Developers can mock HTTP requests/responses, test error handling, and validate request transformations within interceptor tests.
Yes, one of the primary use cases for Angular HTTP interceptors is handling authentication. Interceptors can add authentication tokens or headers to outgoing requests based on user authentication status or other criteria, ensuring secure communication between the frontend and backend services.
Some limitations of Angular HTTP interceptors include Order of Execution: Interceptors execute in the order they are provided, which can affect behaviour if not carefully managed. Performance Impact: Complex logic or multiple interceptors can impact performance, especially with high-frequency HTTP requests. Immutable Requests: Angular HTTP requests are immutable, requiring careful handling when modifying requests within interceptors.